Note
Go to the end to download the full example code.
Compute and visualize ERDS maps#
This example calculates and displays ERDS maps of event-related EEG data. ERDS (sometimes also written as ERD/ERS) is short for event-related desynchronization (ERD) and event-related synchronization (ERS) [1]. Conceptually, ERD corresponds to a decrease in power in a specific frequency band relative to a baseline. Similarly, ERS corresponds to an increase in power. An ERDS map is a time/frequency representation of ERD/ERS over a range of frequencies [2]. ERDS maps are also known as ERSP (event-related spectral perturbation) [3].
In this example, we use an EEG BCI data set containing two different motor imagery tasks (imagined hand and feet movement). Our goal is to generate ERDS maps for each of the two tasks.
First, we load the data and create epochs of 5s length. The data set contains multiple channels, but we will only consider C3, Cz, and C4. We compute maps containing frequencies ranging from 2 to 35Hz. We map ERD to red color and ERS to blue color, which is customary in many ERDS publications. Finally, we perform cluster-based permutation tests to estimate significant ERDS values (corrected for multiple comparisons within channels).
# Authors: Clemens Brunner <clemens.brunner@gmail.com>
# Felix Klotzsche <klotzsche@cbs.mpg.de>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
As usual, we import everything we need.
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import seaborn as sns
from matplotlib.colors import TwoSlopeNorm
import mne
from mne.datasets import eegbci
from mne.io import concatenate_raws, read_raw_edf
from mne.stats import permutation_cluster_1samp_test as pcluster_test
First, we load and preprocess the data. We use runs 6, 10, and 14 from subject 1 (these runs contains hand and feet motor imagery).
fnames = eegbci.load_data(subjects=1, runs=(6, 10, 14))
raw = concatenate_raws([read_raw_edf(f, preload=True) for f in fnames])
raw.rename_channels(lambda x: x.strip(".")) # remove dots from channel names
# rename descriptions to be more easily interpretable
raw.annotations.rename(dict(T1="hands", T2="feet"))
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R06.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 19999 = 0.000 ... 124.994 secs...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R10.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 19999 = 0.000 ... 124.994 secs...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R14.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 19999 = 0.000 ... 124.994 secs...
Now we can create 5-second epochs around events of interest.
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
0 projection items activated
Using data from preloaded Raw for 45 events and 961 original time points ...
0 bad epochs dropped
Here we set suitable values for computing ERDS maps. Note especially the
cnorm
variable, which sets up an asymmetric colormap where the middle
color is mapped to zero, even though zero is not the middle value of the
colormap range. This does two things: it ensures that zero values will be
plotted in white (given that below we select the RdBu
colormap), and it
makes synchronization and desynchronization look equally prominent in the
plots, even though their extreme values are of different magnitudes.
freqs = np.arange(2, 36) # frequencies from 2-35Hz
vmin, vmax = -1, 1.5 # set min and max ERDS values in plot
baseline = (-1, 0) # baseline interval (in s)
cnorm = TwoSlopeNorm(vmin=vmin, vcenter=0, vmax=vmax) # min, center & max ERDS
kwargs = dict(
n_permutations=100, step_down_p=0.05, seed=1, buffer_size=None, out_type="mask"
) # for cluster test
Finally, we perform time/frequency decomposition over all epochs.
