Note
Click here to download the full example code
Decoding in time-frequency space using Common Spatial Patterns (CSP)¶
The time-frequency decomposition is estimated by iterating over raw data that has been band-passed at different frequencies. This is used to compute a covariance matrix over each epoch or a rolling time-window and extract the CSP filtered signals. A linear discriminant classifier is then applied to these signals.
# Authors: Laura Gwilliams <laura.gwilliams@nyu.edu>
# Jean-Remi King <jeanremi.king@gmail.com>
# Alex Barachant <alexandre.barachant@gmail.com>
# Alexandre Gramfort <alexandre.gramfort@inria.fr>
#
# License: BSD (3-clause)
import numpy as np
import matplotlib.pyplot as plt
from mne import Epochs, create_info, events_from_annotations
from mne.io import concatenate_raws, read_raw_edf
from mne.datasets import eegbci
from mne.decoding import CSP
from mne.time_frequency import AverageTFR
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.model_selection import StratifiedKFold, cross_val_score
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import LabelEncoder
Set parameters and read data
event_id = dict(hands=2, feet=3) # motor imagery: hands vs feet
subject = 1
runs = [6, 10, 14]
raw_fnames = eegbci.load_data(subject, runs)
raw = concatenate_raws([read_raw_edf(f) for f in raw_fnames])
# Extract information from the raw file
sfreq = raw.info['sfreq']
events, _ = events_from_annotations(raw, event_id=dict(T1=2, T2=3))
raw.pick_types(meg=False, eeg=True, stim=False, eog=False, exclude='bads')
raw.load_data()
# Assemble the classifier using scikit-learn pipeline
clf = make_pipeline(CSP(n_components=4, reg=None, log=True, norm_trace=False),
LinearDiscriminantAnalysis())
n_splits = 5 # how many folds to use for cross-validation
cv = StratifiedKFold(n_splits=n_splits, shuffle=True, random_state=42)
# Classification & time-frequency parameters
tmin, tmax = -.200, 2.000
n_cycles = 10. # how many complete cycles: used to define window size
min_freq = 5.
max_freq = 25.
n_freqs = 8 # how many frequency bins to use
# Assemble list of frequency range tuples
freqs = np.linspace(min_freq, max_freq, n_freqs) # assemble frequencies
freq_ranges = list(zip(freqs[:-1], freqs[1:])) # make freqs list of tuples
# Infer window spacing from the max freq and number of cycles to avoid gaps
window_spacing = (n_cycles / np.max(freqs) / 2.)
centered_w_times = np.arange(tmin, tmax, window_spacing)[1:]
n_windows = len(centered_w_times)
# Instantiate label encoder
le = LabelEncoder()
Out:
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R06.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R10.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R14.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Used Annotations descriptions: ['T1', 'T2']
Reading 0 ... 59999 = 0.000 ... 374.994 secs...
