Note
Go to the end to download the full example code.
Read BIDS datasets#
When working with electrophysiological data in the BIDS format, an important resource is the OpenNeuro database. OpenNeuro works great with MNE-BIDS because every dataset must pass a validator that tests to ensure its format meets BIDS specifications before the dataset can be uploaded, so you know the data will work with a script like in this example without modification.
We have various data types that can be loaded via the read_raw_bids
function:
MEG
EEG (scalp electrodes)
iEEG (ECoG and SEEG)
the anatomical MRI scan of a study participant
In this tutorial, we show how read_raw_bids
can be used to load and
inspect BIDS-formatted data.
# Authors: The MNE-BIDS developers
# SPDX-License-Identifier: BSD-3-Clause
Imports#
We are importing everything we need for this example:
import os
import os.path as op
import openneuro
from mne.datasets import sample
from mne_bids import (
BIDSPath,
find_matching_paths,
get_entity_vals,
make_report,
print_dir_tree,
read_raw_bids,
)
Download a subject’s data from an OpenNeuro BIDS dataset#
Download the data, storing each in a target_dir
target directory, which,
in mne-bids
terminology, is the root of each BIDS dataset. This example
uses this EEG dataset of
resting-state recordings of patients with Parkinson’s disease.
# .. note: If the keyword argument include is left out of
# ``openneuro.download``, the whole dataset will be downloaded.
# We're just using data from one subject to reduce the time
# it takes to run the example.
dataset = "ds002778"
subject = "pd6"
# Download one subject's data from each dataset
bids_root = op.join(op.dirname(sample.data_path()), dataset)
if not op.isdir(bids_root):
os.makedirs(bids_root)
openneuro.download(dataset=dataset, target_dir=bids_root, include=[f"sub-{subject}"])
Using default location ~/mne_data for sample...
Creating /home/runner/mne_data
Downloading file 'MNE-sample-data-processed.tar.gz' from 'https://osf.io/86qa2/download?version=6' to '/home/runner/mne_data'.
0%| | 0.00/1.65G [00:00<?, ?B/s]
0%| | 5.32M/1.65G [00:00<00:30, 53.2MB/s]
1%|▎ | 11.2M/1.65G [00:00<00:29, 56.4MB/s]
1%|▍ | 17.1M/1.65G [00:00<00:28, 57.6MB/s]
1%|▌ | 23.0M/1.65G [00:00<00:27, 58.4MB/s]
2%|▋ | 28.9M/1.65G [00:00<00:27, 58.5MB/s]
2%|▊ | 34.9M/1.65G [00:00<00:27, 58.8MB/s]
2%|▉ | 40.8M/1.65G [00:00<00:27, 59.1MB/s]
3%|█ | 46.8M/1.65G [00:00<00:27, 59.3MB/s]
3%|█▏ | 52.8M/1.65G [00:00<00:26, 59.5MB/s]
4%|█▎ | 58.7M/1.65G [00:01<00:26, 59.3MB/s]
4%|█▍ | 64.7M/1.65G [00:01<00:26, 59.4MB/s]
4%|█▌ | 70.6M/1.65G [00:01<00:26, 59.4MB/s]
5%|█▋ | 76.6M/1.65G [00:01<00:26, 59.2MB/s]
5%|█▊ | 82.6M/1.65G [00:01<00:26, 59.4MB/s]
5%|█▉ | 88.6M/1.65G [00:01<00:26, 59.6MB/s]
6%|██ | 94.6M/1.65G [00:01<00:26, 59.7MB/s]
6%|██▎ | 101M/1.65G [00:01<00:26, 59.7MB/s]
6%|██▍ | 107M/1.65G [00:01<00:25, 59.6MB/s]
7%|██▌ | 112M/1.65G [00:01<00:25, 59.6MB/s]
7%|██▋ | 118M/1.65G [00:02<00:25, 59.5MB/s]
8%|██▊ | 124M/1.65G [00:02<00:25, 59.4MB/s]
8%|██▉ | 130M/1.65G [00:02<00:25, 59.3MB/s]
8%|███▏ | 136M/1.65G [00:02<00:25, 59.4MB/s]
9%|███▎ | 142M/1.65G [00:02<00:25, 59.5MB/s]
9%|███▍ | 148M/1.65G [00:02<00:25, 59.6MB/s]
9%|███▌ | 154M/1.65G [00:02<00:25, 59.7MB/s]
10%|███▋ | 160M/1.65G [00:02<00:24, 59.7MB/s]
10%|███▊ | 166M/1.65G [00:02<00:24, 59.7MB/s]
10%|███▉ | 172M/1.65G [00:02<00:24, 59.6MB/s]
11%|████ | 178M/1.65G [00:03<00:24, 59.6MB/s]
11%|████▏ | 184M/1.65G [00:03<00:24, 59.7MB/s]
12%|████▎ | 190M/1.65G [00:03<00:24, 59.4MB/s]
12%|████▌ | 196M/1.65G [00:03<00:24, 59.6MB/s]
