Note
Go to the end to download the full example code.
Repairing artifacts with ICA automatically using ICLabel Model#
This tutorial covers automatically repairing signals using ICA with the ICLabel model[1], which originates in EEGLab. For conceptual background on ICA, see this scikit-learn tutorial. For a basic understanding of how to use ICA to remove artifacts, see the tutorial in MNE-Python.
ICLabel is designed to classify ICs fitted with an extended infomax ICA decomposition algorithm on EEG datasets referenced to a common average and filtered between [1., 100.] Hz. It is possible to run ICLabel on datasets that do not meet those specification, but the classification performance might be negatively impacted. Moreover, the ICLabel paper did not study the effects of these preprocessing steps.
Note
This example involves running the ICA Infomax algorithm, which requires scikit-learn to be installed. Please install this optional dependency before running the example.
We begin as always by importing the necessary Python modules and loading some
example data. Because ICA can be computationally
intense, we’ll also crop the data to 60 seconds; and to save ourselves from
repeatedly typing mne.preprocessing
we’ll directly import a few functions
and classes from that submodule.
import mne
from mne.preprocessing import ICA
from mne_icalabel import label_components
sample_data_folder = mne.datasets.sample.data_path() / "MEG" / "sample"
sample_data_raw_file = sample_data_folder / "sample_audvis_raw.fif"
raw = mne.io.read_raw_fif(sample_data_raw_file)
# we'll crop to 60 seconds and drop MEG channels
raw.crop(tmax=60.0).pick_types(eeg=True, stim=True, eog=True)
raw.load_data()
Using default location ~/mne_data for sample...
Creating /home/runner/mne_data
0%| | 0.00/1.65G [00:00<?, ?B/s]
0%| | 1.58M/1.65G [00:00<01:52, 14.6MB/s]
0%| | 3.14M/1.65G [00:00<01:48, 15.2MB/s]
0%| | 4.66M/1.65G [00:00<02:10, 12.6MB/s]
0%|▏ | 5.96M/1.65G [00:00<02:41, 10.2MB/s]
0%|▏ | 7.05M/1.65G [00:00<02:41, 10.2MB/s]
0%|▏ | 8.12M/1.65G [00:00<02:43, 10.0MB/s]
1%|▎ | 11.4M/1.65G [00:00<01:39, 16.5MB/s]
1%|▎ | 14.2M/1.65G [00:00<01:23, 19.7MB/s]
1%|▎ | 16.2M/1.65G [00:01<01:49, 14.9MB/s]
1%|▍ | 18.0M/1.65G [00:01<01:59, 13.7MB/s]
1%|▍ | 19.6M/1.65G [00:01<01:54, 14.3MB/s]
1%|▍ | 21.2M/1.65G [00:01<01:52, 14.5MB/s]
1%|▌ | 22.8M/1.65G [00:01<01:51, 14.6MB/s]
1%|▌ | 24.6M/1.65G [00:01<01:43, 15.7MB/s]
2%|▌ | 26.4M/1.65G [00:01<01:40, 16.2MB/s]
2%|▋ | 28.1M/1.65G [00:01<01:40, 16.1MB/s]
2%|▋ | 29.8M/1.65G [00:02<01:43, 15.7MB/s]
2%|▋ | 31.4M/1.65G [00:02<01:45, 15.3MB/s]
2%|▋ | 33.3M/1.65G [00:02<01:40, 16.2MB/s]
2%|▊ | 36.1M/1.65G [00:02<01:21, 19.8MB/s]
3%|▉ | 42.1M/1.65G [00:02<00:51, 31.2MB/s]
3%|█ | 48.0M/1.65G [00:02<00:40, 39.6MB/s]
3%|█▏ | 54.5M/1.65G [00:02<00:34, 47.0MB/s]
4%|█▎ | 61.2M/1.65G [00:02<00:30, 52.8MB/s]
4%|█▌ | 67.6M/1.65G [00:02<00:28, 56.3MB/s]
4%|█▋ | 73.3M/1.65G [00:03<00:47, 33.3MB/s]
5%|█▋ | 77.8M/1.65G [00:03<01:16, 20.7MB/s]
5%|█▊ | 81.2M/1.65G [00:03<01:31, 17.2MB/s]
5%|█▉ | 83.9M/1.65G [00:04<01:49, 14.4MB/s]
5%|█▉ | 86.1M/1.65G [00:04<01:55, 13.5MB/s]
5%|█▉ | 87.9M/1.65G [00:04<01:53, 13.8MB/s]
6%|██ | 93.1M/1.65G [00:04<01:17, 20.1MB/s]
6%|██▏ | 99.2M/1.65G [00:04<00:55, 27.7MB/s]
6%|██▍ | 106M/1.65G [00:04<00:43, 35.4MB/s]
7%|██▌ | 112M/1.65G [00:05<00:36, 42.1MB/s]
7%|██▋ | 118M/1.65G [00:05<00:32, 47.5MB/s]
7%|██▊ | 124M/1.65G [00:05<00:48, 31.3MB/s]
8%|██▉ | 128M/1.65G [00:05<01:06, 23.0MB/s]
8%|███ | 132M/1.65G [00:06<01:18, 19.3MB/s]
8%|███ | 134M/1.65G [00:06<01:20, 18.9MB/s]
8%|███▏ | 137M/1.65G [00:06<01:25, 17.8MB/s]
8%|███▏ | 139M/1.65G [00:06<01:30, 16.8MB/s]
9%|███▏ | 141M/1.65G [00:06<01:28, 17.1MB/s]
9%|███▎ | 143M/1.65G [00:06<01:30, 16.7MB/s]
9%|███▎ | 145M/1.65G [00:06<01:27, 17.2MB/s]
9%|███▎ | 147M/1.65G [00:06<01:27, 17.2MB/s]
9%|███▍ | 149M/1.65G [00:07<01:24, 17.8MB/s]
9%|███▍ | 151M/1.65G [00:07<01:31, 16.3MB/s]
9%|███▌ | 152M/1.65G [00:07<01:33, 16.1MB/s]
