mne.Annotations#
- class mne.Annotations(onset, duration, description, orig_time=None, ch_names=None, *, extras=None)[source]#
Annotation object for annotating segments of raw data.
Note
To convert events to
Annotations
, useannotations_from_events
. To convert existingAnnotations
to events, useevents_from_annotations
.- Parameters:
- onset
array
offloat
, shape (n_annotations,) The starting time of annotations in seconds after
orig_time
.- duration
array
offloat
, shape (n_annotations,) |float
Durations of the annotations in seconds. If a float, all the annotations are given the same duration.
- description
array
ofstr
, shape (n_annotations,) |str
Array of strings containing description for each annotation. If a string, all the annotations are given the same description. To reject epochs, use description starting with keyword ‘bad’. See example above.
- orig_time
float
|str
|datetime
|tuple
ofint
|None
A POSIX Timestamp, datetime or a tuple containing the timestamp as the first element and microseconds as the second element. Determines the starting time of annotation acquisition. If None (default), starting time is determined from beginning of raw data acquisition. In general,
raw.info['meas_date']
(or None) can be used for syncing the annotations with raw data if their acquisition is started at the same time. If it is a string, it should conform to the ISO8601 format. More precisely to this ‘%Y-%m-%d %H:%M:%S.%f’ particular case of the ISO8601 format where the delimiter between date and time is ‘ ‘.- ch_names
list
|None
List of lists of channel names associated with the annotations. Empty entries are assumed to be associated with no specific channel, i.e., with all channels or with the time slice itself. None (default) is the same as passing all empty lists. For example, this creates three annotations, associating the first with the time interval itself, the second with two channels, and the third with a single channel:
Annotations(onset=[0, 3, 10], duration=[1, 0.25, 0.5], description=['Start', 'BAD_flux', 'BAD_noise'], ch_names=[[], ['MEG0111', 'MEG2563'], ['MEG1443']])
New in v0.23.
- extras
list
[dict
[str
,int
|float
|str
|None
] |None
] |None
Optional list of dicts containing extra fields for each annotation. The number of items must match the number of annotations.
New in v1.10.
- onset
- Attributes:
Methods
__add__
(other)Add (concatencate) two Annotation objects.
__getitem__
(key, *[, with_ch_names, with_extras])Propagate indexing and slicing to the underlying numpy structure.
__iter__
()Iterate over the annotations.
__len__
()Return the number of annotations.
append
(onset, duration, description[, ...])Add an annotated segment.
copy
()Return a copy of the Annotations.
count
()Count annotations.
crop
([tmin, tmax, emit_warning, ...])Remove all annotation that are outside of [tmin, tmax].
delete
(idx)Remove an annotation.
rename
(mapping[, verbose])Rename annotation description(s).
save
(fname, *[, overwrite, verbose])Save annotations to FIF, CSV or TXT.
set_durations
(mapping[, verbose])Set annotation duration(s).
to_data_frame
([time_format])Export annotations in tabular structure as a pandas DataFrame.
Notes
Annotations are added to instance of
mne.io.Raw
as the attributeraw.annotations
.To reject bad epochs using annotations, use annotation description starting with ‘bad’ keyword. The epochs with overlapping bad segments are then rejected automatically by default.
