Note
Go to the end to download the full example code.
Save and load T1-weighted MRI scan along with anatomical landmarks in BIDS#
When working with MEEG data in the domain of source localization, we usually have to deal with aligning several coordinate systems, such as the coordinate systems of …
the head of a study participant
the recording device (in the case of MEG)
the anatomical MRI scan of a study participant
The process of aligning these frames is also called coregistration, and is
performed with the help of a transformation matrix, called trans
in MNE.
In this tutorial, we show how MNE-BIDS
can be used to save a T1 weighted
MRI scan in BIDS format, and to encode all information of the trans
object
in a BIDS compatible way.
Finally, we will automatically reproduce our trans
object from a BIDS
directory.
See the documentation pages in the MNE docs for more information on source alignment and coordinate frames
Note
For this example you will need to install matplotlib
and
nilearn
on top of your usual mne-bids
installation.
# Authors: The MNE-BIDS developers
# SPDX-License-Identifier: BSD-3-Clause
Let’s import everything we need for this example:
import os.path as op
import shutil
import matplotlib.pyplot as plt
import mne
import numpy as np
from mne import head_to_mri
from mne.datasets import sample
from nilearn.plotting import plot_anat
from mne_bids import (
BIDSPath,
get_anat_landmarks,
get_head_mri_trans,
print_dir_tree,
write_anat,
write_raw_bids,
)
We will be using the MNE sample data and write a basic BIDS dataset. For more information, you can checkout the respective example.
data_path = sample.data_path()
event_id = {
"Auditory/Left": 1,
"Auditory/Right": 2,
"Visual/Left": 3,
"Visual/Right": 4,
"Smiley": 5,
"Button": 32,
}
raw_fname = op.join(data_path, "MEG", "sample", "sample_audvis_raw.fif")
events_fname = op.join(data_path, "MEG", "sample", "sample_audvis_raw-eve.fif")
output_path = op.abspath(op.join(data_path, "..", "MNE-sample-data-bids"))
fs_subjects_dir = op.join(data_path, "subjects") # FreeSurfer subjects dir
To ensure the output path doesn’t contain any leftover files from previous tests and example runs, we simply delete it.
Warning
Do not delete directories that may contain important data!
Read the input data and store it as BIDS data.
raw = mne.io.read_raw_fif(raw_fname)
raw.info["line_freq"] = 60 # specify power line frequency as required by BIDS
sub = "01"
ses = "01"
task = "audiovisual"
run = "01"
bids_path = BIDSPath(subject=sub, session=ses, task=task, run=run, root=output_path)
write_raw_bids(raw, bids_path, events=events_fname, event_id=event_id, overwrite=True)
Opening raw data file /home/runner/mne_data/MNE-sample-data/MEG/sample/sample_audvis_raw.fif...
Read a total of 3 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Range : 25800 ... 192599 = 42.956 ... 320.670 secs
Ready.
Opening raw data file /home/runner/mne_data/MNE-sample-data/MEG/sample/sample_audvis_raw.fif...
Read a total of 3 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Range : 25800 ... 192599 = 42.956 ... 320.670 secs
Ready.
Writing '/home/runner/mne_data/MNE-sample-data-bids/README'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/participants.tsv'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/participants.json'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_coordsystem.json'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_coordsystem.json'...
Used Annotations descriptions: [np.str_('Auditory/Left'), np.str_('Auditory/Right'), np.str_('Button'), np.str_('Smiley'), np.str_('Visual/Left'), np.str_('Visual/Right')]
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_events.tsv'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_events.json'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/dataset_description.json'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_meg.json'...
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_channels.tsv'...
Copying data files to sub-01_ses-01_task-audiovisual_run-01_meg.fif
Reserving possible split file sub-01_ses-01_task-audiovisual_run-01_split-01_meg.fif
Writing /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_meg.fif
Closing /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_meg.fif
[done]
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/sub-01_ses-01_scans.tsv'...