9%|███▌ | 154M/1.65G [00:07<01:31, 16.4MB/s]
9%|███▌ | 156M/1.65G [00:07<01:31, 16.4MB/s]
10%|███▌ | 157M/1.65G [00:07<01:30, 16.6MB/s]
10%|███▋ | 159M/1.65G [00:07<01:29, 16.7MB/s]
10%|███▋ | 161M/1.65G [00:07<01:28, 16.9MB/s]
10%|███▋ | 163M/1.65G [00:07<01:28, 16.9MB/s]
10%|███▊ | 165M/1.65G [00:08<01:28, 16.9MB/s]
10%|███▊ | 167M/1.65G [00:08<01:25, 17.3MB/s]
10%|███▉ | 169M/1.65G [00:08<01:21, 18.3MB/s]
10%|███▉ | 171M/1.65G [00:08<01:21, 18.2MB/s]
10%|███▉ | 172M/1.65G [00:08<01:26, 17.1MB/s]
11%|████ | 174M/1.65G [00:08<01:37, 15.2MB/s]
11%|████ | 176M/1.65G [00:08<01:38, 14.9MB/s]
11%|████ | 177M/1.65G [00:08<01:39, 14.8MB/s]
11%|████▏ | 182M/1.65G [00:08<00:59, 24.6MB/s]
11%|████▎ | 188M/1.65G [00:09<00:43, 33.4MB/s]
12%|████▍ | 194M/1.65G [00:09<00:35, 41.5MB/s]
12%|████▌ | 200M/1.65G [00:09<00:30, 47.2MB/s]
12%|████▋ | 206M/1.65G [00:09<00:28, 51.5MB/s]
13%|████▊ | 212M/1.65G [00:09<00:27, 53.3MB/s]
13%|█████ | 218M/1.65G [00:09<00:26, 54.8MB/s]
14%|█████▏ | 223M/1.65G [00:09<00:25, 55.1MB/s]
14%|█████▎ | 229M/1.65G [00:09<00:25, 55.4MB/s]
14%|█████▍ | 235M/1.65G [00:09<00:25, 55.4MB/s]
15%|█████▌ | 240M/1.65G [00:09<00:25, 55.4MB/s]
15%|█████▋ | 246M/1.65G [00:10<01:02, 22.5MB/s]
15%|█████▋ | 250M/1.65G [00:10<01:14, 18.8MB/s]
15%|█████▊ | 253M/1.65G [00:11<01:30, 15.4MB/s]
15%|█████▉ | 256M/1.65G [00:11<01:39, 14.1MB/s]
16%|█████▉ | 258M/1.65G [00:11<01:47, 13.0MB/s]
16%|█████▉ | 260M/1.65G [00:11<01:48, 12.9MB/s]
16%|██████ | 261M/1.65G [00:11<01:46, 13.0MB/s]
16%|██████ | 263M/1.65G [00:12<01:42, 13.5MB/s]
16%|██████ | 265M/1.65G [00:12<01:39, 13.9MB/s]
16%|██████▏ | 266M/1.65G [00:12<01:32, 14.9MB/s]
16%|██████▏ | 268M/1.65G [00:12<01:26, 16.1MB/s]
16%|██████▏ | 270M/1.65G [00:12<01:24, 16.3MB/s]
16%|██████▏ | 272M/1.65G [00:12<01:24, 16.3MB/s]
17%|██████▎ | 274M/1.65G [00:12<01:30, 15.2MB/s]
17%|██████▎ | 275M/1.65G [00:12<01:36, 14.3MB/s]
17%|██████▎ | 277M/1.65G [00:12<01:47, 12.7MB/s]
17%|██████▍ | 278M/1.65G [00:13<01:53, 12.2MB/s]
17%|██████▍ | 279M/1.65G [00:13<01:52, 12.2MB/s]
17%|██████▍ | 280M/1.65G [00:13<02:02, 11.2MB/s]
17%|██████▍ | 282M/1.65G [00:13<02:02, 11.2MB/s]
17%|██████▌ | 283M/1.65G [00:13<01:53, 12.1MB/s]
17%|██████▌ | 284M/1.65G [00:13<01:50, 12.4MB/s]
17%|██████▌ | 286M/1.65G [00:13<01:45, 12.9MB/s]
17%|██████▌ | 287M/1.65G [00:13<01:41, 13.4MB/s]
17%|██████▋ | 289M/1.65G [00:13<01:42, 13.3MB/s]
18%|██████▋ | 290M/1.65G [00:14<01:43, 13.1MB/s]
18%|██████▋ | 291M/1.65G [00:14<01:52, 12.1MB/s]
18%|██████▊ | 295M/1.65G [00:14<01:12, 18.8MB/s]
18%|██████▉ | 300M/1.65G [00:14<00:54, 24.8MB/s]
18%|██████▉ | 302M/1.65G [00:14<00:58, 23.2MB/s]
18%|███████ | 304M/1.65G [00:14<01:00, 22.4MB/s]
19%|███████ | 307M/1.65G [00:14<01:22, 16.3MB/s]
19%|███████ | 309M/1.65G [00:15<01:32, 14.5MB/s]
19%|███████▏ | 310M/1.65G [00:15<01:43, 13.0MB/s]
19%|███████▏ | 312M/1.65G [00:15<01:48, 12.4MB/s]
19%|███████▏ | 313M/1.65G [00:15<01:52, 11.9MB/s]
19%|███████▏ | 314M/1.65G [00:15<01:56, 11.5MB/s]
19%|███████▎ | 315M/1.65G [00:15<01:57, 11.3MB/s]
19%|███████▎ | 317M/1.65G [00:15<01:57, 11.4MB/s]
19%|███████▎ | 318M/1.65G [00:15<01:56, 11.5MB/s]
19%|███████▎ | 319M/1.65G [00:16<01:59, 11.2MB/s]
19%|███████▎ | 320M/1.65G [00:16<01:59, 11.2MB/s]
19%|███████▍ | 322M/1.65G [00:16<02:06, 10.5MB/s]
20%|███████▍ | 324M/1.65G [00:16<01:31, 14.5MB/s]
20%|███████▌ | 330M/1.65G [00:16<00:50, 26.2MB/s]
20%|███████▋ | 336M/1.65G [00:16<00:36, 36.0MB/s]
21%|███████▊ | 340M/1.65G [00:16<00:52, 25.0MB/s]
21%|███████▉ | 343M/1.65G [00:17<01:04, 20.3MB/s]
21%|███████▉ | 345M/1.65G [00:17<01:15, 17.3MB/s]
21%|███████▉ | 347M/1.65G [00:17<01:19, 16.4MB/s]
21%|████████ | 349M/1.65G [00:17<01:27, 14.9MB/s]
21%|████████ | 351M/1.65G [00:17<01:31, 14.2MB/s]
21%|████████ | 353M/1.65G [00:17<01:33, 13.8MB/s]
21%|████████▏ | 354M/1.65G [00:18<01:41, 12.8MB/s]
22%|████████▏ | 355M/1.65G [00:18<01:44, 12.4MB/s]
22%|████████▏ | 358M/1.65G [00:18<01:27, 14.7MB/s]
22%|████████▎ | 364M/1.65G [00:18<00:48, 26.4MB/s]
