mne.DipoleFixed#
- class mne.DipoleFixed(info, data, times, nave, aspect_kind, comment='', *, verbose=None)[source]#
Dipole class for fixed-position dipole fits.
Note
This class should usually not be instantiated directly via
mne.DipoleFixed(...)
. Instead, use one of the functions listed in the See Also section below.- Parameters:
- info
mne.Info
The
mne.Info
object with information about the sensors and methods of measurement.- data
array
, shape (n_channels, n_times) The dipole data.
- times
array
, shape (n_times,) The time points.
- nave
int
Number of averages.
- aspect_kind
int
The kind of data.
- comment
str
The dipole comment.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- info
- Attributes:
Methods
copy
()Copy the DipoleFixed object.
crop
([tmin, tmax, include_tmax, verbose])Crop data to a given time interval.
decimate
(decim[, offset, verbose])Decimate the time-series data.
plot
([show, time_unit])Plot dipole data.
save
(fname[, verbose])Save dipole in a .fif file.
shift_time
(tshift[, relative])Shift time scale in epoched or evoked data.
time_as_index
(times[, use_rounding])Convert time to indices.
See also
Notes
This class is for fixed-position dipole fits, where the position (and maybe orientation) is static over time. For sequential dipole fits, where the position can change a function of time, use
mne.Dipole
.New in v0.12.
- property ch_names#
Channel names.
- copy()[source]#
Copy the DipoleFixed object.
- Returns:
- instinstance of
DipoleFixed
The copy.
- instinstance of
Notes
New in v0.16.
- crop(tmin=None, tmax=None, include_tmax=True, verbose=None)[source]#
Crop data to a given time interval.
- Parameters:
- tmin
float
|None
Start time of selection in seconds.
- tmax
float
|None
End time of selection in seconds.
- include_tmaxbool
If True (default), include tmax. If False, exclude tmax (similar to how Python indexing typically works).
New in v0.19.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- tmin
- Returns:
- instinstance of
Raw
,Epochs
,Evoked
,AverageTFR
, orSourceEstimate
The cropped time-series object, modified in-place.
- instinstance of
Notes
Unlike Python slices, MNE time intervals by default include both their end points;
crop(tmin, tmax)
returns the intervaltmin <= t <= tmax
. Passinclude_tmax=False
to specify the half-open intervaltmin <= t < tmax
instead.
- decimate(decim, offset=0, *, verbose=None)[source]#
Decimate the time-series data.
- Parameters:
- decim
int
Factor by which to subsample the data.
Warning
Low-pass filtering is not performed, this simply selects every Nth sample (where N is the value passed to
decim
), i.e., it compresses the signal (see Notes). If the data are not properly filtered, aliasing artifacts may occur. See Resampling and decimating data for more information.- offset
int
Apply an offset to where the decimation starts relative to the sample corresponding to t=0. The offset is in samples at the current sampling rate.
New in v0.12.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- decim
- Returns:
- instMNE-object
The decimated object.
See also
Notes
For historical reasons,
decim
/ “decimation” refers to simply subselecting samples from a given signal. This contrasts with the broader signal processing literature, where decimation is defined as (quoting [1], p. 172; which cites [2]):“… a general system for downsampling by a factor of M is the one shown in Figure 4.23. Such a system is called a decimator, and downsampling by lowpass filtering followed by compression [i.e, subselecting samples] has been termed decimation (Crochiere and Rabiner, 1983).”
Hence “decimation” in MNE is what is considered “compression” in the signal processing community.
Decimation can be done multiple times. For example,
inst.decimate(2).decimate(2)
will be the same asinst.decimate(4)
.If
decim
is 1, this method does not copy the underlying data.New in v0.10.0.
References
- plot(show=True, time_unit='s')[source]#
Plot dipole data.
- Parameters:
- Returns:
- figinstance of
matplotlib.figure.Figure
The figure containing the time courses.
- figinstance of
Examples using
plot
:Source localization with equivalent current dipole (ECD) fit
Source localization with equivalent current dipole (ECD) fit
- save(fname, verbose=None)[source]#
Save dipole in a .fif file.
- Parameters:
- fnamepath-like
The name of the .fif file. Must end with
'.fif'
or'.fif.gz'
to make it explicit that the file contains dipole information in FIF format.- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- shift_time(tshift, relative=True)[source]#
Shift time scale in epoched or evoked data.
- Parameters:
- tshift
float
The (absolute or relative) time shift in seconds. If
relative
is True, positive tshift increases the time value associated with each sample, while negative tshift decreases it.- relativebool
If True, increase or decrease time values by
tshift
seconds. Otherwise, shift the time values such that the time of the first sample equalstshift
.
- tshift
- Returns:
- epochsMNE-object
The modified instance.
Notes
This method allows you to shift the time values associated with each data sample by an arbitrary amount. It does not resample the signal or change the data values in any way.
- property times#
Time vector in seconds.
- property tmax#
Last time point.
- property tmin#
First time point.
Examples using mne.DipoleFixed
#
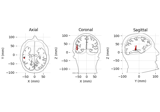
Source localization with equivalent current dipole (ECD) fit