Note
Click here to download the full example code
Decoding sensor space data with generalization across time and conditions¶
This example runs the analysis described in 1. It illustrates how one can fit a linear classifier to identify a discriminatory topography at a given time instant and subsequently assess whether this linear model can accurately predict all of the time samples of a second set of conditions.
# Authors: Jean-Remi King <jeanremi.king@gmail.com>
# Alexandre Gramfort <alexandre.gramfort@inria.fr>
# Denis Engemann <denis.engemann@gmail.com>
#
# License: BSD (3-clause)
import matplotlib.pyplot as plt
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
import mne
from mne.datasets import sample
from mne.decoding import GeneralizingEstimator
print(__doc__)
# Preprocess data
data_path = sample.data_path()
# Load and filter data, set up epochs
raw_fname = data_path + '/MEG/sample/sample_audvis_filt-0-40_raw.fif'
events_fname = data_path + '/MEG/sample/sample_audvis_filt-0-40_raw-eve.fif'
raw = mne.io.read_raw_fif(raw_fname, preload=True)
picks = mne.pick_types(raw.info, meg=True, exclude='bads') # Pick MEG channels
raw.filter(1., 30., fir_design='firwin') # Band pass filtering signals
events = mne.read_events(events_fname)
event_id = {'Auditory/Left': 1, 'Auditory/Right': 2,
'Visual/Left': 3, 'Visual/Right': 4}
tmin = -0.050
tmax = 0.400
# decimate to make the example faster to run, but then use verbose='error' in
# the Epochs constructor to suppress warning about decimation causing aliasing
decim = 2
epochs = mne.Epochs(raw, events, event_id=event_id, tmin=tmin, tmax=tmax,
proj=True, picks=picks, baseline=None, preload=True,
reject=dict(mag=5e-12), decim=decim, verbose='error')
Out:
Opening raw data file /home/circleci/mne_data/MNE-sample-data/MEG/sample/sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Reading 0 ... 41699 = 0.000 ... 277.709 secs...
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 0.50 Hz)
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 497 samples (3.310 sec)
We will train the classifier on all left visual vs auditory trials and test on all right visual vs auditory trials.
clf = make_pipeline(StandardScaler(), LogisticRegression(solver='lbfgs'))
time_gen = GeneralizingEstimator(clf, scoring='roc_auc', n_jobs=1,
verbose=True)
# Fit classifiers on the epochs where the stimulus was presented to the left.
# Note that the experimental condition y indicates auditory or visual
time_gen.fit(X=epochs['Left'].get_data(),
y=epochs['Left'].events[:, 2] > 2)
Out:
0%| | Fitting GeneralizingEstimator : 0/35 [00:00<?, ?it/s]
3%|2 | Fitting GeneralizingEstimator : 1/35 [00:00<00:01, 29.21it/s]
6%|5 | Fitting GeneralizingEstimator : 2/35 [00:00<00:01, 28.70it/s]
9%|8 | Fitting GeneralizingEstimator : 3/35 [00:00<00:01, 20.18it/s]
14%|#4 | Fitting GeneralizingEstimator : 5/35 [00:00<00:01, 27.98it/s]
20%|## | Fitting GeneralizingEstimator : 7/35 [00:00<00:01, 24.97it/s]
23%|##2 | Fitting GeneralizingEstimator : 8/35 [00:00<00:01, 22.85it/s]
26%|##5 | Fitting GeneralizingEstimator : 9/35 [00:00<00:01, 23.51it/s]
29%|##8 | Fitting GeneralizingEstimator : 10/35 [00:00<00:01, 22.07it/s]
34%|###4 | Fitting GeneralizingEstimator : 12/35 [00:00<00:00, 25.18it/s]
37%|###7 | Fitting GeneralizingEstimator : 13/35 [00:00<00:00, 25.54it/s]
40%|#### | Fitting GeneralizingEstimator : 14/35 [00:00<00:00, 23.90it/s]
43%|####2 | Fitting GeneralizingEstimator : 15/35 [00:00<00:00, 24.30it/s]
49%|####8 | Fitting GeneralizingEstimator : 17/35 [00:00<00:00, 24.78it/s]
51%|#####1 | Fitting GeneralizingEstimator : 18/35 [00:00<00:00, 22.18it/s]
54%|#####4 | Fitting GeneralizingEstimator : 19/35 [00:00<00:00, 21.85it/s]
60%|###### | Fitting GeneralizingEstimator : 21/35 [00:00<00:00, 23.86it/s]
63%|######2 | Fitting GeneralizingEstimator : 22/35 [00:00<00:00, 23.01it/s]
66%|######5 | Fitting GeneralizingEstimator : 23/35 [00:00<00:00, 23.30it/s]
69%|######8 | Fitting GeneralizingEstimator : 24/35 [00:01<00:00, 22.56it/s]
74%|#######4 | Fitting GeneralizingEstimator : 26/35 [00:01<00:00, 24.35it/s]
77%|#######7 | Fitting GeneralizingEstimator : 27/35 [00:01<00:00, 22.38it/s]
80%|######## | Fitting GeneralizingEstimator : 28/35 [00:01<00:00, 21.84it/s]
83%|########2 | Fitting GeneralizingEstimator : 29/35 [00:01<00:00, 22.00it/s]
86%|########5 | Fitting GeneralizingEstimator : 30/35 [00:01<00:00, 21.60it/s]
89%|########8 | Fitting GeneralizingEstimator : 31/35 [00:01<00:00, 21.85it/s]
91%|#########1| Fitting GeneralizingEstimator : 32/35 [00:01<00:00, 21.40it/s]
97%|#########7| Fitting GeneralizingEstimator : 34/35 [00:01<00:00, 22.65it/s]
100%|##########| Fitting GeneralizingEstimator : 35/35 [00:01<00:00, 21.88it/s]
100%|##########| Fitting GeneralizingEstimator : 35/35 [00:01<00:00, 22.36it/s]
Score on the epochs where the stimulus was presented to the right.