tfr = epochs.compute_tfr(
method="multitaper",
freqs=freqs,
n_cycles=freqs,
use_fft=True,
return_itc=False,
average=False,
decim=2,
)
tfr.crop(tmin, tmax).apply_baseline(baseline, mode="percent")
for event in event_ids:
# select desired epochs for visualization
tfr_ev = tfr[event]
fig, axes = plt.subplots(
1, 4, figsize=(12, 4), gridspec_kw={"width_ratios": [10, 10, 10, 1]}
)
for ch, ax in enumerate(axes[:-1]): # for each channel
# positive clusters
_, c1, p1, _ = pcluster_test(tfr_ev.data[:, ch], tail=1, **kwargs)
# negative clusters
_, c2, p2, _ = pcluster_test(tfr_ev.data[:, ch], tail=-1, **kwargs)
# note that we keep clusters with p <= 0.05 from the combined clusters
# of two independent tests; in this example, we do not correct for
# these two comparisons
c = np.stack(c1 + c2, axis=2) # combined clusters
p = np.concatenate((p1, p2)) # combined p-values
mask = c[..., p <= 0.05].any(axis=-1)
# plot TFR (ERDS map with masking)
tfr_ev.average().plot(
[ch],
cmap="RdBu",
cnorm=cnorm,
axes=ax,
colorbar=False,
show=False,
mask=mask,
mask_style="mask",
)
ax.set_title(epochs.ch_names[ch], fontsize=10)
ax.axvline(0, linewidth=1, color="black", linestyle=":") # event
if ch != 0:
ax.set_ylabel("")
ax.set_yticklabels("")
fig.colorbar(axes[0].images[-1], cax=axes[-1]).ax.set_yscale("linear")
fig.suptitle(f"ERDS ({event})")
plt.show()
Applying baseline correction (mode: percent)
Using a threshold of 1.724718
stat_fun(H1): min=-8.559572924066778 max=3.1802691358477366
Running initial clustering …
Found 78 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
9%|▉ | Permuting : 9/99 [00:00<00:00, 254.53it/s]
18%|█▊ | Permuting : 18/99 [00:00<00:00, 260.92it/s]
27%|██▋ | Permuting : 27/99 [00:00<00:00, 263.17it/s]
37%|███▋ | Permuting : 37/99 [00:00<00:00, 272.22it/s]
47%|████▋ | Permuting : 47/99 [00:00<00:00, 277.67it/s]
58%|█████▊ | Permuting : 57/99 [00:00<00:00, 281.34it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 290.80it/s]
79%|███████▉ | Permuting : 78/99 [00:00<00:00, 287.36it/s]
89%|████████▉ | Permuting : 88/99 [00:00<00:00, 288.65it/s]
99%|█████████▉| Permuting : 98/99 [00:00<00:00, 289.67it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 289.52it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
Using a threshold of -1.724718
stat_fun(H1): min=-8.559572924066778 max=3.1802691358477366
Running initial clustering …
Found 71 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
9%|▉ | Permuting : 9/99 [00:00<00:00, 248.09it/s]
19%|█▉ | Permuting : 19/99 [00:00<00:00, 272.25it/s]
30%|███ | Permuting : 30/99 [00:00<00:00, 290.90it/s]
40%|████ | Permuting : 40/99 [00:00<00:00, 292.58it/s]
51%|█████ | Permuting : 50/99 [00:00<00:00, 293.53it/s]
61%|██████ | Permuting : 60/99 [00:00<00:00, 291.52it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 287.61it/s]
80%|███████▉ | Permuting : 79/99 [00:00<00:00, 289.01it/s]
90%|████████▉ | Permuting : 89/99 [00:00<00:00, 290.09it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 292.57it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 291.50it/s]
Step-down-in-jumps iteration #1 found 1 cluster to exclude from subsequent iterations
0%| | Permuting : 0/99 [00:00<?, ?it/s]
10%|█ | Permuting : 10/99 [00:00<00:00, 279.73it/s]
21%|██ | Permuting : 21/99 [00:00<00:00, 303.03it/s]
32%|███▏ | Permuting : 32/99 [00:00<00:00, 311.08it/s]
42%|████▏ | Permuting : 42/99 [00:00<00:00, 307.23it/s]
54%|█████▎ | Permuting : 53/99 [00:00<00:00, 311.49it/s]
63%|██████▎ | Permuting : 62/99 [00:00<00:00, 303.19it/s]