12%|████▋ | 202M/1.65G [00:03<00:24, 59.6MB/s]
13%|████▊ | 208M/1.65G [00:03<00:24, 59.6MB/s]
13%|████▉ | 214M/1.65G [00:03<00:24, 59.7MB/s]
13%|█████ | 220M/1.65G [00:03<00:24, 59.6MB/s]
14%|█████▏ | 226M/1.65G [00:03<00:23, 59.5MB/s]
14%|█████▎ | 232M/1.65G [00:03<00:23, 59.5MB/s]
14%|█████▍ | 238M/1.65G [00:04<00:23, 59.3MB/s]
15%|█████▌ | 244M/1.65G [00:04<00:23, 58.7MB/s]
15%|█████▋ | 250M/1.65G [00:04<00:23, 58.5MB/s]
15%|█████▉ | 256M/1.65G [00:04<00:23, 58.4MB/s]
16%|██████ | 261M/1.65G [00:04<00:23, 58.3MB/s]
16%|██████▏ | 267M/1.65G [00:04<00:23, 58.2MB/s]
17%|██████▎ | 273M/1.65G [00:04<00:23, 58.2MB/s]
17%|██████▍ | 279M/1.65G [00:04<00:23, 57.8MB/s]
17%|██████▌ | 285M/1.65G [00:04<00:23, 57.8MB/s]
18%|██████▋ | 290M/1.65G [00:04<00:23, 57.7MB/s]
18%|██████▊ | 296M/1.65G [00:05<00:23, 57.7MB/s]
18%|██████▉ | 302M/1.65G [00:05<00:23, 57.7MB/s]
19%|███████ | 308M/1.65G [00:05<00:23, 57.6MB/s]
19%|███████▏ | 314M/1.65G [00:05<00:23, 57.7MB/s]
19%|███████▎ | 319M/1.65G [00:05<00:23, 57.7MB/s]
20%|███████▍ | 325M/1.65G [00:05<00:23, 57.6MB/s]
20%|███████▌ | 331M/1.65G [00:05<00:22, 57.7MB/s]
20%|███████▋ | 337M/1.65G [00:05<00:22, 57.8MB/s]
21%|███████▊ | 342M/1.65G [00:05<00:22, 57.9MB/s]
21%|████████ | 348M/1.65G [00:05<00:22, 57.9MB/s]
21%|████████▏ | 354M/1.65G [00:06<00:22, 58.0MB/s]
22%|████████▎ | 360M/1.65G [00:06<00:22, 58.0MB/s]
22%|████████▍ | 366M/1.65G [00:06<00:22, 58.0MB/s]
22%|████████▌ | 372M/1.65G [00:06<00:22, 58.0MB/s]
23%|████████▋ | 377M/1.65G [00:06<00:21, 58.1MB/s]
23%|████████▊ | 383M/1.65G [00:06<00:21, 58.2MB/s]
24%|████████▉ | 389M/1.65G [00:06<00:21, 58.1MB/s]
24%|█████████ | 395M/1.65G [00:06<00:21, 57.6MB/s]
24%|█████████▏ | 401M/1.65G [00:06<00:21, 57.8MB/s]
25%|█████████▎ | 406M/1.65G [00:06<00:21, 58.0MB/s]
25%|█████████▍ | 412M/1.65G [00:07<00:21, 58.2MB/s]
25%|█████████▌ | 418M/1.65G [00:07<00:21, 58.2MB/s]
26%|█████████▋ | 424M/1.65G [00:07<00:21, 58.3MB/s]
26%|█████████▉ | 430M/1.65G [00:07<00:21, 58.1MB/s]
26%|██████████ | 436M/1.65G [00:07<00:21, 57.8MB/s]
27%|██████████▏ | 441M/1.65G [00:07<00:20, 57.7MB/s]
27%|██████████▎ | 447M/1.65G [00:07<00:20, 57.8MB/s]
27%|██████████▍ | 453M/1.65G [00:07<00:20, 58.0MB/s]
28%|██████████▌ | 459M/1.65G [00:07<00:20, 58.6MB/s]
28%|██████████▋ | 465M/1.65G [00:07<00:20, 59.0MB/s]
29%|██████████▊ | 471M/1.65G [00:08<00:19, 59.3MB/s]
29%|██████████▉ | 477M/1.65G [00:08<00:19, 59.5MB/s]
29%|███████████ | 483M/1.65G [00:08<00:19, 59.6MB/s]
30%|███████████▏ | 489M/1.65G [00:08<00:19, 59.8MB/s]
30%|███████████▍ | 495M/1.65G [00:08<00:19, 59.9MB/s]
30%|███████████▌ | 501M/1.65G [00:08<00:19, 60.0MB/s]
31%|███████████▋ | 507M/1.65G [00:08<00:19, 60.1MB/s]
31%|███████████▊ | 513M/1.65G [00:08<00:19, 59.8MB/s]
31%|███████████▉ | 519M/1.65G [00:08<00:19, 59.2MB/s]
32%|████████████ | 525M/1.65G [00:08<00:19, 58.8MB/s]
32%|████████████▏ | 531M/1.65G [00:09<00:19, 58.6MB/s]
32%|████████████▎ | 537M/1.65G [00:09<00:19, 58.3MB/s]
33%|████████████▍ | 543M/1.65G [00:09<00:19, 58.1MB/s]
33%|████████████▌ | 548M/1.65G [00:09<00:19, 58.1MB/s]
34%|████████████▋ | 554M/1.65G [00:09<00:18, 58.0MB/s]
34%|████████████▉ | 560M/1.65G [00:09<00:18, 58.0MB/s]
34%|█████████████ | 566M/1.65G [00:09<00:18, 58.0MB/s]
35%|█████████████▏ | 572M/1.65G [00:09<00:18, 58.0MB/s]
35%|█████████████▎ | 578M/1.65G [00:09<00:18, 58.1MB/s]
35%|█████████████▍ | 583M/1.65G [00:09<00:18, 57.9MB/s]
36%|█████████████▌ | 589M/1.65G [00:10<00:18, 57.6MB/s]
36%|█████████████▋ | 595M/1.65G [00:10<00:18, 57.8MB/s]
