Note
Go to the end to download the full example code.
Time-frequency on simulated data (Multitaper vs. Morlet vs. Stockwell vs. Hilbert)#
This example demonstrates the different time-frequency estimation methods on simulated data. It shows the time-frequency resolution trade-off and the problem of estimation variance. In addition it highlights alternative functions for generating TFRs without averaging across trials, or by operating on numpy arrays.
# Authors: Hari Bharadwaj <hari@nmr.mgh.harvard.edu>
# Denis Engemann <denis.engemann@gmail.com>
# Chris Holdgraf <choldgraf@berkeley.edu>
# Alex Rockhill <aprockhill@mailbox.org>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
import numpy as np
from matplotlib import pyplot as plt
from mne import Epochs, create_info
from mne.io import RawArray
from mne.time_frequency import AverageTFRArray, EpochsTFRArray, tfr_array_morlet
print(__doc__)
Simulate data#
We’ll simulate data with a known spectro-temporal structure.
sfreq = 1000.0
ch_names = ["SIM0001", "SIM0002"]
ch_types = ["grad", "grad"]
info = create_info(ch_names=ch_names, sfreq=sfreq, ch_types=ch_types)
n_times = 1024 # Just over 1 second epochs
n_epochs = 40
seed = 42
rng = np.random.RandomState(seed)
data = rng.randn(len(ch_names), n_times * n_epochs + 200) # buffer
# Add a 50 Hz sinusoidal burst to the noise and ramp it.
t = np.arange(n_times, dtype=np.float64) / sfreq
signal = np.sin(np.pi * 2.0 * 50.0 * t) # 50 Hz sinusoid signal
signal[np.logical_or(t < 0.45, t > 0.55)] = 0.0 # hard windowing
on_time = np.logical_and(t >= 0.45, t <= 0.55)
signal[on_time] *= np.hanning(on_time.sum()) # ramping
data[:, 100:-100] += np.tile(signal, n_epochs) # add signal
raw = RawArray(data, info)
events = np.zeros((n_epochs, 3), dtype=int)
events[:, 0] = np.arange(n_epochs) * n_times
epochs = Epochs(
raw,
events,
dict(sin50hz=0),
tmin=0,
tmax=n_times / sfreq,
reject=dict(grad=4000),
baseline=None,
)
epochs.average().plot()
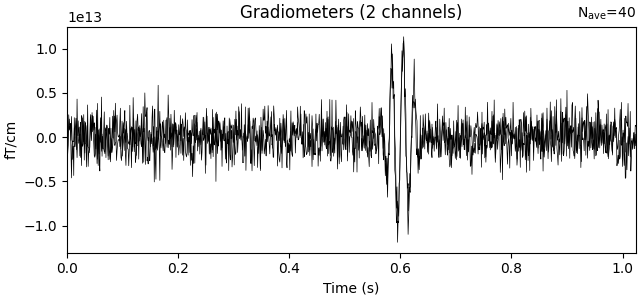
Creating RawArray with float64 data, n_channels=2, n_times=41160
Range : 0 ... 41159 = 0.000 ... 41.159 secs
Ready.
Not setting metadata
40 matching events found
No baseline correction applied
0 projection items activated
Need more than one channel to make topography for grad. Disabling interactivity.
Calculate a time-frequency representation (TFR)#
Below we’ll demonstrate the output of several TFR functions in MNE:
Multitaper transform#
First we’ll use the multitaper method for calculating the TFR.
This creates several orthogonal tapering windows in the TFR estimation,
which reduces variance. We’ll also show some of the parameters that can be
tweaked (e.g., time_bandwidth
) that will result in different multitaper
properties, and thus a different TFR. You can trade time resolution or
frequency resolution or both in order to get a reduction in variance.
freqs = np.arange(5.0, 100.0, 3.0)
vmin, vmax = -3.0, 3.0 # Define our color limits.
