Note
Click here to download the full example code or to run this example in your browser via Binder
01. Read BIDS datasets#
When working with electrophysiological data in the BIDS format, an important resource is the OpenNeuro database. OpenNeuro works great with MNE-BIDS because every dataset must pass a validator that tests to ensure its format meets BIDS specifications before the dataset can be uploaded, so you know the data will work with a script like in this example without modification.
We have various data types that can be loaded via the read_raw_bids
function:
MEG
EEG (scalp electrodes)
iEEG (ECoG and SEEG)
the anatomical MRI scan of a study participant
In this tutorial, we show how read_raw_bids
can be used to load and
inspect BIDS-formatted data.
# Authors: Adam Li <adam2392@gmail.com>
# Richard Höchenberger <richard.hoechenberger@gmail.com>
# Alex Rockhill <aprockhill@mailbox.org>
#
# License: BSD-3-Clause
Imports#
We are importing everything we need for this example:
import os
import os.path as op
import openneuro
from mne.datasets import sample
from mne_bids import (BIDSPath, read_raw_bids, print_dir_tree, make_report,
find_matching_paths, get_entity_vals)
Download a subject’s data from an OpenNeuro BIDS dataset#
Download the data, storing each in a target_dir
target directory, which,
in mne-bids
terminology, is the root of each BIDS dataset. This example
uses this EEG dataset of
resting-state recordings of patients with Parkinson’s disease.
# .. note: If the keyword argument include is left out of
# ``openneuro.download``, the whole dataset will be downloaded.
# We're just using data from one subject to reduce the time
# it takes to run the example.
dataset = 'ds002778'
subject = 'pd6'
# Download one subject's data from each dataset
bids_root = op.join(op.dirname(sample.data_path()), dataset)
if not op.isdir(bids_root):
os.makedirs(bids_root)
openneuro.download(dataset=dataset, target_dir=bids_root,
include=[f'sub-{subject}'])
Using default location ~/mne_data for sample...
Creating ~/mne_data
Downloading file 'MNE-sample-data-processed.tar.gz' from 'https://osf.io/86qa2/download?version=6' to 'C:\Users\stefan\mne_data'.
0%| | 0.00/1.65G [00:00<?, ?B/s]
0%| | 47.1k/1.65G [00:00<1:02:21, 442kB/s]
0%| | 163k/1.65G [00:00<32:44, 841kB/s]
0%| | 306k/1.65G [00:00<25:10, 1.09MB/s]
0%| | 645k/1.65G [00:00<13:57, 1.97MB/s]
0%| | 1.28M/1.65G [00:00<07:46, 3.54MB/s]
0%| | 1.81M/1.65G [00:00<07:13, 3.81MB/s]
0%| | 3.86M/1.65G [00:00<03:03, 8.97MB/s]
0%|1 | 4.92M/1.65G [00:00<03:52, 7.08MB/s]
0%|1 | 6.39M/1.65G [00:01<03:03, 8.96MB/s]
0%|1 | 7.54M/1.65G [00:01<02:51, 9.61MB/s]
1%|1 | 8.59M/1.65G [00:01<02:51, 9.57MB/s]
1%|2 | 9.61M/1.65G [00:01<03:07, 8.74MB/s]
1%|2 | 11.0M/1.65G [00:01<02:41, 10.2MB/s]
1%|2 | 12.1M/1.65G [00:01<02:59, 9.16MB/s]
1%|2 | 13.1M/1.65G [00:01<02:53, 9.43MB/s]
1%|3 | 15.1M/1.65G [00:01<02:40, 10.2MB/s]
1%|3 | 16.5M/1.65G [00:02<02:26, 11.2MB/s]
1%|3 | 17.7M/1.65G [00:02<02:35, 10.5MB/s]
1%|4 | 19.7M/1.65G [00:02<02:11, 12.4MB/s]
1%|4 | 20.9M/1.65G [00:02<02:35, 10.5MB/s]
1%|5 | 22.8M/1.65G [00:02<02:35, 10.5MB/s]
1%|5 | 24.0M/1.65G [00:02<02:34, 10.5MB/s]
2%|5 | 25.7M/1.65G [00:02<02:16, 11.9MB/s]
2%|6 | 27.4M/1.65G [00:03<02:28, 10.9MB/s]
2%|6 | 29.3M/1.65G [00:03<02:24, 11.3MB/s]
2%|6 | 30.5M/1.65G [00:03<02:23, 11.3MB/s]
2%|7 | 32.8M/1.65G [00:03<01:55, 14.0MB/s]
2%|7 | 34.3M/1.65G [00:03<02:30, 10.8MB/s]
2%|7 | 35.5M/1.65G [00:03<03:00, 8.97MB/s]
2%|8 | 36.6M/1.65G [00:03<02:54, 9.26MB/s]
2%|8 | 38.2M/1.65G [00:04<02:43, 9.87MB/s]
2%|9 | 40.3M/1.65G [00:04<02:30, 10.7MB/s]
3%|9 | 42.3M/1.65G [00:04<02:27, 10.9MB/s]
3%|9 | 43.9M/1.65G [00:04<02:13, 12.1MB/s]
3%|# | 45.2M/1.65G [00:04<02:16, 11.8MB/s]
3%|# | 46.6M/1.65G [00:04<02:21, 11.4MB/s]
3%|# | 48.9M/1.65G [00:04<01:53, 14.1MB/s]
3%|#1 | 50.4M/1.65G [00:05<02:15, 11.8MB/s]
3%|#1 | 52.2M/1.65G [00:05<02:00, 13.2MB/s]
3%|#2 | 53.6M/1.65G [00:05<02:56, 9.04MB/s]
3%|#2 | 54.8M/1.65G [00:05<03:13, 8.27MB/s]
3%|#2 | 55.8M/1.65G [00:05<03:42, 7.19MB/s]
4%|#2 | 57.9M/1.65G [00:05<02:41, 9.86MB/s]
4%|#3 | 59.2M/1.65G [00:06<03:54, 6.79MB/s]
4%|#3 | 60.2M/1.65G [00:06<04:23, 6.05MB/s]
4%|#3 | 61.0M/1.65G [00:06<04:18, 6.17MB/s]
4%|#3 | 62.5M/1.65G [00:06<03:23, 7.81MB/s]
4%|#4 | 63.5M/1.65G [00:06<03:40, 7.21MB/s]
4%|#4 | 65.0M/1.65G [00:06<02:58, 8.89MB/s]
4%|#4 | 66.9M/1.65G [00:07<02:22, 11.1MB/s]
4%|#5 | 68.2M/1.65G [00:07<02:53, 9.14MB/s]
4%|#5 | 69.3M/1.65G [00:07<04:00, 6.57MB/s]
4%|#5 | 71.2M/1.65G [00:07<03:00, 8.76MB/s]
4%|#6 | 72.4M/1.65G [00:08<04:56, 5.32MB/s]
4%|#6 | 74.3M/1.65G [00:08<03:39, 7.18MB/s]
5%|#6 | 75.4M/1.65G [00:08<04:52, 5.39MB/s]
5%|#7 | 76.8M/1.65G [00:08<04:13, 6.20MB/s]
5%|#7 | 78.2M/1.65G [00:08<03:28, 7.54MB/s]
5%|#7 | 79.3M/1.65G [00:09<04:35, 5.72MB/s]
5%|#8 | 80.8M/1.65G [00:09<03:39, 7.17MB/s]
5%|#8 | 81.9M/1.65G [00:09<05:02, 5.19MB/s]
5%|#8 | 82.7M/1.65G [00:09<05:02, 5.20MB/s]
5%|#8 | 83.9M/1.65G [00:10<04:39, 5.60MB/s]
5%|#8 | 84.6M/1.65G [00:10<04:51, 5.38MB/s]
5%|#9 | 87.1M/1.65G [00:10<03:39, 7.13MB/s]
5%|#9 | 88.2M/1.65G [00:10<03:19, 7.83MB/s]
5%|#9 | 89.1M/1.65G [00:10<03:33, 7.33MB/s]
5%|## | 90.4M/1.65G [00:10<03:05, 8.44MB/s]
6%|## | 91.3M/1.65G [00:10<03:36, 7.22MB/s]
6%|## | 92.1M/1.65G [00:11<03:51, 6.74MB/s]
6%|## | 93.3M/1.65G [00:11<03:18, 7.85MB/s]
6%|##1 | 94.8M/1.65G [00:11<02:53, 8.99MB/s]
6%|##1 | 97.2M/1.65G [00:11<02:04, 12.5MB/s]
6%|##2 | 98.6M/1.65G [00:11<02:34, 10.1MB/s]
6%|##3 | 101M/1.65G [00:11<02:28, 10.5MB/s]
6%|##3 | 103M/1.65G [00:11<02:09, 12.0MB/s]
6%|##3 | 104M/1.65G [00:12<02:15, 11.4MB/s]
6%|##4 | 105M/1.65G [00:12<02:14, 11.5MB/s]
7%|##4 | 108M/1.65G [00:12<01:44, 14.8MB/s]
7%|##5 | 110M/1.65G [00:12<02:33, 10.1MB/s]
7%|##5 | 111M/1.65G [00:12<02:10, 11.8MB/s]
7%|##5 | 113M/1.65G [00:12<02:21, 10.9MB/s]
7%|##6 | 115M/1.65G [00:13<02:26, 10.5MB/s]
7%|##6 | 117M/1.65G [00:13<02:23, 10.7MB/s]
7%|##7 | 119M/1.65G [00:13<02:17, 11.1MB/s]
7%|##7 | 120M/1.65G [00:13<02:25, 10.5MB/s]
7%|##7 | 122M/1.65G [00:13<02:39, 9.61MB/s]
7%|##8 | 122M/1.65G [00:13<02:50, 8.97MB/s]
7%|##8 | 124M/1.65G [00:13<02:39, 9.58MB/s]
8%|##8 | 125M/1.65G [00:14<02:29, 10.3MB/s]
8%|##9 | 126M/1.65G [00:14<02:21, 10.8MB/s]
8%|##9 | 127M/1.65G [00:14<02:45, 9.22MB/s]
8%|##9 | 129M/1.65G [00:14<02:32, 10.0MB/s]
8%|### | 131M/1.65G [00:14<02:20, 10.8MB/s]
8%|### | 132M/1.65G [00:14<02:10, 11.7MB/s]
8%|### | 134M/1.65G [00:14<01:55, 13.1MB/s]
8%|###1 | 135M/1.65G [00:14<01:57, 13.0MB/s]
8%|###1 | 137M/1.65G [00:15<01:58, 12.8MB/s]
8%|###1 | 138M/1.65G [00:15<01:59, 12.7MB/s]
8%|###1 | 139M/1.65G [00:15<02:00, 12.6MB/s]
8%|###2 | 140M/1.65G [00:15<02:00, 12.5MB/s]
9%|###2 | 142M/1.65G [00:15<02:01, 12.5MB/s]
9%|###2 | 143M/1.65G [00:15<02:01, 12.5MB/s]
9%|###3 | 144M/1.65G [00:15<02:56, 8.56MB/s]
9%|###3 | 145M/1.65G [00:15<03:07, 8.05MB/s]
9%|###3 | 146M/1.65G [00:16<02:44, 9.16MB/s]
9%|###3 | 148M/1.65G [00:16<02:30, 9.98MB/s]
9%|###4 | 149M/1.65G [00:16<02:53, 8.66MB/s]
9%|###4 | 151M/1.65G [00:16<02:05, 11.9MB/s]
9%|###5 | 152M/1.65G [00:16<02:31, 9.87MB/s]
9%|###5 | 154M/1.65G [00:16<02:22, 10.5MB/s]
9%|###5 | 155M/1.65G [00:16<02:19, 10.8MB/s]
10%|###6 | 157M/1.65G [00:16<01:45, 14.1MB/s]
10%|###6 | 159M/1.65G [00:17<02:10, 11.5MB/s]
10%|###6 | 160M/1.65G [00:17<02:11, 11.3MB/s]
10%|###7 | 162M/1.65G [00:17<02:06, 11.8MB/s]
10%|###7 | 163M/1.65G [00:17<02:09, 11.5MB/s]
10%|###7 | 164M/1.65G [00:17<02:24, 10.3MB/s]
10%|###8 | 167M/1.65G [00:17<01:44, 14.3MB/s]
10%|###8 | 168M/1.65G [00:17<01:47, 13.8MB/s]
10%|###9 | 170M/1.65G [00:18<02:15, 11.0MB/s]
10%|###9 | 171M/1.65G [00:18<02:07, 11.6MB/s]
10%|###9 | 173M/1.65G [00:18<02:05, 11.8MB/s]
11%|#### | 175M/1.65G [00:18<01:42, 14.4MB/s]
11%|#### | 177M/1.65G [00:18<01:54, 12.9MB/s]
11%|#### | 178M/1.65G [00:18<01:54, 12.8MB/s]
11%|####1 | 179M/1.65G [00:18<02:02, 12.0MB/s]
11%|####1 | 181M/1.65G [00:18<01:49, 13.5MB/s]
11%|####1 | 182M/1.65G [00:19<01:55, 12.7MB/s]
11%|####2 | 184M/1.65G [00:19<01:51, 13.2MB/s]
11%|####2 | 185M/1.65G [00:19<02:27, 9.97MB/s]
11%|####2 | 187M/1.65G [00:19<02:08, 11.4MB/s]
11%|####3 | 189M/1.65G [00:19<02:09, 11.3MB/s]
12%|####3 | 190M/1.65G [00:19<02:17, 10.6MB/s]
12%|####4 | 192M/1.65G [00:19<02:08, 11.4MB/s]
12%|####4 | 193M/1.65G [00:20<02:06, 11.5MB/s]
12%|####4 | 195M/1.65G [00:20<01:46, 13.7MB/s]
12%|####5 | 197M/1.65G [00:20<01:49, 13.3MB/s]
12%|####5 | 198M/1.65G [00:20<02:29, 9.76MB/s]
12%|####5 | 200M/1.65G [00:20<02:15, 10.7MB/s]
12%|####6 | 201M/1.65G [00:20<02:23, 10.1MB/s]
12%|####6 | 203M/1.65G [00:20<02:00, 12.1MB/s]
12%|####6 | 204M/1.65G [00:21<02:35, 9.33MB/s]
12%|####7 | 206M/1.65G [00:21<02:11, 11.0MB/s]
13%|####7 | 208M/1.65G [00:21<02:11, 11.0MB/s]
13%|####8 | 210M/1.65G [00:21<02:12, 10.9MB/s]
13%|####8 | 212M/1.65G [00:21<02:02, 11.8MB/s]
13%|####9 | 214M/1.65G [00:21<01:42, 14.1MB/s]
13%|####9 | 215M/1.65G [00:21<01:45, 13.6MB/s]
13%|####9 | 217M/1.65G [00:21<01:47, 13.3MB/s]
13%|##### | 218M/1.65G [00:22<02:22, 10.1MB/s]
13%|##### | 220M/1.65G [00:22<02:22, 10.1MB/s]
13%|#####1 | 222M/1.65G [00:22<01:57, 12.2MB/s]
14%|#####1 | 225M/1.65G [00:22<01:38, 14.6MB/s]
14%|#####2 | 226M/1.65G [00:22<01:54, 12.4MB/s]
14%|#####2 | 228M/1.65G [00:22<02:03, 11.6MB/s]
14%|#####2 | 229M/1.65G [00:23<02:21, 10.0MB/s]
14%|#####2 | 230M/1.65G [00:23<02:51, 8.32MB/s]
14%|#####3 | 232M/1.65G [00:23<02:19, 10.2MB/s]
14%|#####3 | 233M/1.65G [00:23<02:20, 10.1MB/s]
14%|#####3 | 235M/1.65G [00:23<02:14, 10.5MB/s]
14%|#####4 | 237M/1.65G [00:23<01:48, 13.1MB/s]
14%|#####4 | 238M/1.65G [00:23<01:46, 13.3MB/s]
14%|#####5 | 240M/1.65G [00:24<01:48, 13.1MB/s]
15%|#####5 | 241M/1.65G [00:24<01:57, 12.1MB/s]
15%|#####5 | 242M/1.65G [00:24<02:04, 11.3MB/s]
15%|#####5 | 243M/1.65G [00:24<02:04, 11.3MB/s]
15%|#####6 | 245M/1.65G [00:24<01:43, 13.6MB/s]
15%|#####6 | 247M/1.65G [00:24<01:45, 13.3MB/s]
15%|#####7 | 248M/1.65G [00:24<02:27, 9.50MB/s]
15%|#####7 | 249M/1.65G [00:25<02:55, 8.02MB/s]
15%|#####7 | 250M/1.65G [00:25<03:03, 7.66MB/s]
15%|#####7 | 252M/1.65G [00:25<02:20, 9.98MB/s]
15%|#####8 | 254M/1.65G [00:25<02:00, 11.6MB/s]
15%|#####8 | 255M/1.65G [00:25<01:57, 11.9MB/s]
16%|#####8 | 256M/1.65G [00:25<02:24, 9.69MB/s]
16%|#####9 | 258M/1.65G [00:25<02:18, 10.1MB/s]
16%|#####9 | 260M/1.65G [00:25<01:41, 13.7MB/s]
16%|###### | 262M/1.65G [00:26<01:44, 13.3MB/s]
16%|###### | 263M/1.65G [00:26<01:46, 13.1MB/s]
16%|###### | 264M/1.65G [00:26<01:50, 12.6MB/s]
16%|######1 | 266M/1.65G [00:26<02:20, 9.87MB/s]
16%|######1 | 268M/1.65G [00:26<01:42, 13.6MB/s]
16%|######2 | 270M/1.65G [00:26<01:44, 13.2MB/s]
16%|######2 | 271M/1.65G [00:26<01:57, 11.8MB/s]
16%|######2 | 273M/1.65G [00:26<02:04, 11.1MB/s]
17%|######3 | 275M/1.65G [00:27<01:41, 13.6MB/s]
17%|######3 | 276M/1.65G [00:27<01:43, 13.3MB/s]
17%|######3 | 278M/1.65G [00:27<02:06, 10.9MB/s]
17%|######4 | 279M/1.65G [00:27<02:07, 10.7MB/s]
17%|######4 | 281M/1.65G [00:27<02:19, 9.84MB/s]
17%|######5 | 283M/1.65G [00:27<01:58, 11.6MB/s]
17%|######5 | 285M/1.65G [00:27<01:38, 13.9MB/s]
17%|######5 | 287M/1.65G [00:28<01:52, 12.1MB/s]
17%|######6 | 289M/1.65G [00:28<01:59, 11.4MB/s]
18%|######6 | 291M/1.65G [00:28<01:56, 11.7MB/s]
18%|######7 | 292M/1.65G [00:28<01:55, 11.8MB/s]
18%|######7 | 293M/1.65G [00:28<01:49, 12.4MB/s]
18%|######7 | 296M/1.65G [00:28<01:32, 14.6MB/s]
18%|######8 | 297M/1.65G [00:28<01:37, 13.9MB/s]
18%|######8 | 299M/1.65G [00:29<01:40, 13.5MB/s]
18%|######8 | 300M/1.65G [00:29<01:42, 13.2MB/s]
18%|######9 | 301M/1.65G [00:29<01:52, 12.0MB/s]
18%|######9 | 303M/1.65G [00:29<01:56, 11.6MB/s]
18%|######9 | 304M/1.65G [00:29<01:41, 13.3MB/s]
18%|####### | 306M/1.65G [00:29<02:00, 11.2MB/s]
19%|####### | 307M/1.65G [00:29<02:04, 10.8MB/s]
19%|#######1 | 309M/1.65G [00:29<01:36, 13.9MB/s]