Loop through frequencies, apply classifier and save scores
# init scores
freq_scores = np.zeros((n_freqs - 1,))
# Loop through each frequency range of interest
for freq, (fmin, fmax) in enumerate(freq_ranges):
# Infer window size based on the frequency being used
w_size = n_cycles / ((fmax + fmin) / 2.) # in seconds
# Apply band-pass filter to isolate the specified frequencies
raw_filter = raw.copy().filter(fmin, fmax, n_jobs=1, fir_design='firwin',
skip_by_annotation='edge')
# Extract epochs from filtered data, padded by window size
epochs = Epochs(raw_filter, events, event_id, tmin - w_size, tmax + w_size,
proj=False, baseline=None, preload=True)
epochs.drop_bad()
y = le.fit_transform(epochs.events[:, 2])
X = epochs.get_data()
# Save mean scores over folds for each frequency and time window
freq_scores[freq] = np.mean(cross_val_score(estimator=clf, X=X, y=y,
scoring='roc_auc', cv=cv,
n_jobs=1), axis=0)
Out:
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 5 - 7.9 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 5.00
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 4.00 Hz)
- Upper passband edge: 7.86 Hz
- Upper transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 8.86 Hz)
- Filter length: 265 samples (1.656 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 851 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00018 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00019 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00018 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0002 (2.2e-16 eps * 64 dim * 1.4e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00019 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0002 (2.2e-16 eps * 64 dim * 1.4e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00019 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00019 (2.2e-16 eps * 64 dim * 1.4e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00018 (2.2e-16 eps * 64 dim * 1.3e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0002 (2.2e-16 eps * 64 dim * 1.4e+10 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 7.9 - 11 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 7.86
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 6.86 Hz)
- Upper passband edge: 10.71 Hz
- Upper transition bandwidth: 2.68 Hz (-6 dB cutoff frequency: 12.05 Hz)
- Filter length: 265 samples (1.656 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 697 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00013 (2.2e-16 eps * 64 dim * 9.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00013 (2.2e-16 eps * 64 dim * 9.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00013 (2.2e-16 eps * 64 dim * 9.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 11 - 14 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.71
- Lower transition bandwidth: 2.68 Hz (-6 dB cutoff frequency: 9.38 Hz)
- Upper passband edge: 13.57 Hz
- Upper transition bandwidth: 3.39 Hz (-6 dB cutoff frequency: 15.27 Hz)
- Filter length: 199 samples (1.244 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 617 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00012 (2.2e-16 eps * 64 dim * 8.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00013 (2.2e-16 eps * 64 dim * 8.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 14 - 16 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 13.57
- Lower transition bandwidth: 3.39 Hz (-6 dB cutoff frequency: 11.88 Hz)
- Upper passband edge: 16.43 Hz
- Upper transition bandwidth: 4.11 Hz (-6 dB cutoff frequency: 18.48 Hz)
- Filter length: 157 samples (0.981 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 567 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 9.6e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.2e-05 (2.2e-16 eps * 64 dim * 6.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.7e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.8e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.3e-05 (2.2e-16 eps * 64 dim * 6.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 16 - 19 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 16.43
- Lower transition bandwidth: 4.11 Hz (-6 dB cutoff frequency: 14.38 Hz)
- Upper passband edge: 19.29 Hz
- Upper transition bandwidth: 4.82 Hz (-6 dB cutoff frequency: 21.70 Hz)
- Filter length: 129 samples (0.806 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 533 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.1e-05 (2.2e-16 eps * 64 dim * 5.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.4e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.2e-05 (2.2e-16 eps * 64 dim * 5.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.2e-05 (2.2e-16 eps * 64 dim * 5.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.1e-05 (2.2e-16 eps * 64 dim * 5.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.2e-05 (2.2e-16 eps * 64 dim * 5.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 19 - 22 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 19.29