fig, axs = plt.subplots(1, 3, figsize=(15, 5), sharey=True, layout="constrained")
for n_cycles, time_bandwidth, ax, title in zip(
[freqs / 2, freqs, freqs / 2], # number of cycles
[2.0, 4.0, 8.0], # time bandwidth
axs,
[
"Sim: Least smoothing, most variance",
"Sim: Less frequency smoothing,\nmore time smoothing",
"Sim: Less time smoothing,\nmore frequency smoothing",
],
):
power = epochs.compute_tfr(
method="multitaper",
freqs=freqs,
n_cycles=n_cycles,
time_bandwidth=time_bandwidth,
return_itc=False,
average=True,
)
ax.set_title(title)
# Plot results. Baseline correct based on first 100 ms.
power.plot(
[0],
baseline=(0.0, 0.1),
mode="mean",
vlim=(vmin, vmax),
axes=ax,
show=False,
colorbar=False,
)
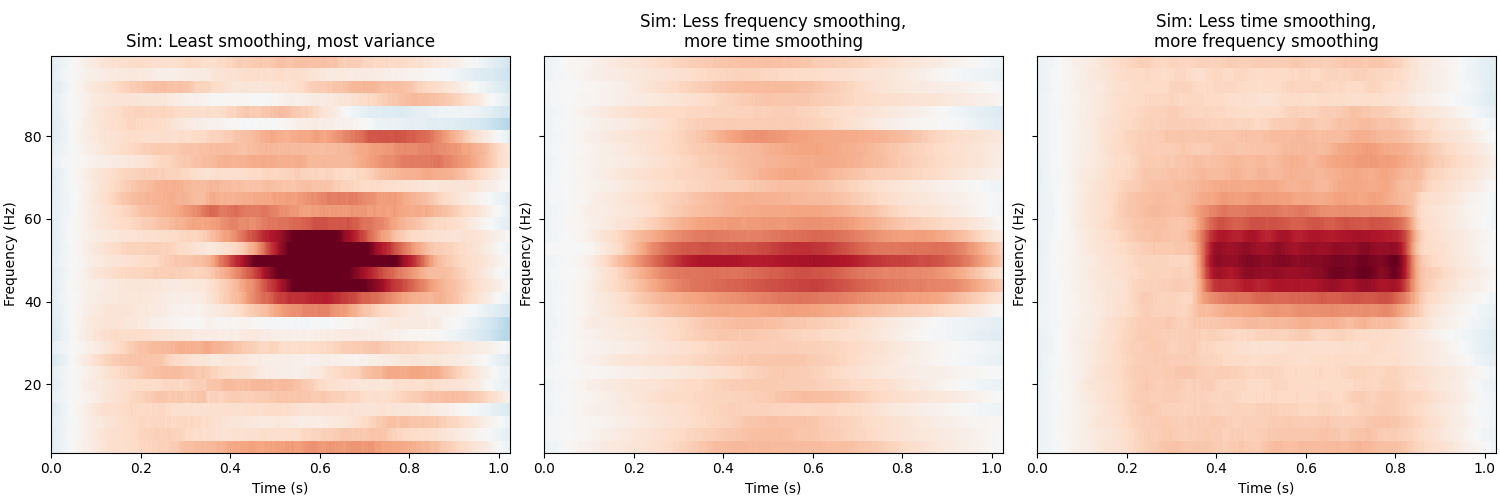
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Applying baseline correction (mode: mean)
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Stockwell (S) transform#
Stockwell uses a Gaussian window to balance temporal and spectral resolution.
Importantly, frequency bands are phase-normalized, hence strictly comparable
with regard to timing, and, the input signal can be recoverd from the
transform in a lossless way if we disregard numerical errors. In this case,
we control the spectral / temporal resolution by specifying different widths
of the gaussian window using the width
parameter.