Wrote /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/sub-01_ses-01_scans.tsv entry with meg/sub-01_ses-01_task-audiovisual_run-01_meg.fif.
BIDSPath(
root: /home/runner/mne_data/MNE-sample-data-bids
datatype: meg
basename: sub-01_ses-01_task-audiovisual_run-01_meg.fif)
Print the directory tree
|MNE-sample-data-bids/
|--- README
|--- dataset_description.json
|--- participants.json
|--- participants.tsv
|--- sub-01/
|------ ses-01/
|--------- sub-01_ses-01_scans.tsv
|--------- meg/
|------------ sub-01_ses-01_coordsystem.json
|------------ sub-01_ses-01_task-audiovisual_run-01_channels.tsv
|------------ sub-01_ses-01_task-audiovisual_run-01_events.json
|------------ sub-01_ses-01_task-audiovisual_run-01_events.tsv
|------------ sub-01_ses-01_task-audiovisual_run-01_meg.fif
|------------ sub-01_ses-01_task-audiovisual_run-01_meg.json
Writing T1 image#
Now let’s assume that we have also collected some T1 weighted MRI data for
our subject. And furthermore, that we have already aligned our coordinate
frames (using e.g., the coregistration GUI) and obtained a transformation
matrix trans
.
# Get the path to our MRI scan
t1_fname = op.join(fs_subjects_dir, "sample", "mri", "T1.mgz")
# Load the transformation matrix and show what it looks like
trans_fname = op.join(data_path, "MEG", "sample", "sample_audvis_raw-trans.fif")
trans = mne.read_trans(trans_fname)
print(trans)
<Transform | head->MRI (surface RAS)>
[[ 0.99930954 0.01275934 0.0348942 0.00206991]
[ 0.00998479 0.81240475 -0.58300853 0.01130214]
[-0.03578702 0.58295429 0.81171638 -0.02755522]
[ 0. 0. 0. 1. ]]
We can save the MRI to our existing BIDS directory and at the same time
create a JSON sidecar file that contains metadata, we will later use to
retrieve our transformation matrix trans
. The metadata will here
consist of the coordinates of three anatomical landmarks (LPA, Nasion and
RPA (=left and right preauricular points) expressed in voxel coordinates
w.r.t. the T1 image.
# First create the BIDSPath object.
t1w_bids_path = BIDSPath(subject=sub, session=ses, root=output_path, suffix="T1w")
# use ``trans`` to transform landmarks from the ``raw`` file to
# the voxel space of the image
landmarks = get_anat_landmarks(
t1_fname, # path to the MRI scan
info=raw.info, # the MEG data file info from the same subject as the MRI
trans=trans, # our transformation matrix
fs_subject="sample", # FreeSurfer subject
fs_subjects_dir=fs_subjects_dir, # FreeSurfer subjects directory
)
# We use the write_anat function
t1w_bids_path = write_anat(
image=t1_fname, # path to the MRI scan
bids_path=t1w_bids_path,
landmarks=landmarks, # the landmarks in MRI voxel space
verbose=True, # this will print out the sidecar file
)
anat_dir = t1w_bids_path.directory
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/anat/sub-01_ses-01_T1w.json'...
Let’s have another look at our BIDS directory
|MNE-sample-data-bids/
|--- README
|--- dataset_description.json
|--- participants.json
|--- participants.tsv
|--- sub-01/
|------ ses-01/
|--------- sub-01_ses-01_scans.tsv
|--------- anat/
|------------ sub-01_ses-01_T1w.json
|------------ sub-01_ses-01_T1w.nii.gz
|--------- meg/
|------------ sub-01_ses-01_coordsystem.json
|------------ sub-01_ses-01_task-audiovisual_run-01_channels.tsv
|------------ sub-01_ses-01_task-audiovisual_run-01_events.json
|------------ sub-01_ses-01_task-audiovisual_run-01_events.tsv
|------------ sub-01_ses-01_task-audiovisual_run-01_meg.fif
|------------ sub-01_ses-01_task-audiovisual_run-01_meg.json
Our BIDS dataset is now ready to be shared. We can easily estimate the
transformation matrix using MNE-BIDS
and the BIDS dataset.
