Note
Click here to download the full example code
Reading and writing raw files¶
In this example, we read a raw file. Plot a segment of MEG data restricted to MEG channels. And save these data in a new raw file.
# Author: Alexandre Gramfort <alexandre.gramfort@inria.fr>
#
# License: BSD (3-clause)
import mne
from mne.datasets import sample
print(__doc__)
data_path = sample.data_path()
fname = data_path + '/MEG/sample/sample_audvis_raw.fif'
raw = mne.io.read_raw_fif(fname)
# Set up pick list: MEG + STI 014 - bad channels
want_meg = True
want_eeg = False
want_stim = False
include = ['STI 014']
raw.info['bads'] += ['MEG 2443', 'EEG 053'] # bad channels + 2 more
picks = mne.pick_types(raw.info, meg=want_meg, eeg=want_eeg, stim=want_stim,
include=include, exclude='bads')
some_picks = picks[:5] # take 5 first
start, stop = raw.time_as_index([0, 15]) # read the first 15s of data
data, times = raw[some_picks, start:(stop + 1)]
# save 150s of MEG data in FIF file
raw.save('sample_audvis_meg_trunc_raw.fif', tmin=0, tmax=150, picks=picks,
overwrite=True)
Out:
Opening raw data file /home/circleci/mne_data/MNE-sample-data/MEG/sample/sample_audvis_raw.fif...
Read a total of 3 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Range : 25800 ... 192599 = 42.956 ... 320.670 secs
Ready.
Writing /home/circleci/project/examples/io/sample_audvis_meg_trunc_raw.fif
Closing /home/circleci/project/examples/io/sample_audvis_meg_trunc_raw.fif
[done]
Show MEG data
raw.plot()
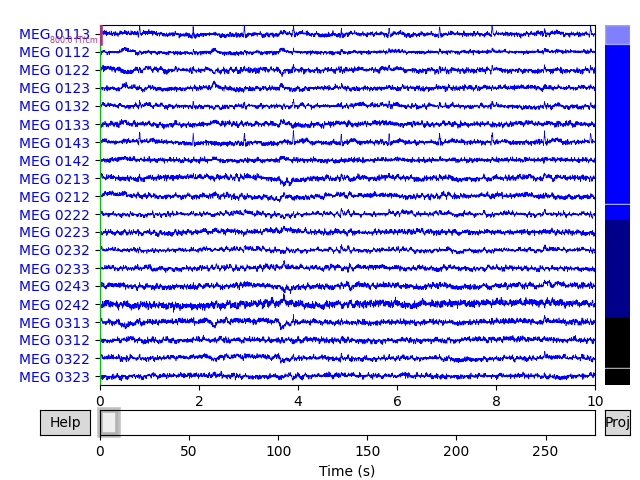
Total running time of the script: ( 0 minutes 2.769 seconds)
Estimated memory usage: 36 MB