fig, axs = plt.subplots(1, 3, figsize=(15, 5), sharey=True, layout="constrained")
fmin, fmax = freqs[[0, -1]]
for width, ax in zip((0.2, 0.7, 3.0), axs):
power = epochs.compute_tfr(method="stockwell", freqs=(fmin, fmax), width=width)
power.plot(
[0], baseline=(0.0, 0.1), mode="mean", axes=ax, show=False, colorbar=False
)
ax.set_title(f"Sim: Using S transform, width = {width:0.1f}")
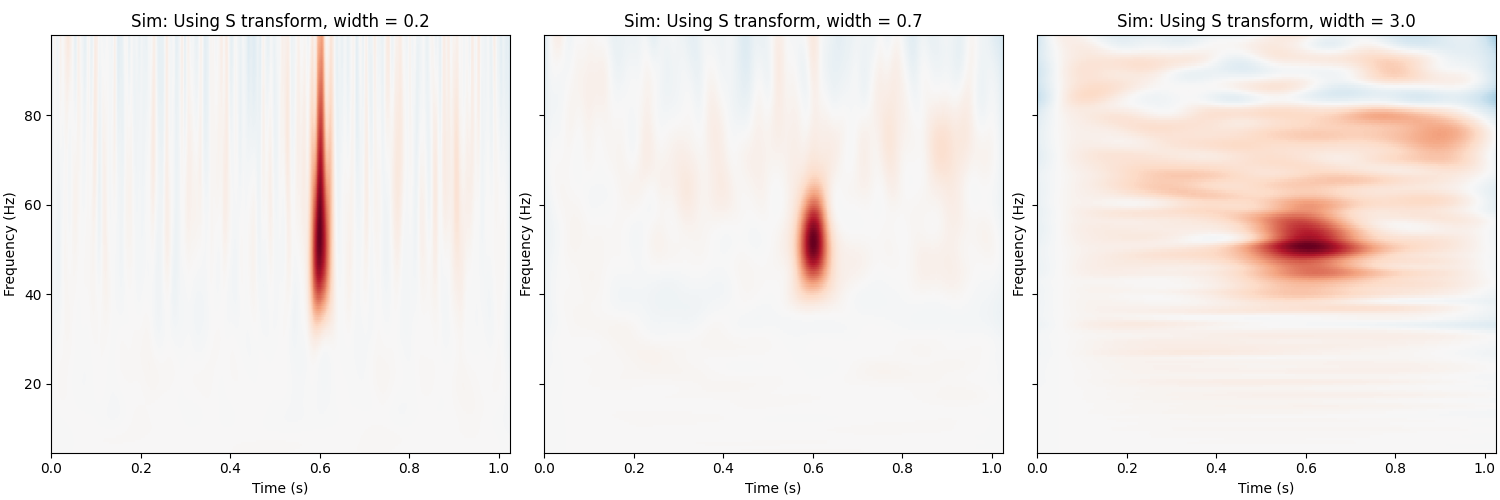
Requested `method="stockwell"` so ignoring parameter `average=False`.
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Using data from preloaded Raw for 40 events and 1025 original time points ...
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Applying baseline correction (mode: mean)
Requested `method="stockwell"` so ignoring parameter `average=False`.
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Using data from preloaded Raw for 40 events and 1025 original time points ...
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Applying baseline correction (mode: mean)
Requested `method="stockwell"` so ignoring parameter `average=False`.
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Using data from preloaded Raw for 40 events and 1025 original time points ...
The input signal is shorter (1025) than "n_fft" (2048). Applying zero padding.
Applying baseline correction (mode: mean)
Morlet Wavelets#
Next, we’ll show the TFR using morlet wavelets, which are a sinusoidal wave
with a gaussian envelope. We can control the balance between spectral and
temporal resolution with the n_cycles
parameter, which defines the
number of cycles to include in the window.
fig, axs = plt.subplots(1, 3, figsize=(15, 5), sharey=True, layout="constrained")
all_n_cycles = [1, 3, freqs / 2.0]
for n_cycles, ax in zip(all_n_cycles, axs):
power = epochs.compute_tfr(
method="morlet", freqs=freqs, n_cycles=n_cycles, return_itc=False, average=True
)
power.plot(
[0],
baseline=(0.0, 0.1),
mode="mean",
vlim=(vmin, vmax),
axes=ax,
show=False,
colorbar=False,
)
n_cycles = "scaled by freqs" if not isinstance(n_cycles, int) else n_cycles
ax.set_title(f"Sim: Using Morlet wavelet, n_cycles = {n_cycles}")
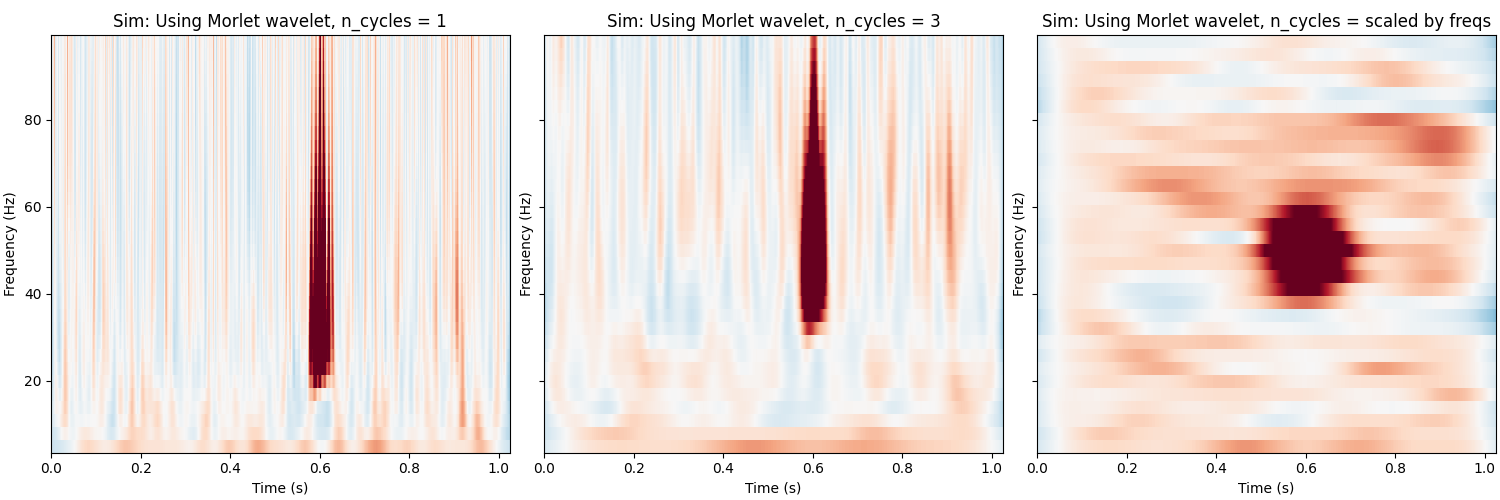
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Narrow-bandpass Filter and Hilbert Transform#
Finally, we’ll show a time-frequency representation using a narrow bandpass filter and the Hilbert transform. Choosing the right filter parameters is important so that you isolate only one oscillation of interest, generally the width of this filter is recommended to be about 2 Hz.
fig, axs = plt.subplots(1, 3, figsize=(15, 5), sharey=True, layout="constrained")
bandwidths = [1.0, 2.0, 4.0]
for bandwidth, ax in zip(bandwidths, axs):
data = np.zeros(
(len(epochs), len(ch_names), freqs.size, epochs.times.size), dtype=complex
)
for idx, freq in enumerate(freqs):
# Filter raw data and re-epoch to avoid the filter being longer than
# the epoch data for low frequencies and short epochs, such as here.
raw_filter = raw.copy()
# NOTE: The bandwidths of the filters are changed from their defaults
# to exaggerate differences. With the default transition bandwidths,
# these are all very similar because the filters are almost the same.
# In practice, using the default is usually a wise choice.
raw_filter.filter(
l_freq=freq - bandwidth / 2,
h_freq=freq + bandwidth / 2,
# no negative values for large bandwidth and low freq
l_trans_bandwidth=min([4 * bandwidth, freq - bandwidth]),
h_trans_bandwidth=4 * bandwidth,
)
raw_filter.apply_hilbert()
epochs_hilb = Epochs(
raw_filter, events, tmin=0, tmax=n_times / sfreq, baseline=(0, 0.1)
)
data[:, :, idx] = epochs_hilb.get_data()
power = EpochsTFRArray(epochs.info, data, epochs.times, freqs, method="hilbert")
power.average().plot(
[0],
baseline=(0.0, 0.1),
mode="mean",
vlim=(0, 0.1),
axes=ax,
show=False,
colorbar=False,
)
n_cycles = "scaled by freqs" if not isinstance(n_cycles, int) else n_cycles
ax.set_title(
"Sim: Using narrow bandpass filter Hilbert,\n"
f"bandwidth = {bandwidth}, "
f"transition bandwidth = {4 * bandwidth}"
)
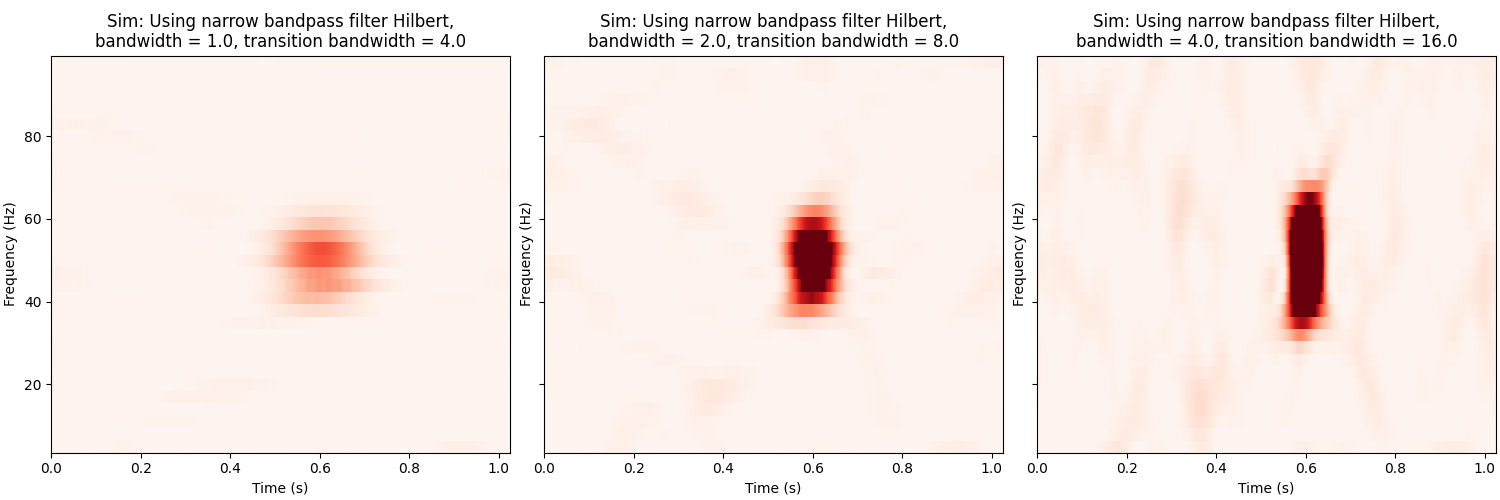
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 4.5 - 5.5 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 4.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 2.50 Hz)
- Upper passband edge: 5.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 7.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 7.5 - 8.5 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 7.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 5.50 Hz)
- Upper passband edge: 8.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 10.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 10 - 12 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 8.50 Hz)
- Upper passband edge: 11.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 13.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 14 - 14 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 13.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 11.50 Hz)
- Upper passband edge: 14.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 16.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 16 - 18 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 16.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 14.50 Hz)
- Upper passband edge: 17.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 19.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 20 - 20 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 19.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 17.50 Hz)
- Upper passband edge: 20.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 22.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 22 - 24 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 22.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 20.50 Hz)
- Upper passband edge: 23.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 25.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 26 - 26 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 25.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 23.50 Hz)