19%|#######1 | 311M/1.65G [00:30<01:39, 13.5MB/s]
19%|#######1 | 312M/1.65G [00:30<01:41, 13.2MB/s]
19%|#######2 | 314M/1.65G [00:30<01:43, 12.9MB/s]
19%|#######2 | 315M/1.65G [00:30<01:44, 12.8MB/s]
19%|#######2 | 316M/1.65G [00:30<01:45, 12.7MB/s]
19%|#######2 | 317M/1.65G [00:30<01:45, 12.6MB/s]
19%|#######3 | 319M/1.65G [00:30<02:11, 10.1MB/s]
19%|#######3 | 321M/1.65G [00:30<02:06, 10.5MB/s]
20%|#######4 | 323M/1.65G [00:31<01:45, 12.6MB/s]
20%|#######4 | 324M/1.65G [00:31<01:45, 12.6MB/s]
20%|#######4 | 326M/1.65G [00:31<01:36, 13.8MB/s]
20%|#######5 | 327M/1.65G [00:31<01:58, 11.2MB/s]
20%|#######5 | 329M/1.65G [00:31<01:54, 11.6MB/s]
20%|#######6 | 331M/1.65G [00:31<01:52, 11.7MB/s]
20%|#######6 | 333M/1.65G [00:31<01:33, 14.1MB/s]
20%|#######6 | 335M/1.65G [00:32<01:58, 11.1MB/s]
20%|#######7 | 337M/1.65G [00:32<01:34, 13.9MB/s]
20%|#######7 | 339M/1.65G [00:32<01:37, 13.5MB/s]
21%|#######8 | 340M/1.65G [00:32<02:14, 9.72MB/s]
21%|#######8 | 341M/1.65G [00:32<02:12, 9.88MB/s]
21%|#######8 | 343M/1.65G [00:32<02:22, 9.19MB/s]
21%|#######9 | 344M/1.65G [00:32<02:19, 9.38MB/s]
21%|#######9 | 346M/1.65G [00:33<01:44, 12.5MB/s]
21%|#######9 | 348M/1.65G [00:33<01:53, 11.5MB/s]
21%|######## | 349M/1.65G [00:33<01:56, 11.2MB/s]
21%|######## | 350M/1.65G [00:33<01:59, 10.9MB/s]
21%|########1 | 353M/1.65G [00:33<01:35, 13.7MB/s]
21%|########1 | 354M/1.65G [00:33<01:37, 13.3MB/s]
22%|########1 | 355M/1.65G [00:33<01:59, 10.9MB/s]
22%|########2 | 358M/1.65G [00:34<01:59, 10.8MB/s]
22%|########2 | 360M/1.65G [00:34<01:32, 13.9MB/s]
22%|########3 | 362M/1.65G [00:34<01:35, 13.5MB/s]
22%|########3 | 363M/1.65G [00:34<02:00, 10.7MB/s]
22%|########3 | 364M/1.65G [00:34<02:11, 9.78MB/s]
22%|########4 | 367M/1.65G [00:34<01:39, 13.0MB/s]
22%|########4 | 368M/1.65G [00:34<01:53, 11.3MB/s]
22%|########5 | 370M/1.65G [00:35<01:58, 10.8MB/s]
22%|########5 | 371M/1.65G [00:35<01:56, 11.0MB/s]
23%|########5 | 372M/1.65G [00:35<01:50, 11.6MB/s]
23%|########5 | 374M/1.65G [00:35<01:50, 11.6MB/s]
23%|########6 | 375M/1.65G [00:35<01:36, 13.2MB/s]
23%|########6 | 377M/1.65G [00:35<01:25, 14.9MB/s]
23%|########7 | 379M/1.65G [00:35<02:07, 10.0MB/s]
23%|########7 | 380M/1.65G [00:36<01:53, 11.2MB/s]
23%|########7 | 382M/1.65G [00:36<02:11, 9.67MB/s]
23%|########8 | 384M/1.65G [00:36<01:42, 12.4MB/s]
23%|########8 | 386M/1.65G [00:36<01:41, 12.5MB/s]
23%|########8 | 387M/1.65G [00:36<01:49, 11.5MB/s]
23%|########9 | 388M/1.65G [00:36<02:33, 8.21MB/s]
24%|########9 | 391M/1.65G [00:36<01:54, 11.0MB/s]
24%|######### | 392M/1.65G [00:37<01:51, 11.3MB/s]
24%|######### | 393M/1.65G [00:37<01:48, 11.6MB/s]
24%|######### | 395M/1.65G [00:37<01:46, 11.8MB/s]
24%|#########1 | 396M/1.65G [00:37<01:45, 11.9MB/s]
24%|#########1 | 397M/1.65G [00:37<02:00, 10.4MB/s]
24%|#########1 | 398M/1.65G [00:37<02:11, 9.52MB/s]
24%|#########1 | 400M/1.65G [00:37<01:51, 11.2MB/s]
24%|#########2 | 402M/1.65G [00:37<01:33, 13.4MB/s]
24%|#########2 | 404M/1.65G [00:38<01:35, 13.1MB/s]
24%|#########3 | 405M/1.65G [00:38<01:36, 12.9MB/s]
25%|#########3 | 406M/1.65G [00:38<01:37, 12.8MB/s]
25%|#########3 | 408M/1.65G [00:38<02:11, 9.46MB/s]
25%|#########4 | 409M/1.65G [00:38<01:53, 11.0MB/s]
25%|#########4 | 410M/1.65G [00:38<02:11, 9.43MB/s]
25%|#########4 | 411M/1.65G [00:38<02:08, 9.68MB/s]
25%|#########5 | 414M/1.65G [00:39<01:50, 11.2MB/s]
25%|#########5 | 416M/1.65G [00:39<01:30, 13.7MB/s]
25%|#########5 | 417M/1.65G [00:39<01:32, 13.4MB/s]
25%|#########6 | 419M/1.65G [00:39<01:59, 10.3MB/s]
25%|#########6 | 421M/1.65G [00:39<01:49, 11.3MB/s]
26%|#########7 | 423M/1.65G [00:39<01:27, 14.0MB/s]
26%|#########7 | 425M/1.65G [00:39<01:38, 12.4MB/s]
26%|#########8 | 426M/1.65G [00:40<01:42, 11.9MB/s]
26%|#########8 | 428M/1.65G [00:40<01:29, 13.8MB/s]
26%|#########8 | 430M/1.65G [00:40<02:01, 10.1MB/s]
26%|#########9 | 431M/1.65G [00:40<01:57, 10.4MB/s]
26%|#########9 | 432M/1.65G [00:40<02:14, 9.07MB/s]
26%|#########9 | 434M/1.65G [00:40<02:03, 9.87MB/s]
26%|########## | 436M/1.65G [00:40<01:39, 12.2MB/s]
26%|########## | 438M/1.65G [00:41<01:33, 13.0MB/s]
27%|########## | 439M/1.65G [00:41<01:44, 11.6MB/s]
27%|##########1 | 441M/1.65G [00:41<01:49, 11.0MB/s]
27%|##########1 | 443M/1.65G [00:41<01:29, 13.5MB/s]
27%|##########2 | 444M/1.65G [00:41<01:36, 12.6MB/s]
27%|##########2 | 446M/1.65G [00:41<01:41, 11.9MB/s]
27%|##########2 | 447M/1.65G [00:41<01:30, 13.3MB/s]
27%|##########3 | 449M/1.65G [00:41<01:36, 12.4MB/s]
27%|##########3 | 450M/1.65G [00:42<01:51, 10.8MB/s]
27%|##########3 | 452M/1.65G [00:42<01:48, 11.1MB/s]
27%|##########4 | 453M/1.65G [00:42<01:40, 12.0MB/s]
28%|##########4 | 455M/1.65G [00:42<01:40, 11.9MB/s]
28%|##########5 | 457M/1.65G [00:42<01:25, 14.1MB/s]
28%|##########5 | 459M/1.65G [00:42<01:29, 13.3MB/s]
28%|##########5 | 460M/1.65G [00:42<01:29, 13.3MB/s]
28%|##########6 | 462M/1.65G [00:43<01:37, 12.2MB/s]
28%|##########6 | 463M/1.65G [00:43<01:54, 10.4MB/s]
28%|##########6 | 465M/1.65G [00:43<01:56, 10.2MB/s]
28%|##########7 | 467M/1.65G [00:43<01:44, 11.4MB/s]
28%|##########7 | 468M/1.65G [00:43<01:49, 10.9MB/s]
28%|##########7 | 470M/1.65G [00:43<02:05, 9.45MB/s]
29%|##########8 | 472M/1.65G [00:43<01:36, 12.2MB/s]
29%|##########8 | 473M/1.65G [00:44<01:51, 10.6MB/s]
29%|##########9 | 475M/1.65G [00:44<01:31, 12.8MB/s]
29%|##########9 | 477M/1.65G [00:44<01:32, 12.8MB/s]
29%|##########9 | 478M/1.65G [00:44<01:32, 12.7MB/s]
29%|########### | 479M/1.65G [00:44<01:45, 11.1MB/s]
29%|########### | 481M/1.65G [00:44<01:30, 12.9MB/s]
29%|########### | 483M/1.65G [00:44<01:32, 12.6MB/s]
29%|###########1 | 484M/1.65G [00:44<01:34, 12.4MB/s]
29%|###########1 | 485M/1.65G [00:45<01:52, 10.4MB/s]
29%|###########1 | 487M/1.65G [00:45<01:49, 10.6MB/s]
30%|###########2 | 488M/1.65G [00:45<01:38, 11.8MB/s]
30%|###########2 | 490M/1.65G [00:45<01:21, 14.2MB/s]
30%|###########3 | 492M/1.65G [00:45<01:39, 11.7MB/s]
30%|###########3 | 493M/1.65G [00:45<01:54, 10.1MB/s]
30%|###########3 | 496M/1.65G [00:45<01:35, 12.1MB/s]
30%|###########4 | 497M/1.65G [00:46<01:23, 13.8MB/s]
30%|###########4 | 499M/1.65G [00:46<01:42, 11.2MB/s]
30%|###########5 | 500M/1.65G [00:46<01:46, 10.8MB/s]
30%|###########5 | 501M/1.65G [00:46<01:46, 10.8MB/s]
30%|###########5 | 504M/1.65G [00:46<01:40, 11.4MB/s]
31%|###########6 | 506M/1.65G [00:46<01:33, 12.3MB/s]
31%|###########6 | 507M/1.65G [00:46<01:48, 10.6MB/s]
31%|###########6 | 508M/1.65G [00:47<01:57, 9.72MB/s]
31%|###########7 | 510M/1.65G [00:47<01:42, 11.1MB/s]
31%|###########7 | 511M/1.65G [00:47<01:39, 11.4MB/s]
31%|###########7 | 512M/1.65G [00:47<01:37, 11.7MB/s]
31%|###########8 | 514M/1.65G [00:47<02:22, 7.98MB/s]
31%|###########8 | 514M/1.65G [00:47<02:44, 6.93MB/s]
31%|###########8 | 516M/1.65G [00:48<02:19, 8.13MB/s]
31%|###########8 | 517M/1.65G [00:48<02:05, 9.08MB/s]
31%|###########9 | 518M/1.65G [00:48<02:25, 7.81MB/s]
31%|###########9 | 519M/1.65G [00:48<02:09, 8.76MB/s]
31%|###########9 | 520M/1.65G [00:48<02:06, 8.92MB/s]
32%|###########9 | 522M/1.65G [00:48<02:04, 9.08MB/s]
32%|############ | 523M/1.65G [00:48<01:51, 10.1MB/s]
32%|############ | 524M/1.65G [00:48<01:50, 10.2MB/s]
32%|############ | 526M/1.65G [00:49<01:46, 10.6MB/s]
32%|############1 | 528M/1.65G [00:49<01:24, 13.3MB/s]
32%|############1 | 530M/1.65G [00:49<01:26, 13.0MB/s]
32%|############2 | 531M/1.65G [00:49<01:38, 11.3MB/s]
32%|############2 | 532M/1.65G [00:49<01:42, 10.9MB/s]
32%|############2 | 534M/1.65G [00:49<01:23, 13.5MB/s]
32%|############3 | 536M/1.65G [00:49<01:24, 13.2MB/s]
32%|############3 | 537M/1.65G [00:49<01:41, 11.0MB/s]
33%|############3 | 538M/1.65G [00:50<01:36, 11.6MB/s]
33%|############4 | 540M/1.65G [00:50<01:56, 9.54MB/s]
33%|############4 | 542M/1.65G [00:50<01:33, 11.8MB/s]
33%|############4 | 543M/1.65G [00:50<01:31, 12.1MB/s]
33%|############5 | 544M/1.65G [00:50<01:34, 11.8MB/s]
33%|############5 | 546M/1.65G [00:50<01:30, 12.3MB/s]
33%|############5 | 547M/1.65G [00:50<01:30, 12.2MB/s]
33%|############6 | 548M/1.65G [00:50<01:49, 10.1MB/s]
33%|############6 | 549M/1.65G [00:51<01:49, 10.1MB/s]
33%|############6 | 551M/1.65G [00:51<01:55, 9.52MB/s]
33%|############6 | 552M/1.65G [00:51<01:51, 9.85MB/s]
33%|############7 | 553M/1.65G [00:51<01:46, 10.3MB/s]
34%|############7 | 554M/1.65G [00:51<01:42, 10.7MB/s]
34%|############7 | 555M/1.65G [00:51<01:39, 11.0MB/s]
34%|############7 | 556M/1.65G [00:51<01:37, 11.3MB/s]
34%|############8 | 558M/1.65G [00:51<02:06, 8.64MB/s]
34%|############8 | 559M/1.65G [00:52<01:48, 10.0MB/s]
34%|############8 | 560M/1.65G [00:52<01:44, 10.5MB/s]
34%|############9 | 561M/1.65G [00:52<01:53, 9.59MB/s]
34%|############9 | 563M/1.65G [00:52<01:46, 10.2MB/s]
34%|############9 | 564M/1.65G [00:52<01:49, 9.92MB/s]
34%|############# | 566M/1.65G [00:52<01:26, 12.6MB/s]
34%|############# | 568M/1.65G [00:52<01:32, 11.7MB/s]
34%|############# | 569M/1.65G [00:52<01:30, 11.9MB/s]
34%|#############1 | 570M/1.65G [00:52<01:31, 11.9MB/s]
35%|#############1 | 571M/1.65G [00:53<01:40, 10.8MB/s]
35%|#############1 | 573M/1.65G [00:53<01:27, 12.3MB/s]
35%|#############2 | 574M/1.65G [00:53<01:27, 12.3MB/s]
35%|#############2 | 576M/1.65G [00:53<01:27, 12.3MB/s]
35%|#############2 | 577M/1.65G [00:53<01:42, 10.5MB/s]
35%|#############3 | 579M/1.65G [00:53<01:26, 12.5MB/s]
35%|#############3 | 580M/1.65G [00:53<01:43, 10.4MB/s]
35%|#############3 | 582M/1.65G [00:53<01:30, 11.9MB/s]
35%|#############4 | 583M/1.65G [00:54<01:29, 12.0MB/s]
35%|#############4 | 584M/1.65G [00:54<01:28, 12.1MB/s]
35%|#############4 | 586M/1.65G [00:54<01:28, 12.1MB/s]
36%|#############4 | 587M/1.65G [00:54<01:52, 9.46MB/s]
36%|#############5 | 589M/1.65G [00:54<01:41, 10.5MB/s]
36%|#############5 | 592M/1.65G [00:54<01:18, 13.5MB/s]
36%|#############6 | 593M/1.65G [00:54<01:23, 12.7MB/s]
36%|#############6 | 594M/1.65G [00:55<01:21, 12.9MB/s]
36%|#############6 | 596M/1.65G [00:55<01:52, 9.40MB/s]
36%|#############7 | 597M/1.65G [00:55<01:50, 9.59MB/s]
36%|#############7 | 599M/1.65G [00:55<01:27, 12.1MB/s]
36%|#############8 | 600M/1.65G [00:55<01:48, 9.66MB/s]
36%|#############8 | 603M/1.65G [00:55<01:20, 13.0MB/s]
37%|#############8 | 605M/1.65G [00:56<01:55, 9.10MB/s]
37%|#############9 | 606M/1.65G [00:56<01:41, 10.3MB/s]
37%|#############9 | 607M/1.65G [00:56<01:46, 9.80MB/s]
37%|#############9 | 609M/1.65G [00:56<01:54, 9.13MB/s]
37%|############## | 610M/1.65G [00:56<02:13, 7.81MB/s]
37%|############## | 612M/1.65G [00:56<01:59, 8.72MB/s]
37%|##############1 | 614M/1.65G [00:57<01:35, 10.8MB/s]
37%|##############1 | 615M/1.65G [00:57<01:41, 10.3MB/s]
37%|##############1 | 616M/1.65G [00:57<01:40, 10.4MB/s]
37%|##############2 | 618M/1.65G [00:57<01:26, 11.9MB/s]
37%|##############2 | 619M/1.65G [00:57<01:30, 11.4MB/s]
38%|##############2 | 622M/1.65G [00:57<01:13, 14.0MB/s]
38%|##############3 | 623M/1.65G [00:57<01:21, 12.6MB/s]
38%|##############3 | 625M/1.65G [00:57<01:17, 13.3MB/s]
38%|##############3 | 626M/1.65G [00:58<01:32, 11.1MB/s]
38%|##############4 | 628M/1.65G [00:58<01:19, 12.9MB/s]
38%|##############4 | 629M/1.65G [00:58<01:33, 10.9MB/s]
38%|##############5 | 631M/1.65G [00:58<01:30, 11.3MB/s]
38%|##############5 | 633M/1.65G [00:58<01:24, 12.1MB/s]
38%|##############5 | 634M/1.65G [00:58<01:14, 13.6MB/s]
38%|##############6 | 636M/1.65G [00:58<01:33, 10.8MB/s]
39%|##############6 | 638M/1.65G [00:59<01:14, 13.7MB/s]
39%|##############7 | 640M/1.65G [00:59<01:15, 13.3MB/s]
39%|##############7 | 641M/1.65G [00:59<01:50, 9.17MB/s]
39%|##############7 | 643M/1.65G [00:59<01:52, 8.97MB/s]
39%|##############7 | 644M/1.65G [00:59<01:48, 9.29MB/s]
39%|##############8 | 645M/1.65G [00:59<02:00, 8.35MB/s]