22%|████████▌ | 370M/1.65G [00:18<00:36, 35.5MB/s]
23%|████████▌ | 374M/1.65G [00:18<00:47, 27.1MB/s]
23%|████████▋ | 377M/1.65G [00:18<00:57, 22.4MB/s]
23%|████████▋ | 380M/1.65G [00:19<01:08, 18.7MB/s]
23%|████████▊ | 382M/1.65G [00:19<01:17, 16.4MB/s]
23%|████████▊ | 384M/1.65G [00:19<01:23, 15.3MB/s]
23%|████████▊ | 386M/1.65G [00:19<01:19, 16.0MB/s]
23%|████████▉ | 387M/1.65G [00:19<01:20, 15.8MB/s]
24%|████████▉ | 389M/1.65G [00:19<01:19, 15.9MB/s]
24%|████████▉ | 391M/1.65G [00:19<01:16, 16.5MB/s]
24%|█████████ | 393M/1.65G [00:20<01:14, 17.0MB/s]
24%|█████████ | 395M/1.65G [00:20<01:12, 17.3MB/s]
24%|█████████ | 396M/1.65G [00:20<01:11, 17.5MB/s]
24%|█████████▏ | 398M/1.65G [00:20<01:23, 15.0MB/s]
24%|█████████▏ | 400M/1.65G [00:20<01:28, 14.2MB/s]
24%|█████████▏ | 401M/1.65G [00:20<01:25, 14.7MB/s]
24%|█████████▎ | 403M/1.65G [00:20<01:23, 14.9MB/s]
24%|█████████▎ | 405M/1.65G [00:20<01:18, 15.8MB/s]
25%|█████████▎ | 406M/1.65G [00:20<01:19, 15.7MB/s]
25%|█████████▍ | 408M/1.65G [00:21<01:16, 16.2MB/s]
25%|█████████▍ | 410M/1.65G [00:21<01:18, 15.9MB/s]
25%|█████████▍ | 411M/1.65G [00:21<01:22, 15.0MB/s]
25%|█████████▍ | 413M/1.65G [00:21<01:27, 14.2MB/s]
25%|█████████▌ | 414M/1.65G [00:21<01:24, 14.7MB/s]
25%|█████████▌ | 416M/1.65G [00:21<01:26, 14.3MB/s]
25%|█████████▌ | 418M/1.65G [00:21<01:16, 16.1MB/s]
25%|█████████▋ | 420M/1.65G [00:21<01:14, 16.5MB/s]
26%|█████████▋ | 421M/1.65G [00:21<01:16, 16.1MB/s]
26%|█████████▋ | 423M/1.65G [00:22<01:24, 14.5MB/s]
26%|█████████▊ | 429M/1.65G [00:22<00:46, 26.3MB/s]
26%|██████████ | 435M/1.65G [00:22<00:33, 35.8MB/s]
27%|██████████▏ | 441M/1.65G [00:22<00:27, 43.9MB/s]
27%|██████████▎ | 448M/1.65G [00:22<00:24, 48.8MB/s]
27%|██████████▍ | 453M/1.65G [00:22<00:23, 51.6MB/s]
28%|██████████▌ | 460M/1.65G [00:22<00:21, 54.5MB/s]
28%|██████████▋ | 466M/1.65G [00:22<00:20, 56.6MB/s]
29%|██████████▊ | 472M/1.65G [00:22<00:19, 59.1MB/s]
29%|███████████ | 479M/1.65G [00:22<00:19, 60.9MB/s]
29%|███████████▏ | 485M/1.65G [00:23<00:18, 62.5MB/s]
30%|███████████▎ | 492M/1.65G [00:23<00:27, 42.2MB/s]
30%|███████████▍ | 497M/1.65G [00:23<00:41, 27.6MB/s]
30%|███████████▌ | 501M/1.65G [00:23<00:49, 23.1MB/s]
30%|███████████▌ | 504M/1.65G [00:24<00:54, 21.2MB/s]
31%|███████████▋ | 507M/1.65G [00:24<00:56, 20.2MB/s]
31%|███████████▋ | 509M/1.65G [00:24<01:02, 18.3MB/s]
31%|███████████▊ | 511M/1.65G [00:24<01:03, 18.0MB/s]
31%|███████████▊ | 513M/1.65G [00:24<01:07, 16.9MB/s]
31%|███████████▊ | 515M/1.65G [00:24<01:08, 16.7MB/s]
31%|███████████▉ | 517M/1.65G [00:24<01:09, 16.4MB/s]
31%|███████████▉ | 518M/1.65G [00:25<01:08, 16.6MB/s]
31%|███████████▉ | 520M/1.65G [00:25<01:07, 16.8MB/s]
32%|████████████ | 522M/1.65G [00:25<01:05, 17.2MB/s]
32%|████████████ | 524M/1.65G [00:25<01:05, 17.3MB/s]
32%|████████████ | 526M/1.65G [00:25<01:06, 16.9MB/s]
32%|████████████▏ | 528M/1.65G [00:25<01:05, 17.2MB/s]
32%|████████████▏ | 529M/1.65G [00:25<01:09, 16.1MB/s]
32%|████████████▏ | 531M/1.65G [00:25<01:07, 16.7MB/s]
32%|████████████▏ | 533M/1.65G [00:25<01:07, 16.5MB/s]
32%|████████████▎ | 534M/1.65G [00:26<01:13, 15.2MB/s]
32%|████████████▎ | 536M/1.65G [00:26<01:18, 14.3MB/s]
33%|████████████▎ | 537M/1.65G [00:26<01:19, 14.1MB/s]
33%|████████████▍ | 539M/1.65G [00:26<01:16, 14.6MB/s]
33%|████████████▍ | 541M/1.65G [00:26<01:15, 14.8MB/s]
33%|████████████▌ | 546M/1.65G [00:26<00:41, 26.6MB/s]
33%|████████████▋ | 552M/1.65G [00:26<00:30, 36.2MB/s]
34%|████████████▊ | 558M/1.65G [00:26<00:25, 42.5MB/s]
34%|████████████▉ | 563M/1.65G [00:27<00:51, 21.0MB/s]
34%|█████████████ | 566M/1.65G [00:27<01:03, 17.1MB/s]
34%|█████████████ | 569M/1.65G [00:27<01:10, 15.4MB/s]
35%|█████████████ | 571M/1.65G [00:27<01:13, 14.6MB/s]
35%|█████████████▏ | 573M/1.65G [00:28<01:16, 14.0MB/s]
35%|█████████████▏ | 574M/1.65G [00:28<01:19, 13.6MB/s]
35%|█████████████▏ | 576M/1.65G [00:28<01:21, 13.2MB/s]
35%|█████████████▎ | 577M/1.65G [00:28<01:25, 12.5MB/s]
35%|█████████████▍ | 583M/1.65G [00:28<00:49, 21.5MB/s]