36%|█████████████▊ | 601M/1.65G [00:10<00:19, 54.8MB/s]
37%|█████████████▉ | 606M/1.65G [00:10<00:20, 50.5MB/s]
37%|██████████████ | 612M/1.65G [00:10<00:19, 52.6MB/s]
37%|██████████████▏ | 618M/1.65G [00:10<00:19, 54.3MB/s]
38%|██████████████▎ | 624M/1.65G [00:10<00:18, 55.1MB/s]
38%|██████████████▍ | 629M/1.65G [00:10<00:18, 56.0MB/s]
38%|██████████████▌ | 635M/1.65G [00:10<00:17, 56.6MB/s]
39%|██████████████▋ | 641M/1.65G [00:10<00:17, 57.2MB/s]
39%|██████████████▊ | 647M/1.65G [00:11<00:17, 57.5MB/s]
40%|███████████████ | 653M/1.65G [00:11<00:17, 58.2MB/s]
40%|███████████████▏ | 659M/1.65G [00:11<00:16, 58.9MB/s]
40%|███████████████▎ | 665M/1.65G [00:11<00:16, 59.3MB/s]
41%|███████████████▍ | 671M/1.65G [00:11<00:16, 59.6MB/s]
41%|███████████████▌ | 677M/1.65G [00:11<00:16, 59.7MB/s]
41%|███████████████▋ | 683M/1.65G [00:11<00:16, 59.9MB/s]
42%|███████████████▊ | 689M/1.65G [00:11<00:16, 59.8MB/s]
42%|███████████████▉ | 695M/1.65G [00:11<00:16, 59.5MB/s]
42%|████████████████ | 701M/1.65G [00:11<00:16, 59.5MB/s]
43%|████████████████▎ | 707M/1.65G [00:12<00:15, 59.5MB/s]
43%|████████████████▍ | 713M/1.65G [00:12<00:15, 59.6MB/s]
43%|████████████████▌ | 719M/1.65G [00:12<00:15, 59.7MB/s]
44%|████████████████▋ | 725M/1.65G [00:12<00:15, 59.7MB/s]
44%|████████████████▊ | 731M/1.65G [00:12<00:15, 59.5MB/s]
45%|████████████████▉ | 737M/1.65G [00:12<00:15, 59.4MB/s]
45%|█████████████████ | 743M/1.65G [00:12<00:15, 59.6MB/s]
45%|█████████████████▏ | 749M/1.65G [00:12<00:15, 59.6MB/s]
46%|█████████████████▎ | 755M/1.65G [00:12<00:15, 59.8MB/s]
46%|█████████████████▍ | 761M/1.65G [00:12<00:14, 59.8MB/s]
46%|█████████████████▋ | 767M/1.65G [00:13<00:14, 59.9MB/s]
47%|█████████████████▊ | 773M/1.65G [00:13<00:14, 59.9MB/s]
47%|█████████████████▉ | 779M/1.65G [00:13<00:14, 59.8MB/s]
47%|██████████████████ | 785M/1.65G [00:13<00:14, 59.8MB/s]
48%|██████████████████▏ | 791M/1.65G [00:13<00:14, 59.7MB/s]
48%|██████████████████▎ | 797M/1.65G [00:13<00:14, 59.7MB/s]
49%|██████████████████▍ | 803M/1.65G [00:13<00:14, 59.8MB/s]
49%|██████████████████▌ | 809M/1.65G [00:13<00:14, 59.1MB/s]
49%|██████████████████▋ | 815M/1.65G [00:13<00:14, 59.4MB/s]
50%|██████████████████▊ | 821M/1.65G [00:14<00:14, 59.4MB/s]
50%|███████████████████ | 827M/1.65G [00:14<00:14, 56.2MB/s]
50%|███████████████████▏ | 832M/1.65G [00:14<00:14, 56.9MB/s]
51%|███████████████████▎ | 839M/1.65G [00:14<00:13, 59.4MB/s]
51%|███████████████████▍ | 846M/1.65G [00:14<00:13, 61.3MB/s]
52%|███████████████████▌ | 852M/1.65G [00:14<00:12, 62.6MB/s]
52%|███████████████████▋ | 859M/1.65G [00:14<00:12, 63.6MB/s]
52%|███████████████████▉ | 865M/1.65G [00:14<00:12, 64.2MB/s]
53%|████████████████████ | 872M/1.65G [00:14<00:12, 64.7MB/s]
53%|████████████████████▏ | 879M/1.65G [00:14<00:11, 65.2MB/s]
54%|████████████████████▎ | 885M/1.65G [00:15<00:11, 65.4MB/s]
54%|████████████████████▌ | 892M/1.65G [00:15<00:11, 65.6MB/s]
54%|████████████████████▋ | 898M/1.65G [00:15<00:12, 59.7MB/s]
55%|████████████████████▊ | 905M/1.65G [00:15<00:12, 61.4MB/s]
55%|████████████████████▉ | 911M/1.65G [00:15<00:11, 62.6MB/s]
56%|█████████████████████ | 918M/1.65G [00:15<00:11, 63.6MB/s]
56%|█████████████████████▎ | 925M/1.65G [00:15<00:11, 64.3MB/s]
56%|█████████████████████▍ | 931M/1.65G [00:15<00:11, 64.0MB/s]
57%|█████████████████████▌ | 938M/1.65G [00:15<00:11, 64.6MB/s]
57%|█████████████████████▋ | 944M/1.65G [00:15<00:10, 65.0MB/s]
58%|█████████████████████▊ | 951M/1.65G [00:16<00:10, 64.8MB/s]
58%|██████████████████████ | 957M/1.65G [00:16<00:10, 65.1MB/s]