72%|███████▏ | Permuting : 71/99 [00:00<00:00, 296.19it/s]
82%|████████▏ | Permuting : 81/99 [00:00<00:00, 296.29it/s]
93%|█████████▎| Permuting : 92/99 [00:00<00:00, 300.45it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 300.23it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 300.30it/s]
Step-down-in-jumps iteration #2 found 0 additional clusters to exclude from subsequent iterations
No baseline correction applied
Using a threshold of 1.724718
stat_fun(H1): min=-4.519473510988147 max=3.6995798232707275
Running initial clustering …
Found 83 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
11%|█ | Permuting : 11/99 [00:00<00:00, 309.91it/s]
21%|██ | Permuting : 21/99 [00:00<00:00, 303.44it/s]
30%|███ | Permuting : 30/99 [00:00<00:00, 291.00it/s]
40%|████ | Permuting : 40/99 [00:00<00:00, 292.64it/s]
52%|█████▏ | Permuting : 51/99 [00:00<00:00, 300.17it/s]
63%|██████▎ | Permuting : 62/99 [00:00<00:00, 305.20it/s]
72%|███████▏ | Permuting : 71/99 [00:00<00:00, 298.94it/s]
81%|████████ | Permuting : 80/99 [00:00<00:00, 291.20it/s]
93%|█████████▎| Permuting : 92/99 [00:00<00:00, 299.93it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 303.17it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 301.91it/s]
Step-down-in-jumps iteration #1 found 1 cluster to exclude from subsequent iterations
0%| | Permuting : 0/99 [00:00<?, ?it/s]
8%|▊ | Permuting : 8/99 [00:00<00:00, 223.96it/s]
19%|█▉ | Permuting : 19/99 [00:00<00:00, 275.09it/s]
29%|██▉ | Permuting : 29/99 [00:00<00:00, 282.69it/s]
39%|███▉ | Permuting : 39/99 [00:00<00:00, 286.54it/s]
51%|█████ | Permuting : 50/99 [00:00<00:00, 295.41it/s]
62%|██████▏ | Permuting : 61/99 [00:00<00:00, 301.33it/s]
71%|███████ | Permuting : 70/99 [00:00<00:00, 295.71it/s]
81%|████████ | Permuting : 80/99 [00:00<00:00, 292.77it/s]
92%|█████████▏| Permuting : 91/99 [00:00<00:00, 297.31it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 299.71it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 297.83it/s]
Step-down-in-jumps iteration #2 found 0 additional clusters to exclude from subsequent iterations
Using a threshold of -1.724718
stat_fun(H1): min=-4.519473510988147 max=3.6995798232707275
Running initial clustering …
Found 56 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
9%|▉ | Permuting : 9/99 [00:00<00:00, 245.32it/s]
20%|██ | Permuting : 20/99 [00:00<00:00, 285.37it/s]
29%|██▉ | Permuting : 29/99 [00:00<00:00, 279.28it/s]
39%|███▉ | Permuting : 39/99 [00:00<00:00, 283.93it/s]
49%|████▉ | Permuting : 49/99 [00:00<00:00, 282.47it/s]
60%|█████▉ | Permuting : 59/99 [00:00<00:00, 285.16it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 287.10it/s]
79%|███████▉ | Permuting : 78/99 [00:00<00:00, 284.24it/s]
90%|████████▉ | Permuting : 89/99 [00:00<00:00, 289.93it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 293.09it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 291.10it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
No baseline correction applied
Using a threshold of 1.724718
stat_fun(H1): min=-6.4592553718811 max=3.334719139698971
Running initial clustering …
Found 61 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
8%|▊ | Permuting : 8/99 [00:00<00:00, 228.59it/s]
18%|█▊ | Permuting : 18/99 [00:00<00:00, 263.02it/s]
28%|██▊ | Permuting : 28/99 [00:00<00:00, 274.85it/s]
38%|███▊ | Permuting : 38/99 [00:00<00:00, 280.79it/s]
47%|████▋ | Permuting : 47/99 [00:00<00:00, 277.83it/s]
60%|█████▉ | Permuting : 59/99 [00:00<00:00, 288.28it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 289.73it/s]