39%|##############8 | 646M/1.65G [00:59<01:45, 9.51MB/s]
39%|##############9 | 648M/1.65G [01:00<01:37, 10.3MB/s]
39%|##############9 | 650M/1.65G [01:00<01:28, 11.3MB/s]
39%|##############9 | 651M/1.65G [01:00<01:24, 11.9MB/s]
40%|############### | 653M/1.65G [01:00<01:14, 13.5MB/s]
40%|############### | 654M/1.65G [01:00<01:15, 13.2MB/s]
40%|############### | 656M/1.65G [01:00<01:22, 12.1MB/s]
40%|###############1 | 657M/1.65G [01:00<01:40, 9.87MB/s]
40%|###############1 | 660M/1.65G [01:00<01:11, 13.8MB/s]
40%|###############2 | 661M/1.65G [01:01<01:42, 9.68MB/s]
40%|###############2 | 663M/1.65G [01:01<01:37, 10.2MB/s]
40%|###############2 | 664M/1.65G [01:01<01:25, 11.5MB/s]
40%|###############3 | 666M/1.65G [01:01<01:31, 10.8MB/s]
40%|###############3 | 667M/1.65G [01:01<01:20, 12.3MB/s]
40%|###############3 | 669M/1.65G [01:01<01:20, 12.3MB/s]
41%|###############4 | 670M/1.65G [01:01<01:19, 12.3MB/s]
41%|###############4 | 671M/1.65G [01:02<01:19, 12.4MB/s]
41%|###############4 | 673M/1.65G [01:02<01:52, 8.75MB/s]
41%|###############5 | 674M/1.65G [01:02<01:35, 10.3MB/s]
41%|###############5 | 676M/1.65G [01:02<01:30, 10.8MB/s]
41%|###############5 | 677M/1.65G [01:02<01:27, 11.2MB/s]
41%|###############5 | 678M/1.65G [01:02<01:42, 9.52MB/s]
41%|###############6 | 679M/1.65G [01:02<01:40, 9.71MB/s]
41%|###############6 | 681M/1.65G [01:03<01:31, 10.7MB/s]
41%|###############7 | 683M/1.65G [01:03<01:09, 14.0MB/s]
41%|###############7 | 685M/1.65G [01:03<01:19, 12.1MB/s]
42%|###############7 | 686M/1.65G [01:03<01:28, 10.9MB/s]
42%|###############8 | 688M/1.65G [01:03<01:30, 10.7MB/s]
42%|###############8 | 689M/1.65G [01:03<01:36, 10.0MB/s]
42%|###############8 | 691M/1.65G [01:03<01:11, 13.4MB/s]
42%|###############9 | 693M/1.65G [01:03<01:13, 13.1MB/s]
42%|###############9 | 694M/1.65G [01:04<01:14, 13.0MB/s]
42%|###############9 | 695M/1.65G [01:04<01:14, 12.8MB/s]
42%|################ | 697M/1.65G [01:04<01:37, 9.83MB/s]
42%|################ | 698M/1.65G [01:04<01:32, 10.3MB/s]
42%|################ | 700M/1.65G [01:04<01:29, 10.7MB/s]
42%|################1 | 702M/1.65G [01:04<01:27, 10.8MB/s]
43%|################1 | 703M/1.65G [01:04<01:32, 10.3MB/s]
43%|################1 | 704M/1.65G [01:05<01:44, 9.07MB/s]
43%|################2 | 705M/1.65G [01:05<01:31, 10.3MB/s]
43%|################2 | 706M/1.65G [01:05<01:27, 10.8MB/s]
43%|################2 | 708M/1.65G [01:05<01:24, 11.2MB/s]
43%|################2 | 709M/1.65G [01:05<01:32, 10.2MB/s]
43%|################3 | 710M/1.65G [01:05<01:44, 9.00MB/s]
43%|################3 | 711M/1.65G [01:05<01:35, 9.85MB/s]
43%|################3 | 712M/1.65G [01:05<01:29, 10.5MB/s]
43%|################4 | 713M/1.65G [01:06<01:35, 9.86MB/s]
43%|################4 | 715M/1.65G [01:06<01:19, 11.8MB/s]
43%|################4 | 716M/1.65G [01:06<01:18, 12.0MB/s]
43%|################5 | 718M/1.65G [01:06<01:17, 12.1MB/s]
44%|################5 | 719M/1.65G [01:06<01:16, 12.2MB/s]
44%|################5 | 720M/1.65G [01:06<01:16, 12.2MB/s]
44%|################5 | 721M/1.65G [01:06<01:37, 9.51MB/s]
44%|################6 | 723M/1.65G [01:06<01:46, 8.71MB/s]
44%|################6 | 724M/1.65G [01:07<01:25, 10.9MB/s]
44%|################7 | 726M/1.65G [01:07<01:09, 13.4MB/s]
44%|################7 | 728M/1.65G [01:07<01:18, 11.8MB/s]
44%|################7 | 729M/1.65G [01:07<01:20, 11.4MB/s]
44%|################8 | 731M/1.65G [01:07<01:12, 12.7MB/s]
44%|################8 | 733M/1.65G [01:07<01:07, 13.6MB/s]
44%|################8 | 734M/1.65G [01:07<01:15, 12.1MB/s]
45%|################9 | 736M/1.65G [01:07<01:08, 13.3MB/s]
45%|################9 | 737M/1.65G [01:07<01:10, 13.1MB/s]
45%|################9 | 738M/1.65G [01:08<01:20, 11.4MB/s]
45%|################# | 740M/1.65G [01:08<01:09, 13.2MB/s]
45%|################# | 742M/1.65G [01:08<01:39, 9.16MB/s]
45%|################# | 743M/1.65G [01:08<01:31, 9.93MB/s]
45%|#################1 | 744M/1.65G [01:08<01:23, 10.8MB/s]
45%|#################1 | 746M/1.65G [01:08<01:28, 10.3MB/s]
45%|#################1 | 747M/1.65G [01:08<01:15, 11.9MB/s]
45%|#################2 | 749M/1.65G [01:09<01:21, 11.1MB/s]
45%|#################2 | 750M/1.65G [01:09<01:27, 10.3MB/s]
46%|#################2 | 752M/1.65G [01:09<01:08, 13.1MB/s]
46%|#################3 | 754M/1.65G [01:09<01:20, 11.1MB/s]
46%|#################3 | 755M/1.65G [01:09<01:22, 10.9MB/s]
46%|#################4 | 758M/1.65G [01:09<01:07, 13.2MB/s]
46%|#################4 | 759M/1.65G [01:09<01:15, 11.8MB/s]
46%|#################4 | 761M/1.65G [01:10<01:07, 13.2MB/s]
46%|#################5 | 762M/1.65G [01:10<01:08, 13.0MB/s]
46%|#################5 | 764M/1.65G [01:10<01:09, 12.9MB/s]
46%|#################5 | 765M/1.65G [01:10<01:21, 10.9MB/s]
46%|#################6 | 766M/1.65G [01:10<01:25, 10.4MB/s]
46%|#################6 | 768M/1.65G [01:10<01:19, 11.1MB/s]
47%|#################6 | 769M/1.65G [01:10<01:14, 11.8MB/s]
47%|#################7 | 770M/1.65G [01:11<01:46, 8.29MB/s]
47%|#################7 | 771M/1.65G [01:11<01:36, 9.16MB/s]
47%|#################7 | 773M/1.65G [01:11<01:28, 9.95MB/s]
47%|#################7 | 774M/1.65G [01:11<01:37, 9.04MB/s]
47%|#################8 | 776M/1.65G [01:11<01:14, 11.7MB/s]
47%|#################8 | 777M/1.65G [01:11<01:13, 11.9MB/s]
47%|#################8 | 778M/1.65G [01:11<01:30, 9.63MB/s]
47%|#################9 | 781M/1.65G [01:11<01:08, 12.7MB/s]
47%|#################9 | 782M/1.65G [01:12<01:07, 12.9MB/s]
47%|################## | 784M/1.65G [01:12<01:26, 10.0MB/s]
47%|################## | 785M/1.65G [01:12<01:39, 8.74MB/s]
48%|################## | 786M/1.65G [01:12<01:30, 9.59MB/s]
48%|##################1 | 790M/1.65G [01:12<00:54, 15.7MB/s]
48%|##################2 | 792M/1.65G [01:12<00:58, 14.7MB/s]
48%|##################2 | 794M/1.65G [01:12<01:01, 14.0MB/s]
48%|##################2 | 795M/1.65G [01:13<01:03, 13.5MB/s]
48%|##################3 | 797M/1.65G [01:13<01:04, 13.2MB/s]
48%|##################3 | 798M/1.65G [01:13<01:31, 9.38MB/s]
48%|##################3 | 799M/1.65G [01:13<01:37, 8.78MB/s]
48%|##################4 | 801M/1.65G [01:13<01:35, 8.93MB/s]
49%|##################4 | 803M/1.65G [01:13<01:12, 11.8MB/s]
49%|##################4 | 805M/1.65G [01:13<01:10, 12.0MB/s]
49%|##################5 | 806M/1.65G [01:14<01:21, 10.4MB/s]
49%|##################5 | 808M/1.65G [01:14<01:06, 12.7MB/s]
49%|##################6 | 809M/1.65G [01:14<01:06, 12.7MB/s]
49%|##################6 | 811M/1.65G [01:14<01:18, 10.7MB/s]
49%|##################6 | 812M/1.65G [01:14<01:17, 10.8MB/s]
49%|##################7 | 814M/1.65G [01:14<01:01, 13.7MB/s]
49%|##################7 | 816M/1.65G [01:14<01:03, 13.3MB/s]
49%|##################7 | 817M/1.65G [01:15<01:15, 11.0MB/s]
50%|##################8 | 819M/1.65G [01:15<01:16, 10.9MB/s]
50%|##################8 | 820M/1.65G [01:15<01:13, 11.3MB/s]
50%|##################8 | 822M/1.65G [01:15<01:04, 12.9MB/s]
50%|##################9 | 823M/1.65G [01:15<01:13, 11.2MB/s]
50%|##################9 | 825M/1.65G [01:15<00:56, 14.5MB/s]
50%|################### | 827M/1.65G [01:15<00:59, 13.9MB/s]
50%|################### | 829M/1.65G [01:16<01:17, 10.7MB/s]
50%|################### | 830M/1.65G [01:16<01:12, 11.4MB/s]
50%|###################1 | 832M/1.65G [01:16<01:11, 11.5MB/s]
50%|###################1 | 834M/1.65G [01:16<00:55, 14.6MB/s]
51%|###################2 | 836M/1.65G [01:16<01:02, 13.0MB/s]
51%|###################2 | 837M/1.65G [01:16<01:12, 11.2MB/s]
51%|###################3 | 840M/1.65G [01:16<00:57, 14.1MB/s]
51%|###################3 | 841M/1.65G [01:17<01:08, 11.8MB/s]
51%|###################3 | 843M/1.65G [01:17<01:18, 10.4MB/s]
51%|###################4 | 845M/1.65G [01:17<01:06, 12.2MB/s]
51%|###################4 | 846M/1.65G [01:17<01:05, 12.3MB/s]
51%|###################4 | 847M/1.65G [01:17<01:14, 10.8MB/s]
51%|###################5 | 849M/1.65G [01:17<01:02, 12.8MB/s]
51%|###################5 | 851M/1.65G [01:17<01:16, 10.4MB/s]
52%|###################5 | 852M/1.65G [01:17<01:11, 11.2MB/s]
52%|###################6 | 854M/1.65G [01:18<01:02, 12.8MB/s]
52%|###################6 | 855M/1.65G [01:18<01:02, 12.7MB/s]
52%|###################6 | 857M/1.65G [01:18<01:07, 11.9MB/s]
52%|###################7 | 858M/1.65G [01:18<01:02, 12.8MB/s]
52%|###################7 | 860M/1.65G [01:18<01:02, 12.7MB/s]
52%|###################7 | 861M/1.65G [01:18<01:02, 12.6MB/s]
52%|###################8 | 862M/1.65G [01:18<01:22, 9.64MB/s]
52%|###################8 | 863M/1.65G [01:18<01:20, 9.82MB/s]
52%|###################8 | 864M/1.65G [01:19<01:18, 10.1MB/s]
52%|###################9 | 866M/1.65G [01:19<01:13, 10.6MB/s]
52%|###################9 | 867M/1.65G [01:19<01:07, 11.6MB/s]
53%|###################9 | 868M/1.65G [01:19<01:14, 10.6MB/s]
53%|#################### | 870M/1.65G [01:19<01:03, 12.4MB/s]
53%|#################### | 871M/1.65G [01:19<01:02, 12.4MB/s]
53%|#################### | 873M/1.65G [01:19<01:32, 8.47MB/s]
53%|#################### | 874M/1.65G [01:20<01:36, 8.05MB/s]
53%|####################1 | 875M/1.65G [01:20<01:42, 7.61MB/s]
53%|####################1 | 877M/1.65G [01:20<01:15, 10.3MB/s]
53%|####################1 | 878M/1.65G [01:20<01:16, 10.1MB/s]
53%|####################2 | 880M/1.65G [01:20<01:06, 11.6MB/s]
53%|####################2 | 881M/1.65G [01:20<01:14, 10.3MB/s]
53%|####################2 | 883M/1.65G [01:20<01:15, 10.3MB/s]
53%|####################3 | 884M/1.65G [01:20<01:11, 10.8MB/s]
54%|####################3 | 886M/1.65G [01:21<00:56, 13.5MB/s]
54%|####################4 | 888M/1.65G [01:21<01:06, 11.6MB/s]
54%|####################4 | 889M/1.65G [01:21<01:08, 11.2MB/s]
54%|####################4 | 891M/1.65G [01:21<00:54, 13.9MB/s]
54%|####################5 | 893M/1.65G [01:21<00:56, 13.4MB/s]
54%|####################5 | 894M/1.65G [01:21<01:09, 10.9MB/s]
54%|####################6 | 896M/1.65G [01:21<00:55, 13.6MB/s]
54%|####################6 | 898M/1.65G [01:22<00:57, 13.2MB/s]
54%|####################6 | 899M/1.65G [01:22<00:57, 13.0MB/s]
54%|####################7 | 901M/1.65G [01:22<00:59, 12.7MB/s]
55%|####################7 | 902M/1.65G [01:22<01:16, 9.80MB/s]
55%|####################7 | 903M/1.65G [01:22<01:11, 10.5MB/s]
55%|####################7 | 905M/1.65G [01:22<01:26, 8.62MB/s]
55%|####################8 | 907M/1.65G [01:22<01:12, 10.3MB/s]
55%|####################8 | 909M/1.65G [01:23<00:58, 12.8MB/s]
55%|####################9 | 910M/1.65G [01:23<01:00, 12.2MB/s]
55%|####################9 | 912M/1.65G [01:23<00:58, 12.7MB/s]
55%|####################9 | 913M/1.65G [01:23<00:53, 13.9MB/s]
55%|##################### | 915M/1.65G [01:23<00:54, 13.5MB/s]
55%|##################### | 916M/1.65G [01:23<00:55, 13.2MB/s]
56%|##################### | 918M/1.65G [01:23<00:56, 13.0MB/s]
56%|#####################1 | 919M/1.65G [01:23<00:57, 12.8MB/s]
56%|#####################1 | 920M/1.65G [01:23<01:10, 10.3MB/s]
56%|#####################1 | 921M/1.65G [01:24<01:26, 8.47MB/s]
56%|#####################2 | 923M/1.65G [01:24<01:14, 9.74MB/s]
56%|#####################2 | 924M/1.65G [01:24<01:07, 10.8MB/s]
56%|#####################2 | 925M/1.65G [01:24<01:18, 9.27MB/s]
56%|#####################3 | 928M/1.65G [01:24<00:58, 12.3MB/s]
56%|#####################3 | 929M/1.65G [01:24<00:58, 12.4MB/s]
56%|#####################3 | 930M/1.65G [01:24<01:07, 10.8MB/s]
56%|#####################4 | 932M/1.65G [01:25<00:55, 12.9MB/s]
56%|#####################4 | 934M/1.65G [01:25<00:56, 12.8MB/s]
57%|#####################4 | 935M/1.65G [01:25<01:08, 10.5MB/s]
57%|#####################5 | 936M/1.65G [01:25<01:09, 10.4MB/s]
57%|#####################5 | 939M/1.65G [01:25<00:51, 13.9MB/s]
