mne.decoding.TimeDelayingRidge#
- class mne.decoding.TimeDelayingRidge(tmin, tmax, sfreq, alpha=0.0, reg_type='ridge', fit_intercept=True, n_jobs=None, edge_correction=True)[source]#
Ridge regression of data with time delays.
- Parameters:
- tmin
int
|float
The starting lag, in seconds (or samples if
sfreq
== 1). Negative values correspond to times in the past.- tmax
int
|float
The ending lag, in seconds (or samples if
sfreq
== 1). Positive values correspond to times in the future. Must be >= tmin.- sfreq
float
The sampling frequency used to convert times into samples.
- alpha
float
The ridge (or laplacian) regularization factor.
- reg_type
str
|list
Can be
"ridge"
(default) or"laplacian"
. Can also be a 2-element list specifying how to regularize in time and across adjacent features.- fit_interceptbool
If True (default), the sample mean is removed before fitting.
- n_jobs
int
|str
The number of jobs to use. Can be an int (default 1) or
'cuda'
.New in v0.18.
- edge_correctionbool
If True (default), correct the autocorrelation coefficients for non-zero delays for the fact that fewer samples are available. Disabling this speeds up performance at the cost of accuracy depending on the relationship between epoch length and model duration. Only used if
estimator
is float or None.New in v0.18.
- tmin
Methods
fit
(X, y)Estimate the coefficients of the linear model.
Get metadata routing of this object.
get_params
([deep])Get parameters for this estimator.
predict
(X)Predict the output.
score
(X, y[, sample_weight])Return coefficient of determination on test data.
set_params
(**params)Set the parameters of this estimator.
set_score_request
(*[, sample_weight])Configure whether metadata should be requested to be passed to the
score
method.See also
Notes
This class is meant to be used with
mne.decoding.ReceptiveField
by only implicitly doing the time delaying. For reasonable receptive field and input signal sizes, it should be more CPU and memory efficient by using frequency-domain methods (FFTs) to compute the auto- and cross-correlations.- fit(X, y)[source]#
Estimate the coefficients of the linear model.
- Parameters:
- Returns:
- selfinstance of
TimeDelayingRidge
Returns the modified instance.
- selfinstance of
- get_metadata_routing()[source]#
Get metadata routing of this object.
Please check User Guide on how the routing mechanism works.
- Returns:
- routing
MetadataRequest
A
MetadataRequest
encapsulating routing information.
- routing
- score(X, y, sample_weight=None)[source]#
Return coefficient of determination on test data.
The coefficient of determination, \(R^2\), is defined as \((1 - \frac{u}{v})\), where \(u\) is the residual sum of squares
((y_true - y_pred)** 2).sum()
and \(v\) is the total sum of squares((y_true - y_true.mean()) ** 2).sum()
. The best possible score is 1.0 and it can be negative (because the model can be arbitrarily worse). A constant model that always predicts the expected value of y, disregarding the input features, would get a \(R^2\) score of 0.0.- Parameters:
- Xarray_like of shape (n_samples, n_features)
Test samples. For some estimators this may be a precomputed kernel matrix or a list of generic objects instead with shape
(n_samples, n_samples_fitted)
, wheren_samples_fitted
is the number of samples used in the fitting for the estimator.- yarray_like of shape (n_samples,) or (n_samples, n_outputs)
True values for X.
- sample_weightarray_like of shape (n_samples,), default=None
Sample weights.
- Returns:
- score
float
\(R^2\) of
self.predict(X)
w.r.t. y.
- score
Notes
The \(R^2\) score used when calling
score
on a regressor usesmultioutput='uniform_average'
from version 0.23 to keep consistent with default value ofr2_score()
. This influences thescore
method of all the multioutput regressors (except forMultiOutputRegressor
).
- set_params(**params)[source]#
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.
- set_score_request(*, sample_weight: bool | None | str = '$UNCHANGED$') TimeDelayingRidge [source]#
Configure whether metadata should be requested to be passed to the
score
method.Note that this method is only relevant when this estimator is used as a sub-estimator within a meta-estimator and metadata routing is enabled with
enable_metadata_routing=True
(seesklearn.set_config()
). Please check the User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed toscore
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it toscore
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in v1.3.
Examples using mne.decoding.TimeDelayingRidge
#
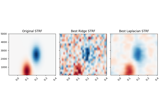
Spectro-temporal receptive field (STRF) estimation on continuous data