- Upper passband edge: 26.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 28.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 28 - 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 28.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 26.50 Hz)
- Upper passband edge: 29.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 31.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 32 - 32 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 31.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 29.50 Hz)
- Upper passband edge: 32.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 34.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 34 - 36 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 34.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 32.50 Hz)
- Upper passband edge: 35.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 37.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 38 - 38 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 37.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 35.50 Hz)
- Upper passband edge: 38.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 40.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 40 - 42 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 40.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 38.50 Hz)
- Upper passband edge: 41.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 43.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 44 - 44 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 43.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 41.50 Hz)
- Upper passband edge: 44.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 46.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 46 - 48 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 46.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 44.50 Hz)
- Upper passband edge: 47.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 49.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 50 - 50 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 49.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 47.50 Hz)
- Upper passband edge: 50.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 52.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 52 - 54 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 52.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 50.50 Hz)
- Upper passband edge: 53.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 55.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 56 - 56 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 55.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 53.50 Hz)
- Upper passband edge: 56.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 58.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 58 - 60 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 58.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 56.50 Hz)
- Upper passband edge: 59.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 61.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 62 - 62 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 61.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 59.50 Hz)
- Upper passband edge: 62.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 64.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 64 - 66 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 64.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 62.50 Hz)
- Upper passband edge: 65.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 67.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 68 - 68 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 67.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 65.50 Hz)
- Upper passband edge: 68.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 70.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 70 - 72 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 70.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 68.50 Hz)
- Upper passband edge: 71.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 73.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 74 - 74 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 73.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 71.50 Hz)
- Upper passband edge: 74.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 76.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 76 - 78 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 76.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 74.50 Hz)
- Upper passband edge: 77.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 79.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 80 - 80 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 79.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 77.50 Hz)
- Upper passband edge: 80.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 82.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 82 - 84 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 82.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 80.50 Hz)
- Upper passband edge: 83.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 85.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 86 - 86 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 85.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 83.50 Hz)
- Upper passband edge: 86.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 88.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 88 - 90 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 88.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 86.50 Hz)
- Upper passband edge: 89.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 91.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 92 - 92 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 91.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 89.50 Hz)
- Upper passband edge: 92.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 94.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 94 - 96 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 94.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 92.50 Hz)