36%|█████████████▌ | 589M/1.65G [00:28<00:34, 30.6MB/s]
36%|█████████████▋ | 595M/1.65G [00:28<00:27, 38.3MB/s]
36%|█████████████▊ | 602M/1.65G [00:28<00:23, 45.6MB/s]
37%|█████████████▉ | 608M/1.65G [00:29<00:20, 50.8MB/s]
37%|██████████████▏ | 614M/1.65G [00:29<00:19, 54.5MB/s]
38%|██████████████▎ | 621M/1.65G [00:29<00:17, 57.8MB/s]
38%|██████████████▍ | 628M/1.65G [00:29<00:16, 60.4MB/s]
38%|██████████████▌ | 634M/1.65G [00:29<00:16, 61.9MB/s]
39%|██████████████▋ | 641M/1.65G [00:29<00:15, 63.3MB/s]
39%|██████████████▉ | 647M/1.65G [00:29<00:15, 63.8MB/s]
40%|███████████████ | 654M/1.65G [00:29<00:15, 64.2MB/s]
40%|███████████████▏ | 660M/1.65G [00:29<00:15, 64.1MB/s]
40%|███████████████▎ | 667M/1.65G [00:29<00:15, 62.5MB/s]
41%|███████████████▍ | 673M/1.65G [00:30<00:16, 60.9MB/s]
41%|███████████████▌ | 679M/1.65G [00:30<00:16, 60.3MB/s]
41%|███████████████▊ | 685M/1.65G [00:30<00:16, 59.9MB/s]
42%|███████████████▉ | 691M/1.65G [00:30<00:16, 60.1MB/s]
42%|████████████████ | 697M/1.65G [00:30<00:15, 60.2MB/s]
43%|████████████████▏ | 703M/1.65G [00:30<00:16, 58.9MB/s]
43%|████████████████▎ | 709M/1.65G [00:30<00:17, 54.8MB/s]
43%|████████████████▍ | 715M/1.65G [00:30<00:17, 54.0MB/s]
44%|████████████████▌ | 721M/1.65G [00:30<00:16, 55.0MB/s]
44%|████████████████▋ | 726M/1.65G [00:30<00:16, 55.2MB/s]
44%|████████████████▊ | 732M/1.65G [00:31<00:17, 52.9MB/s]
45%|████████████████▉ | 737M/1.65G [00:31<00:16, 54.0MB/s]
45%|█████████████████ | 743M/1.65G [00:31<00:16, 55.1MB/s]
45%|█████████████████▏ | 749M/1.65G [00:31<00:16, 56.0MB/s]
46%|█████████████████▎ | 755M/1.65G [00:31<00:15, 57.1MB/s]
46%|█████████████████▍ | 761M/1.65G [00:31<00:15, 57.3MB/s]
46%|█████████████████▋ | 767M/1.65G [00:31<00:15, 57.7MB/s]
47%|█████████████████▊ | 772M/1.65G [00:31<00:15, 56.3MB/s]
47%|█████████████████▉ | 778M/1.65G [00:31<00:15, 56.9MB/s]
47%|██████████████████ | 784M/1.65G [00:32<00:15, 56.7MB/s]
48%|██████████████████▏ | 790M/1.65G [00:32<00:15, 56.4MB/s]
48%|██████████████████▎ | 795M/1.65G [00:32<00:15, 54.7MB/s]
48%|██████████████████▍ | 801M/1.65G [00:32<00:15, 55.8MB/s]
49%|██████████████████▌ | 807M/1.65G [00:32<00:14, 57.1MB/s]
49%|██████████████████▋ | 813M/1.65G [00:32<00:14, 58.3MB/s]
50%|██████████████████▊ | 820M/1.65G [00:32<00:13, 60.6MB/s]
50%|███████████████████ | 826M/1.65G [00:32<00:13, 62.1MB/s]
50%|███████████████████▏ | 833M/1.65G [00:32<00:12, 63.3MB/s]
51%|███████████████████▎ | 840M/1.65G [00:32<00:12, 63.8MB/s]
51%|███████████████████▍ | 846M/1.65G [00:33<00:12, 63.8MB/s]
52%|███████████████████▌ | 852M/1.65G [00:33<00:12, 63.9MB/s]
52%|███████████████████▋ | 859M/1.65G [00:33<00:12, 64.2MB/s]
52%|███████████████████▉ | 865M/1.65G [00:33<00:12, 65.0MB/s]
53%|████████████████████ | 872M/1.65G [00:33<00:12, 64.8MB/s]
53%|████████████████████▏ | 879M/1.65G [00:33<00:11, 65.0MB/s]
54%|████████████████████▎ | 885M/1.65G [00:33<00:11, 64.7MB/s]
54%|████████████████████▍ | 891M/1.65G [00:33<00:11, 64.4MB/s]
54%|████████████████████▋ | 898M/1.65G [00:33<00:13, 57.2MB/s]
55%|████████████████████▊ | 904M/1.65G [00:34<00:36, 20.8MB/s]
55%|████████████████████▉ | 908M/1.65G [00:35<00:47, 15.6MB/s]
55%|████████████████████▉ | 911M/1.65G [00:35<00:54, 13.6MB/s]
55%|█████████████████████ | 914M/1.65G [00:35<00:57, 12.9MB/s]
56%|█████████████████████▏ | 919M/1.65G [00:35<00:41, 17.6MB/s]
56%|█████████████████████▏ | 923M/1.65G [00:35<00:35, 20.8MB/s]
56%|█████████████████████▎ | 927M/1.65G [00:36<00:31, 23.3MB/s]
56%|█████████████████████▍ | 933M/1.65G [00:36<00:24, 29.5MB/s]
57%|█████████████████████▌ | 939M/1.65G [00:36<00:19, 36.4MB/s]
57%|█████████████████████▋ | 945M/1.65G [00:36<00:16, 42.8MB/s]
58%|█████████████████████▉ | 952M/1.65G [00:36<00:14, 48.7MB/s]
58%|██████████████████████ | 958M/1.65G [00:36<00:13, 53.1MB/s]
58%|██████████████████████▏ | 965M/1.65G [00:36<00:12, 56.9MB/s]
59%|██████████████████████▎ | 972M/1.65G [00:36<00:11, 59.1MB/s]