57%|#####################6 | 940M/1.65G [01:25<01:00, 11.8MB/s]
57%|#####################6 | 942M/1.65G [01:25<01:02, 11.4MB/s]
57%|#####################7 | 944M/1.65G [01:25<00:50, 14.0MB/s]
57%|#####################7 | 945M/1.65G [01:26<00:52, 13.6MB/s]
57%|#####################7 | 947M/1.65G [01:26<01:12, 9.80MB/s]
57%|#####################8 | 948M/1.65G [01:26<01:05, 10.8MB/s]
57%|#####################8 | 950M/1.65G [01:26<01:22, 8.49MB/s]
58%|#####################8 | 951M/1.65G [01:26<01:13, 9.61MB/s]
58%|#####################8 | 952M/1.65G [01:26<01:08, 10.2MB/s]
58%|#####################9 | 954M/1.65G [01:27<01:05, 10.7MB/s]
58%|#####################9 | 955M/1.65G [01:27<01:16, 9.18MB/s]
58%|#####################9 | 956M/1.65G [01:27<01:14, 9.32MB/s]
58%|###################### | 959M/1.65G [01:27<00:52, 13.3MB/s]
58%|###################### | 960M/1.65G [01:27<00:52, 13.1MB/s]
58%|######################1 | 962M/1.65G [01:27<01:19, 8.65MB/s]
58%|######################1 | 963M/1.65G [01:27<01:18, 8.78MB/s]
58%|######################1 | 964M/1.65G [01:28<01:23, 8.28MB/s]
58%|######################2 | 966M/1.65G [01:28<01:04, 10.7MB/s]
59%|######################2 | 967M/1.65G [01:28<01:01, 11.1MB/s]
59%|######################2 | 968M/1.65G [01:28<00:59, 11.4MB/s]
59%|######################2 | 970M/1.65G [01:28<01:13, 9.31MB/s]
59%|######################3 | 972M/1.65G [01:28<00:53, 12.8MB/s]
59%|######################3 | 974M/1.65G [01:28<01:01, 11.0MB/s]
59%|######################4 | 975M/1.65G [01:29<01:00, 11.1MB/s]
59%|######################4 | 976M/1.65G [01:29<01:04, 10.5MB/s]
59%|######################4 | 978M/1.65G [01:29<01:00, 11.2MB/s]
59%|######################5 | 980M/1.65G [01:29<00:49, 13.5MB/s]
59%|######################5 | 981M/1.65G [01:29<00:55, 12.1MB/s]
59%|######################5 | 982M/1.65G [01:29<01:04, 10.3MB/s]
60%|######################6 | 984M/1.65G [01:29<01:01, 10.8MB/s]
60%|######################6 | 985M/1.65G [01:29<01:10, 9.52MB/s]
60%|######################6 | 987M/1.65G [01:30<00:56, 11.8MB/s]
60%|######################7 | 988M/1.65G [01:30<00:53, 12.4MB/s]
60%|######################7 | 989M/1.65G [01:30<00:53, 12.4MB/s]
60%|######################7 | 991M/1.65G [01:30<00:53, 12.4MB/s]
60%|######################8 | 992M/1.65G [01:30<00:53, 12.4MB/s]
60%|######################8 | 993M/1.65G [01:30<00:53, 12.4MB/s]
60%|######################8 | 995M/1.65G [01:30<01:07, 9.77MB/s]
60%|######################8 | 996M/1.65G [01:30<01:04, 10.2MB/s]
60%|######################9 | 998M/1.65G [01:30<00:46, 14.0MB/s]
60%|######################3 | 1.00G/1.65G [01:31<01:02, 10.4MB/s]
61%|######################4 | 1.00G/1.65G [01:31<01:03, 10.2MB/s]
61%|######################4 | 1.00G/1.65G [01:31<00:48, 13.4MB/s]
61%|######################4 | 1.00G/1.65G [01:31<00:49, 13.1MB/s]
61%|######################5 | 1.01G/1.65G [01:31<00:50, 12.9MB/s]
61%|######################5 | 1.01G/1.65G [01:31<00:50, 12.8MB/s]
61%|######################5 | 1.01G/1.65G [01:31<00:50, 12.6MB/s]
61%|######################6 | 1.01G/1.65G [01:32<00:51, 12.6MB/s]
61%|######################6 | 1.01G/1.65G [01:32<01:22, 7.73MB/s]
61%|######################6 | 1.01G/1.65G [01:32<01:12, 8.83MB/s]
61%|######################7 | 1.01G/1.65G [01:32<01:20, 7.94MB/s]
62%|######################7 | 1.02G/1.65G [01:32<00:53, 11.9MB/s]
62%|######################7 | 1.02G/1.65G [01:32<01:02, 10.1MB/s]
62%|######################8 | 1.02G/1.65G [01:33<00:52, 12.0MB/s]
62%|######################8 | 1.02G/1.65G [01:33<00:58, 10.8MB/s]
62%|######################9 | 1.02G/1.65G [01:33<00:46, 13.5MB/s]
62%|######################9 | 1.03G/1.65G [01:33<00:52, 12.0MB/s]
62%|######################9 | 1.03G/1.65G [01:33<00:51, 12.1MB/s]
62%|####################### | 1.03G/1.65G [01:33<00:58, 10.7MB/s]
62%|####################### | 1.03G/1.65G [01:33<00:55, 11.3MB/s]
62%|####################### | 1.03G/1.65G [01:33<00:50, 12.3MB/s]
63%|#######################1 | 1.03G/1.65G [01:34<00:42, 14.4MB/s]
63%|#######################1 | 1.03G/1.65G [01:34<00:57, 10.7MB/s]
63%|#######################1 | 1.04G/1.65G [01:34<00:56, 10.8MB/s]
63%|#######################2 | 1.04G/1.65G [01:34<00:41, 14.8MB/s]
63%|#######################2 | 1.04G/1.65G [01:34<00:59, 10.3MB/s]
63%|#######################3 | 1.04G/1.65G [01:34<00:52, 11.7MB/s]
63%|#######################3 | 1.04G/1.65G [01:35<00:55, 11.0MB/s]
63%|#######################4 | 1.05G/1.65G [01:35<00:53, 11.3MB/s]
63%|#######################4 | 1.05G/1.65G [01:35<00:52, 11.5MB/s]
63%|#######################4 | 1.05G/1.65G [01:35<00:51, 11.8MB/s]
63%|#######################4 | 1.05G/1.65G [01:35<00:50, 11.9MB/s]
64%|#######################5 | 1.05G/1.65G [01:35<01:02, 9.62MB/s]
64%|#######################5 | 1.05G/1.65G [01:35<01:13, 8.23MB/s]
64%|#######################5 | 1.05G/1.65G [01:36<01:09, 8.69MB/s]
64%|#######################5 | 1.05G/1.65G [01:36<00:58, 10.2MB/s]
64%|#######################6 | 1.06G/1.65G [01:36<00:55, 10.8MB/s]
64%|#######################6 | 1.06G/1.65G [01:36<00:53, 11.2MB/s]
64%|#######################6 | 1.06G/1.65G [01:36<01:00, 9.88MB/s]
64%|#######################7 | 1.06G/1.65G [01:36<00:47, 12.4MB/s]
64%|#######################7 | 1.06G/1.65G [01:36<00:47, 12.4MB/s]
64%|#######################7 | 1.06G/1.65G [01:36<00:47, 12.4MB/s]
64%|#######################8 | 1.06G/1.65G [01:36<00:47, 12.4MB/s]
64%|#######################8 | 1.06G/1.65G [01:36<00:47, 12.3MB/s]
65%|#######################8 | 1.07G/1.65G [01:37<00:47, 12.3MB/s]
65%|#######################8 | 1.07G/1.65G [01:37<00:56, 10.4MB/s]
65%|#######################9 | 1.07G/1.65G [01:37<00:50, 11.5MB/s]
65%|#######################9 | 1.07G/1.65G [01:37<00:44, 13.1MB/s]
65%|#######################9 | 1.07G/1.65G [01:37<00:48, 12.0MB/s]
65%|######################## | 1.07G/1.65G [01:37<00:47, 12.2MB/s]
65%|######################## | 1.07G/1.65G [01:37<00:49, 11.7MB/s]
65%|######################## | 1.08G/1.65G [01:37<00:56, 10.3MB/s]
65%|########################1 | 1.08G/1.65G [01:38<00:44, 13.0MB/s]
65%|########################1 | 1.08G/1.65G [01:38<00:49, 11.6MB/s]
65%|########################2 | 1.08G/1.65G [01:38<00:42, 13.4MB/s]
65%|########################2 | 1.08G/1.65G [01:38<00:43, 13.1MB/s]
66%|########################2 | 1.08G/1.65G [01:38<00:55, 10.2MB/s]
66%|########################3 | 1.09G/1.65G [01:38<00:48, 11.6MB/s]
66%|########################3 | 1.09G/1.65G [01:38<00:55, 10.2MB/s]
66%|########################3 | 1.09G/1.65G [01:39<00:38, 14.4MB/s]
66%|########################4 | 1.09G/1.65G [01:39<00:40, 13.9MB/s]
66%|########################4 | 1.09G/1.65G [01:39<01:01, 9.07MB/s]
66%|########################4 | 1.09G/1.65G [01:39<01:02, 8.89MB/s]
66%|########################5 | 1.10G/1.65G [01:39<01:09, 8.04MB/s]
66%|########################5 | 1.10G/1.65G [01:39<00:49, 11.2MB/s]
66%|########################6 | 1.10G/1.65G [01:40<01:07, 8.23MB/s]
67%|########################6 | 1.10G/1.65G [01:40<01:11, 7.74MB/s]
67%|########################6 | 1.10G/1.65G [01:40<01:03, 8.69MB/s]
67%|########################6 | 1.10G/1.65G [01:40<00:58, 9.46MB/s]
67%|########################7 | 1.10G/1.65G [01:40<00:54, 10.1MB/s]
67%|########################7 | 1.11G/1.65G [01:40<00:51, 10.7MB/s]
67%|########################7 | 1.11G/1.65G [01:40<01:02, 8.77MB/s]
67%|########################8 | 1.11G/1.65G [01:41<00:43, 12.5MB/s]
67%|########################8 | 1.11G/1.65G [01:41<00:43, 12.5MB/s]
67%|########################8 | 1.11G/1.65G [01:41<00:48, 11.2MB/s]
67%|########################9 | 1.11G/1.65G [01:41<00:51, 10.4MB/s]
67%|########################9 | 1.12G/1.65G [01:41<00:39, 13.4MB/s]
68%|######################### | 1.12G/1.65G [01:41<00:45, 11.7MB/s]
68%|######################### | 1.12G/1.65G [01:41<00:51, 10.4MB/s]
68%|######################### | 1.12G/1.65G [01:42<00:46, 11.4MB/s]
68%|#########################1 | 1.12G/1.65G [01:42<00:37, 14.2MB/s]
68%|#########################1 | 1.12G/1.65G [01:42<00:38, 13.7MB/s]
68%|#########################1 | 1.13G/1.65G [01:42<00:49, 10.7MB/s]
68%|#########################2 | 1.13G/1.65G [01:42<00:38, 13.8MB/s]
68%|#########################2 | 1.13G/1.65G [01:42<00:39, 13.4MB/s]
68%|#########################3 | 1.13G/1.65G [01:43<00:56, 9.29MB/s]
68%|#########################3 | 1.13G/1.65G [01:43<01:04, 8.06MB/s]
69%|#########################3 | 1.13G/1.65G [01:43<01:05, 7.90MB/s]
69%|#########################3 | 1.13G/1.65G [01:43<01:10, 7.40MB/s]
69%|#########################4 | 1.14G/1.65G [01:43<00:49, 10.4MB/s]
69%|#########################4 | 1.14G/1.65G [01:43<00:47, 10.9MB/s]
69%|#########################4 | 1.14G/1.65G [01:43<00:59, 8.68MB/s]
69%|#########################5 | 1.14G/1.65G [01:44<00:45, 11.2MB/s]
69%|#########################5 | 1.14G/1.65G [01:44<00:50, 10.2MB/s]
69%|#########################6 | 1.14G/1.65G [01:44<00:48, 10.4MB/s]
69%|#########################6 | 1.15G/1.65G [01:44<00:43, 11.7MB/s]
69%|#########################6 | 1.15G/1.65G [01:44<00:53, 9.48MB/s]
70%|#########################7 | 1.15G/1.65G [01:44<00:45, 11.0MB/s]
70%|#########################7 | 1.15G/1.65G [01:44<00:44, 11.3MB/s]
70%|#########################7 | 1.15G/1.65G [01:45<00:37, 13.4MB/s]
70%|#########################8 | 1.15G/1.65G [01:45<00:40, 12.4MB/s]
70%|#########################8 | 1.16G/1.65G [01:45<00:38, 13.1MB/s]
70%|#########################8 | 1.16G/1.65G [01:45<00:38, 13.0MB/s]
70%|#########################9 | 1.16G/1.65G [01:45<00:38, 12.9MB/s]
70%|#########################9 | 1.16G/1.65G [01:45<01:00, 8.13MB/s]
70%|#########################9 | 1.16G/1.65G [01:46<01:00, 8.10MB/s]
70%|########################## | 1.16G/1.65G [01:46<00:45, 10.8MB/s]
70%|########################## | 1.16G/1.65G [01:46<00:44, 11.1MB/s]
71%|########################## | 1.17G/1.65G [01:46<00:42, 11.6MB/s]
71%|##########################1 | 1.17G/1.65G [01:46<01:01, 7.84MB/s]
71%|##########################1 | 1.17G/1.65G [01:46<01:01, 7.92MB/s]
71%|##########################1 | 1.17G/1.65G [01:46<00:55, 8.74MB/s]
71%|##########################1 | 1.17G/1.65G [01:46<00:55, 8.71MB/s]
71%|##########################2 | 1.17G/1.65G [01:47<00:47, 10.2MB/s]
71%|##########################2 | 1.17G/1.65G [01:47<00:44, 10.8MB/s]
71%|##########################2 | 1.17G/1.65G [01:47<00:43, 11.1MB/s]
71%|##########################3 | 1.18G/1.65G [01:47<00:51, 9.33MB/s]
71%|##########################3 | 1.18G/1.65G [01:47<00:37, 12.6MB/s]
71%|##########################3 | 1.18G/1.65G [01:47<00:37, 12.6MB/s]
71%|##########################4 | 1.18G/1.65G [01:47<00:54, 8.66MB/s]
71%|##########################4 | 1.18G/1.65G [01:48<00:52, 8.90MB/s]
72%|##########################4 | 1.18G/1.65G [01:48<00:41, 11.2MB/s]
72%|##########################5 | 1.18G/1.65G [01:48<00:49, 9.42MB/s]
72%|##########################5 | 1.19G/1.65G [01:48<00:36, 12.6MB/s]
72%|##########################6 | 1.19G/1.65G [01:48<00:37, 12.5MB/s]
72%|##########################6 | 1.19G/1.65G [01:48<00:36, 12.5MB/s]
72%|##########################6 | 1.19G/1.65G [01:48<00:37, 12.4MB/s]
72%|##########################7 | 1.19G/1.65G [01:49<00:54, 8.48MB/s]
72%|##########################7 | 1.19G/1.65G [01:49<01:00, 7.61MB/s]
72%|##########################7 | 1.20G/1.65G [01:49<00:52, 8.71MB/s]
72%|##########################7 | 1.20G/1.65G [01:49<00:46, 9.71MB/s]
72%|##########################8 | 1.20G/1.65G [01:49<00:44, 10.2MB/s]
73%|##########################8 | 1.20G/1.65G [01:49<00:53, 8.45MB/s]
73%|##########################9 | 1.20G/1.65G [01:49<00:35, 12.6MB/s]