- Upper passband edge: 95.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 97.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 98 - 98 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 97.50
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 95.50 Hz)
- Upper passband edge: 98.50 Hz
- Upper transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 100.50 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Applying baseline correction (mode: mean)
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 4 - 6 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 4.00
- Lower transition bandwidth: 3.00 Hz (-6 dB cutoff frequency: 2.50 Hz)
- Upper passband edge: 6.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 10.00 Hz)
- Filter length: 1101 samples (1.101 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 7 - 9 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 7.00
- Lower transition bandwidth: 6.00 Hz (-6 dB cutoff frequency: 4.00 Hz)
- Upper passband edge: 9.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 13.00 Hz)
- Filter length: 551 samples (0.551 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 10 - 12 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 6.00 Hz)
- Upper passband edge: 12.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 16.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 13 - 15 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 13.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 9.00 Hz)
- Upper passband edge: 15.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 19.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 16 - 18 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 16.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 12.00 Hz)
- Upper passband edge: 18.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 22.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 19 - 21 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 19.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 15.00 Hz)
- Upper passband edge: 21.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 25.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 22 - 24 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 22.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 18.00 Hz)
- Upper passband edge: 24.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 28.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 25 - 27 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 25.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 21.00 Hz)
- Upper passband edge: 27.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 31.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 28 - 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 28.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 24.00 Hz)
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 34.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 31 - 33 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 31.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 27.00 Hz)
- Upper passband edge: 33.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 37.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 34 - 36 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 34.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 30.00 Hz)
- Upper passband edge: 36.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 40.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 37 - 39 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 37.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 33.00 Hz)
- Upper passband edge: 39.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 43.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 40 - 42 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 40.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 36.00 Hz)
- Upper passband edge: 42.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 46.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 43 - 45 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 43.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 39.00 Hz)
- Upper passband edge: 45.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 49.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 46 - 48 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 46.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 42.00 Hz)
- Upper passband edge: 48.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 52.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 49 - 51 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 49.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Upper passband edge: 51.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 55.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 52 - 54 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 52.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 48.00 Hz)
- Upper passband edge: 54.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 58.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 55 - 57 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 55.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 51.00 Hz)
- Upper passband edge: 57.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 61.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 58 - 60 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 58.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 54.00 Hz)
- Upper passband edge: 60.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 64.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 61 - 63 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 61.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 57.00 Hz)
- Upper passband edge: 63.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 67.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 64 - 66 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 64.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 60.00 Hz)
