Note
Go to the end to download the full example code.
Decoding sensor space data with generalization across time and conditions#
This example runs the analysis described in [1]. It illustrates how one can fit a linear classifier to identify a discriminatory topography at a given time instant and subsequently assess whether this linear model can accurately predict all of the time samples of a second set of conditions.
# Authors: Jean-Rémi King <jeanremi.king@gmail.com>
# Alexandre Gramfort <alexandre.gramfort@inria.fr>
# Denis Engemann <denis.engemann@gmail.com>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
import matplotlib.pyplot as plt
from sklearn.linear_model import LogisticRegression
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
import mne
from mne.datasets import sample
from mne.decoding import GeneralizingEstimator
print(__doc__)
# Preprocess data
data_path = sample.data_path()
# Load and filter data, set up epochs
meg_path = data_path / "MEG" / "sample"
raw_fname = meg_path / "sample_audvis_filt-0-40_raw.fif"
events_fname = meg_path / "sample_audvis_filt-0-40_raw-eve.fif"
raw = mne.io.read_raw_fif(raw_fname, preload=True)
picks = mne.pick_types(raw.info, meg=True, exclude="bads") # Pick MEG channels
raw.filter(1.0, 30.0, fir_design="firwin") # Band pass filtering signals
events = mne.read_events(events_fname)
event_id = {
"Auditory/Left": 1,
"Auditory/Right": 2,
"Visual/Left": 3,
"Visual/Right": 4,
}
tmin = -0.050
tmax = 0.400
# decimate to make the example faster to run, but then use verbose='error' in
# the Epochs constructor to suppress warning about decimation causing aliasing
decim = 2
epochs = mne.Epochs(
raw,
events,
event_id=event_id,
tmin=tmin,
tmax=tmax,
proj=True,
picks=picks,
baseline=None,
preload=True,
reject=dict(mag=5e-12),
decim=decim,
verbose="error",
)
Opening raw data file /home/circleci/mne_data/MNE-sample-data/MEG/sample/sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Reading 0 ... 41699 = 0.000 ... 277.709 secs...
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 0.50 Hz)
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 497 samples (3.310 s)
[Parallel(n_jobs=1)]: Done 17 tasks | elapsed: 0.0s
[Parallel(n_jobs=1)]: Done 71 tasks | elapsed: 0.1s
[Parallel(n_jobs=1)]: Done 161 tasks | elapsed: 0.3s
[Parallel(n_jobs=1)]: Done 287 tasks | elapsed: 0.5s
We will train the classifier on all left visual vs auditory trials and test on all right visual vs auditory trials.
clf = make_pipeline(
StandardScaler(),
LogisticRegression(solver="liblinear"), # liblinear is faster than lbfgs
)
time_gen = GeneralizingEstimator(clf, scoring="roc_auc", n_jobs=None, verbose=True)
# Fit classifiers on the epochs where the stimulus was presented to the left.
# Note that the experimental condition y indicates auditory or visual
time_gen.fit(X=epochs["Left"].get_data(copy=False), y=epochs["Left"].events[:, 2] > 2)
0%| | Fitting GeneralizingEstimator : 0/35 [00:00<?, ?it/s]
6%|▌ | Fitting GeneralizingEstimator : 2/35 [00:00<00:00, 56.93it/s]
11%|█▏ | Fitting GeneralizingEstimator : 4/35 [00:00<00:00, 58.15it/s]
17%|█▋ | Fitting GeneralizingEstimator : 6/35 [00:00<00:00, 58.57it/s]
23%|██▎ | Fitting GeneralizingEstimator : 8/35 [00:00<00:00, 58.72it/s]
31%|███▏ | Fitting GeneralizingEstimator : 11/35 [00:00<00:00, 64.90it/s]
40%|████ | Fitting GeneralizingEstimator : 14/35 [00:00<00:00, 69.41it/s]
49%|████▊ | Fitting GeneralizingEstimator : 17/35 [00:00<00:00, 72.63it/s]
57%|█████▋ | Fitting GeneralizingEstimator : 20/35 [00:00<00:00, 75.06it/s]
66%|██████▌ | Fitting GeneralizingEstimator : 23/35 [00:00<00:00, 76.24it/s]
71%|███████▏ | Fitting GeneralizingEstimator : 25/35 [00:00<00:00, 74.18it/s]
80%|████████ | Fitting GeneralizingEstimator : 28/35 [00:00<00:00, 75.84it/s]
86%|████████▌ | Fitting GeneralizingEstimator : 30/35 [00:00<00:00, 74.08it/s]
94%|█████████▍| Fitting GeneralizingEstimator : 33/35 [00:00<00:00, 75.61it/s]
100%|██████████| Fitting GeneralizingEstimator : 35/35 [00:00<00:00, 76.27it/s]
100%|██████████| Fitting GeneralizingEstimator : 35/35 [00:00<00:00, 75.00it/s]
Score on the epochs where the stimulus was presented to the right.