73%|##########################9 | 1.20G/1.65G [01:50<00:50, 8.86MB/s]
73%|##########################9 | 1.20G/1.65G [01:50<01:00, 7.41MB/s]
73%|##########################9 | 1.21G/1.65G [01:50<00:54, 8.28MB/s]
73%|########################### | 1.21G/1.65G [01:50<00:48, 9.11MB/s]
73%|########################### | 1.21G/1.65G [01:50<00:45, 9.85MB/s]
73%|########################### | 1.21G/1.65G [01:50<00:43, 10.2MB/s]
73%|###########################1 | 1.21G/1.65G [01:50<00:39, 11.1MB/s]
73%|###########################1 | 1.21G/1.65G [01:51<00:38, 11.4MB/s]
73%|###########################1 | 1.21G/1.65G [01:51<00:37, 11.7MB/s]
73%|###########################1 | 1.21G/1.65G [01:51<00:36, 11.9MB/s]
74%|###########################2 | 1.22G/1.65G [01:51<00:36, 12.0MB/s]
74%|###########################2 | 1.22G/1.65G [01:51<00:35, 12.1MB/s]
74%|###########################2 | 1.22G/1.65G [01:51<00:35, 12.2MB/s]
74%|###########################2 | 1.22G/1.65G [01:51<00:46, 9.23MB/s]
74%|###########################3 | 1.22G/1.65G [01:51<00:41, 10.5MB/s]
74%|###########################3 | 1.22G/1.65G [01:51<00:30, 14.0MB/s]
74%|###########################4 | 1.22G/1.65G [01:52<00:39, 10.8MB/s]
74%|###########################4 | 1.23G/1.65G [01:52<00:45, 9.28MB/s]
74%|###########################4 | 1.23G/1.65G [01:52<00:42, 9.94MB/s]
74%|###########################5 | 1.23G/1.65G [01:52<00:40, 10.5MB/s]
74%|###########################5 | 1.23G/1.65G [01:52<00:38, 11.0MB/s]
74%|###########################5 | 1.23G/1.65G [01:52<00:37, 11.4MB/s]
75%|###########################5 | 1.23G/1.65G [01:52<00:44, 9.55MB/s]
75%|###########################6 | 1.23G/1.65G [01:53<00:38, 11.0MB/s]
75%|###########################6 | 1.24G/1.65G [01:53<00:32, 12.7MB/s]
75%|###########################6 | 1.24G/1.65G [01:53<00:33, 12.6MB/s]
75%|###########################7 | 1.24G/1.65G [01:53<00:38, 10.6MB/s]
75%|###########################7 | 1.24G/1.65G [01:53<00:38, 10.7MB/s]
75%|###########################7 | 1.24G/1.65G [01:53<00:30, 13.4MB/s]
75%|###########################8 | 1.24G/1.65G [01:53<00:34, 11.9MB/s]
75%|###########################8 | 1.24G/1.65G [01:53<00:37, 10.8MB/s]
75%|###########################9 | 1.25G/1.65G [01:54<00:29, 13.7MB/s]
76%|###########################9 | 1.25G/1.65G [01:54<00:33, 12.1MB/s]
76%|###########################9 | 1.25G/1.65G [01:54<00:30, 13.1MB/s]
76%|############################ | 1.25G/1.65G [01:54<00:36, 11.0MB/s]
76%|############################ | 1.25G/1.65G [01:54<00:29, 13.5MB/s]
76%|############################ | 1.25G/1.65G [01:54<00:36, 10.8MB/s]
76%|############################1 | 1.26G/1.65G [01:55<00:36, 11.0MB/s]
76%|############################1 | 1.26G/1.65G [01:55<00:28, 13.9MB/s]
76%|############################2 | 1.26G/1.65G [01:55<00:29, 13.5MB/s]
76%|############################2 | 1.26G/1.65G [01:55<00:33, 11.7MB/s]
77%|############################3 | 1.26G/1.65G [01:55<00:28, 13.4MB/s]
77%|############################3 | 1.27G/1.65G [01:55<00:32, 12.0MB/s]
77%|############################3 | 1.27G/1.65G [01:55<00:29, 13.2MB/s]
77%|############################4 | 1.27G/1.65G [01:55<00:29, 13.0MB/s]
77%|############################4 | 1.27G/1.65G [01:56<00:29, 12.8MB/s]
77%|############################4 | 1.27G/1.65G [01:56<00:37, 10.1MB/s]
77%|############################5 | 1.27G/1.65G [01:56<00:37, 10.1MB/s]
77%|############################5 | 1.27G/1.65G [01:56<00:43, 8.77MB/s]
77%|############################5 | 1.28G/1.65G [01:56<00:35, 10.5MB/s]
77%|############################6 | 1.28G/1.65G [01:56<00:28, 13.1MB/s]
77%|############################6 | 1.28G/1.65G [01:56<00:27, 13.4MB/s]
78%|############################6 | 1.28G/1.65G [01:56<00:28, 13.1MB/s]
78%|############################7 | 1.28G/1.65G [01:57<00:33, 11.0MB/s]
78%|############################7 | 1.28G/1.65G [01:57<00:31, 11.8MB/s]
78%|############################7 | 1.29G/1.65G [01:57<00:28, 12.7MB/s]
78%|############################8 | 1.29G/1.65G [01:57<00:31, 11.5MB/s]
78%|############################8 | 1.29G/1.65G [01:57<00:32, 11.3MB/s]
78%|############################8 | 1.29G/1.65G [01:57<00:27, 13.1MB/s]
78%|############################9 | 1.29G/1.65G [01:57<00:27, 12.9MB/s]
78%|############################9 | 1.29G/1.65G [01:57<00:28, 12.8MB/s]
78%|############################9 | 1.29G/1.65G [01:58<00:28, 12.6MB/s]
78%|############################9 | 1.30G/1.65G [01:58<00:28, 12.5MB/s]
78%|############################# | 1.30G/1.65G [01:58<00:28, 12.5MB/s]
79%|############################# | 1.30G/1.65G [01:58<00:28, 12.5MB/s]
79%|############################# | 1.30G/1.65G [01:58<00:37, 9.34MB/s]
79%|#############################1 | 1.30G/1.65G [01:58<00:26, 13.4MB/s]
79%|#############################1 | 1.30G/1.65G [01:58<00:34, 10.2MB/s]
79%|#############################2 | 1.30G/1.65G [01:58<00:32, 10.8MB/s]
79%|#############################2 | 1.31G/1.65G [01:59<00:34, 9.97MB/s]
79%|#############################2 | 1.31G/1.65G [01:59<00:30, 11.4MB/s]
79%|#############################3 | 1.31G/1.65G [01:59<00:26, 13.0MB/s]
79%|#############################3 | 1.31G/1.65G [01:59<00:26, 12.9MB/s]
79%|#############################3 | 1.31G/1.65G [01:59<00:22, 15.1MB/s]
80%|#############################4 | 1.31G/1.65G [01:59<00:23, 14.2MB/s]
80%|#############################4 | 1.32G/1.65G [01:59<00:34, 9.75MB/s]
80%|#############################4 | 1.32G/1.65G [02:00<00:33, 10.1MB/s]
80%|#############################5 | 1.32G/1.65G [02:00<00:38, 8.79MB/s]
80%|#############################5 | 1.32G/1.65G [02:00<00:32, 10.2MB/s]
80%|#############################5 | 1.32G/1.65G [02:00<00:26, 12.4MB/s]
80%|#############################6 | 1.32G/1.65G [02:00<00:26, 12.3MB/s]
80%|#############################6 | 1.32G/1.65G [02:00<00:33, 9.85MB/s]
80%|#############################6 | 1.33G/1.65G [02:00<00:32, 10.1MB/s]
80%|#############################7 | 1.33G/1.65G [02:01<00:35, 9.23MB/s]
80%|#############################7 | 1.33G/1.65G [02:01<00:37, 8.70MB/s]
81%|#############################7 | 1.33G/1.65G [02:01<00:29, 10.8MB/s]
81%|#############################8 | 1.33G/1.65G [02:01<00:28, 11.2MB/s]
81%|#############################8 | 1.33G/1.65G [02:01<00:35, 9.11MB/s]
81%|#############################8 | 1.33G/1.65G [02:01<00:31, 10.2MB/s]
81%|#############################9 | 1.34G/1.65G [02:01<00:32, 9.66MB/s]
81%|#############################9 | 1.34G/1.65G [02:02<00:37, 8.45MB/s]
81%|#############################9 | 1.34G/1.65G [02:02<00:27, 11.4MB/s]
81%|############################## | 1.34G/1.65G [02:02<00:31, 9.79MB/s]
81%|############################## | 1.34G/1.65G [02:02<00:25, 12.0MB/s]
81%|############################## | 1.34G/1.65G [02:02<00:25, 12.2MB/s]
81%|##############################1 | 1.35G/1.65G [02:02<00:27, 11.1MB/s]
81%|##############################1 | 1.35G/1.65G [02:02<00:31, 9.82MB/s]
82%|##############################1 | 1.35G/1.65G [02:03<00:28, 10.7MB/s]
82%|##############################2 | 1.35G/1.65G [02:03<00:24, 12.4MB/s]
82%|##############################2 | 1.35G/1.65G [02:03<00:22, 13.4MB/s]
82%|##############################2 | 1.35G/1.65G [02:03<00:21, 14.0MB/s]
82%|##############################3 | 1.35G/1.65G [02:03<00:22, 13.5MB/s]
82%|##############################3 | 1.36G/1.65G [02:03<00:22, 13.2MB/s]
82%|##############################3 | 1.36G/1.65G [02:03<00:34, 8.66MB/s]
82%|##############################4 | 1.36G/1.65G [02:04<00:26, 10.9MB/s]
82%|##############################4 | 1.36G/1.65G [02:04<00:29, 9.94MB/s]
82%|##############################4 | 1.36G/1.65G [02:04<00:29, 9.94MB/s]
83%|##############################5 | 1.36G/1.65G [02:04<00:27, 10.5MB/s]
83%|##############################5 | 1.37G/1.65G [02:04<00:25, 11.3MB/s]
83%|##############################6 | 1.37G/1.65G [02:04<00:20, 13.7MB/s]
83%|##############################6 | 1.37G/1.65G [02:04<00:21, 13.4MB/s]
83%|##############################6 | 1.37G/1.65G [02:04<00:21, 13.0MB/s]
83%|##############################7 | 1.37G/1.65G [02:05<00:21, 12.9MB/s]
83%|##############################7 | 1.37G/1.65G [02:05<00:21, 12.8MB/s]
83%|##############################7 | 1.37G/1.65G [02:05<00:27, 10.2MB/s]
83%|##############################8 | 1.38G/1.65G [02:05<00:20, 13.3MB/s]
83%|##############################8 | 1.38G/1.65G [02:05<00:21, 12.5MB/s]
84%|##############################8 | 1.38G/1.65G [02:05<00:20, 13.0MB/s]
84%|##############################9 | 1.38G/1.65G [02:05<00:22, 11.9MB/s]
84%|##############################9 | 1.38G/1.65G [02:05<00:22, 11.8MB/s]
84%|##############################9 | 1.38G/1.65G [02:06<00:20, 13.2MB/s]
84%|############################### | 1.39G/1.65G [02:06<00:20, 13.0MB/s]
84%|############################### | 1.39G/1.65G [02:06<00:20, 12.8MB/s]
84%|############################### | 1.39G/1.65G [02:06<00:20, 12.7MB/s]
84%|###############################1 | 1.39G/1.65G [02:06<00:25, 10.2MB/s]
84%|###############################1 | 1.39G/1.65G [02:06<00:25, 10.2MB/s]
84%|###############################1 | 1.39G/1.65G [02:06<00:20, 12.7MB/s]
84%|###############################2 | 1.39G/1.65G [02:06<00:22, 11.7MB/s]
84%|###############################2 | 1.40G/1.65G [02:07<00:23, 11.1MB/s]
85%|###############################2 | 1.40G/1.65G [02:07<00:17, 14.5MB/s]
85%|###############################3 | 1.40G/1.65G [02:07<00:18, 13.8MB/s]
85%|###############################3 | 1.40G/1.65G [02:07<00:22, 11.4MB/s]
85%|###############################4 | 1.40G/1.65G [02:07<00:18, 13.6MB/s]
85%|###############################4 | 1.40G/1.65G [02:07<00:18, 13.3MB/s]
85%|###############################4 | 1.41G/1.65G [02:07<00:23, 10.4MB/s]
85%|###############################5 | 1.41G/1.65G [02:08<00:22, 11.1MB/s]
85%|###############################5 | 1.41G/1.65G [02:08<00:17, 13.6MB/s]
85%|###############################6 | 1.41G/1.65G [02:08<00:21, 11.4MB/s]
86%|###############################6 | 1.41G/1.65G [02:08<00:17, 13.6MB/s]
86%|###############################6 | 1.42G/1.65G [02:08<00:20, 11.6MB/s]
86%|###############################7 | 1.42G/1.65G [02:08<00:17, 13.2MB/s]
86%|###############################7 | 1.42G/1.65G [02:08<00:18, 12.7MB/s]
86%|###############################7 | 1.42G/1.65G [02:09<00:21, 11.1MB/s]
86%|###############################8 | 1.42G/1.65G [02:09<00:17, 13.3MB/s]
86%|###############################8 | 1.42G/1.65G [02:09<00:18, 12.1MB/s]
86%|###############################9 | 1.43G/1.65G [02:09<00:17, 13.2MB/s]
86%|###############################9 | 1.43G/1.65G [02:09<00:17, 12.8MB/s]
86%|###############################9 | 1.43G/1.65G [02:09<00:17, 12.7MB/s]
86%|################################ | 1.43G/1.65G [02:09<00:17, 12.6MB/s]
87%|################################ | 1.43G/1.65G [02:09<00:21, 10.2MB/s]
87%|################################ | 1.43G/1.65G [02:10<00:23, 9.49MB/s]
87%|################################ | 1.43G/1.65G [02:10<00:26, 8.21MB/s]
87%|################################1 | 1.44G/1.65G [02:10<00:18, 11.7MB/s]
87%|################################1 | 1.44G/1.65G [02:10<00:18, 11.9MB/s]
87%|################################1 | 1.44G/1.65G [02:10<00:17, 12.0MB/s]
87%|################################2 | 1.44G/1.65G [02:10<00:17, 12.2MB/s]
87%|################################2 | 1.44G/1.65G [02:10<00:17, 12.2MB/s]
87%|################################2 | 1.44G/1.65G [02:10<00:17, 12.3MB/s]
87%|################################3 | 1.44G/1.65G [02:10<00:17, 12.3MB/s]
87%|################################3 | 1.44G/1.65G [02:11<00:16, 12.3MB/s]