59%|██████████████████████▍ | 978M/1.65G [00:36<00:11, 61.2MB/s]
60%|██████████████████████▋ | 985M/1.65G [00:36<00:10, 62.8MB/s]
60%|██████████████████████▊ | 992M/1.65G [00:37<00:10, 64.0MB/s]
60%|██████████████████████▉ | 998M/1.65G [00:37<00:10, 65.2MB/s]
61%|██████████████████████▍ | 1.01G/1.65G [00:37<00:09, 65.8MB/s]
61%|██████████████████████▋ | 1.01G/1.65G [00:37<00:09, 66.5MB/s]
62%|██████████████████████▊ | 1.02G/1.65G [00:37<00:09, 66.5MB/s]
62%|██████████████████████▉ | 1.03G/1.65G [00:37<00:09, 66.3MB/s]
62%|███████████████████████ | 1.03G/1.65G [00:37<00:09, 66.1MB/s]
63%|███████████████████████▏ | 1.04G/1.65G [00:37<00:09, 66.3MB/s]
63%|███████████████████████▍ | 1.05G/1.65G [00:37<00:09, 66.7MB/s]
64%|███████████████████████▌ | 1.05G/1.65G [00:37<00:09, 66.7MB/s]
64%|███████████████████████▋ | 1.06G/1.65G [00:38<00:08, 67.1MB/s]
64%|███████████████████████▊ | 1.07G/1.65G [00:38<00:08, 66.7MB/s]
65%|████████████████████████ | 1.07G/1.65G [00:38<00:08, 66.9MB/s]
65%|████████████████████████▏ | 1.08G/1.65G [00:38<00:08, 66.9MB/s]
66%|████████████████████████▎ | 1.09G/1.65G [00:38<00:08, 67.0MB/s]
66%|████████████████████████▍ | 1.09G/1.65G [00:38<00:08, 67.3MB/s]
67%|████████████████████████▌ | 1.10G/1.65G [00:38<00:08, 67.4MB/s]
67%|████████████████████████▊ | 1.11G/1.65G [00:38<00:08, 67.2MB/s]
67%|████████████████████████▉ | 1.11G/1.65G [00:38<00:08, 67.2MB/s]
68%|█████████████████████████ | 1.12G/1.65G [00:38<00:07, 67.1MB/s]
68%|█████████████████████████▏ | 1.13G/1.65G [00:39<00:07, 67.0MB/s]
69%|█████████████████████████▎ | 1.13G/1.65G [00:39<00:07, 66.9MB/s]
69%|█████████████████████████▌ | 1.14G/1.65G [00:39<00:07, 66.9MB/s]
69%|█████████████████████████▋ | 1.15G/1.65G [00:39<00:07, 67.1MB/s]
70%|█████████████████████████▊ | 1.15G/1.65G [00:39<00:07, 67.4MB/s]
70%|█████████████████████████▉ | 1.16G/1.65G [00:39<00:07, 67.3MB/s]
71%|██████████████████████████ | 1.17G/1.65G [00:39<00:07, 67.2MB/s]
71%|██████████████████████████▎ | 1.17G/1.65G [00:39<00:07, 64.2MB/s]
71%|██████████████████████████▍ | 1.18G/1.65G [00:39<00:07, 63.9MB/s]
72%|██████████████████████████▌ | 1.19G/1.65G [00:39<00:07, 63.3MB/s]
72%|██████████████████████████▋ | 1.19G/1.65G [00:40<00:07, 63.0MB/s]
73%|██████████████████████████▊ | 1.20G/1.65G [00:40<00:07, 62.8MB/s]
73%|██████████████████████████▉ | 1.21G/1.65G [00:40<00:07, 63.6MB/s]
73%|███████████████████████████▏ | 1.21G/1.65G [00:40<00:06, 63.5MB/s]
74%|███████████████████████████▎ | 1.22G/1.65G [00:40<00:06, 62.9MB/s]
74%|███████████████████████████▍ | 1.22G/1.65G [00:40<00:06, 62.6MB/s]
74%|███████████████████████████▌ | 1.23G/1.65G [00:40<00:06, 62.4MB/s]
75%|███████████████████████████▋ | 1.24G/1.65G [00:40<00:06, 61.6MB/s]
75%|███████████████████████████▊ | 1.24G/1.65G [00:41<00:09, 45.2MB/s]
76%|███████████████████████████▉ | 1.25G/1.65G [00:41<00:08, 45.6MB/s]
76%|████████████████████████████ | 1.25G/1.65G [00:41<00:08, 47.5MB/s]
76%|████████████████████████████▏ | 1.26G/1.65G [00:41<00:07, 50.5MB/s]
77%|████████████████████████████▎ | 1.27G/1.65G [00:41<00:07, 52.9MB/s]
77%|████████████████████████████▍ | 1.27G/1.65G [00:41<00:11, 32.6MB/s]
77%|████████████████████████████▌ | 1.28G/1.65G [00:41<00:09, 37.6MB/s]
78%|████████████████████████████▋ | 1.28G/1.65G [00:41<00:08, 42.7MB/s]
78%|████████████████████████████▊ | 1.29G/1.65G [00:42<00:07, 48.4MB/s]
78%|█████████████████████████████ | 1.30G/1.65G [00:42<00:06, 53.0MB/s]
79%|█████████████████████████████▏ | 1.30G/1.65G [00:42<00:06, 56.9MB/s]
79%|█████████████████████████████▎ | 1.31G/1.65G [00:42<00:05, 58.4MB/s]
80%|█████████████████████████████▍ | 1.32G/1.65G [00:42<00:05, 59.6MB/s]
80%|█████████████████████████████▌ | 1.32G/1.65G [00:42<00:05, 61.9MB/s]
80%|█████████████████████████████▋ | 1.33G/1.65G [00:42<00:05, 63.5MB/s]
81%|█████████████████████████████▉ | 1.34G/1.65G [00:42<00:04, 64.6MB/s]
81%|██████████████████████████████ | 1.34G/1.65G [00:42<00:04, 65.5MB/s]