58%|██████████████████████▏ | 964M/1.65G [00:16<00:10, 65.3MB/s]
59%|██████████████████████▎ | 971M/1.65G [00:16<00:10, 65.4MB/s]
59%|██████████████████████▍ | 977M/1.65G [00:16<00:10, 65.3MB/s]
60%|██████████████████████▌ | 984M/1.65G [00:16<00:10, 65.5MB/s]
60%|██████████████████████▊ | 990M/1.65G [00:16<00:10, 65.5MB/s]
60%|██████████████████████▉ | 997M/1.65G [00:16<00:10, 64.9MB/s]
61%|██████████████████████▍ | 1.00G/1.65G [00:16<00:09, 65.1MB/s]
61%|██████████████████████▌ | 1.01G/1.65G [00:16<00:09, 65.3MB/s]
62%|██████████████████████▊ | 1.02G/1.65G [00:17<00:09, 65.5MB/s]
62%|██████████████████████▉ | 1.02G/1.65G [00:17<00:09, 65.5MB/s]
62%|███████████████████████ | 1.03G/1.65G [00:17<00:09, 65.6MB/s]
63%|███████████████████████▏ | 1.04G/1.65G [00:17<00:09, 65.7MB/s]
63%|███████████████████████▎ | 1.04G/1.65G [00:17<00:09, 65.8MB/s]
63%|███████████████████████▍ | 1.05G/1.65G [00:17<00:09, 65.8MB/s]
64%|███████████████████████▋ | 1.06G/1.65G [00:17<00:09, 65.7MB/s]
64%|███████████████████████▊ | 1.06G/1.65G [00:17<00:08, 65.7MB/s]
65%|███████████████████████▉ | 1.07G/1.65G [00:17<00:08, 65.5MB/s]
65%|████████████████████████ | 1.08G/1.65G [00:17<00:08, 65.4MB/s]
65%|████████████████████████▏ | 1.08G/1.65G [00:18<00:08, 65.5MB/s]
66%|████████████████████████▍ | 1.09G/1.65G [00:18<00:08, 64.8MB/s]
66%|████████████████████████▌ | 1.10G/1.65G [00:18<00:08, 64.9MB/s]
67%|████████████████████████▋ | 1.10G/1.65G [00:18<00:08, 65.1MB/s]
67%|████████████████████████▊ | 1.11G/1.65G [00:18<00:08, 65.1MB/s]
67%|████████████████████████▉ | 1.11G/1.65G [00:18<00:08, 65.2MB/s]
68%|█████████████████████████ | 1.12G/1.65G [00:18<00:08, 65.3MB/s]
68%|█████████████████████████▎ | 1.13G/1.65G [00:18<00:08, 64.7MB/s]
69%|█████████████████████████▍ | 1.13G/1.65G [00:18<00:07, 64.8MB/s]
69%|█████████████████████████▌ | 1.14G/1.65G [00:18<00:07, 64.8MB/s]
69%|█████████████████████████▋ | 1.15G/1.65G [00:19<00:07, 65.0MB/s]
70%|█████████████████████████▊ | 1.15G/1.65G [00:19<00:07, 65.2MB/s]
70%|█████████████████████████▉ | 1.16G/1.65G [00:19<00:07, 65.2MB/s]
71%|██████████████████████████▏ | 1.17G/1.65G [00:19<00:07, 65.3MB/s]
71%|██████████████████████████▎ | 1.17G/1.65G [00:19<00:07, 61.5MB/s]
71%|██████████████████████████▍ | 1.18G/1.65G [00:19<00:07, 62.5MB/s]
72%|██████████████████████████▌ | 1.19G/1.65G [00:19<00:07, 63.2MB/s]
72%|██████████████████████████▋ | 1.19G/1.65G [00:19<00:07, 63.7MB/s]
73%|██████████████████████████▊ | 1.20G/1.65G [00:19<00:07, 64.1MB/s]
73%|███████████████████████████ | 1.21G/1.65G [00:19<00:06, 64.5MB/s]
73%|███████████████████████████▏ | 1.21G/1.65G [00:20<00:06, 64.8MB/s]
74%|███████████████████████████▎ | 1.22G/1.65G [00:20<00:06, 65.0MB/s]
74%|███████████████████████████▍ | 1.23G/1.65G [00:20<00:06, 65.1MB/s]
75%|███████████████████████████▌ | 1.23G/1.65G [00:20<00:06, 65.2MB/s]
75%|███████████████████████████▋ | 1.24G/1.65G [00:20<00:06, 65.3MB/s]
75%|███████████████████████████▉ | 1.25G/1.65G [00:20<00:06, 65.3MB/s]
76%|████████████████████████████ | 1.25G/1.65G [00:20<00:06, 65.3MB/s]
76%|████████████████████████████▏ | 1.26G/1.65G [00:20<00:06, 65.3MB/s]
77%|████████████████████████████▎ | 1.27G/1.65G [00:20<00:05, 65.4MB/s]
77%|████████████████████████████▍ | 1.27G/1.65G [00:20<00:05, 65.5MB/s]
77%|████████████████████████████▌ | 1.28G/1.65G [00:21<00:05, 65.5MB/s]
78%|████████████████████████████▊ | 1.28G/1.65G [00:21<00:05, 65.4MB/s]
78%|████████████████████████████▉ | 1.29G/1.65G [00:21<00:05, 65.2MB/s]
79%|█████████████████████████████ | 1.30G/1.65G [00:21<00:05, 65.2MB/s]