scores = time_gen.score(X=epochs['Right'].get_data(),
y=epochs['Right'].events[:, 2] > 2)
Out:
0%| | Scoring GeneralizingEstimator : 0/1225 [00:00<?, ?it/s]
1%|1 | Scoring GeneralizingEstimator : 15/1225 [00:00<00:02, 437.11it/s]
3%|3 | Scoring GeneralizingEstimator : 37/1225 [00:00<00:02, 544.09it/s]
3%|3 | Scoring GeneralizingEstimator : 38/1225 [00:00<00:03, 321.01it/s]
5%|4 | Scoring GeneralizingEstimator : 56/1225 [00:00<00:03, 372.16it/s]
5%|5 | Scoring GeneralizingEstimator : 67/1225 [00:00<00:03, 303.34it/s]
7%|7 | Scoring GeneralizingEstimator : 86/1225 [00:00<00:03, 342.28it/s]
8%|7 | Scoring GeneralizingEstimator : 96/1225 [00:00<00:03, 297.37it/s]
9%|9 | Scoring GeneralizingEstimator : 114/1225 [00:00<00:03, 323.85it/s]
10%|# | Scoring GeneralizingEstimator : 124/1225 [00:00<00:03, 291.20it/s]
12%|#1 | Scoring GeneralizingEstimator : 143/1225 [00:00<00:03, 316.17it/s]
13%|#2 | Scoring GeneralizingEstimator : 154/1225 [00:00<00:03, 292.24it/s]
14%|#4 | Scoring GeneralizingEstimator : 174/1225 [00:00<00:03, 315.89it/s]
15%|#5 | Scoring GeneralizingEstimator : 185/1225 [00:00<00:03, 294.97it/s]
17%|#6 | Scoring GeneralizingEstimator : 205/1225 [00:00<00:03, 315.64it/s]
18%|#7 | Scoring GeneralizingEstimator : 215/1225 [00:00<00:03, 294.94it/s]
19%|#8 | Scoring GeneralizingEstimator : 229/1225 [00:00<00:03, 302.37it/s]
19%|#9 | Scoring GeneralizingEstimator : 236/1225 [00:00<00:03, 279.73it/s]
21%|## | Scoring GeneralizingEstimator : 256/1225 [00:00<00:03, 297.89it/s]
22%|##1 | Scoring GeneralizingEstimator : 267/1225 [00:00<00:03, 283.82it/s]
23%|##3 | Scoring GeneralizingEstimator : 287/1225 [00:00<00:03, 300.48it/s]
24%|##4 | Scoring GeneralizingEstimator : 297/1225 [00:01<00:03, 285.53it/s]
26%|##5 | Scoring GeneralizingEstimator : 315/1225 [00:01<00:03, 298.20it/s]
27%|##6 | Scoring GeneralizingEstimator : 325/1225 [00:01<00:03, 298.00it/s]
28%|##7 | Scoring GeneralizingEstimator : 340/1225 [00:01<00:03, 290.43it/s]
28%|##8 | Scoring GeneralizingEstimator : 346/1225 [00:01<00:03, 272.88it/s]
30%|##9 | Scoring GeneralizingEstimator : 365/1225 [00:01<00:03, 286.44it/s]
31%|### | Scoring GeneralizingEstimator : 375/1225 [00:01<00:03, 274.52it/s]
32%|###1 | Scoring GeneralizingEstimator : 389/1225 [00:01<00:02, 280.79it/s]
32%|###2 | Scoring GeneralizingEstimator : 397/1225 [00:01<00:03, 267.16it/s]
34%|###3 | Scoring GeneralizingEstimator : 411/1225 [00:01<00:02, 273.56it/s]
34%|###4 | Scoring GeneralizingEstimator : 417/1225 [00:01<00:03, 258.42it/s]
35%|###5 | Scoring GeneralizingEstimator : 429/1225 [00:01<00:03, 262.46it/s]
36%|###5 | Scoring GeneralizingEstimator : 436/1225 [00:01<00:03, 249.84it/s]