82%|██████████████████████████████▏ | 1.35G/1.65G [00:42<00:04, 66.3MB/s]
82%|██████████████████████████████▎ | 1.36G/1.65G [00:43<00:04, 66.6MB/s]
82%|██████████████████████████████▌ | 1.36G/1.65G [00:43<00:04, 66.9MB/s]
83%|██████████████████████████████▋ | 1.37G/1.65G [00:43<00:04, 67.1MB/s]
83%|██████████████████████████████▊ | 1.38G/1.65G [00:43<00:04, 67.4MB/s]
84%|██████████████████████████████▉ | 1.38G/1.65G [00:43<00:03, 67.5MB/s]
84%|███████████████████████████████ | 1.39G/1.65G [00:43<00:03, 67.7MB/s]
84%|███████████████████████████████▎ | 1.40G/1.65G [00:43<00:03, 67.1MB/s]
85%|███████████████████████████████▍ | 1.40G/1.65G [00:43<00:03, 67.2MB/s]
85%|███████████████████████████████▌ | 1.41G/1.65G [00:43<00:03, 67.1MB/s]
86%|███████████████████████████████▋ | 1.42G/1.65G [00:43<00:03, 67.1MB/s]
86%|███████████████████████████████▊ | 1.42G/1.65G [00:44<00:03, 67.1MB/s]
87%|████████████████████████████████ | 1.43G/1.65G [00:44<00:03, 67.4MB/s]
87%|████████████████████████████████▏ | 1.44G/1.65G [00:44<00:03, 67.1MB/s]
87%|████████████████████████████████▎ | 1.44G/1.65G [00:44<00:03, 67.3MB/s]
88%|████████████████████████████████▍ | 1.45G/1.65G [00:44<00:03, 67.1MB/s]
88%|████████████████████████████████▌ | 1.46G/1.65G [00:44<00:02, 67.3MB/s]
89%|████████████████████████████████▊ | 1.46G/1.65G [00:44<00:02, 67.5MB/s]
89%|████████████████████████████████▉ | 1.47G/1.65G [00:44<00:02, 67.5MB/s]
89%|█████████████████████████████████ | 1.48G/1.65G [00:44<00:02, 67.5MB/s]
90%|█████████████████████████████████▏ | 1.48G/1.65G [00:44<00:02, 67.3MB/s]
90%|█████████████████████████████████▍ | 1.49G/1.65G [00:45<00:02, 67.3MB/s]
91%|█████████████████████████████████▌ | 1.50G/1.65G [00:45<00:02, 67.3MB/s]
91%|█████████████████████████████████▋ | 1.50G/1.65G [00:45<00:02, 67.2MB/s]
91%|█████████████████████████████████▊ | 1.51G/1.65G [00:45<00:02, 67.2MB/s]
92%|█████████████████████████████████▉ | 1.52G/1.65G [00:45<00:02, 67.1MB/s]
92%|██████████████████████████████████▏ | 1.52G/1.65G [00:45<00:01, 64.6MB/s]
93%|██████████████████████████████████▎ | 1.53G/1.65G [00:45<00:01, 63.7MB/s]
93%|██████████████████████████████████▍ | 1.54G/1.65G [00:45<00:01, 60.5MB/s]
93%|██████████████████████████████████▌ | 1.54G/1.65G [00:45<00:01, 60.6MB/s]
94%|██████████████████████████████████▋ | 1.55G/1.65G [00:46<00:01, 61.7MB/s]
94%|██████████████████████████████████▊ | 1.56G/1.65G [00:46<00:01, 48.5MB/s]
95%|██████████████████████████████████▉ | 1.56G/1.65G [00:46<00:01, 51.5MB/s]
95%|███████████████████████████████████ | 1.57G/1.65G [00:46<00:01, 54.4MB/s]
95%|███████████████████████████████████▎ | 1.58G/1.65G [00:46<00:01, 57.5MB/s]
96%|███████████████████████████████████▍ | 1.58G/1.65G [00:46<00:01, 60.2MB/s]
96%|███████████████████████████████████▌ | 1.59G/1.65G [00:46<00:01, 62.1MB/s]
97%|███████████████████████████████████▋ | 1.60G/1.65G [00:46<00:00, 63.6MB/s]
97%|███████████████████████████████████▊ | 1.60G/1.65G [00:46<00:00, 64.7MB/s]
97%|████████████████████████████████████ | 1.61G/1.65G [00:46<00:00, 65.4MB/s]
98%|████████████████████████████████████▏| 1.62G/1.65G [00:47<00:00, 66.1MB/s]
98%|████████████████████████████████████▎| 1.62G/1.65G [00:47<00:00, 66.8MB/s]
99%|████████████████████████████████████▍| 1.63G/1.65G [00:47<00:00, 67.0MB/s]
99%|████████████████████████████████████▌| 1.64G/1.65G [00:47<00:00, 67.2MB/s]
99%|████████████████████████████████████▊| 1.64G/1.65G [00:47<00:00, 67.3MB/s]
100%|████████████████████████████████████▉| 1.65G/1.65G [00:47<00:00, 67.5MB/s]
0%| | 0.00/1.65G [00:00<?, ?B/s]
100%|█████████████████████████████████████| 1.65G/1.65G [00:00<00:00, 7.87TB/s]
Attempting to create new mne-python configuration file:
/home/runner/.mne/mne-python.json
Download complete in 01m17s (1576.2 MB)
Opening raw data file /home/runner/mne_data/MNE-sample-data/MEG/sample/sample_audvis_raw.fif...
Read a total of 3 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Range : 25800 ... 192599 = 42.956 ... 320.670 secs
Ready.