79%|█████████████████████████████▏ | 1.30G/1.65G [00:21<00:05, 65.2MB/s]
79%|█████████████████████████████▎ | 1.31G/1.65G [00:21<00:05, 65.4MB/s]
80%|█████████████████████████████▍ | 1.32G/1.65G [00:21<00:05, 65.4MB/s]
80%|█████████████████████████████▋ | 1.32G/1.65G [00:21<00:05, 65.3MB/s]
81%|█████████████████████████████▊ | 1.33G/1.65G [00:21<00:04, 65.3MB/s]
81%|█████████████████████████████▉ | 1.34G/1.65G [00:21<00:04, 65.3MB/s]
81%|██████████████████████████████ | 1.34G/1.65G [00:22<00:04, 64.7MB/s]
82%|██████████████████████████████▏ | 1.35G/1.65G [00:22<00:04, 64.8MB/s]
82%|██████████████████████████████▎ | 1.36G/1.65G [00:22<00:04, 63.1MB/s]
82%|██████████████████████████████▌ | 1.36G/1.65G [00:22<00:04, 61.4MB/s]
83%|██████████████████████████████▋ | 1.37G/1.65G [00:22<00:04, 62.3MB/s]
83%|██████████████████████████████▊ | 1.38G/1.65G [00:22<00:04, 63.2MB/s]
84%|██████████████████████████████▉ | 1.38G/1.65G [00:22<00:04, 63.9MB/s]
84%|███████████████████████████████ | 1.39G/1.65G [00:22<00:04, 64.1MB/s]
84%|███████████████████████████████▏ | 1.40G/1.65G [00:22<00:03, 64.4MB/s]
85%|███████████████████████████████▍ | 1.40G/1.65G [00:23<00:03, 64.6MB/s]
85%|███████████████████████████████▌ | 1.41G/1.65G [00:23<00:03, 64.7MB/s]
86%|███████████████████████████████▋ | 1.42G/1.65G [00:23<00:03, 64.5MB/s]
86%|███████████████████████████████▊ | 1.42G/1.65G [00:23<00:03, 64.3MB/s]
86%|███████████████████████████████▉ | 1.43G/1.65G [00:23<00:03, 64.5MB/s]
87%|████████████████████████████████ | 1.43G/1.65G [00:23<00:03, 64.6MB/s]
87%|████████████████████████████████▎ | 1.44G/1.65G [00:23<00:03, 64.9MB/s]
88%|████████████████████████████████▍ | 1.45G/1.65G [00:23<00:03, 59.5MB/s]
88%|████████████████████████████████▌ | 1.45G/1.65G [00:23<00:03, 61.0MB/s]
88%|████████████████████████████████▋ | 1.46G/1.65G [00:23<00:03, 62.1MB/s]
89%|████████████████████████████████▊ | 1.47G/1.65G [00:24<00:02, 63.0MB/s]
89%|████████████████████████████████▉ | 1.47G/1.65G [00:24<00:02, 63.7MB/s]
90%|█████████████████████████████████▏ | 1.48G/1.65G [00:24<00:02, 64.2MB/s]
90%|█████████████████████████████████▎ | 1.49G/1.65G [00:24<00:02, 64.4MB/s]
90%|█████████████████████████████████▍ | 1.49G/1.65G [00:24<00:02, 64.5MB/s]
91%|█████████████████████████████████▌ | 1.50G/1.65G [00:24<00:02, 64.5MB/s]
91%|█████████████████████████████████▋ | 1.51G/1.65G [00:24<00:02, 64.6MB/s]
92%|█████████████████████████████████▊ | 1.51G/1.65G [00:24<00:02, 64.6MB/s]
92%|██████████████████████████████████ | 1.52G/1.65G [00:24<00:02, 64.6MB/s]
92%|██████████████████████████████████▏ | 1.53G/1.65G [00:24<00:01, 64.6MB/s]
93%|██████████████████████████████████▎ | 1.53G/1.65G [00:25<00:01, 63.6MB/s]
93%|██████████████████████████████████▍ | 1.54G/1.65G [00:25<00:01, 62.8MB/s]
93%|██████████████████████████████████▌ | 1.54G/1.65G [00:25<00:01, 62.9MB/s]
94%|██████████████████████████████████▋ | 1.55G/1.65G [00:25<00:01, 63.1MB/s]
94%|██████████████████████████████████▊ | 1.56G/1.65G [00:25<00:01, 63.4MB/s]
95%|███████████████████████████████████ | 1.56G/1.65G [00:25<00:01, 63.4MB/s]
95%|███████████████████████████████████▏ | 1.57G/1.65G [00:25<00:01, 63.2MB/s]
95%|███████████████████████████████████▎ | 1.58G/1.65G [00:25<00:01, 63.2MB/s]
96%|███████████████████████████████████▍ | 1.58G/1.65G [00:25<00:01, 63.0MB/s]
96%|███████████████████████████████████▌ | 1.59G/1.65G [00:25<00:01, 63.5MB/s]
97%|███████████████████████████████████▋ | 1.60G/1.65G [00:26<00:00, 63.8MB/s]