- Upper passband edge: 66.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 70.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 67 - 69 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 67.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 63.00 Hz)
- Upper passband edge: 69.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 73.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 70 - 72 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 70.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 66.00 Hz)
- Upper passband edge: 72.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 76.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 73 - 75 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 73.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 69.00 Hz)
- Upper passband edge: 75.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 79.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 76 - 78 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 76.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 72.00 Hz)
- Upper passband edge: 78.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 82.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 79 - 81 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 79.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 75.00 Hz)
- Upper passband edge: 81.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 85.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 82 - 84 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 82.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 78.00 Hz)
- Upper passband edge: 84.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 88.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 85 - 87 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 85.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 81.00 Hz)
- Upper passband edge: 87.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 91.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 88 - 90 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 88.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 84.00 Hz)
- Upper passband edge: 90.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 94.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 91 - 93 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 91.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 87.00 Hz)
- Upper passband edge: 93.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 97.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 94 - 96 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 94.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 90.00 Hz)
- Upper passband edge: 96.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 100.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 97 - 99 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 97.00
- Lower transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 93.00 Hz)
- Upper passband edge: 99.00 Hz
- Upper transition bandwidth: 8.00 Hz (-6 dB cutoff frequency: 103.00 Hz)
- Filter length: 413 samples (0.413 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Applying baseline correction (mode: mean)
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 3 - 7 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 3.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 2.50 Hz)
- Upper passband edge: 7.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 15.00 Hz)
- Filter length: 3301 samples (3.301 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 6 - 10 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 6.00
- Lower transition bandwidth: 4.00 Hz (-6 dB cutoff frequency: 4.00 Hz)
- Upper passband edge: 10.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 18.00 Hz)
- Filter length: 825 samples (0.825 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 9 - 13 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 9.00
- Lower transition bandwidth: 7.00 Hz (-6 dB cutoff frequency: 5.50 Hz)
- Upper passband edge: 13.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 21.00 Hz)
- Filter length: 473 samples (0.473 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 12 - 16 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 12.00
- Lower transition bandwidth: 10.00 Hz (-6 dB cutoff frequency: 7.00 Hz)
- Upper passband edge: 16.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 24.00 Hz)
- Filter length: 331 samples (0.331 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 15 - 19 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 15.00
- Lower transition bandwidth: 13.00 Hz (-6 dB cutoff frequency: 8.50 Hz)
- Upper passband edge: 19.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 27.00 Hz)
- Filter length: 255 samples (0.255 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 18 - 22 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 18.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 10.00 Hz)
- Upper passband edge: 22.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 30.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 21 - 25 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 21.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 13.00 Hz)
- Upper passband edge: 25.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 33.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 24 - 28 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 24.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 16.00 Hz)
- Upper passband edge: 28.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 36.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 27 - 31 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 27.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 19.00 Hz)
- Upper passband edge: 31.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 39.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 30 - 34 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 30.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 22.00 Hz)
