mne.SourceMorph#
- class mne.SourceMorph(subject_from, subject_to, kind, zooms, niter_affine, niter_sdr, spacing, smooth, xhemi, morph_mat, vertices_to, shape, affine, pre_affine, sdr_morph, src_data, vol_morph_mat, *, verbose=None)[source]#
Morph source space data from one subject to another.
Note
This class should not be instantiated directly via
mne.SourceMorph(...)
. Instead, use one of the functions listed in the See Also section below.- Parameters:
- subject_from
str
|None
Name of the subject from which to morph as named in the SUBJECTS_DIR.
- subject_to
str
|array
|list
ofarray
Name of the subject on which to morph as named in the SUBJECTS_DIR. The default is ‘fsaverage’. If morphing a volume source space, subject_to can be the path to a MRI volume. Can also be a list of two arrays if morphing to hemisphere surfaces.
- kind
str
|None
Kind of source estimate. E.g. ‘volume’ or ‘surface’.
- zooms
float
|tuple
- niter_affine
tuple
ofint
Number of levels (
len(niter_affine)
) and number of iterations per level - for each successive stage of iterative refinement - to perform the affine transform.- niter_sdr
tuple
ofint
Number of levels (
len(niter_sdr)
) and number of iterations per level - for each successive stage of iterative refinement - to perform the Symmetric Diffeomorphic Registration (sdr) transform [1].- spacing
int
|list
|None
- smooth
int
|str
|None
- xhemi
bool
Morph across hemisphere.
- morph_mat
scipy.sparse.csr_matrix
The sparse surface morphing matrix for spherical surface based morphing [2].
- vertices_to
list
ofndarray
The destination surface vertices.
- shape
tuple
The volume MRI shape.
- affine
ndarray
The volume MRI affine.
- pre_affineinstance of
dipy.align.AffineMap
The transformation that is applied before the before
sdr_morph
.- sdr_morphinstance of
dipy.align.DiffeomorphicMap
The class that applies the the symmetric diffeomorphic registration (SDR) morph.
- src_data
dict
Additional source data necessary to perform morphing.
- vol_morph_mat
scipy.sparse.csr_matrix
|None
The volumetric morph matrix, if
compute_vol_morph_mat()
was used.- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- subject_from
See also
Notes
New in version 0.17.
References
Methods
apply
(stc_from[, output, mri_resolution, ...])Morph source space data.
compute_vol_morph_mat
(*[, verbose])Compute the sparse matrix representation of the volumetric morph.
save
(fname[, overwrite, verbose])Save the morph for source estimates to a file.
- apply(stc_from, output='stc', mri_resolution=False, mri_space=None, verbose=None)[source]#
Morph source space data.
- Parameters:
- stc_from
VolSourceEstimate
|VolVectorSourceEstimate
|SourceEstimate
|VectorSourceEstimate
The source estimate to morph.
- output
str
Can be ‘stc’ (default) or possibly ‘nifti1’, or ‘nifti2’ when working with a volume source space defined on a regular grid.
- mri_resolution
bool
|tuple
|int
|float
If True the image is saved in MRI resolution. Default False. WARNING: if you have many time points the file produced can be huge. The default is mri_resolution=False.
- mri_space
bool
|None
Whether the image to world registration should be in mri space. The default (None) is mri_space=mri_resolution.
- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- stc_from
- Returns:
- stc_to
VolSourceEstimate
|SourceEstimate
|VectorSourceEstimate
|Nifti1Image
|Nifti2Image
The morphed source estimates.
- stc_to
Examples using
apply
:2 samples permutation test on source data with spatio-temporal clustering
2 samples permutation test on source data with spatio-temporal clusteringCompute sparse inverse solution with mixed norm: MxNE and irMxNE
Compute sparse inverse solution with mixed norm: MxNE and irMxNE
- compute_vol_morph_mat(*, verbose=None)[source]#
Compute the sparse matrix representation of the volumetric morph.
- Parameters:
- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- verbose
- Returns:
- morphinstance of
SourceMorph
The instance (modified in-place).
- morphinstance of
Notes
For a volumetric morph, this will compute the morph for an identity source volume, i.e., with one source vertex active at a time, and store the result as a
sparse
morphing matrix. This takes a long time (minutes) to compute initially, but drastically speeds upapply()
for STCs, so it can be beneficial when many time points or many morphs (i.e., greater than the number of volumetricsrc_from
vertices) will be performed.When calling
save()
, this sparse morphing matrix is saved with the instance, so this only needs to be called once. This function does nothing if the morph matrix has already been computed, or if there is no volume morphing necessary.New in version 0.22.
Examples using
compute_vol_morph_mat
:
- save(fname, overwrite=False, verbose=None)[source]#
Save the morph for source estimates to a file.
- Parameters:
- fname
str
The stem of the file name. ‘-morph.h5’ will be added if fname does not end with ‘.h5’.
- overwrite
bool
If True (default False), overwrite the destination file if it exists.
- verbose
bool
|str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- fname
Examples using mne.SourceMorph
#
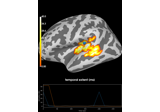
2 samples permutation test on source data with spatio-temporal clustering
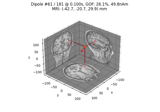
Compute sparse inverse solution with mixed norm: MxNE and irMxNE