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
Reading 0 ... 36037 = 0.000 ... 60.000 secs...
Note
Before applying ICA (or any artifact repair strategy), be sure to observe the artifacts in your data to make sure you choose the right repair tool. Sometimes the right tool is no tool at all — if the artifacts are small enough you may not even need to repair them to get good analysis results. See Overview of artifact detection for guidance on detecting and visualizing various types of artifact.
Example: EOG and ECG artifact repair#
Visualizing the artifacts#
Let’s begin by visualizing the artifacts that we want to repair. In this dataset they are big enough to see easily in the raw data:
# pick some channels that clearly show heartbeats and blinks
artifact_picks = mne.pick_channels_regexp(raw.ch_names, regexp=r"(EEG 00.)")
raw.plot(order=artifact_picks, n_channels=len(artifact_picks), show_scrollbars=False)
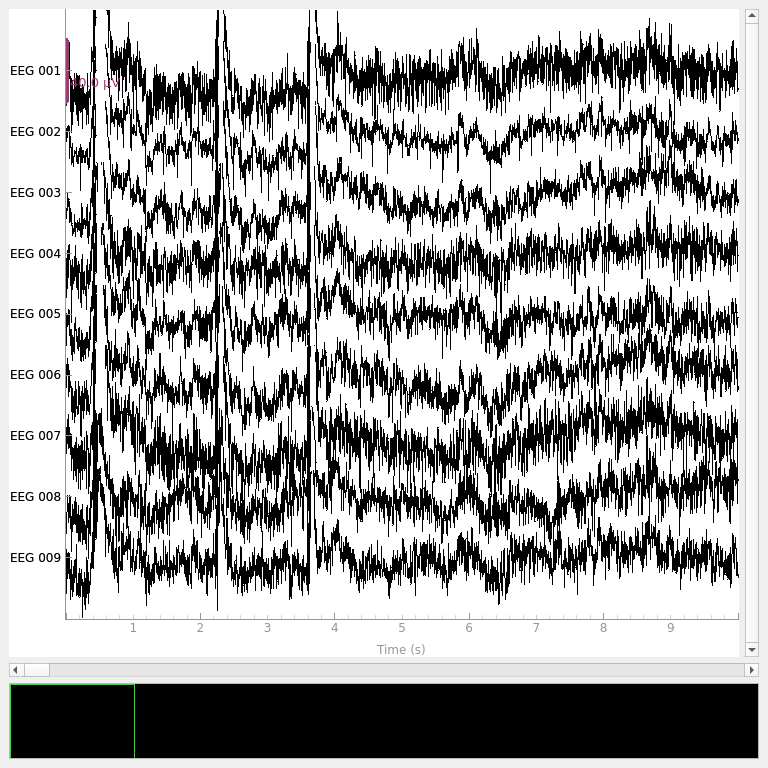
Filtering to remove slow drifts#
Before we run the ICA, an important step is filtering the data to remove
low-frequency drifts, which can negatively affect the quality of the ICA fit.
The slow drifts are problematic because they reduce the independence of the
assumed-to-be-independent sources (e.g., during a slow upward drift, the
neural, heartbeat, blink, and other muscular sources will all tend to have
higher values), making it harder for the algorithm to find an accurate
solution. A high-pass filter with 1 Hz cutoff frequency is recommended.
However, because filtering is a linear operation, the ICA solution found from
the filtered signal can be applied to the unfiltered signal (see
[2] for more information), so we’ll keep a copy of
the unfiltered Raw
object around so we can apply the ICA solution
to it later.
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 1e+02 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 0.50 Hz)
- Upper passband edge: 100.00 Hz
- Upper transition bandwidth: 25.00 Hz (-6 dB cutoff frequency: 112.50 Hz)
- Filter length: 1983 samples (3.302 s)
[Parallel(n_jobs=1)]: Done 17 tasks | elapsed: 0.0s
Fitting and plotting the ICA solution#
Now we’re ready to set up and fit the ICA. Since we know (from observing our
raw data) that the EOG and ECG artifacts are fairly strong, we would expect
those artifacts to be captured in the first few dimensions of the PCA
decomposition that happens before the ICA. Therefore, we probably don’t need
a huge number of components to do a good job of isolating our artifacts
(though it is usually preferable to include more components for a more
accurate solution). As a first guess, we’ll run ICA with n_components=15
(use only the first 15 PCA components to compute the ICA decomposition) — a
very small number given that our data has 59 good EEG channels, but with the
advantage that it will run quickly and we will able to tell easily whether it
worked or not (because we already know what the EOG / ECG artifacts should
look like).
ICA fitting is not deterministic (e.g., the components may get a sign flip on different runs, or may not always be returned in the same order), so we’ll also specify a random seed so that we get identical results each time this tutorial is built by our web servers.
Before fitting ICA, we will apply a common average referencing, to comply with the ICLabel requirements.
filt_raw = filt_raw.set_eeg_reference("average")
EEG channel type selected for re-referencing
Applying average reference.
Applying a custom ('EEG',) reference.
We will use the ‘extended infomax’ method for fitting the ICA, to comply with the ICLabel requirements. ICLabel was not tested with other ICA decomposition algorithm, but its performance and accuracy should not be impacted by the algorithm.
Fitting ICA to data using 59 channels (please be patient, this may take a while)
Selecting by number: 15 components
Computing Extended Infomax ICA
Fitting ICA took 5.5s.
Some optional parameters that we could have passed to the
fit
method include decim
(to use only
every Nth sample in computing the ICs, which can yield a considerable
speed-up) and reject
(for providing a rejection dictionary for maximum
acceptable peak-to-peak amplitudes for each channel type, just like we used
when creating epoched data in the Overview of MEG/EEG analysis with MNE-Python tutorial).