80%|███████▉ | Permuting : 79/99 [00:00<00:00, 290.82it/s]
91%|█████████ | Permuting : 90/99 [00:00<00:00, 295.62it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 295.93it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 293.44it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
Using a threshold of -1.724718
stat_fun(H1): min=-6.4592553718811 max=3.334719139698971
Running initial clustering …
Found 64 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
11%|█ | Permuting : 11/99 [00:00<00:00, 307.65it/s]
21%|██ | Permuting : 21/99 [00:00<00:00, 302.37it/s]
32%|███▏ | Permuting : 32/99 [00:00<00:00, 310.85it/s]
42%|████▏ | Permuting : 42/99 [00:00<00:00, 307.21it/s]
51%|█████ | Permuting : 50/99 [00:00<00:00, 291.97it/s]
61%|██████ | Permuting : 60/99 [00:00<00:00, 292.89it/s]
71%|███████ | Permuting : 70/99 [00:00<00:00, 293.59it/s]
81%|████████ | Permuting : 80/99 [00:00<00:00, 294.14it/s]
90%|████████▉ | Permuting : 89/99 [00:00<00:00, 288.54it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 291.86it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 292.41it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
No baseline correction applied
Using a threshold of 1.713872
stat_fun(H1): min=-3.6760366758717375 max=3.3443493979849728
Running initial clustering …
Found 70 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
10%|█ | Permuting : 10/99 [00:00<00:00, 273.75it/s]
19%|█▉ | Permuting : 19/99 [00:00<00:00, 270.51it/s]
27%|██▋ | Permuting : 27/99 [00:00<00:00, 259.03it/s]
34%|███▍ | Permuting : 34/99 [00:00<00:00, 245.52it/s]
43%|████▎ | Permuting : 43/99 [00:00<00:00, 250.28it/s]
53%|█████▎ | Permuting : 52/99 [00:00<00:00, 253.47it/s]
62%|██████▏ | Permuting : 61/99 [00:00<00:00, 255.75it/s]
71%|███████ | Permuting : 70/99 [00:00<00:00, 257.46it/s]
80%|███████▉ | Permuting : 79/99 [00:00<00:00, 258.76it/s]
89%|████████▉ | Permuting : 88/99 [00:00<00:00, 259.81it/s]
99%|█████████▉| Permuting : 98/99 [00:00<00:00, 263.01it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 262.55it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
Using a threshold of -1.713872
stat_fun(H1): min=-3.6760366758717375 max=3.3443493979849728
Running initial clustering …
Found 77 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
5%|▌ | Permuting : 5/99 [00:00<00:00, 137.37it/s]
13%|█▎ | Permuting : 13/99 [00:00<00:00, 186.83it/s]
21%|██ | Permuting : 21/99 [00:00<00:00, 204.22it/s]
28%|██▊ | Permuting : 28/99 [00:00<00:00, 205.14it/s]
34%|███▍ | Permuting : 34/99 [00:00<00:00, 199.22it/s]
40%|████ | Permuting : 40/99 [00:00<00:00, 195.20it/s]
46%|████▋ | Permuting : 46/99 [00:00<00:00, 192.36it/s]
55%|█████▍ | Permuting : 54/99 [00:00<00:00, 199.00it/s]
63%|██████▎ | Permuting : 62/99 [00:00<00:00, 202.20it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 202.91it/s]
76%|███████▌ | Permuting : 75/99 [00:00<00:00, 200.02it/s]
83%|████████▎ | Permuting : 82/99 [00:00<00:00, 200.84it/s]
90%|████████▉ | Permuting : 89/99 [00:00<00:00, 201.55it/s]
96%|█████████▌| Permuting : 95/99 [00:00<00:00, 199.26it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 200.99it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 200.29it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
No baseline correction applied
Using a threshold of 1.713872
stat_fun(H1): min=-4.956834187075391 max=5.467989936693932
Running initial clustering …
Found 100 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