37%|###6 | Scoring GeneralizingEstimator : 448/1225 [00:01<00:03, 253.93it/s]
37%|###7 | Scoring GeneralizingEstimator : 454/1225 [00:01<00:03, 241.30it/s]
38%|###8 | Scoring GeneralizingEstimator : 470/1225 [00:01<00:03, 250.65it/s]
39%|###9 | Scoring GeneralizingEstimator : 478/1225 [00:01<00:03, 240.68it/s]
41%|#### | Scoring GeneralizingEstimator : 498/1225 [00:01<00:02, 254.67it/s]
42%|####1 | Scoring GeneralizingEstimator : 509/1225 [00:01<00:02, 248.00it/s]
43%|####3 | Scoring GeneralizingEstimator : 527/1225 [00:01<00:02, 259.14it/s]
44%|####3 | Scoring GeneralizingEstimator : 538/1225 [00:02<00:02, 252.21it/s]
45%|####5 | Scoring GeneralizingEstimator : 557/1225 [00:02<00:02, 264.15it/s]
46%|####6 | Scoring GeneralizingEstimator : 569/1225 [00:02<00:02, 258.14it/s]
48%|####8 | Scoring GeneralizingEstimator : 589/1225 [00:02<00:02, 270.87it/s]
49%|####8 | Scoring GeneralizingEstimator : 600/1225 [00:02<00:02, 263.21it/s]
51%|##### | Scoring GeneralizingEstimator : 619/1225 [00:02<00:02, 274.45it/s]
51%|#####1 | Scoring GeneralizingEstimator : 630/1225 [00:02<00:02, 266.69it/s]
53%|#####3 | Scoring GeneralizingEstimator : 650/1225 [00:02<00:02, 278.77it/s]
54%|#####3 | Scoring GeneralizingEstimator : 661/1225 [00:02<00:02, 270.81it/s]
56%|#####5 | Scoring GeneralizingEstimator : 680/1225 [00:02<00:01, 281.57it/s]
56%|#####6 | Scoring GeneralizingEstimator : 691/1225 [00:02<00:01, 273.41it/s]
58%|#####7 | Scoring GeneralizingEstimator : 710/1225 [00:02<00:01, 283.95it/s]
59%|#####8 | Scoring GeneralizingEstimator : 720/1225 [00:02<00:01, 274.00it/s]
60%|###### | Scoring GeneralizingEstimator : 741/1225 [00:02<00:01, 286.59it/s]
61%|######1 | Scoring GeneralizingEstimator : 750/1225 [00:02<00:01, 276.79it/s]
63%|######2 | Scoring GeneralizingEstimator : 769/1225 [00:02<00:01, 286.88it/s]
64%|######3 | Scoring GeneralizingEstimator : 779/1225 [00:02<00:01, 277.70it/s]
65%|######5 | Scoring GeneralizingEstimator : 799/1225 [00:02<00:01, 288.94it/s]
66%|######6 | Scoring GeneralizingEstimator : 809/1225 [00:02<00:01, 279.51it/s]
68%|######7 | Scoring GeneralizingEstimator : 830/1225 [00:02<00:01, 291.72it/s]
69%|######8 | Scoring GeneralizingEstimator : 842/1225 [00:03<00:01, 284.13it/s]
70%|####### | Scoring GeneralizingEstimator : 862/1225 [00:03<00:01, 295.04it/s]
71%|#######1 | Scoring GeneralizingEstimator : 872/1225 [00:03<00:01, 295.06it/s]
73%|#######2 | Scoring GeneralizingEstimator : 893/1225 [00:03<00:01, 296.10it/s]
74%|#######3 | Scoring GeneralizingEstimator : 903/1225 [00:03<00:01, 286.81it/s]
75%|#######4 | Scoring GeneralizingEstimator : 917/1225 [00:03<00:01, 291.21it/s]