To remove epochs with blinks you can do:
>>> eog_events = mne.preprocessing.find_eog_events(raw) >>> n_blinks = len(eog_events) >>> onset = eog_events[:, 0] / raw.info['sfreq'] - 0.25 >>> duration = np.repeat(0.5, n_blinks) >>> description = ['bad blink'] * n_blinks >>> annotations = mne.Annotations(onset, duration, description) >>> raw.set_annotations(annotations) >>> epochs = mne.Epochs(raw, events, event_id, tmin, tmax)
ch_names
Specifying channel names allows the creation of channel-specific annotations. Once the annotations are assigned to a raw instance with
mne.io.Raw.set_annotations()
, if channels are renamed by the raw instance, the annotation channels also get renamed. If channels are dropped from the raw instance, any channel-specific annotation that has no channels left in the raw instance will also be removed.orig_time
If
orig_time
is None, the annotations are synced to the start of the data (0 seconds). Otherwise the annotations are synced to sample 0 andraw.first_samp
is taken into account the same way as with events.When setting annotations, the following alignments between
raw.info['meas_date']
andannotation.orig_time
take place:----------- meas_date=XX, orig_time=YY ----------------------------- | +------------------+ |______________| RAW | | | | | +------------------+ meas_date first_samp . . | +------+ . |_________| ANOT | . | | | . | +------+ . orig_time onset[0] . | +------+ |___________________| | | | | | +------+ orig_time onset[0]' ----------- meas_date=XX, orig_time=None --------------------------- | +------------------+ |______________| RAW | | | | | +------------------+ . N +------+ . o_________| ANOT | . n | | . e +------+ . | +------+ |________________________| | | | | | +------+ orig_time onset[0]' ----------- meas_date=None, orig_time=YY --------------------------- N +------------------+ o______________| RAW | n | | e +------------------+ | +------+ |_________| ANOT | | | | | +------+ [[[ CRASH ]]] ----------- meas_date=None, orig_time=None ------------------------- N +------------------+ o______________| RAW | n | | e +------------------+ . N +------+ . o_________| ANOT | . n | | . e +------+ . N +------+ o________________________| | n | | e +------+ orig_time onset[0]'
Warning
This means that when
raw.info['meas_date'] is None
, doingraw.set_annotations(raw.annotations)
will not alterraw
if and only ifraw.first_samp == 0
. When it’s non-zero,raw.set_annotations
will assume that the “new” annotations refer to the original data (withfirst_samp==0
), and will be re-referenced to the new time offset!Specific annotation
BAD_ACQ_SKIP
annotation leads to specific reading/writing file behaviours. Seemne.io.read_raw_fif()
andRaw.save()
notes for details.- __getitem__(key, *, with_ch_names=None, with_extras=True)[source]#
Propagate indexing and slicing to the underlying numpy structure.
- append(onset, duration, description, ch_names=None, *, extras=None)[source]#
Add an annotated segment. Operates inplace.
- Parameters:
- onset
float
| array_like Annotation time onset from the beginning of the recording in seconds.
- duration
float
| array_like Duration of the annotation in seconds.
- description
str
| array_like Description for the annotation. To reject epochs, use description starting with keyword ‘bad’.
- ch_names
list
|None
List of lists of channel names associated with the annotations. Empty entries are assumed to be associated with no specific channel, i.e., with all channels or with the time slice itself. None (default) is the same as passing all empty lists. For example, this creates three annotations, associating the first with the time interval itself, the second with two channels, and the third with a single channel:
Annotations(onset=[0, 3, 10], duration=[1, 0.25, 0.5], description=['Start', 'BAD_flux', 'BAD_noise'], ch_names=[[], ['MEG0111', 'MEG2563'], ['MEG1443']])
New in v0.23.
- extras
list
[dict
[str
,int
|float
|str
|None
] |None
] |None
Optional list of dicts containing extras fields for each annotation. The number of items must match the number of annotations.
New in v1.10.
- onset
- Returns:
- self
mne.Annotations
The modified Annotations object.
- self
Notes
The array-like support for arguments allows this to be used similarly to not only
list.append
, but also list.extend.Examples using
append
:
- copy()[source]#
Return a copy of the Annotations.
- Returns:
- instinstance of
Annotations
A copy of the object.
- instinstance of
- count()[source]#
Count annotations.
- Returns:
- counts
dict
A dictionary containing unique annotation descriptions as keys with their counts as values.
- counts
- crop(tmin=None, tmax=None, emit_warning=False, use_orig_time=True, verbose=None)[source]#
Remove all annotation that are outside of [tmin, tmax].
The method operates inplace.
- Parameters:
- tmin
float
|datetime
|None
Start time of selection in seconds.