- Upper passband edge: 34.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 42.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 33 - 37 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 33.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 25.00 Hz)
- Upper passband edge: 37.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 36 - 40 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 36.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 28.00 Hz)
- Upper passband edge: 40.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 48.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 39 - 43 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 39.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 31.00 Hz)
- Upper passband edge: 43.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 51.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 42 - 46 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 42.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 34.00 Hz)
- Upper passband edge: 46.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 54.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 45 - 49 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 45.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 37.00 Hz)
- Upper passband edge: 49.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 57.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 48 - 52 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 48.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 40.00 Hz)
- Upper passband edge: 52.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 60.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 51 - 55 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 51.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 43.00 Hz)
- Upper passband edge: 55.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 63.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 54 - 58 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 54.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 46.00 Hz)
- Upper passband edge: 58.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 66.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 57 - 61 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 57.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 49.00 Hz)
- Upper passband edge: 61.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 69.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 60 - 64 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 60.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 52.00 Hz)
- Upper passband edge: 64.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 72.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 63 - 67 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 63.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 55.00 Hz)
- Upper passband edge: 67.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 75.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 66 - 70 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 66.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 58.00 Hz)
- Upper passband edge: 70.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 78.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 69 - 73 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 69.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 61.00 Hz)
- Upper passband edge: 73.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 81.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 72 - 76 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 72.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 64.00 Hz)
- Upper passband edge: 76.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 84.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 75 - 79 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 75.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 67.00 Hz)
- Upper passband edge: 79.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 87.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 78 - 82 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 78.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 70.00 Hz)
- Upper passband edge: 82.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 90.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 81 - 85 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 81.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 73.00 Hz)
- Upper passband edge: 85.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 93.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 84 - 88 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 84.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 76.00 Hz)
- Upper passband edge: 88.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 96.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 87 - 91 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 87.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 79.00 Hz)
- Upper passband edge: 91.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 99.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 90 - 94 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 90.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 82.00 Hz)
- Upper passband edge: 94.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 102.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 93 - 97 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 93.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 85.00 Hz)
- Upper passband edge: 97.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 105.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 96 - 1e+02 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 96.00
- Lower transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 88.00 Hz)
- Upper passband edge: 100.00 Hz
- Upper transition bandwidth: 16.00 Hz (-6 dB cutoff frequency: 108.00 Hz)
- Filter length: 207 samples (0.207 s)
Not setting metadata
40 matching events found
Applying baseline correction (mode: mean)
0 projection items activated
Using data from preloaded Raw for 40 events and 1025 original time points ...
