mne_bids.BIDSPath#
- class mne_bids.BIDSPath(subject=None, session=None, task=None, acquisition=None, run=None, processing=None, recording=None, space=None, split=None, description=None, root=None, suffix=None, extension=None, datatype=None, check=True)[source]#
A BIDS path object.
BIDS filename prefixes have one or more pieces of metadata in them. They must follow a particular order, which is followed by this function. This will generate the prefix for a BIDS filename that can be used with many subsequent files, or you may also give a suffix that will then complete the file name.
BIDSPath allows dynamic updating of its entities in place, and operates similar to
pathlib.Path
. In addition, it can query multiple paths with matching BIDS entities via thematch
method.Note that not all parameters are applicable to each suffix of data. For example, electrode location TSV files do not need a “task” field.
- Parameters:
- subject
str
|None
The subject ID. Corresponds to “sub”.
- session
str
|None
The acquisition session. Corresponds to “ses”.
- task
str
|None
The experimental task. Corresponds to “task”.
- acquisition: str | None
The acquisition parameters. Corresponds to “acq”.
- run
int
|None
The run number. Corresponds to “run”.
- processing
str
|None
The processing label. Corresponds to “proc”.
- recording
str
|None
The recording name. Corresponds to “recording”.
- space
str
|None
The coordinate space for anatomical and sensor location files (e.g.,
*_electrodes.tsv
,*_markers.mrk
). Corresponds to “space”. Note that valid values forspace
must come from a list of BIDS keywords as described in the BIDS specification.- split
int
|None
The split of the continuous recording file for
.fif
data. Corresponds to “split”.- description
str
|None
This corresponds to the BIDS entity
desc
. It is used to provide additional information for derivative data, e.g., preprocessed data may be assigneddescription='cleaned'
.Added in version 0.11.
- suffix
str
|None
The filename suffix. This is the entity after the last
_
before the extension. E.g.,'channels'
. The following filename suffix’s are accepted: ‘meg’, ‘markers’, ‘eeg’, ‘ieeg’, ‘T1w’, ‘participants’, ‘scans’, ‘electrodes’, ‘coordsystem’, ‘channels’, ‘events’, ‘headshape’, ‘digitizer’, ‘beh’, ‘physio’, ‘stim’- extension
str
|None
The extension of the filename. E.g.,
'.json'
.- datatype
str
The BIDS data type, e.g.,
'anat'
,'func'
,'eeg'
,'meg'
,'ieeg'
.- rootpath-like |
None
The root directory of the BIDS dataset.
- check
bool
If
True
, enforces BIDS conformity. Defaults toTrue
.
- subject
- Attributes:
entities
dict
Return dictionary of the BIDS entities.
datatype
str
|None
The BIDS data type, e.g.
basename
str
Path basename.
root
pathlib.Path
The root directory of the BIDS dataset.
directory
pathlib.Path
Get the BIDS parent directory.
fpath
pathlib.Path
Full filepath for this BIDS file.
- check
bool
Whether to enforce BIDS conformity.
Methods
copy
()Copy the instance.
find_empty_room
([use_sidecar_only, verbose])Find the corresponding empty-room file of an MEG recording.
find_matching_sidecar
([suffix, extension, ...])Get the matching sidecar JSON path.
Get the list of empty-room candidates for the given file.
match
(*[, ignore_json, ignore_nosub, check])Get a list of all matching paths in the root directory.
mkdir
([exist_ok])Create the directory structure of the BIDS path.
rm
(*[, safe_remove, verbose])Safely delete a set of files from a BIDS dataset.
update
(*[, check])Update in-place BIDS entity key/value pairs in object.
Notes
BIDS entities are generally separated with a
"_"
character, while entity key/value pairs are separated with a"-"
character. There are checks performed to make sure that there are no'-'
,'_'
, or'/'
characters contained in any entity keys or values.To represent a filename such as
dataset_description.json
, one can setcheck=False
, and passsuffix='dataset_description'
andextension='.json'
.BIDSPath
can also be used to represent file and folder names of data types that are not yet supported through MNE-BIDS, but are recognized by BIDS. For example, one can setdatatype
todwi
orfunc
and passcheck=False
to represent diffusion-weighted imaging and functional MRI paths.Examples
Generate a BIDSPath object and inspect it
>>> bids_path = BIDSPath(subject='test', session='two', task='mytask', ... suffix='ieeg', extension='.edf', datatype='ieeg') >>> print(bids_path.basename) sub-test_ses-two_task-mytask_ieeg.edf >>> bids_path BIDSPath( root: None datatype: ieeg basename: sub-test_ses-two_task-mytask_ieeg.edf)
Copy and update multiple entities at once
>>> new_bids_path = bids_path.copy().update(subject='test2', ... session='one') >>> print(new_bids_path.basename) sub-test2_ses-one_task-mytask_ieeg.edf
Printing a BIDSPath will show a relative path when
root
is not set>>> print(new_bids_path) sub-test2/ses-one/ieeg/sub-test2_ses-one_task-mytask_ieeg.edf
Setting
suffix
without an identifiable datatype will make BIDSPath try to guess the datatype>>> new_bids_path = new_bids_path.update(suffix='channels', ... extension='.tsv') >>> print(new_bids_path) sub-test2/ses-one/ieeg/sub-test2_ses-one_task-mytask_channels.tsv
You can set a new root for the BIDS dataset. Let’s see what the different properties look like for our object:
>>> new_bids_path = new_bids_path.update(root='/bids_dataset') >>> print(new_bids_path.root.as_posix()) /bids_dataset >>> print(new_bids_path.basename) sub-test2_ses-one_task-mytask_channels.tsv >>> print(new_bids_path) /bids_dataset/sub-test2/ses-one/ieeg/sub-test2_ses-one_task-mytask_channels.tsv >>> print(new_bids_path.directory.as_posix()) /bids_dataset/sub-test2/ses-one/ieeg
- property basename#
Path basename.