- Lower transition bandwidth: 4.82 Hz (-6 dB cutoff frequency: 16.88 Hz)
- Upper passband edge: 22.14 Hz
- Upper transition bandwidth: 5.54 Hz (-6 dB cutoff frequency: 24.91 Hz)
- Filter length: 111 samples (0.694 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 507 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 22 - 25 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 22.14
- Lower transition bandwidth: 5.54 Hz (-6 dB cutoff frequency: 19.38 Hz)
- Upper passband edge: 25.00 Hz
- Upper transition bandwidth: 6.25 Hz (-6 dB cutoff frequency: 28.12 Hz)
- Filter length: 97 samples (0.606 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 489 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Plot frequency results
plt.bar(freqs[:-1], freq_scores, width=np.diff(freqs)[0],
align='edge', edgecolor='black')
plt.xticks(freqs)
plt.ylim([0, 1])
plt.axhline(len(epochs['feet']) / len(epochs), color='k', linestyle='--',
label='chance level')
plt.legend()
plt.xlabel('Frequency (Hz)')
plt.ylabel('Decoding Scores')
plt.title('Frequency Decoding Scores')
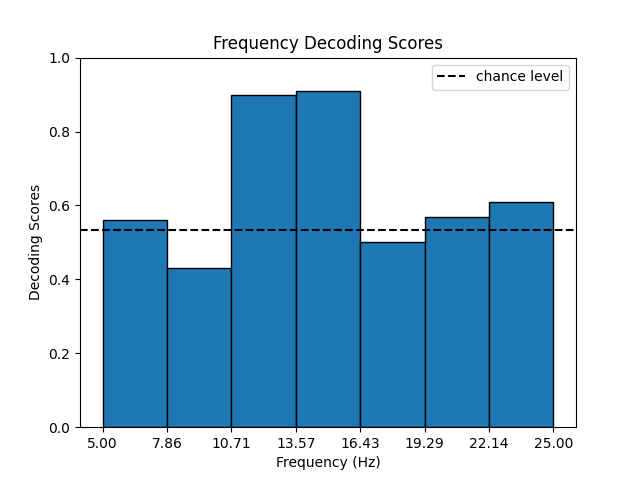
Loop through frequencies and time, apply classifier and save scores
# init scores
tf_scores = np.zeros((n_freqs - 1, n_windows))
# Loop through each frequency range of interest
for freq, (fmin, fmax) in enumerate(freq_ranges):
# Infer window size based on the frequency being used
w_size = n_cycles / ((fmax + fmin) / 2.) # in seconds
# Apply band-pass filter to isolate the specified frequencies
raw_filter = raw.copy().filter(fmin, fmax, n_jobs=1, fir_design='firwin',
skip_by_annotation='edge')
# Extract epochs from filtered data, padded by window size
epochs = Epochs(raw_filter, events, event_id, tmin - w_size, tmax + w_size,
proj=False, baseline=None, preload=True)
epochs.drop_bad()
y = le.fit_transform(epochs.events[:, 2])
# Roll covariance, csp and lda over time
for t, w_time in enumerate(centered_w_times):
# Center the min and max of the window
w_tmin = w_time - w_size / 2.
w_tmax = w_time + w_size / 2.
# Crop data into time-window of interest
X = epochs.copy().crop(w_tmin, w_tmax).get_data()
# Save mean scores over folds for each frequency and time window
tf_scores[freq, t] = np.mean(cross_val_score(estimator=clf, X=X, y=y,
scoring='roc_auc', cv=cv,
n_jobs=1), axis=0)
Out:
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 5 - 7.9 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 5.00
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 4.00 Hz)
- Upper passband edge: 7.86 Hz
- Upper transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 8.86 Hz)
- Filter length: 265 samples (1.656 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 851 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.8e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.6e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.7e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.7e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.6e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.8e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.8e-05 (2.2e-16 eps * 64 dim * 6.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.4e-05 (2.2e-16 eps * 64 dim * 6.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.5e-05 (2.2e-16 eps * 64 dim * 6.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.4e-05 (2.2e-16 eps * 64 dim * 6.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.9e-05 (2.2e-16 eps * 64 dim * 7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.5e-05 (2.2e-16 eps * 64 dim * 6.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.5e-05 (2.2e-16 eps * 64 dim * 6.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.6e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.7e-05 (2.2e-16 eps * 64 dim * 6.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.0001 (2.2e-16 eps * 64 dim * 7.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 7.9 - 11 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 7.86
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 6.86 Hz)
- Upper passband edge: 10.71 Hz
- Upper transition bandwidth: 2.68 Hz (-6 dB cutoff frequency: 12.05 Hz)
- Filter length: 265 samples (1.656 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 697 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.4e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.2e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.6e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.4e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 11 - 14 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.71
- Lower transition bandwidth: 2.68 Hz (-6 dB cutoff frequency: 9.38 Hz)
- Upper passband edge: 13.57 Hz
- Upper transition bandwidth: 3.39 Hz (-6 dB cutoff frequency: 15.27 Hz)