scores = time_gen.score(
X=epochs["Right"].get_data(copy=False), y=epochs["Right"].events[:, 2] > 2
)
0%| | Scoring GeneralizingEstimator : 0/1225 [00:00<?, ?it/s]
1%| | Scoring GeneralizingEstimator : 12/1225 [00:00<00:03, 347.29it/s]
2%|▏ | Scoring GeneralizingEstimator : 25/1225 [00:00<00:03, 366.55it/s]
3%|▎ | Scoring GeneralizingEstimator : 38/1225 [00:00<00:03, 373.11it/s]
4%|▍ | Scoring GeneralizingEstimator : 52/1225 [00:00<00:03, 382.06it/s]
5%|▌ | Scoring GeneralizingEstimator : 66/1225 [00:00<00:02, 389.02it/s]
7%|▋ | Scoring GeneralizingEstimator : 80/1225 [00:00<00:02, 393.42it/s]
8%|▊ | Scoring GeneralizingEstimator : 94/1225 [00:00<00:02, 397.11it/s]
9%|▉ | Scoring GeneralizingEstimator : 108/1225 [00:00<00:02, 398.16it/s]
10%|▉ | Scoring GeneralizingEstimator : 121/1225 [00:00<00:02, 396.55it/s]
11%|█ | Scoring GeneralizingEstimator : 134/1225 [00:00<00:02, 394.97it/s]
12%|█▏ | Scoring GeneralizingEstimator : 147/1225 [00:00<00:02, 394.02it/s]
13%|█▎ | Scoring GeneralizingEstimator : 161/1225 [00:00<00:02, 393.74it/s]
14%|█▍ | Scoring GeneralizingEstimator : 174/1225 [00:00<00:02, 393.00it/s]
15%|█▌ | Scoring GeneralizingEstimator : 188/1225 [00:00<00:02, 395.08it/s]
16%|█▋ | Scoring GeneralizingEstimator : 201/1225 [00:00<00:02, 394.27it/s]
17%|█▋ | Scoring GeneralizingEstimator : 214/1225 [00:00<00:02, 393.28it/s]
19%|█▊ | Scoring GeneralizingEstimator : 228/1225 [00:00<00:02, 394.77it/s]
20%|█▉ | Scoring GeneralizingEstimator : 241/1225 [00:00<00:02, 394.00it/s]
21%|██ | Scoring GeneralizingEstimator : 255/1225 [00:00<00:02, 395.44it/s]
22%|██▏ | Scoring GeneralizingEstimator : 268/1225 [00:00<00:02, 394.75it/s]
23%|██▎ | Scoring GeneralizingEstimator : 281/1225 [00:00<00:02, 393.31it/s]
24%|██▍ | Scoring GeneralizingEstimator : 295/1225 [00:00<00:02, 394.83it/s]
25%|██▌ | Scoring GeneralizingEstimator : 308/1225 [00:00<00:02, 394.19it/s]
26%|██▋ | Scoring GeneralizingEstimator : 322/1225 [00:00<00:02, 394.24it/s]
27%|██▋ | Scoring GeneralizingEstimator : 335/1225 [00:00<00:02, 393.71it/s]
28%|██▊ | Scoring GeneralizingEstimator : 348/1225 [00:00<00:02, 393.21it/s]
29%|██▉ | Scoring GeneralizingEstimator : 361/1225 [00:00<00:02, 392.72it/s]
31%|███ | Scoring GeneralizingEstimator : 375/1225 [00:00<00:02, 394.17it/s]
32%|███▏ | Scoring GeneralizingEstimator : 389/1225 [00:00<00:02, 395.52it/s]
33%|███▎ | Scoring GeneralizingEstimator : 402/1225 [00:01<00:02, 394.95it/s]
34%|███▍ | Scoring GeneralizingEstimator : 416/1225 [00:01<00:02, 396.26it/s]
35%|███▌ | Scoring GeneralizingEstimator : 431/1225 [00:01<00:01, 397.37it/s]
36%|███▌ | Scoring GeneralizingEstimator : 444/1225 [00:01<00:01, 396.70it/s]
37%|███▋ | Scoring GeneralizingEstimator : 457/1225 [00:01<00:01, 396.09it/s]
38%|███▊ | Scoring GeneralizingEstimator : 470/1225 [00:01<00:01, 395.47it/s]