- tmax
float
|datetime
|None
End time of selection in seconds.
- emit_warningbool
Whether to emit warnings when limiting or omitting annotations. Defaults to False.
- use_orig_timebool
Whether to use orig_time as an offset. Defaults to True.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- tmin
- Returns:
- selfinstance of
Annotations
The cropped Annotations object.
- selfinstance of
Examples using
crop
:Sleep stage classification from polysomnography (PSG) data
Sleep stage classification from polysomnography (PSG) data
- delete(idx)[source]#
Remove an annotation. Operates inplace.
- Parameters:
- idx
int
| array_like ofint
Index of the annotation to remove. Can be array-like to remove multiple indices.
- idx
- property extras#
The extras of the Annotations.
The
extras
attribute is a list of dictionaries. It can easily be converted to a pandas DataFrame using:pd.DataFrame(extras)
.
- property orig_time#
The time base of the Annotations.
- rename(mapping, verbose=None)[source]#
Rename annotation description(s). Operates inplace.
- Parameters:
- mapping
dict
A dictionary mapping the old description to a new description, e.g. {‘1.0’ : ‘Control’, ‘2.0’ : ‘Stimulus’}.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- mapping
- Returns:
- self
mne.Annotations
The modified Annotations object.
- self
Notes
New in v0.24.0.
- save(fname, *, overwrite=False, verbose=None)[source]#
Save annotations to FIF, CSV or TXT.
Typically annotations get saved in the FIF file for raw data (e.g., as
raw.annotations
), but this offers the possibility to also save them to disk separately in different file formats which are easier to share between packages.- Parameters:
- fnamepath-like
The filename to use.
- overwritebool
If True (default False), overwrite the destination file if it exists.
New in v0.23.
- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
Notes
The format of the information stored in the saved annotation objects depends on the chosen file format.
.csv
files store the onset as timestamps (e.g.,2002-12-03 19:01:56.676071
), whereas.txt
files store onset as seconds since start of the recording (e.g.,45.95597082905339
).Examples using
save
:
- set_durations(mapping, verbose=None)[source]#
Set annotation duration(s). Operates inplace.
- Parameters:
- mapping
dict
|float
A dictionary mapping the annotation description to a duration in seconds e.g.
{'ShortStimulus' : 3, 'LongStimulus' : 12}
. Alternatively, if a number is provided, then all annotations durations are set to the single provided value.- verbosebool |
str
|int
|None
Control verbosity of the logging output. If
None
, use the default verbosity level. See the logging documentation andmne.verbose()
for details. Should only be passed as a keyword argument.
- mapping
- Returns:
- self
mne.Annotations
The modified Annotations object.
- self
Notes
New in v0.24.0.
- to_data_frame(time_format='datetime')[source]#
Export annotations in tabular structure as a pandas DataFrame.
- Parameters:
- time_format
str
|None
Desired time format. If
None
, no conversion is applied, and time values remain as float values in seconds. If'ms'
, time values will be rounded to the nearest millisecond and converted to integers. If'timedelta'
, time values will be converted topandas.Timedelta
values. If'datetime'
, time values will be converted topandas.Timestamp
values, relative toraw.info['meas_date']
and offset byraw.first_samp
. Default isNone
unless specified otherwise. Default isdatetime
.New in v1.7.
- time_format
- Returns:
- result
pandas.DataFrame
Returns a pandas DataFrame with onset, duration, and description columns. A column named ch_names is added if any annotations are channel-specific.
- result
Examples using mne.Annotations
#
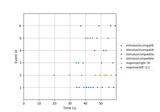
Automated epochs metadata generation with variable time windows
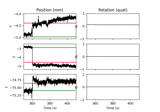
Annotate movement artifacts and reestimate dev_head_t
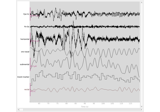
Sleep stage classification from polysomnography (PSG) data
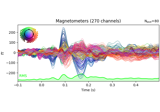
Working with CTF data: the Brainstorm auditory dataset