87%|################################3 | 1.45G/1.65G [02:11<00:17, 12.1MB/s]
88%|################################3 | 1.45G/1.65G [02:11<00:16, 12.4MB/s]
88%|################################4 | 1.45G/1.65G [02:11<00:26, 7.75MB/s]
88%|################################4 | 1.45G/1.65G [02:11<00:17, 11.5MB/s]
88%|################################5 | 1.45G/1.65G [02:11<00:17, 11.2MB/s]
88%|################################5 | 1.45G/1.65G [02:11<00:16, 11.7MB/s]
88%|################################5 | 1.46G/1.65G [02:12<00:16, 11.8MB/s]
88%|################################6 | 1.46G/1.65G [02:12<00:18, 10.5MB/s]
88%|################################6 | 1.46G/1.65G [02:12<00:18, 10.5MB/s]
88%|################################7 | 1.46G/1.65G [02:12<00:17, 11.3MB/s]
89%|################################7 | 1.46G/1.65G [02:12<00:13, 14.3MB/s]
89%|################################8 | 1.47G/1.65G [02:12<00:13, 13.8MB/s]
89%|################################8 | 1.47G/1.65G [02:13<00:17, 10.8MB/s]
89%|################################8 | 1.47G/1.65G [02:13<00:18, 10.1MB/s]
89%|################################8 | 1.47G/1.65G [02:13<00:17, 10.3MB/s]
89%|################################9 | 1.47G/1.65G [02:13<00:17, 10.6MB/s]
89%|################################9 | 1.47G/1.65G [02:13<00:13, 13.2MB/s]
89%|################################# | 1.47G/1.65G [02:13<00:13, 13.0MB/s]
89%|################################# | 1.48G/1.65G [02:13<00:13, 12.8MB/s]
89%|################################# | 1.48G/1.65G [02:13<00:13, 12.7MB/s]
89%|#################################1 | 1.48G/1.65G [02:14<00:18, 9.39MB/s]
90%|#################################1 | 1.48G/1.65G [02:14<00:14, 12.1MB/s]
90%|#################################1 | 1.48G/1.65G [02:14<00:13, 12.2MB/s]
90%|#################################2 | 1.48G/1.65G [02:14<00:16, 10.4MB/s]
90%|#################################2 | 1.49G/1.65G [02:14<00:12, 12.9MB/s]
90%|#################################3 | 1.49G/1.65G [02:14<00:12, 12.8MB/s]
90%|#################################3 | 1.49G/1.65G [02:14<00:12, 12.7MB/s]
90%|#################################3 | 1.49G/1.65G [02:14<00:12, 12.6MB/s]
90%|#################################3 | 1.49G/1.65G [02:15<00:12, 12.5MB/s]
90%|#################################4 | 1.49G/1.65G [02:15<00:14, 11.0MB/s]
90%|#################################4 | 1.49G/1.65G [02:15<00:12, 12.9MB/s]
91%|#################################4 | 1.50G/1.65G [02:15<00:12, 12.8MB/s]
91%|#################################5 | 1.50G/1.65G [02:15<00:13, 11.4MB/s]
91%|#################################5 | 1.50G/1.65G [02:15<00:11, 12.9MB/s]
91%|#################################5 | 1.50G/1.65G [02:15<00:12, 12.0MB/s]
91%|#################################6 | 1.50G/1.65G [02:15<00:11, 12.9MB/s]
91%|#################################6 | 1.50G/1.65G [02:16<00:13, 11.3MB/s]
91%|#################################6 | 1.50G/1.65G [02:16<00:13, 10.6MB/s]
91%|#################################7 | 1.51G/1.65G [02:16<00:13, 11.1MB/s]
91%|#################################7 | 1.51G/1.65G [02:16<00:10, 14.0MB/s]
91%|#################################8 | 1.51G/1.65G [02:16<00:12, 11.8MB/s]
91%|#################################8 | 1.51G/1.65G [02:16<00:12, 11.4MB/s]
92%|#################################8 | 1.51G/1.65G [02:16<00:09, 14.0MB/s]
92%|#################################9 | 1.51G/1.65G [02:16<00:10, 13.5MB/s]
92%|#################################9 | 1.52G/1.65G [02:17<00:10, 13.2MB/s]
92%|#################################9 | 1.52G/1.65G [02:17<00:13, 9.76MB/s]
92%|################################## | 1.52G/1.65G [02:17<00:11, 11.8MB/s]
92%|################################## | 1.52G/1.65G [02:17<00:10, 12.0MB/s]
92%|################################## | 1.52G/1.65G [02:17<00:13, 9.92MB/s]
92%|##################################1 | 1.52G/1.65G [02:17<00:12, 10.4MB/s]
92%|##################################1 | 1.53G/1.65G [02:18<00:11, 10.8MB/s]
92%|##################################2 | 1.53G/1.65G [02:18<00:10, 11.8MB/s]
93%|##################################2 | 1.53G/1.65G [02:18<00:10, 12.3MB/s]
93%|##################################2 | 1.53G/1.65G [02:18<00:10, 11.3MB/s]
93%|##################################3 | 1.53G/1.65G [02:18<00:08, 14.3MB/s]
93%|##################################3 | 1.53G/1.65G [02:18<00:08, 13.8MB/s]
93%|##################################3 | 1.54G/1.65G [02:18<00:08, 13.4MB/s]
93%|##################################4 | 1.54G/1.65G [02:18<00:10, 11.2MB/s]
93%|##################################4 | 1.54G/1.65G [02:19<00:08, 13.4MB/s]
93%|##################################5 | 1.54G/1.65G [02:19<00:08, 13.1MB/s]
93%|##################################5 | 1.54G/1.65G [02:19<00:09, 11.0MB/s]
93%|##################################5 | 1.54G/1.65G [02:19<00:11, 9.77MB/s]
93%|##################################5 | 1.55G/1.65G [02:19<00:10, 10.4MB/s]
94%|##################################6 | 1.55G/1.65G [02:19<00:09, 10.8MB/s]
94%|##################################6 | 1.55G/1.65G [02:19<00:11, 9.18MB/s]
94%|##################################6 | 1.55G/1.65G [02:20<00:10, 10.0MB/s]
94%|##################################7 | 1.55G/1.65G [02:20<00:08, 11.4MB/s]
94%|##################################7 | 1.55G/1.65G [02:20<00:08, 11.1MB/s]
94%|##################################8 | 1.56G/1.65G [02:20<00:06, 13.9MB/s]
94%|##################################8 | 1.56G/1.65G [02:20<00:07, 13.5MB/s]
94%|##################################8 | 1.56G/1.65G [02:20<00:07, 13.2MB/s]
94%|##################################9 | 1.56G/1.65G [02:20<00:07, 13.0MB/s]
94%|##################################9 | 1.56G/1.65G [02:20<00:07, 12.8MB/s]
95%|##################################9 | 1.56G/1.65G [02:21<00:07, 12.7MB/s]
95%|################################### | 1.56G/1.65G [02:21<00:07, 12.6MB/s]
95%|################################### | 1.57G/1.65G [02:21<00:09, 8.76MB/s]
95%|################################### | 1.57G/1.65G [02:21<00:10, 8.36MB/s]
95%|###################################1 | 1.57G/1.65G [02:21<00:07, 10.7MB/s]
95%|###################################1 | 1.57G/1.65G [02:21<00:08, 9.54MB/s]
95%|###################################1 | 1.57G/1.65G [02:22<00:08, 10.1MB/s]
95%|###################################2 | 1.57G/1.65G [02:22<00:06, 12.9MB/s]
95%|###################################2 | 1.58G/1.65G [02:22<00:06, 11.7MB/s]
95%|###################################2 | 1.58G/1.65G [02:22<00:06, 11.6MB/s]
96%|###################################3 | 1.58G/1.65G [02:22<00:05, 13.3MB/s]
96%|###################################3 | 1.58G/1.65G [02:22<00:05, 12.5MB/s]
96%|###################################4 | 1.58G/1.65G [02:22<00:05, 13.0MB/s]
96%|###################################4 | 1.58G/1.65G [02:22<00:05, 12.9MB/s]
96%|###################################4 | 1.58G/1.65G [02:22<00:05, 12.7MB/s]
96%|###################################4 | 1.59G/1.65G [02:23<00:05, 12.6MB/s]
96%|###################################5 | 1.59G/1.65G [02:23<00:05, 12.5MB/s]
96%|###################################5 | 1.59G/1.65G [02:23<00:05, 12.5MB/s]
96%|###################################5 | 1.59G/1.65G [02:23<00:05, 12.5MB/s]
96%|###################################6 | 1.59G/1.65G [02:23<00:04, 12.5MB/s]
96%|###################################6 | 1.59G/1.65G [02:23<00:05, 10.4MB/s]
96%|###################################6 | 1.59G/1.65G [02:23<00:04, 13.0MB/s]
97%|###################################7 | 1.60G/1.65G [02:23<00:05, 10.4MB/s]
97%|###################################7 | 1.60G/1.65G [02:24<00:04, 13.4MB/s]
97%|###################################7 | 1.60G/1.65G [02:24<00:05, 10.7MB/s]
97%|###################################8 | 1.60G/1.65G [02:24<00:04, 11.0MB/s]
97%|###################################8 | 1.60G/1.65G [02:24<00:05, 9.62MB/s]
97%|###################################9 | 1.60G/1.65G [02:24<00:03, 12.7MB/s]
97%|###################################9 | 1.61G/1.65G [02:24<00:04, 11.3MB/s]
97%|###################################9 | 1.61G/1.65G [02:24<00:03, 11.6MB/s]
97%|###################################9 | 1.61G/1.65G [02:25<00:04, 10.6MB/s]
97%|#################################### | 1.61G/1.65G [02:25<00:03, 12.3MB/s]
97%|#################################### | 1.61G/1.65G [02:25<00:03, 11.0MB/s]
98%|#################################### | 1.61G/1.65G [02:25<00:03, 11.0MB/s]
98%|####################################1| 1.61G/1.65G [02:25<00:02, 13.3MB/s]
98%|####################################1| 1.62G/1.65G [02:25<00:03, 10.9MB/s]
98%|####################################2| 1.62G/1.65G [02:25<00:02, 13.4MB/s]
98%|####################################2| 1.62G/1.65G [02:25<00:02, 13.0MB/s]
98%|####################################2| 1.62G/1.65G [02:26<00:02, 13.0MB/s]
98%|####################################3| 1.62G/1.65G [02:26<00:02, 10.4MB/s]
98%|####################################3| 1.62G/1.65G [02:26<00:02, 12.2MB/s]
98%|####################################3| 1.63G/1.65G [02:26<00:01, 13.6MB/s]
98%|####################################4| 1.63G/1.65G [02:26<00:02, 11.3MB/s]
99%|####################################4| 1.63G/1.65G [02:26<00:01, 13.5MB/s]
99%|####################################5| 1.63G/1.65G [02:26<00:02, 10.7MB/s]
99%|####################################5| 1.63G/1.65G [02:27<00:01, 13.7MB/s]
99%|####################################6| 1.63G/1.65G [02:27<00:01, 12.4MB/s]
99%|####################################6| 1.64G/1.65G [02:27<00:01, 11.6MB/s]
99%|####################################6| 1.64G/1.65G [02:27<00:01, 11.1MB/s]
99%|####################################6| 1.64G/1.65G [02:27<00:01, 11.3MB/s]
99%|####################################7| 1.64G/1.65G [02:27<00:00, 14.5MB/s]
99%|####################################7| 1.64G/1.65G [02:27<00:00, 13.9MB/s]
100%|####################################8| 1.64G/1.65G [02:27<00:00, 13.4MB/s]
100%|####################################8| 1.65G/1.65G [02:28<00:00, 11.0MB/s]
100%|####################################8| 1.65G/1.65G [02:28<00:00, 13.5MB/s]
100%|####################################9| 1.65G/1.65G [02:28<00:00, 13.2MB/s]
100%|####################################9| 1.65G/1.65G [02:28<00:00, 10.8MB/s]
0%| | 0.00/1.65G [00:00<?, ?B/s]
100%|#############################################| 1.65G/1.65G [00:00<?, ?B/s]
Untarring contents of 'C:\Users\stefan\mne_data\MNE-sample-data-processed.tar.gz' to 'C:\Users\stefan\mne_data'
Attempting to create new mne-python configuration file:
C:\Users\stefan\.mne\mne-python.json
Hello! This is openneuro-py 2022.4.0. Great to see you!
Please report problems and bugs at
https://github.com/hoechenberger/openneuro-py/issues
Preparing to download ds002778 ...
Traversing directories for ds002778: 0 entities [00:00, ? entities/s]
Traversing directories for ds002778: 7 entities [00:09, 1.39s/ entities]
Traversing directories for ds002778: 8 entities [00:10, 1.22s/ entities]
Traversing directories for ds002778: 10 entities [00:10, 1.15 entities/s]
Traversing directories for ds002778: 14 entities [00:10, 2.04 entities/s]
Traversing directories for ds002778: 15 entities [00:11, 2.17 entities/s]
Traversing directories for ds002778: 17 entities [00:11, 2.73 entities/s]
Traversing directories for ds002778: 20 entities [00:11, 1.72 entities/s]
Retrieving up to 19 files (5 concurrent downloads).
CHANGES: 0.00B [00:00, ?B/s]
dataset_description.json: 0.00B [00:00, ?B/s]
README: 0.00B [00:00, ?B/s]
sub-pd6_ses-off_task-rest_beh.json: 0.00B [00:00, ?B/s]
participants.json: 0%| | 0.00/1.24k [00:00<?, ?B/s]
participants.tsv: 0%| | 0.00/1.62k [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_beh.tsv: 0%| | 0.00/10.0 [00:00<?, ?B/s]