This function converts the anatomical landmarks stored in the T1 sidecar
file into FreeSurfer surface RAS space, and aligns the landmarks in the
electrophysiology data with them. This way your electrophysiology channel
locations can be transformed to surface RAS space using the trans
which
is crucial for source localization and other uses of the FreeSurfer surfaces.
Note
If this dataset were shared with you, you would first have to use the T1 image as input for the FreeSurfer recon-all, see How MNE uses FreeSurfer’s outputs.
estim_trans = get_head_mri_trans(
bids_path=bids_path, fs_subject="sample", fs_subjects_dir=fs_subjects_dir
)
Opening raw data file /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_meg.fif...
Read a total of 3 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Range : 25800 ... 192599 = 42.956 ... 320.670 secs
Ready.
Reading events from /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_events.tsv.
Reading channel info from /home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/meg/sub-01_ses-01_task-audiovisual_run-01_channels.tsv.
Finally, let’s use the T1 weighted MRI image and plot the anatomical
landmarks Nasion, LPA, and RPA onto the brain image. For that, we can
extract the location of Nasion, LPA, and RPA from the MEG file, apply our
transformation matrix trans
, and plot the results.
# Get Landmarks from MEG file, 0, 1, and 2 correspond to LPA, NAS, RPA
# and the 'r' key will provide us with the xyz coordinates. The coordinates
# are expressed here in MEG Head coordinate system.
pos = np.asarray(
(raw.info["dig"][0]["r"], raw.info["dig"][1]["r"], raw.info["dig"][2]["r"])
)
# We now use the ``head_to_mri`` function from MNE-Python to convert MEG
# coordinates to MRI scanner RAS space. For the conversion we use our
# estimated transformation matrix and the MEG coordinates extracted from the
# raw file. `subjects` and `subjects_dir` are used internally, to point to
# the T1-weighted MRI file: `t1_mgh_fname`. Coordinates are is mm.
mri_pos = head_to_mri(