Now we can examine the ICs to see what they captured.
plot_sources
will show the time series of the
ICs. Note that in our call to plot_sources
we
can use the original, unfiltered Raw
object:
raw.load_data()
ica.plot_sources(raw, show_scrollbars=False, show=True)
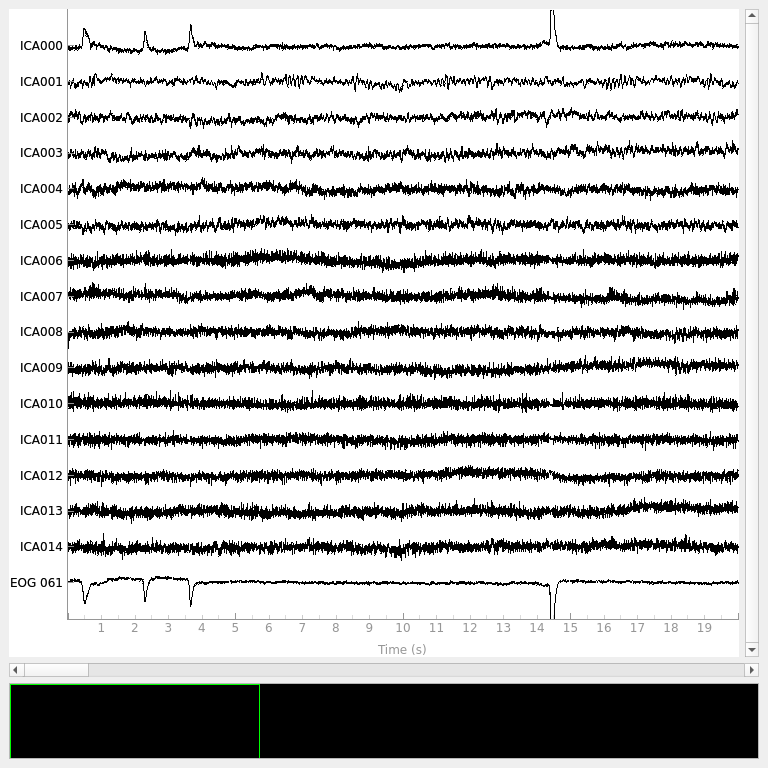
Creating RawArray with float64 data, n_channels=16, n_times=36038
Range : 25800 ... 61837 = 42.956 ... 102.956 secs
Ready.
Here we can pretty clearly see that the first component (ICA000
) captures
the EOG signal quite well (for more info on visually identifying Independent
Components, this EEGLAB tutorial is a good resource). We can also
visualize the scalp field distribution of each component using
plot_components
. These are interpolated based
on the values in the ICA mixing matrix:
ica.plot_components()
# blinks
ica.plot_overlay(raw, exclude=[0], picks="eeg")
Applying ICA to Raw instance
Transforming to ICA space (15 components)
Zeroing out 1 ICA component
Projecting back using 59 PCA components
We can also plot some diagnostics of IC using
plot_properties
:
ica.plot_properties(raw, picks=[0])
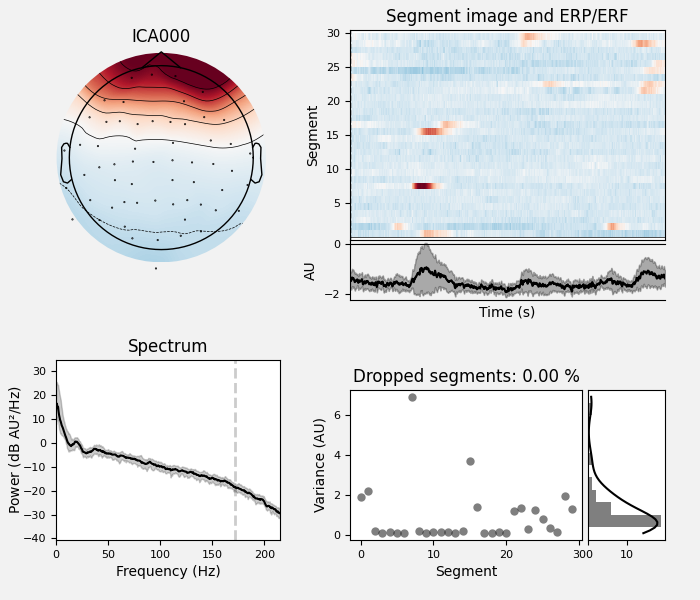
Using multitaper spectrum estimation with 7 DPSS windows
Not setting metadata
30 matching events found
No baseline correction applied
0 projection items activated
Selecting ICA components automatically#
Now that we’ve explored what components need to be removed, we can apply the automatic ICA component labeling algorithm, which will assign a probability value for each component being one of:
brain
muscle artifact
eye blink
heart beat
line noise
channel noise
other
The output of the ICLabel label_components
function produces
predicted probability values for each of these classes in that order.
See [1] for full details.
ic_labels = label_components(filt_raw, ica, method="iclabel")
# ICA0 was correctly identified as an eye blink, whereas ICA12 was
# classified as a muscle artifact.
print(ic_labels["labels"])
ica.plot_properties(raw, picks=[0, 12], verbose=False)
['eye blink', 'brain', 'brain', 'brain', 'brain', 'brain', 'muscle artifact', 'muscle artifact', 'other', 'brain', 'muscle artifact', 'muscle artifact', 'muscle artifact', 'muscle artifact', 'eye blink']
Extract Labels and Reconstruct Raw Data#
We can extract the labels of each component and exclude non-brain classified components, keeping ‘brain’ and ‘other’. “Other” is a catch-all that for non-classifiable components. We will stay on the side of caution and assume we cannot blindly remove these.
labels = ic_labels["labels"]
exclude_idx = [
idx for idx, label in enumerate(labels) if label not in ["brain", "other"]
]
print(f"Excluding these ICA components: {exclude_idx}")
Excluding these ICA components: [0, 6, 7, 10, 11, 12, 13, 14]
Now that the exclusions have been set, we can reconstruct the sensor signals
with artifacts removed using the apply
method
(remember, we’re applying the ICA solution from the filtered data to the
original unfiltered signal). Plotting the original raw data alongside the
reconstructed data shows that the heartbeat and blink artifacts are repaired.
# ica.apply() changes the Raw object in-place, so let's make a copy first:
reconst_raw = raw.copy()
ica.apply(reconst_raw, exclude=exclude_idx)
raw.plot(order=artifact_picks, n_channels=len(artifact_picks), show_scrollbars=False)
reconst_raw.plot(
order=artifact_picks, n_channels=len(artifact_picks), show_scrollbars=False
)
del reconst_raw
Applying ICA to Raw instance
Transforming to ICA space (15 components)
Zeroing out 8 ICA components
Projecting back using 59 PCA components
References#
Total running time of the script: (1 minutes 37.743 seconds)
Estimated memory usage: 505 MB