75%|#######5 | Scoring GeneralizingEstimator : 924/1225 [00:03<00:01, 278.69it/s]
77%|#######7 | Scoring GeneralizingEstimator : 944/1225 [00:03<00:00, 289.64it/s]
78%|#######7 | Scoring GeneralizingEstimator : 955/1225 [00:03<00:00, 281.34it/s]
80%|#######9 | Scoring GeneralizingEstimator : 974/1225 [00:03<00:00, 291.14it/s]
80%|######## | Scoring GeneralizingEstimator : 985/1225 [00:03<00:00, 282.77it/s]
81%|########1 | Scoring GeneralizingEstimator : 997/1225 [00:03<00:00, 285.17it/s]
82%|########1 | Scoring GeneralizingEstimator : 1002/1225 [00:03<00:00, 280.28it/s]
83%|########3 | Scoring GeneralizingEstimator : 1019/1225 [00:03<00:00, 278.31it/s]
84%|########3 | Scoring GeneralizingEstimator : 1028/1225 [00:03<00:00, 269.29it/s]
86%|########5 | Scoring GeneralizingEstimator : 1049/1225 [00:03<00:00, 281.48it/s]
87%|########6 | Scoring GeneralizingEstimator : 1060/1225 [00:03<00:00, 273.81it/s]
88%|########8 | Scoring GeneralizingEstimator : 1080/1225 [00:03<00:00, 284.77it/s]
89%|########9 | Scoring GeneralizingEstimator : 1091/1225 [00:03<00:00, 276.93it/s]
91%|######### | Scoring GeneralizingEstimator : 1111/1225 [00:03<00:00, 287.79it/s]
92%|#########1| Scoring GeneralizingEstimator : 1123/1225 [00:04<00:00, 280.72it/s]
93%|#########2| Scoring GeneralizingEstimator : 1137/1225 [00:04<00:00, 285.30it/s]
93%|#########3| Scoring GeneralizingEstimator : 1144/1225 [00:04<00:00, 273.40it/s]
95%|#########4| Scoring GeneralizingEstimator : 1163/1225 [00:04<00:00, 283.33it/s]
96%|#########5| Scoring GeneralizingEstimator : 1172/1225 [00:04<00:00, 273.61it/s]
97%|#########7| Scoring GeneralizingEstimator : 1191/1225 [00:04<00:00, 283.52it/s]
98%|#########8| Scoring GeneralizingEstimator : 1201/1225 [00:04<00:00, 274.74it/s]
100%|#########9| Scoring GeneralizingEstimator : 1220/1225 [00:04<00:00, 284.59it/s]
100%|##########| Scoring GeneralizingEstimator : 1225/1225 [00:04<00:00, 281.24it/s]
Plot
fig, ax = plt.subplots(1)
im = ax.matshow(scores, vmin=0, vmax=1., cmap='RdBu_r', origin='lower',
extent=epochs.times[[0, -1, 0, -1]])
ax.axhline(0., color='k')
ax.axvline(0., color='k')
ax.xaxis.set_ticks_position('bottom')
ax.set_xlabel('Testing Time (s)')
ax.set_ylabel('Training Time (s)')
ax.set_title('Generalization across time and condition')
plt.colorbar(im, ax=ax)
plt.show()
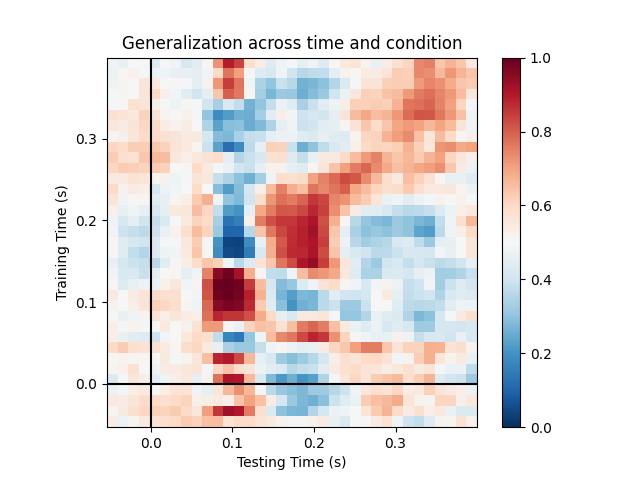
References¶
- 1
Jean-Rémi King and Stanislas Dehaene. Characterizing the dynamics of mental representations: the temporal generalization method. Trends in Cognitive Sciences, 18(4):203–210, 2014. doi:10.1016/j.tics.2014.01.002.
Total running time of the script: ( 0 minutes 11.512 seconds)
Estimated memory usage: 128 MB