pos=pos, subject="sample", mri_head_t=estim_trans, subjects_dir=fs_subjects_dir
)
# Our MRI written to BIDS, we got `anat_dir` from our `write_anat` function
t1_nii_fname = op.join(anat_dir, "sub-01_ses-01_T1w.nii.gz")
# Plot it
fig, axs = plt.subplots(3, 1, figsize=(7, 7), facecolor="k")
for point_idx, label in enumerate(("LPA", "NAS", "RPA")):
plot_anat(
t1_nii_fname,
axes=axs[point_idx],
cut_coords=mri_pos[point_idx, :],
title=label,
vmax=160,
)
plt.show()
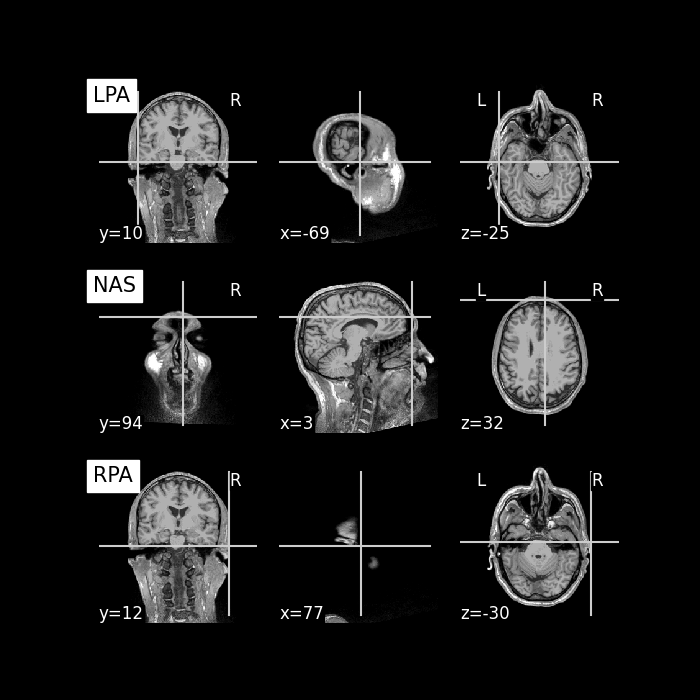
Writing FLASH MRI image#
We can write another types of MRI data such as FLASH images for BEM models
flash_fname = op.join(fs_subjects_dir, "sample", "mri", "flash", "mef05.mgz")
flash_bids_path = BIDSPath(subject=sub, session=ses, root=output_path, suffix="FLASH")
write_anat(image=flash_fname, bids_path=flash_bids_path, verbose=True)
BIDSPath(
root: /home/runner/mne_data/MNE-sample-data-bids
datatype: anat
basename: sub-01_ses-01_FLASH.nii.gz)
Writing defaced and anonymized T1 image#
We can deface the MRI for anonymization by passing deface=True
.
t1w_bids_path = write_anat(
image=t1_fname, # path to the MRI scan
bids_path=bids_path,
landmarks=landmarks,
deface=True,
overwrite=True,
verbose=True, # this will print out the sidecar file
)
anat_dir = t1w_bids_path.directory
# Our MRI written to BIDS, we got `anat_dir` from our `write_anat` function
t1_nii_fname = op.join(anat_dir, "sub-01_ses-01_T1w.nii.gz")
# Plot it
fig, ax = plt.subplots()
plot_anat(t1_nii_fname, axes=ax, title="Defaced", vmax=160)
plt.show()
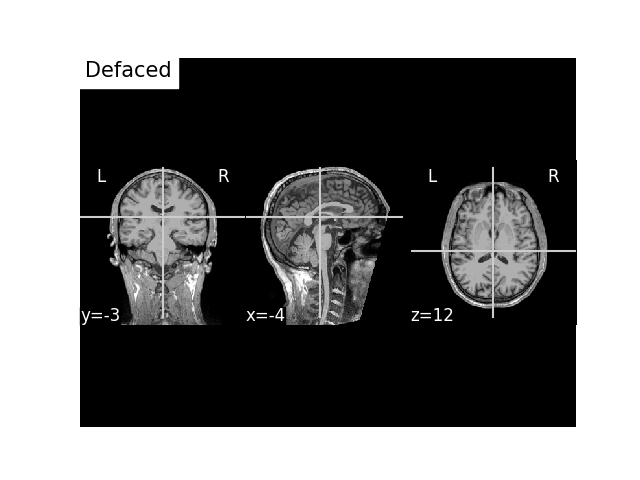
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/anat/sub-01_ses-01_T1w.json'...
Writing defaced and anonymized FLASH MRI image#
Defacing the FLASH works just like the T1 as long as they are aligned.