97%|███████████████████████████████████▊ | 1.60G/1.65G [00:26<00:00, 63.9MB/s]
97%|████████████████████████████████████ | 1.61G/1.65G [00:26<00:00, 63.9MB/s]
98%|████████████████████████████████████▏| 1.61G/1.65G [00:26<00:00, 64.0MB/s]
98%|████████████████████████████████████▎| 1.62G/1.65G [00:26<00:00, 64.1MB/s]
98%|████████████████████████████████████▍| 1.63G/1.65G [00:26<00:00, 64.1MB/s]
99%|████████████████████████████████████▌| 1.63G/1.65G [00:26<00:00, 64.3MB/s]
99%|████████████████████████████████████▋| 1.64G/1.65G [00:26<00:00, 64.4MB/s]
100%|████████████████████████████████████▊| 1.65G/1.65G [00:26<00:00, 63.9MB/s]
0%| | 0.00/1.65G [00:00<?, ?B/s]
100%|█████████████████████████████████████| 1.65G/1.65G [00:00<00:00, 6.84TB/s]
Untarring contents of '/home/runner/mne_data/MNE-sample-data-processed.tar.gz' to '/home/runner/mne_data'
Attempting to create new mne-python configuration file:
/home/runner/.mne/mne-python.json
Download complete in 58s (1576.2 MB)
👋 Hello! This is openneuro-py 2024.2.0. Great to see you! 🤗
👉 Please report problems 🤯 and bugs 🪲 at
https://github.com/hoechenberger/openneuro-py/issues
🌍 Preparing to download ds002778 …
📁 Traversing directories for ds002778 : 0 entities [00:00, ? entities/s]
📁 Traversing directories for ds002778 : 7 entities [00:03, 1.87 entities/s]
📁 Traversing directories for ds002778 : 8 entities [00:03, 2.09 entities/s]
📁 Traversing directories for ds002778 : 14 entities [00:04, 4.73 entities/s]
📁 Traversing directories for ds002778 : 17 entities [00:04, 6.09 entities/s]
📁 Traversing directories for ds002778 : 20 entities [00:04, 4.61 entities/s]
📥 Retrieving up to 19 files (5 concurrent downloads).
README: 0.00B [00:00, ?B/s]
dataset_description.json: 0.00B [00:00, ?B/s]
CHANGES: 0.00B [00:00, ?B/s]
participants.tsv: 0%| | 0.00/1.62k [00:00<?, ?B/s]
participants.json: 0%| | 0.00/1.24k [00:00<?, ?B/s]
sub-pd6_ses-off_scans.tsv: 0%| | 0.00/75.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_beh.json: 0.00B [00:00, ?B/s]
sub-pd6_ses-off_task-rest_beh.tsv: 0%| | 0.00/10.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.json: 0%| | 0.00/471 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_channels.tsv: 0%| | 0.00/2.22k [00:00<?, ?B/s]
sub-pd6_ses-on_scans.tsv: 0%| | 0.00/74.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_events.tsv: 0%| | 0.00/66.0 [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_beh.tsv: 0%| | 0.00/10.0 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 0%| | 0.00/11.5M [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_eeg.json: 0%| | 0.00/471 [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_beh.json: 0%| | 0.00/433 [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 11%|█ | 1.23M/11.5M [00:00<00:00, 12.8MB/s]
sub-pd6_ses-on_task-rest_channels.tsv: 0%| | 0.00/2.22k [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 0%| | 0.00/17.4M [00:00<?, ?B/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 41%|████ | 4.65M/11.5M [00:00<00:00, 26.3MB/s]
sub-pd6_ses-on_task-rest_events.tsv: 0%| | 0.00/51.0 [00:00<?, ?B/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 3%|▎ | 527k/17.4M [00:00<00:03, 5.38MB/s]
sub-pd6_ses-off_task-rest_eeg.bdf: 78%|███████▊ | 8.93M/11.5M [00:00<00:00, 34.8MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 15%|█▍ | 2.54M/17.4M [00:00<00:01, 14.7MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 46%|████▋ | 8.04M/17.4M [00:00<00:00, 34.2MB/s]
sub-pd6_ses-on_task-rest_eeg.bdf: 81%|████████ | 14.0M/17.4M [00:00<00:00, 45.4MB/s]
✅ Finished downloading ds002778.