- property directory#
Get the BIDS parent directory.
If
subject
,session
anddatatype
are set, then they will be used to construct the directory location. For example, ifsubject='01'
,session='02'
anddatatype='ieeg'
, then the directory would be:<root>/sub-01/ses-02/ieeg
- Returns:
- data_path
pathlib.Path
The path of the BIDS directory.
- data_path
- property entities#
Return dictionary of the BIDS entities.
- find_empty_room(use_sidecar_only=False, *, verbose=None)[source]#
Find the corresponding empty-room file of an MEG recording.
This will only work if the
.root
attribute of themne_bids.BIDSPath
instance has been set.- Parameters:
- use_sidecar_only
bool
Whether to only check the
AssociatedEmptyRoom
entry in the sidecar JSON file or not. IfFalse
, first look for the entry, and if unsuccessful, try to find the best-matching empty-room recording in the dataset based on the measurement date.- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- use_sidecar_only
- Returns:
- find_matching_sidecar(suffix=None, extension=None, *, on_error='raise')[source]#
Get the matching sidecar JSON path.
- Parameters:
- suffix
str
|None
The filename suffix. This is the entity after the last
_
before the extension. E.g.,'ieeg'
.- extension
str
|None
The extension of the filename. E.g.,
'.json'
.- on_error‘raise’ | ‘warn’ | ‘ignore’
If no matching sidecar file was found and this is set to
'raise'
, raise aRuntimeError
. If'warn'
, emit a warning, and if'ignore'
, neither raise an exception nor a warning, and returnNone
in both cases.
- suffix
- Returns:
- sidecar_path
pathlib.Path
|None
The path to the sidecar JSON file.
- sidecar_path
- property fpath#
Full filepath for this BIDS file.
Getting the file path consists of the entities passed in and will get the relative (or full if
root
is passed) path.- Returns:
- bids_fpath
pathlib.Path
Either the relative, or full path to the dataset.
- bids_fpath
- get_empty_room_candidates()[source]#
Get the list of empty-room candidates for the given file.
- Returns:
Notes
Added in version 0.12.0.
- match(*, ignore_json=True, ignore_nosub=False, check=False)[source]#
Get a list of all matching paths in the root directory.
Performs a recursive search, starting in
.root
(if set), based onBIDSPath.entities
object. Ignores.json
files.- Parameters:
- ignore_json
bool
If
True
, ignores json files. Defaults toTrue
.- ignore_nosub
bool
If
True
, ignores all files that are not of the formroot/sub-*
. Defaults toFalse
.- check
bool
If
True
, only returns paths that conform to BIDS. IfFalse
(default), the.check
attribute of the returnedmne_bids.BIDSPath
object will be set toTrue
for paths that do conform to BIDS, and toFalse
for those that don’t.
- ignore_json
- Returns:
- bids_paths
list
ofmne_bids.BIDSPath
The matching paths.
- bids_paths
- property meg_calibration_fpath#
Find the matching Elekta/Neuromag/MEGIN fine-calibration file.
This requires that at least
root
andsubject
are set, and thatdatatype
is either'meg'
orNone
.- Returns:
- path
pathlib.Path
|None
The path of the fine-calibration file, or
None
if it couldn’t be found.
- path
- property meg_crosstalk_fpath#
Find the matching Elekta/Neuromag/MEGIN crosstalk file.
This requires that at least
root
andsubject
are set, and thatdatatype
is either'meg'
orNone
.- Returns:
- path
pathlib.Path
|None
The path of the crosstalk file, or
None
if it couldn’t be found.
- path
- rm(*, safe_remove=True, verbose=None)[source]#
Safely delete a set of files from a BIDS dataset.
Deleting a scan that conforms to the bids-validator will remove the respective row in
*_scans.tsv
, the corresponding sidecar files, and the data file itself.Deleting all files of a subject will update the
*_participants.tsv
file.- Parameters:
- safe_remove
bool
If
False
, directly delete and update the files. Otherwise, displays the list of operations planned and asks for user confirmation before executing them (default).- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- safe_remove
- Returns:
- self
BIDSPath
The BIDSPath object.