39%|███▉ | Scoring GeneralizingEstimator : 483/1225 [00:01<00:01, 394.94it/s]
40%|████ | Scoring GeneralizingEstimator : 495/1225 [00:01<00:01, 392.67it/s]
41%|████▏ | Scoring GeneralizingEstimator : 508/1225 [00:01<00:01, 392.32it/s]
43%|████▎ | Scoring GeneralizingEstimator : 521/1225 [00:01<00:01, 391.71it/s]
44%|████▎ | Scoring GeneralizingEstimator : 533/1225 [00:01<00:01, 389.70it/s]
45%|████▍ | Scoring GeneralizingEstimator : 546/1225 [00:01<00:01, 389.47it/s]
46%|████▌ | Scoring GeneralizingEstimator : 560/1225 [00:01<00:01, 390.30it/s]
47%|████▋ | Scoring GeneralizingEstimator : 573/1225 [00:01<00:01, 390.10it/s]
48%|████▊ | Scoring GeneralizingEstimator : 586/1225 [00:01<00:01, 389.86it/s]
49%|████▉ | Scoring GeneralizingEstimator : 599/1225 [00:01<00:01, 389.60it/s]
50%|████▉ | Scoring GeneralizingEstimator : 612/1225 [00:01<00:01, 388.68it/s]
51%|█████ | Scoring GeneralizingEstimator : 625/1225 [00:01<00:01, 388.49it/s]
52%|█████▏ | Scoring GeneralizingEstimator : 637/1225 [00:01<00:01, 386.72it/s]
53%|█████▎ | Scoring GeneralizingEstimator : 650/1225 [00:01<00:01, 386.72it/s]
54%|█████▍ | Scoring GeneralizingEstimator : 663/1225 [00:01<00:01, 386.70it/s]
55%|█████▌ | Scoring GeneralizingEstimator : 676/1225 [00:01<00:01, 385.38it/s]
56%|█████▌ | Scoring GeneralizingEstimator : 687/1225 [00:01<00:01, 382.25it/s]
57%|█████▋ | Scoring GeneralizingEstimator : 698/1225 [00:01<00:01, 379.30it/s]
58%|█████▊ | Scoring GeneralizingEstimator : 711/1225 [00:01<00:01, 379.65it/s]
59%|█████▉ | Scoring GeneralizingEstimator : 724/1225 [00:01<00:01, 379.54it/s]
60%|██████ | Scoring GeneralizingEstimator : 736/1225 [00:01<00:01, 378.29it/s]
61%|██████ | Scoring GeneralizingEstimator : 747/1225 [00:01<00:01, 375.56it/s]
62%|██████▏ | Scoring GeneralizingEstimator : 760/1225 [00:01<00:01, 374.52it/s]
63%|██████▎ | Scoring GeneralizingEstimator : 774/1225 [00:02<00:01, 376.68it/s]
64%|██████▍ | Scoring GeneralizingEstimator : 787/1225 [00:02<00:01, 377.13it/s]
65%|██████▌ | Scoring GeneralizingEstimator : 799/1225 [00:02<00:01, 375.95it/s]
66%|██████▋ | Scoring GeneralizingEstimator : 812/1225 [00:02<00:01, 376.42it/s]
67%|██████▋ | Scoring GeneralizingEstimator : 825/1225 [00:02<00:01, 376.90it/s]
68%|██████▊ | Scoring GeneralizingEstimator : 837/1225 [00:02<00:01, 375.87it/s]
69%|██████▉ | Scoring GeneralizingEstimator : 848/1225 [00:02<00:01, 371.53it/s]
70%|███████ | Scoring GeneralizingEstimator : 860/1225 [00:02<00:00, 370.72it/s]
71%|███████▏ | Scoring GeneralizingEstimator : 873/1225 [00:02<00:00, 371.45it/s]
72%|███████▏ | Scoring GeneralizingEstimator : 886/1225 [00:02<00:00, 372.05it/s]
73%|███████▎ | Scoring GeneralizingEstimator : 898/1225 [00:02<00:00, 371.09it/s]
74%|███████▍ | Scoring GeneralizingEstimator : 908/1225 [00:02<00:00, 367.33it/s]
75%|███████▌ | Scoring GeneralizingEstimator : 921/1225 [00:02<00:00, 368.28it/s]