8%|▊ | Permuting : 8/99 [00:00<00:00, 225.70it/s]
17%|█▋ | Permuting : 17/99 [00:00<00:00, 246.47it/s]
26%|██▋ | Permuting : 26/99 [00:00<00:00, 253.69it/s]
33%|███▎ | Permuting : 33/99 [00:00<00:00, 241.50it/s]
41%|████▏ | Permuting : 41/99 [00:00<00:00, 240.68it/s]
49%|████▉ | Permuting : 49/99 [00:00<00:00, 240.04it/s]
60%|█████▉ | Permuting : 59/99 [00:00<00:00, 247.06it/s]
68%|██████▊ | Permuting : 67/99 [00:00<00:00, 245.66it/s]
77%|███████▋ | Permuting : 76/99 [00:00<00:00, 248.56it/s]
84%|████████▍ | Permuting : 83/99 [00:00<00:00, 243.56it/s]
92%|█████████▏| Permuting : 91/99 [00:00<00:00, 242.88it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 245.55it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 245.12it/s]
Step-down-in-jumps iteration #1 found 1 cluster to exclude from subsequent iterations
0%| | Permuting : 0/99 [00:00<?, ?it/s]
8%|▊ | Permuting : 8/99 [00:00<00:00, 221.29it/s]
17%|█▋ | Permuting : 17/99 [00:00<00:00, 243.96it/s]
25%|██▌ | Permuting : 25/99 [00:00<00:00, 241.79it/s]
34%|███▍ | Permuting : 34/99 [00:00<00:00, 248.65it/s]
42%|████▏ | Permuting : 42/99 [00:00<00:00, 246.23it/s]
52%|█████▏ | Permuting : 51/99 [00:00<00:00, 250.23it/s]
61%|██████ | Permuting : 60/99 [00:00<00:00, 253.06it/s]
70%|██████▉ | Permuting : 69/99 [00:00<00:00, 255.21it/s]
78%|███████▊ | Permuting : 77/99 [00:00<00:00, 252.86it/s]
87%|████████▋ | Permuting : 86/99 [00:00<00:00, 253.60it/s]
96%|█████████▌| Permuting : 95/99 [00:00<00:00, 253.12it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 255.02it/s]
Step-down-in-jumps iteration #2 found 0 additional clusters to exclude from subsequent iterations
Using a threshold of -1.713872
stat_fun(H1): min=-4.956834187075391 max=5.467989936693932
Running initial clustering …
Found 66 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
9%|▉ | Permuting : 9/99 [00:00<00:00, 249.03it/s]
19%|█▉ | Permuting : 19/99 [00:00<00:00, 272.82it/s]
30%|███ | Permuting : 30/99 [00:00<00:00, 291.39it/s]
40%|████ | Permuting : 40/99 [00:00<00:00, 292.98it/s]
48%|████▊ | Permuting : 48/99 [00:00<00:00, 280.88it/s]
58%|█████▊ | Permuting : 57/99 [00:00<00:00, 278.33it/s]
67%|██████▋ | Permuting : 66/99 [00:00<00:00, 276.48it/s]
75%|███████▍ | Permuting : 74/99 [00:00<00:00, 270.68it/s]
83%|████████▎ | Permuting : 82/99 [00:00<00:00, 266.25it/s]
93%|█████████▎| Permuting : 92/99 [00:00<00:00, 268.12it/s]
99%|█████████▉| Permuting : 98/99 [00:00<00:00, 255.77it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 261.08it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
No baseline correction applied
Using a threshold of 1.713872
stat_fun(H1): min=-5.954856152984689 max=4.091828652270905
Running initial clustering …
Found 93 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
8%|▊ | Permuting : 8/99 [00:00<00:00, 221.04it/s]
17%|█▋ | Permuting : 17/99 [00:00<00:00, 243.80it/s]
27%|██▋ | Permuting : 27/99 [00:00<00:00, 262.09it/s]
36%|███▋ | Permuting : 36/99 [00:00<00:00, 263.49it/s]
45%|████▌ | Permuting : 45/99 [00:00<00:00, 264.35it/s]
56%|█████▌ | Permuting : 55/99 [00:00<00:00, 270.43it/s]
64%|██████▎ | Permuting : 63/99 [00:00<00:00, 265.07it/s]
74%|███████▎ | Permuting : 73/99 [00:00<00:00, 269.82it/s]
83%|████████▎ | Permuting : 82/99 [00:00<00:00, 269.51it/s]
90%|████████▉ | Permuting : 89/99 [00:00<00:00, 259.92it/s]
96%|█████████▌| Permuting : 95/99 [00:00<00:00, 250.57it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 256.26it/s]
Step-down-in-jumps iteration #1 found 1 cluster to exclude from subsequent iterations