- self
Examples
Remove one specific run:
>>> bids_path = BIDSPath(subject='01', session='01', run="01", ... root='/bids_dataset').rm() Please, confirm you want to execute the following operations: Delete: /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-01_channels.tsv /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-01_events.json /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-01_events.tsv /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-01_meg.fif /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-01_meg.json Update: /bids_dataset/sub-01/ses-01/sub-01_ses-01_scans.tsv I confirm [y/N]>? y
Remove all the files of a specific subject:
>>> bids_path = BIDSPath(subject='01', root='/bids_dataset', ... check=False).rm() Please, confirm you want to execute the following operations: Delete: /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_acq-calibration_meg.dat /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_acq-crosstalk_meg.fif /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_coordsystem.json /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-02_channels.tsv /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-02_events.json /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-02_events.tsv /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-02_meg.fif /bids_dataset/sub-01/ses-01/meg/sub-01_ses-01_run-02_meg.json /bids_dataset/sub-01/ses-01/sub-01_ses-01_scans.tsv /bids_dataset/sub-01 Update: /bids_dataset/participants.tsv I confirm [y/N]>? y
- update(*, check=None, **kwargs)[source]#
Update in-place BIDS entity key/value pairs in object.
run
andsplit
are auto-converted to have two digits. For example, ifrun=1
, then it will becomerun='01'
.Also performs error checks on various entities to adhere to the BIDS specification. Specifically: -
datatype
should be one of:anat
,eeg
,ieeg
,meg
-extension
should be one of the accepted file extensions in the file path:.con
,.sqd
,.fif
,.pdf
,.ds
,.vhdr
,.edf
,.bdf
,.set
,.edf
,.set
,.mef
,.nwb
-suffix
should be one of the acceptable file suffixes in:meg
,markers
,eeg
,ieeg
,T1w
,participants
,scans
,electrodes
,channels
,coordsystem
,events
,headshape
,digitizer
,beh
,physio
,stim
- Depending on the modality of the data (EEG, MEG, iEEG),space
should be a valid string according to Appendix VIII in the BIDS specification.- Parameters:
- check
None
|bool
If a boolean, controls whether to enforce BIDS conformity. This will set the
.check
attribute accordingly. IfNone
, rely on the existing.check
attribute instead, which is set uponmne_bids.BIDSPath
instantiation. Defaults toNone
.- **kwargs
dict
It can contain updates for valid BIDSPath entities: ‘subject’, ‘session’, ‘task’, ‘acquisition’, ‘processing’, ‘run’, ‘recording’, ‘space’, ‘suffix’, ‘split’, ‘extension’, or updates for ‘root’ or ‘datatype’.
- check
- Returns:
- bidspath
BIDSPath
The updated instance of BIDSPath.
- bidspath
Examples
If one creates a bids basename using
mne_bids.BIDSPath()
:>>> bids_path = BIDSPath(subject='test', session='two', ... task='mytask', suffix='channels', ... extension='.tsv') >>> print(bids_path.basename) sub-test_ses-two_task-mytask_channels.tsv >>> # Then, one can update this `BIDSPath` object in place >>> bids_path.update(acquisition='test', suffix='ieeg', ... datatype='ieeg', ... extension='.vhdr', task=None) BIDSPath( root: None datatype: ieeg basename: sub-test_ses-two_acq-test_ieeg.vhdr) >>> print(bids_path.basename) sub-test_ses-two_acq-test_ieeg.vhdr
Examples using mne_bids.BIDSPath
#
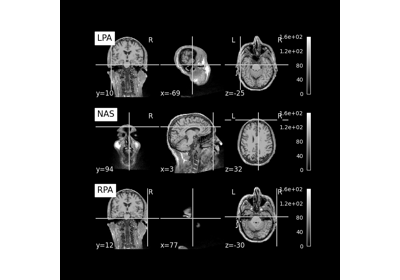
Save and load T1-weighted MRI scan along with anatomical landmarks in BIDS
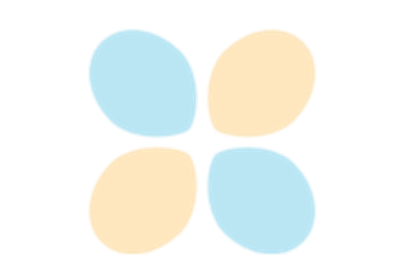
Creating BIDS-compatible folder names and filenames
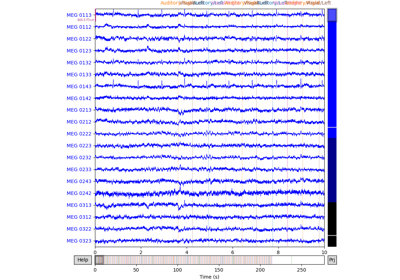
Interactive data inspection and bad channel selection