76%|███████▋ | Scoring GeneralizingEstimator : 935/1225 [00:02<00:00, 370.64it/s]
77%|███████▋ | Scoring GeneralizingEstimator : 948/1225 [00:02<00:00, 371.14it/s]
78%|███████▊ | Scoring GeneralizingEstimator : 960/1225 [00:02<00:00, 370.39it/s]
79%|███████▉ | Scoring GeneralizingEstimator : 973/1225 [00:02<00:00, 371.12it/s]
80%|████████ | Scoring GeneralizingEstimator : 985/1225 [00:02<00:00, 370.37it/s]
81%|████████▏ | Scoring GeneralizingEstimator : 997/1225 [00:02<00:00, 368.39it/s]
82%|████████▏ | Scoring GeneralizingEstimator : 1007/1225 [00:02<00:00, 364.74it/s]
83%|████████▎ | Scoring GeneralizingEstimator : 1020/1225 [00:02<00:00, 365.83it/s]
84%|████████▍ | Scoring GeneralizingEstimator : 1033/1225 [00:02<00:00, 366.79it/s]
85%|████████▌ | Scoring GeneralizingEstimator : 1045/1225 [00:02<00:00, 366.24it/s]
86%|████████▌ | Scoring GeneralizingEstimator : 1056/1225 [00:02<00:00, 364.22it/s]
87%|████████▋ | Scoring GeneralizingEstimator : 1067/1225 [00:02<00:00, 362.34it/s]
88%|████████▊ | Scoring GeneralizingEstimator : 1080/1225 [00:02<00:00, 362.35it/s]
89%|████████▉ | Scoring GeneralizingEstimator : 1093/1225 [00:02<00:00, 363.53it/s]
90%|█████████ | Scoring GeneralizingEstimator : 1106/1225 [00:02<00:00, 364.65it/s]
91%|█████████▏| Scoring GeneralizingEstimator : 1119/1225 [00:02<00:00, 365.70it/s]
92%|█████████▏| Scoring GeneralizingEstimator : 1131/1225 [00:02<00:00, 365.24it/s]
93%|█████████▎| Scoring GeneralizingEstimator : 1144/1225 [00:03<00:00, 366.30it/s]
94%|█████████▍| Scoring GeneralizingEstimator : 1157/1225 [00:03<00:00, 367.23it/s]
95%|█████████▌| Scoring GeneralizingEstimator : 1168/1225 [00:03<00:00, 365.16it/s]
96%|█████████▋| Scoring GeneralizingEstimator : 1180/1225 [00:03<00:00, 363.44it/s]
97%|█████████▋| Scoring GeneralizingEstimator : 1192/1225 [00:03<00:00, 363.09it/s]
98%|█████████▊| Scoring GeneralizingEstimator : 1205/1225 [00:03<00:00, 364.26it/s]
99%|█████████▉| Scoring GeneralizingEstimator : 1217/1225 [00:03<00:00, 363.86it/s]
100%|██████████| Scoring GeneralizingEstimator : 1225/1225 [00:03<00:00, 364.45it/s]
100%|██████████| Scoring GeneralizingEstimator : 1225/1225 [00:03<00:00, 376.61it/s]
Plot
fig, ax = plt.subplots(layout="constrained")
im = ax.matshow(
scores,
vmin=0,
vmax=1.0,
cmap="RdBu_r",
origin="lower",
extent=epochs.times[[0, -1, 0, -1]],
)
ax.axhline(0.0, color="k")
ax.axvline(0.0, color="k")
ax.xaxis.set_ticks_position("bottom")
ax.set_xlabel(
'Condition: "Right"\nTesting Time (s)',
)
ax.set_ylabel('Condition: "Left"\nTraining Time (s)')
ax.set_title("Generalization across time and condition", fontweight="bold")
fig.colorbar(im, ax=ax, label="Performance (ROC AUC)")
plt.show()
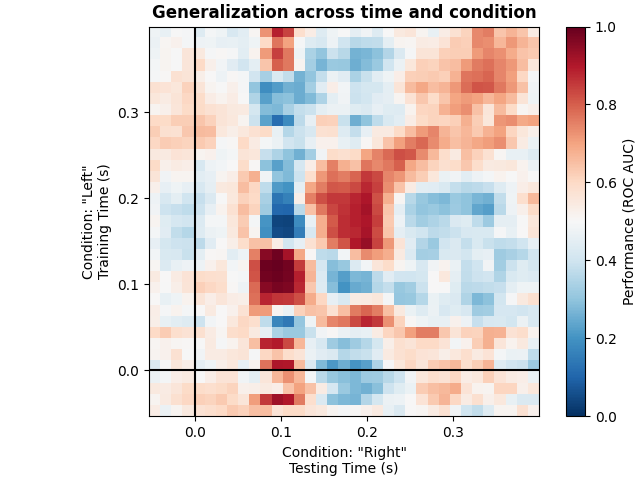
References#
Total running time of the script: (0 minutes 5.586 seconds)