0 bad epochs dropped
Applying baseline correction (mode: mean)
Calculating a TFR without averaging over epochs#
It is also possible to calculate a TFR without averaging across trials.
We can do this by using average=False
. In this case, an instance of
mne.time_frequency.EpochsTFR
is returned.
n_cycles = freqs / 2.0
power = epochs.compute_tfr(
method="morlet", freqs=freqs, n_cycles=n_cycles, return_itc=False, average=False
)
print(type(power))
avgpower = power.average()
avgpower.plot(
[0],
baseline=(0.0, 0.1),
mode="mean",
vlim=(vmin, vmax),
title="Using Morlet wavelets and EpochsTFR",
show=False,
)
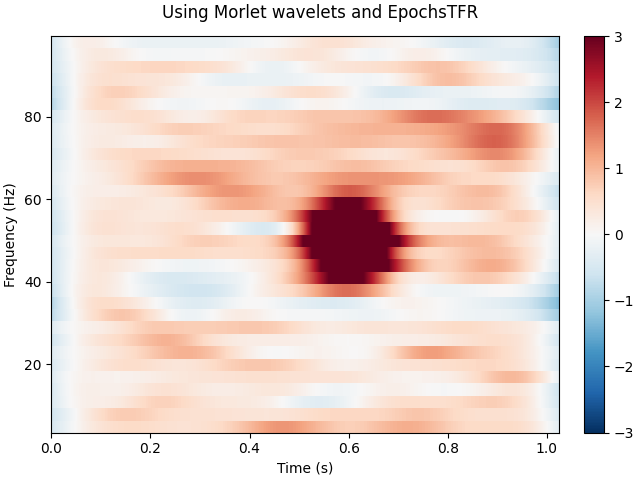
Using data from preloaded Raw for 40 events and 1025 original time points ...
<class 'mne.time_frequency.tfr.EpochsTFR'>
Applying baseline correction (mode: mean)
Operating on arrays#
MNE-Python also has functions that operate on NumPy arrays
instead of MNE-Python objects. These are tfr_array_morlet()
and tfr_array_multitaper()
. They expect inputs of the shape
(n_epochs, n_channels, n_times)
and return an array of shape
(n_epochs, n_channels, n_freqs, n_times)
(or optionally, can collapse the epochs
dimension if you want average power or inter-trial coherence; see output
param).
power = tfr_array_morlet(
epochs.get_data(),
sfreq=epochs.info["sfreq"],
freqs=freqs,
n_cycles=n_cycles,
output="avg_power",
zero_mean=False,
)
# Put it into a TFR container for easy plotting
tfr = AverageTFRArray(
info=epochs.info, data=power, times=epochs.times, freqs=freqs, nave=len(epochs)
)
tfr.plot(
baseline=(0.0, 0.1),
picks=[0],
mode="mean",
vlim=(vmin, vmax),
title="TFR calculated on a NumPy array",
)
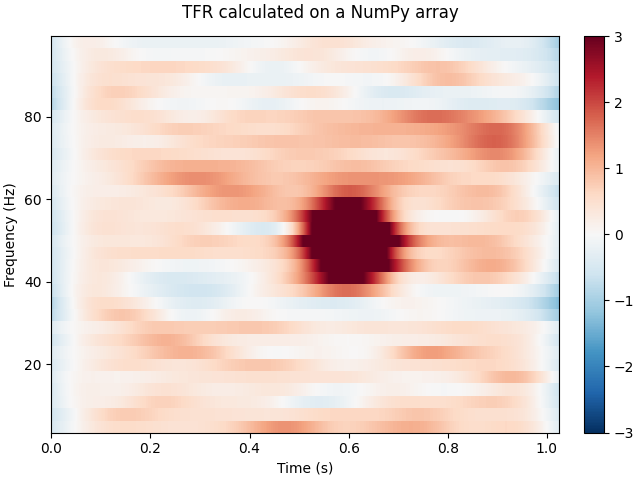
Using data from preloaded Raw for 40 events and 1025 original time points ...
Applying baseline correction (mode: mean)
Total running time of the script: (0 minutes 14.199 seconds)