sub-pd6_ses-off_scans.tsv: 0%| | 0.00/75.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.json: 0%| | 0.00/471 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_channels.tsv: 0%| | 0.00/2.22k [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 0%| | 0.00/11.5M [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 0%|1 | 18.5k/11.5M [00:00<01:24, 143kB/s]
sub-pd6_ses-on_scans.tsv: 0%| | 0.00/74.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_events.tsv: 0%| | 0.00/66.0 [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_beh.tsv: 0%| | 0.00/10.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 1%|4 | 66.5k/11.5M [00:00<00:43, 276kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 1%|# | 151k/11.5M [00:00<00:26, 441kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 3%|## | 304k/11.5M [00:00<00:16, 726kB/s]
sub-pd6_ses-on_task-rest_beh.json: 0%| | 0.00/433 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 5%|####2 | 627k/11.5M [00:00<00:08, 1.32MB/s]
sub-pd6_ses-on_task-rest_channels.tsv: 0%| | 0.00/2.22k [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_eeg.json: 0%| | 0.00/471 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 11%|########5 | 1.24M/11.5M [00:00<00:04, 2.52MB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 21%|################7 | 2.43M/11.5M [00:00<00:01, 5.11MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 0%| | 0.00/17.4M [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 29%|######################6 | 3.29M/11.5M [00:01<00:01, 6.16MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 0%|1 | 23.5k/17.4M [00:00<02:25, 125kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 38%|############################## | 4.37M/11.5M [00:01<00:01, 5.13MB/s]
sub-pd6_ses-on_task-rest_events.tsv: 0%| | 0.00/51.0 [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 0%|1 | 40.5k/17.4M [00:00<03:55, 77.3kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 57%|############################################9 | 6.54M/11.5M [00:01<00:00, 8.15MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|4 | 91.5k/17.4M [00:00<01:44, 173kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 72%|########################################################7 | 8.24M/11.5M [00:01<00:00, 9.57MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|5 | 125k/17.4M [00:00<01:30, 200kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 81%|###############################################################9 | 9.29M/11.5M [00:01<00:00, 9.25MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|7 | 159k/17.4M [00:00<01:22, 220kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 90%|#######################################################################3 | 10.4M/11.5M [00:01<00:00, 8.91MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|8 | 193k/17.4M [00:01<01:16, 235kB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 100%|##############################################################################7| 11.4M/11.5M [00:01<00:00, 8.80MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|# | 227k/17.4M [00:01<01:12, 247kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 1%|#2 | 261k/17.4M [00:01<01:10, 256kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 2%|#3 | 295k/17.4M [00:01<01:08, 262kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 2%|#5 | 329k/17.4M [00:01<01:07, 265kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 2%|#6 | 363k/17.4M [00:01<01:06, 267kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 2%|#8 | 397k/17.4M [00:01<01:05, 272kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 2%|#9 | 431k/17.4M [00:01<01:04, 276kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|##1 | 465k/17.4M [00:02<01:01, 288kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|##3 | 499k/17.4M [00:02<01:01, 289kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|##5 | 550k/17.4M [00:02<00:57, 305kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|##6 | 584k/17.4M [00:02<00:55, 315kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|##8 | 618k/17.4M [00:02<00:58, 301kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 4%|### | 669k/17.4M [00:02<00:55, 313kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 4%|###2 | 703k/17.4M [00:02<00:55, 317kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 4%|###4 | 737k/17.4M [00:02<00:57, 301kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 4%|###6 | 788k/17.4M [00:03<00:55, 313kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 5%|###7 | 822k/17.4M [00:03<00:55, 315kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 5%|###9 | 857k/17.4M [00:03<00:57, 303kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 5%|####1 | 907k/17.4M [00:03<00:54, 316kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 5%|####3 | 941k/17.4M [00:03<00:54, 317kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 6%|####5 | 992k/17.4M [00:03<00:53, 323kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 6%|####6 | 1.00M/17.4M [00:03<00:53, 319kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 6%|####9 | 1.05M/17.4M [00:03<00:52, 325kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 6%|##### | 1.09M/17.4M [00:04<00:52, 323kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 7%|#####2 | 1.14M/17.4M [00:04<00:52, 327kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 7%|#####4 | 1.17M/17.4M [00:04<00:52, 326kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 7%|#####6 | 1.22M/17.4M [00:04<00:49, 341kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 7%|#####9 | 1.27M/17.4M [00:04<00:50, 337kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 8%|######1 | 1.32M/17.4M [00:04<00:48, 349kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 8%|######3 | 1.35M/17.4M [00:04<00:49, 342kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 8%|######5 | 1.40M/17.4M [00:05<00:47, 354kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 8%|######7 | 1.45M/17.4M [00:05<00:45, 366kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 9%|######9 | 1.50M/17.4M [00:05<00:44, 372kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 9%|#######2 | 1.55M/17.4M [00:05<00:44, 369kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 9%|#######4 | 1.60M/17.4M [00:05<00:43, 376kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 10%|#######6 | 1.65M/17.4M [00:05<00:43, 379kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 10%|#######9 | 1.70M/17.4M [00:05<00:40, 402kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 10%|########1 | 1.75M/17.4M [00:05<00:41, 391kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 10%|########3 | 1.80M/17.4M [00:06<00:40, 401kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 11%|########6 | 1.85M/17.4M [00:06<00:41, 396kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 11%|########8 | 1.90M/17.4M [00:06<00:40, 402kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 11%|#########1 | 1.97M/17.4M [00:06<00:36, 440kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 12%|#########3 | 2.02M/17.4M [00:06<00:37, 432kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 12%|#########6 | 2.06M/17.4M [00:06<00:38, 422kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 12%|#########8 | 2.11M/17.4M [00:06<00:35, 447kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 12%|########## | 2.16M/17.4M [00:06<00:35, 447kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 13%|##########4 | 2.23M/17.4M [00:07<00:34, 459kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 13%|##########7 | 2.30M/17.4M [00:07<00:32, 480kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 14%|########### | 2.36M/17.4M [00:07<00:31, 497kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 14%|###########3 | 2.43M/17.4M [00:07<00:30, 509kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 14%|###########6 | 2.50M/17.4M [00:07<00:30, 519kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 15%|###########9 | 2.56M/17.4M [00:07<00:28, 539kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 15%|############3 | 2.65M/17.4M [00:07<00:26, 578kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 16%|############7 | 2.73M/17.4M [00:08<00:25, 600kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 16%|#############1 | 2.81M/17.4M [00:08<00:24, 619kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 17%|#############5 | 2.91M/17.4M [00:08<00:23, 649kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 17%|#############9 | 2.99M/17.4M [00:08<00:22, 672kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 18%|##############4 | 3.09M/17.4M [00:08<00:21, 692kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 18%|##############8 | 3.19M/17.4M [00:08<00:20, 722kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 19%|###############4 | 3.31M/17.4M [00:08<00:19, 775kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 20%|###############9 | 3.43M/17.4M [00:08<00:17, 818kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 20%|################5 | 3.54M/17.4M [00:09<00:16, 855kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 21%|################# | 3.66M/17.4M [00:09<00:16, 882kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 22%|#################6 | 3.79M/17.4M [00:09<00:15, 939kB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 23%|##################1 | 3.94M/17.4M [00:09<00:13, 1.02MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 23%|##################7 | 4.07M/17.4M [00:09<00:13, 1.04MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 24%|###################5 | 4.24M/17.4M [00:09<00:12, 1.12MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 25%|####################2 | 4.39M/17.4M [00:09<00:11, 1.14MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 26%|####################9 | 4.56M/17.4M [00:09<00:11, 1.19MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 27%|#####################8 | 4.74M/17.4M [00:10<00:10, 1.26MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 28%|######################6 | 4.92M/17.4M [00:10<00:09, 1.32MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 29%|#######################5 | 5.10M/17.4M [00:10<00:09, 1.36MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 31%|########################5 | 5.32M/17.4M [00:10<00:08, 1.45MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 32%|#########################4 | 5.52M/17.4M [00:10<00:08, 1.48MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 33%|##########################4 | 5.75M/17.4M [00:10<00:07, 1.58MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 34%|###########################5 | 5.98M/17.4M [00:10<00:07, 1.65MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 36%|############################6 | 6.22M/17.4M [00:11<00:06, 1.83MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 37%|#############################4 | 6.40M/17.4M [00:11<00:06, 1.72MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 38%|##############################4 | 6.61M/17.4M [00:11<00:06, 1.79MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 40%|###############################6 | 6.88M/17.4M [00:11<00:05, 1.90MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 41%|################################# | 7.18M/17.4M [00:11<00:05, 2.00MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 43%|##################################3 | 7.46M/17.4M [00:11<00:04, 2.12MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 45%|###################################8 | 7.78M/17.4M [00:11<00:04, 2.25MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 47%|#####################################3 | 8.11M/17.4M [00:11<00:04, 2.37MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 49%|######################################8 | 8.44M/17.4M [00:12<00:03, 2.47MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 51%|########################################4 | 8.79M/17.4M [00:12<00:03, 2.59MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 53%|##########################################1 | 9.15M/17.4M [00:12<00:03, 2.69MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 55%|###########################################9 | 9.54M/17.4M [00:12<00:02, 2.80MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 57%|#############################################7 | 9.93M/17.4M [00:12<00:02, 2.91MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 60%|###############################################6 | 10.3M/17.4M [00:12<00:02, 3.01MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 62%|#################################################5 | 10.7M/17.4M [00:12<00:02, 3.30MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 64%|###################################################1 | 11.1M/17.4M [00:12<00:01, 3.37MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 66%|####################################################6 | 11.4M/17.4M [00:13<00:01, 3.36MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 68%|######################################################1 | 11.8M/17.4M [00:13<00:01, 3.20MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 70%|######################################################## | 12.2M/17.4M [00:13<00:01, 3.38MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 73%|##########################################################3 | 12.7M/17.4M [00:13<00:01, 3.82MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 75%|############################################################ | 13.0M/17.4M [00:13<00:01, 3.06MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 78%|##############################################################2 | 13.5M/17.4M [00:13<00:01, 3.45MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 80%|################################################################ | 13.9M/17.4M [00:13<00:01, 3.58MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 82%|#################################################################7 | 14.3M/17.4M [00:13<00:00, 3.40MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 84%|###################################################################3 | 14.6M/17.4M [00:14<00:00, 3.38MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 86%|####################################################################9 | 15.0M/17.4M [00:14<00:00, 3.23MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 88%|######################################################################4 | 15.3M/17.4M [00:14<00:00, 3.09MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 90%|#######################################################################8 | 15.6M/17.4M [00:14<00:00, 2.94MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 92%|#########################################################################2 | 15.9M/17.4M [00:14<00:00, 2.62MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 94%|##########################################################################9 | 16.3M/17.4M [00:14<00:00, 2.77MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 95%|############################################################################3 | 16.6M/17.4M [00:14<00:00, 2.79MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 97%|#############################################################################6 | 16.8M/17.4M [00:14<00:00, 2.73MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 99%|##############################################################################8 | 17.1M/17.4M [00:15<00:00, 2.10MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 100%|###############################################################################9| 17.3M/17.4M [00:15<00:00, 2.02MB/s]
Finished downloading ds002778.
Please enjoy your brains.
Explore the dataset contents#
We can use MNE-BIDS to print a tree of all
included files and folders. We pass the max_depth
parameter to
mne_bids.print_dir_tree
to the output to four levels of folders, for
better readability in this example.
print_dir_tree(bids_root, max_depth=4)
|ds002778\
|--- CHANGES
|--- README
|--- dataset_description.json
|--- participants.json
|--- participants.tsv
|--- sub-pd6\
|------ ses-off\
|--------- sub-pd6_ses-off_scans.tsv
|--------- beh\
|------------ sub-pd6_ses-off_task-rest_beh.json
|------------ sub-pd6_ses-off_task-rest_beh.tsv
|--------- eeg\
|------------ sub-pd6_ses-off_task-rest_channels.tsv
|------------ sub-pd6_ses-off_task-rest_eeg.bdf
|------------ sub-pd6_ses-off_task-rest_eeg.json
|------------ sub-pd6_ses-off_task-rest_events.tsv
|------ ses-on\
|--------- sub-pd6_ses-on_scans.tsv
|--------- beh\
|------------ sub-pd6_ses-on_task-rest_beh.json
|------------ sub-pd6_ses-on_task-rest_beh.tsv
|--------- eeg\
|------------ sub-pd6_ses-on_task-rest_channels.tsv
|------------ sub-pd6_ses-on_task-rest_eeg.bdf
|------------ sub-pd6_ses-on_task-rest_eeg.json
|------------ sub-pd6_ses-on_task-rest_events.tsv
We can even ask MNE-BIDS to produce a human-readbale summary report on the dataset contents.
print(make_report(bids_root))
Summarizing participants.tsv C:\Users\stefan\mne_data\ds002778\participants.tsv...
Summarizing scans.tsv files [WindowsPath('C:/Users/stefan/mne_data/ds002778/sub-pd6/ses-off/sub-pd6_ses-off_scans.tsv'), WindowsPath('C:/Users/stefan/mne_data/ds002778/sub-pd6/ses-on/sub-pd6_ses-on_scans.tsv')]...
The participant template found: comprised of 14 male and 17 female participants;
comprised of 31 right hand, 0 left hand and 0 ambidextrous;
ages ranged from 47.0 to 82.0 (mean = 63.39, std = 8.69)
The UC San Diego Resting State EEG Data from Patients with Parkinson's Disease
dataset was created by Alexander P. Rockhill, Nicko Jackson, Jobi George, Adam
Aron, and Nicole C. Swann and conforms to BIDS version 1.2.2. This report was
generated with MNE-BIDS (https://doi.org/10.21105/joss.01896). The dataset
consists of 1 participants (comprised of 14 male and 17 female participants;
comprised of 31 right hand, 0 left hand and 0 ambidextrous; ages ranged from
47.0 to 82.0 (mean = 63.39, std = 8.69)) and 2 recording sessions: off, and on.
Data was recorded using an EEG system (Biosemi) sampled at 512.0 Hz with line
noise at 50, and 60 Hz. There were 2 scans in total. Recording durations ranged
from 191.0 to 289.0 seconds (mean = 240.0, std = 49.0), for a total of 480.0
seconds of data recorded over all scans. For each dataset, there were on average
41.0 (std = 0.0) recording channels per scan, out of which 41.0 (std = 0.0) were
used in analysis (0.0 +/- 0.0 were removed from analysis).
Now it’s time to get ready for reading some of the data! First, we need to
create an mne_bids.BIDSPath
, which is the workhorse object of
MNE-BIDS when it comes to file and folder operations.
For now, we’re interested only in the EEG data in the BIDS root directory of the Parkinson’s disease patient dataset. There were two sessions, one where the patients took their regular anti-Parkinsonian medications and one where they abstained for more than twelve hours. For now, we are not interested in the on-medication session.
sessions = get_entity_vals(bids_root, 'session', ignore_sessions='on')
datatype = 'eeg'
extensions = [".bdf", ".tsv"] # ignore .json files
bids_paths = find_matching_paths(bids_root, datatypes=datatype,
sessions=sessions, extensions=extensions)
We can now retrieve a list of all MEG-related files in the dataset:
print(bids_paths)
[BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_channels.tsv), BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg.bdf), BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_events.tsv)]
Note that this is the same as running:
[BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_channels.tsv), BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg.bdf), BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_events.tsv)]
The returned list contains BIDSpaths
of 3 files:
sub-pd6_ses-off_task-rest_channels.tsv
,
sub-pd6_ses-off_task-rest_events.tsv
, and
sub-pd6_ses-off_task-rest_eeg.bdf
.
The first two are so-called sidecar files that contain information on the
recording channels and experimental events, and the third one is the actual
data file.
Prepare reading the data#
There is only one subject and one experimental task (rest
).
Let’s use this knowledge to create a new BIDSPath
with
all the information required to actually read the EEG data. We also need to
pass a suffix
, which is the last part of the filename just before the
extension – 'channels'
and 'events'
for the two TSV files in
our example, and 'eeg'
for EEG raw data. For MEG and EEG raw data, the
suffix is identical to the datatype, so don’t let yourself be confused here!
Now let’s print the contents of bids_path
.
print(bids_path)
C:/Users/stefan/mne_data/ds002778/sub-pd6/ses-off/eeg/sub-pd6_ses-off_task-rest_eeg.bdf
You probably noticed two things: Firstly, this looks like an ordinary string now, not like the more-or-less neatly formatted output we saw before. And secondly, that there’s suddenly a filename extension which we never specified anywhere!
The reason is that when you call print(bids_path)
, BIDSPath
returns
a string representation of BIDSPath.fpath
, which looks different. If,
instead, you simply typed bids_path
(or print(repr(bids_path))
, which
is the same) into your Python console, you would get the nicely formatted
output:
BIDSPath(
root: C:/Users/stefan/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg)
The root
here is – you guessed it – the directory we passed via the
root
parameter: the “home” of our BIDS dataset. The datatype
, again,
is self-explanatory. The basename
, on the other hand, is created
automatically based on the suffix and BIDS entities we passed to
BIDSPath
: in our case, subject
, session
and task
.
Note
There are many more supported entities, the most-commonly used among them
probably being acquisition
. Please see
our introduction to BIDSPath to learn more
about entities, basename
, and BIDSPath
in general.
But what about that filename extension, now? BIDSPath.fpath
, which –
as you hopefully remember – is invoked when you run print(bids_path)
–
employs some heuristics to auto-detect some missing filename components.
Omitting the filename extension in your script can make your code
more portable. Note that, however, you can explicitly specify an
extension too, by passing e.g. extension='.bdf'
to BIDSPath
.
Read the data#
Let’s read the data! It’s just a single line of code.
raw = read_raw_bids(bids_path=bids_path, verbose=False)
c:\users\stefan\desktop\mne-bids\mne_bids\read.py:621: RuntimeWarning: The unit for channel(s) Status has changed from NA to V.
raw.set_channel_types(channel_type_bids_mne_map_available_channels)
Now we can inspect the raw
object to check that it contains to correct
metadata.
Basic subject metadata is here.
print(raw.info['subject_info'])
{'his_id': 'sub-pd6', 'age': '62', 'gender': 'f', 'hand': 1, 'MMSE': '30', 'NAART': '42', 'disease_duration': '8', 'rl_deficits': 'L OFF meds, more R ON meds', 'notes': 'Used preprocessed data from EEGLAB .mat file instead of raw data for pd on'}
Power line frequency is here.
print(raw.info['line_freq'])
60.0
Sampling frequency is here.
print(raw.info['sfreq'])
512.0
Events are now Annotations
print(raw.annotations)
<Annotations | 2 segments: 1 (1), 65536 (1)>
Plot the raw data.
raw.plot()
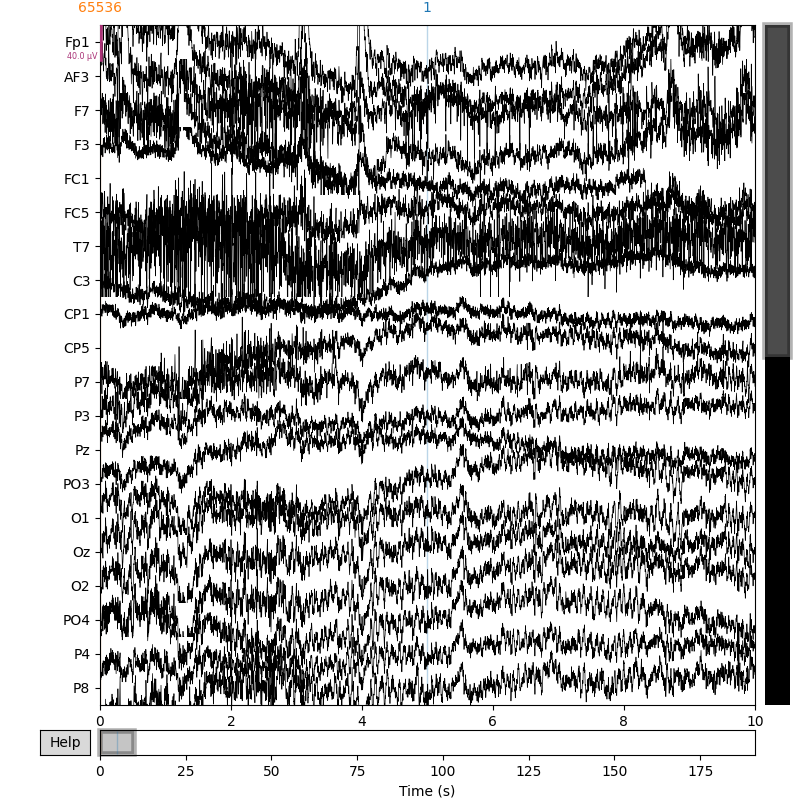
Using matplotlib as 2D backend.
<MNEBrowseFigure size 800x800 with 4 Axes>
Total running time of the script: ( 3 minutes 47.022 seconds)