# use ``trans`` to transform landmarks from the ``raw`` file to
# the voxel space of the image
landmarks = get_anat_landmarks(
flash_fname, # path to the FLASH scan
info=raw.info, # the MEG data file info from the same subject as the MRI
trans=trans, # our transformation matrix
fs_subject="sample", # freesurfer subject
fs_subjects_dir=fs_subjects_dir, # freesurfer subjects directory
)
flash_bids_path = write_anat(
image=flash_fname, # path to the MRI scan
bids_path=flash_bids_path,
landmarks=landmarks,
deface=True,
overwrite=True,
verbose=True, # this will print out the sidecar file
)
# Our MRI written to BIDS, we got `anat_dir` from our `write_anat` function
flash_nii_fname = op.join(anat_dir, "sub-01_ses-01_FLASH.nii.gz")
# Plot it
fig, ax = plt.subplots()
plot_anat(flash_nii_fname, axes=ax, title="Defaced", vmax=700)
plt.show()
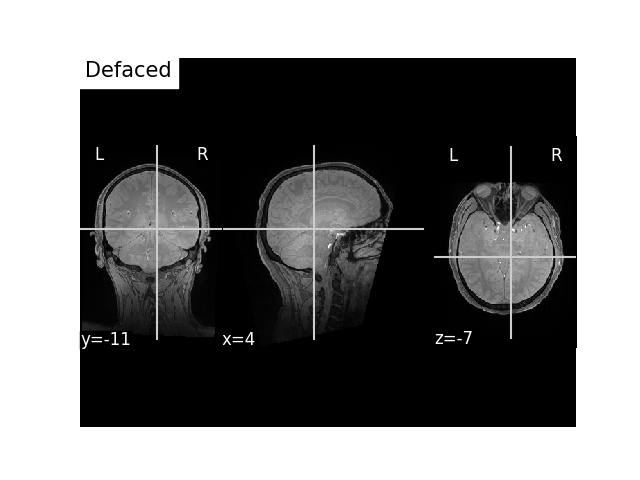
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/anat/sub-01_ses-01_FLASH.json'...
Using manual landmark coordinates in scanner RAS#
You can also find landmarks with a 3D image viewer (e.g. FreeView) if you have not aligned the channel locations (including fiducials) using the coregistration GUI or if this is just more convenient.
Note
In FreeView, you need to use “RAS” and not “TkReg RAS” for this. You can also use voxel coordinates but, in FreeView, they are integers and so not as precise as the “RAS” decimal numbers.
flash_ras_landmarks = (
np.array(
[
[-74.53102838, 19.62854953, -52.2888194],
[-1.89454315, 103.69850925, 4.97120376],
[72.01200673, 21.09274883, -57.53678375],
]
)
/ 1e3
) # mm -> m
landmarks = mne.channels.make_dig_montage(
lpa=flash_ras_landmarks[0],
nasion=flash_ras_landmarks[1],
rpa=flash_ras_landmarks[2],
coord_frame="ras",
)
flash_bids_path = write_anat(
image=flash_fname, # path to the MRI scan
bids_path=flash_bids_path,
landmarks=landmarks,
deface=True,
overwrite=True,
verbose=True, # this will print out the sidecar file
)
# Plot it
fig, ax = plt.subplots()
plot_anat(flash_nii_fname, axes=ax, title="Defaced", vmax=700)
plt.show()
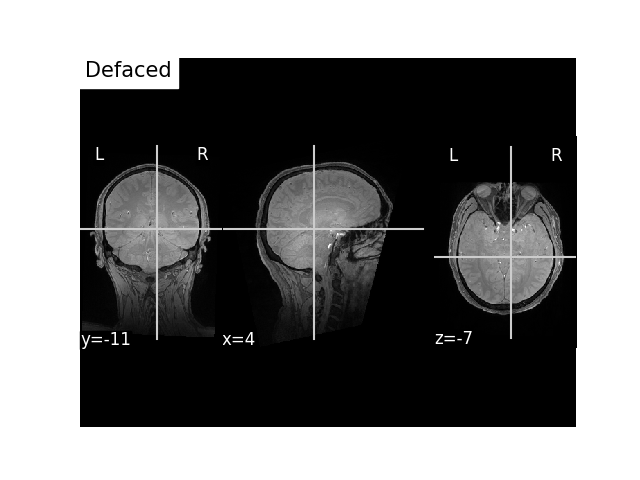
Writing '/home/runner/mne_data/MNE-sample-data-bids/sub-01/ses-01/anat/sub-01_ses-01_FLASH.json'...
Total running time of the script: (0 minutes 23.979 seconds)