🧠 Please enjoy your brains.
Explore the dataset contents#
We can use MNE-BIDS to print a tree of all
included files and folders. We pass the max_depth
parameter to
mne_bids.print_dir_tree()
to the output to four levels of folders, for
better readability in this example.
print_dir_tree(bids_root, max_depth=4)
|ds002778/
|--- CHANGES
|--- README
|--- dataset_description.json
|--- participants.json
|--- participants.tsv
|--- sub-pd6/
|------ ses-off/
|--------- sub-pd6_ses-off_scans.tsv
|--------- beh/
|------------ sub-pd6_ses-off_task-rest_beh.json
|------------ sub-pd6_ses-off_task-rest_beh.tsv
|--------- eeg/
|------------ sub-pd6_ses-off_task-rest_channels.tsv
|------------ sub-pd6_ses-off_task-rest_eeg.bdf
|------------ sub-pd6_ses-off_task-rest_eeg.json
|------------ sub-pd6_ses-off_task-rest_events.tsv
|------ ses-on/
|--------- sub-pd6_ses-on_scans.tsv
|--------- beh/
|------------ sub-pd6_ses-on_task-rest_beh.json
|------------ sub-pd6_ses-on_task-rest_beh.tsv
|--------- eeg/
|------------ sub-pd6_ses-on_task-rest_channels.tsv
|------------ sub-pd6_ses-on_task-rest_eeg.bdf
|------------ sub-pd6_ses-on_task-rest_eeg.json
|------------ sub-pd6_ses-on_task-rest_events.tsv
We can even ask MNE-BIDS to produce a human-readbale summary report on the dataset contents.
print(make_report(bids_root))
Summarizing participants.tsv /home/runner/mne_data/ds002778/participants.tsv...
Summarizing scans.tsv files [PosixPath('/home/runner/mne_data/ds002778/sub-pd6/ses-on/sub-pd6_ses-on_scans.tsv'), PosixPath('/home/runner/mne_data/ds002778/sub-pd6/ses-off/sub-pd6_ses-off_scans.tsv')]...
The participant template found: comprised of 14 male and 17 female participants;
comprised of 31 right hand, 0 left hand and 0 ambidextrous;
ages ranged from 47.0 to 82.0 (mean = 63.39, std = 8.69)
The UC San Diego Resting State EEG Data from Patients with Parkinson's Disease
dataset was created by Alexander P. Rockhill, Nicko Jackson, Jobi George, Adam
Aron, and Nicole C. Swann and conforms to BIDS version 1.2.2. This report was
generated with MNE-BIDS (https://doi.org/10.21105/joss.01896). The dataset
consists of 1 participants (comprised of 14 male and 17 female participants;
comprised of 31 right hand, 0 left hand and 0 ambidextrous; ages ranged from
47.0 to 82.0 (mean = 63.39, std = 8.69)) and 2 recording sessions: off, and on.
Data was recorded using an EEG system (Biosemi) sampled at 512.0 Hz with line
noise at 50, and 60 Hz. There were 2 scans in total. Recording durations ranged
from 191.0 to 289.0 seconds (mean = 240.0, std = 49.0), for a total of 480.0
seconds of data recorded over all scans. For each dataset, there were on average
41.0 (std = 0.0) recording channels per scan, out of which 41.0 (std = 0.0) were
used in analysis (0.0 +/- 0.0 were removed from analysis).
Now it’s time to get ready for reading some of the data! First, we need to
create an mne_bids.BIDSPath
, which is the workhorse object of
MNE-BIDS when it comes to file and folder operations.
For now, we’re interested only in the EEG data in the BIDS root directory of the Parkinson’s disease patient dataset. There were two sessions, one where the patients took their regular anti-Parkinsonian medications and one where they abstained for more than twelve hours. For now, we are not interested in the on-medication session.
sessions = get_entity_vals(bids_root, "session", ignore_sessions="on")
datatype = "eeg"
extensions = [".bdf", ".tsv"] # ignore .json files
bids_paths = find_matching_paths(
bids_root, datatypes=datatype, sessions=sessions, extensions=extensions
)
We can now retrieve a list of all MEG-related files in the dataset:
print(bids_paths)
[BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_channels.tsv), BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg.bdf), BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_events.tsv)]
Note that this is the same as running:
[BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_channels.tsv), BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg.bdf), BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_events.tsv)]
The returned list contains BIDSpaths
of 3 files:
sub-pd6_ses-off_task-rest_channels.tsv
,
sub-pd6_ses-off_task-rest_events.tsv
, and
sub-pd6_ses-off_task-rest_eeg.bdf
.
The first two are so-called sidecar files that contain information on the
recording channels and experimental events, and the third one is the actual
data file.
Prepare reading the data#
There is only one subject and one experimental task (rest
).