- Filter length: 199 samples (1.244 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 617 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.9e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.2e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.1e-05 (2.2e-16 eps * 64 dim * 3.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.9e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.2e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.1e-05 (2.2e-16 eps * 64 dim * 3.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.3e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.2e-05 (2.2e-16 eps * 64 dim * 3.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.4e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 14 - 16 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 13.57
- Lower transition bandwidth: 3.39 Hz (-6 dB cutoff frequency: 11.88 Hz)
- Upper passband edge: 16.43 Hz
- Upper transition bandwidth: 4.11 Hz (-6 dB cutoff frequency: 18.48 Hz)
- Filter length: 157 samples (0.981 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 567 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.8e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.9e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.8e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.8e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.9e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.8e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.8e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 16 - 19 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 16.43
- Lower transition bandwidth: 4.11 Hz (-6 dB cutoff frequency: 14.38 Hz)
- Upper passband edge: 19.29 Hz
- Upper transition bandwidth: 4.82 Hz (-6 dB cutoff frequency: 21.70 Hz)
- Filter length: 129 samples (0.806 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 533 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.4e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.8e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.5e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 19 - 22 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 19.29
- Lower transition bandwidth: 4.82 Hz (-6 dB cutoff frequency: 16.88 Hz)
- Upper passband edge: 22.14 Hz
- Upper transition bandwidth: 5.54 Hz (-6 dB cutoff frequency: 24.91 Hz)
- Filter length: 111 samples (0.694 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 507 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.9e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.3e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.2e-05 (2.2e-16 eps * 64 dim * 2.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.1e-05 (2.2e-16 eps * 64 dim * 2.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3e-05 (2.2e-16 eps * 64 dim * 2.1e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 22 - 25 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 22.14
- Lower transition bandwidth: 5.54 Hz (-6 dB cutoff frequency: 19.38 Hz)
- Upper passband edge: 25.00 Hz
- Upper transition bandwidth: 6.25 Hz (-6 dB cutoff frequency: 28.12 Hz)
- Filter length: 97 samples (0.606 sec)
Not setting metadata
Not setting metadata
45 matching events found
No baseline correction applied
Loading data for 45 events and 489 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.8e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.7e-05 (2.2e-16 eps * 64 dim * 1.2e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.8e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.8e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 1.9e-05 (2.2e-16 eps * 64 dim * 1.3e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2e-05 (2.2e-16 eps * 64 dim * 1.4e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.5e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.1e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.2e-05 (2.2e-16 eps * 64 dim * 1.5e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.7e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.8e-05 (2.2e-16 eps * 64 dim * 1.9e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.3e-05 (2.2e-16 eps * 64 dim * 1.6e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.6e-05 (2.2e-16 eps * 64 dim * 1.8e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 2.4e-05 (2.2e-16 eps * 64 dim * 1.7e+09 max singular value)
Estimated rank (mag): 64
MAG: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating covariance using EMPIRICAL
Done.
Plot time-frequency results
# Set up time frequency object
av_tfr = AverageTFR(create_info(['freq'], sfreq), tf_scores[np.newaxis, :],
centered_w_times, freqs[1:], 1)
chance = np.mean(y) # set chance level to white in the plot
av_tfr.plot([0], vmin=chance, title="Time-Frequency Decoding Scores",
cmap=plt.cm.Reds)
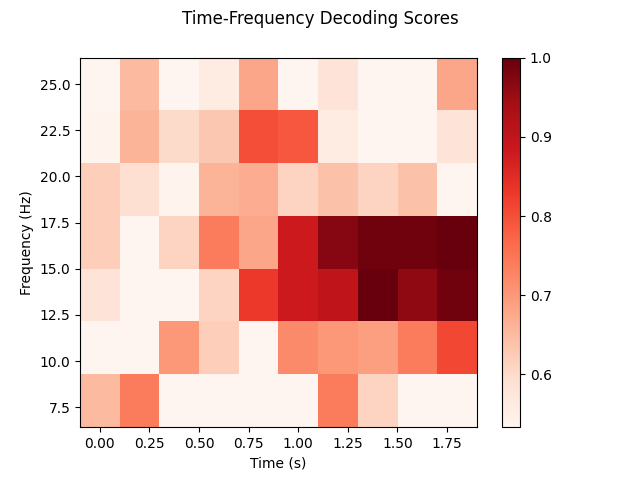
Out:
No baseline correction applied
Total running time of the script: ( 1 minutes 29.610 seconds)
Estimated memory usage: 8 MB