Let’s use this knowledge to create a new BIDSPath
with
all the information required to actually read the EEG data. We also need to
pass a suffix
, which is the last part of the filename just before the
extension – 'channels'
and 'events'
for the two TSV files in
our example, and 'eeg'
for EEG raw data. For MEG and EEG raw data, the
suffix is identical to the datatype, so don’t let yourself be confused here!
Now let’s print the contents of bids_path
.
print(bids_path)
/home/runner/mne_data/ds002778/sub-pd6/ses-off/eeg/sub-pd6_ses-off_task-rest_eeg.bdf
You probably noticed two things: Firstly, this looks like an ordinary string now, not like the more-or-less neatly formatted output we saw before. And secondly, that there’s suddenly a filename extension which we never specified anywhere!
The reason is that when you call print(bids_path)
, BIDSPath
returns
a string representation of BIDSPath.fpath
, which looks different. If,
instead, you simply typed bids_path
(or print(repr(bids_path))
, which
is the same) into your Python console, you would get the nicely formatted
output:
BIDSPath(
root: /home/runner/mne_data/ds002778
datatype: eeg
basename: sub-pd6_ses-off_task-rest_eeg)
The root
here is – you guessed it – the directory we passed via the
root
parameter: the “home” of our BIDS dataset. The datatype
, again,
is self-explanatory. The basename
, on the other hand, is created
automatically based on the suffix and BIDS entities we passed to
BIDSPath
: in our case, subject
, session
and task
.
Note
There are many more supported entities, the most-commonly used among them
probably being acquisition
. Please see
our introduction to BIDSPath to learn more
about entities, basename
, and BIDSPath
in general.
But what about that filename extension, now? BIDSPath.fpath
, which –
as you hopefully remember – is invoked when you run print(bids_path)
–
employs some heuristics to auto-detect some missing filename components.
Omitting the filename extension in your script can make your code
more portable. Note that, however, you can explicitly specify an
extension too, by passing e.g. extension='.bdf'
to BIDSPath
.
Read the data#
Let’s read the data! It’s just a single line of code.
raw = read_raw_bids(bids_path=bids_path, verbose=False)
/home/runner/work/mne-bids/mne-bids/examples/read_bids_datasets.py:189: RuntimeWarning: Unable to map the following column(s) to to MNE:
gender: f
MMSE: 30
NAART: 42
disease_duration: 8
rl_deficits: L OFF meds, more R ON meds
notes: Used preprocessed data from EEGLAB .mat file instead of raw data for pd on
raw = read_raw_bids(bids_path=bids_path, verbose=False)
Now we can inspect the raw
object to check that it contains to correct
metadata.
Basic subject metadata is here.
print(raw.info["subject_info"])
<subject_info | his_id: sub-pd6, birthday: 1949-02-17, hand: 1>
Power line frequency is here.
print(raw.info["line_freq"])
60.0
Sampling frequency is here.
print(raw.info["sfreq"])
512.0
Events are now Annotations
print(raw.annotations)
<Annotations | 2 segments: 1 (1), 65536 (1)>
Plot the raw data.
raw.plot()
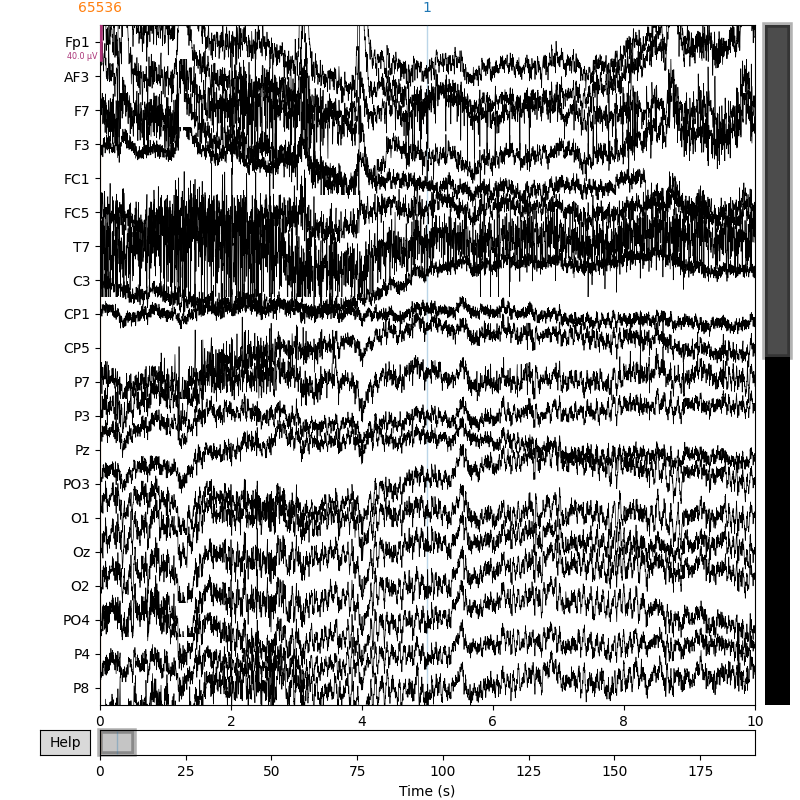
Using matplotlib as 2D backend.
<MNEBrowseFigure size 800x800 with 4 Axes>
Total running time of the script: (1 minutes 6.658 seconds)