0%| | Permuting : 0/99 [00:00<?, ?it/s]
4%|▍ | Permuting : 4/99 [00:00<00:00, 110.41it/s]
14%|█▍ | Permuting : 14/99 [00:00<00:00, 197.43it/s]
24%|██▍ | Permuting : 24/99 [00:00<00:00, 231.01it/s]
32%|███▏ | Permuting : 32/99 [00:00<00:00, 232.75it/s]
41%|████▏ | Permuting : 41/99 [00:00<00:00, 240.28it/s]
48%|████▊ | Permuting : 48/99 [00:00<00:00, 229.99it/s]
59%|█████▊ | Permuting : 58/99 [00:00<00:00, 240.80it/s]
68%|██████▊ | Permuting : 67/99 [00:00<00:00, 244.65it/s]
78%|███████▊ | Permuting : 77/99 [00:00<00:00, 251.60it/s]
88%|████████▊ | Permuting : 87/99 [00:00<00:00, 257.17it/s]
97%|█████████▋| Permuting : 96/99 [00:00<00:00, 258.36it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 256.62it/s]
Step-down-in-jumps iteration #2 found 0 additional clusters to exclude from subsequent iterations
Using a threshold of -1.713872
stat_fun(H1): min=-5.954856152984689 max=4.091828652270905
Running initial clustering …
Found 53 clusters
0%| | Permuting : 0/99 [00:00<?, ?it/s]
7%|▋ | Permuting : 7/99 [00:00<00:00, 198.17it/s]
16%|█▌ | Permuting : 16/99 [00:00<00:00, 232.74it/s]
19%|█▉ | Permuting : 19/99 [00:00<00:00, 183.17it/s]
26%|██▋ | Permuting : 26/99 [00:00<00:00, 189.74it/s]
36%|███▋ | Permuting : 36/99 [00:00<00:00, 213.30it/s]
46%|████▋ | Permuting : 46/99 [00:00<00:00, 229.03it/s]
57%|█████▋ | Permuting : 56/99 [00:00<00:00, 237.32it/s]
62%|██████▏ | Permuting : 61/99 [00:00<00:00, 224.36it/s]
68%|██████▊ | Permuting : 67/99 [00:00<00:00, 218.21it/s]
77%|███████▋ | Permuting : 76/99 [00:00<00:00, 224.29it/s]
86%|████████▌ | Permuting : 85/99 [00:00<00:00, 229.23it/s]
95%|█████████▍| Permuting : 94/99 [00:00<00:00, 233.34it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 237.03it/s]
100%|██████████| Permuting : 99/99 [00:00<00:00, 233.04it/s]
Step-down-in-jumps iteration #1 found 0 clusters to exclude from subsequent iterations
No baseline correction applied
Similar to Epochs
objects, we can also export data from
EpochsTFR
and AverageTFR
objects
to a Pandas DataFrame
. By default, the time
column of the exported data frame is in milliseconds. Here, to be consistent
with the time-frequency plots, we want to keep it in seconds, which we can
achieve by setting time_format=None
:
df = tfr.to_data_frame(time_format=None)
df.head()
This allows us to use additional plotting functions like
seaborn.lineplot()
to plot confidence bands:
df = tfr.to_data_frame(time_format=None, long_format=True)
# Map to frequency bands:
freq_bounds = {"_": 0, "delta": 3, "theta": 7, "alpha": 13, "beta": 35, "gamma": 140}
df["band"] = pd.cut(
df["freq"], list(freq_bounds.values()), labels=list(freq_bounds)[1:]
)
# Filter to retain only relevant frequency bands:
freq_bands_of_interest = ["delta", "theta", "alpha", "beta"]
df = df[df.band.isin(freq_bands_of_interest)]
df["band"] = df["band"].cat.remove_unused_categories()
# Order channels for plotting:
df["channel"] = df["channel"].cat.reorder_categories(("C3", "Cz", "C4"), ordered=True)
g = sns.FacetGrid(df, row="band", col="channel", margin_titles=True)
g.map(sns.lineplot, "time", "value", "condition", n_boot=10)
axline_kw = dict(color="black", linestyle="dashed", linewidth=0.5, alpha=0.5)
g.map(plt.axhline, y=0, **axline_kw)
g.map(plt.axvline, x=0, **axline_kw)
g.set(ylim=(None, 1.5))
g.set_axis_labels("Time (s)", "ERDS")
g.set_titles(col_template="{col_name}", row_template="{row_name}")
g.add_legend(ncol=2, loc="lower center")
g.fig.subplots_adjust(left=0.1, right=0.9, top=0.9, bottom=0.08)
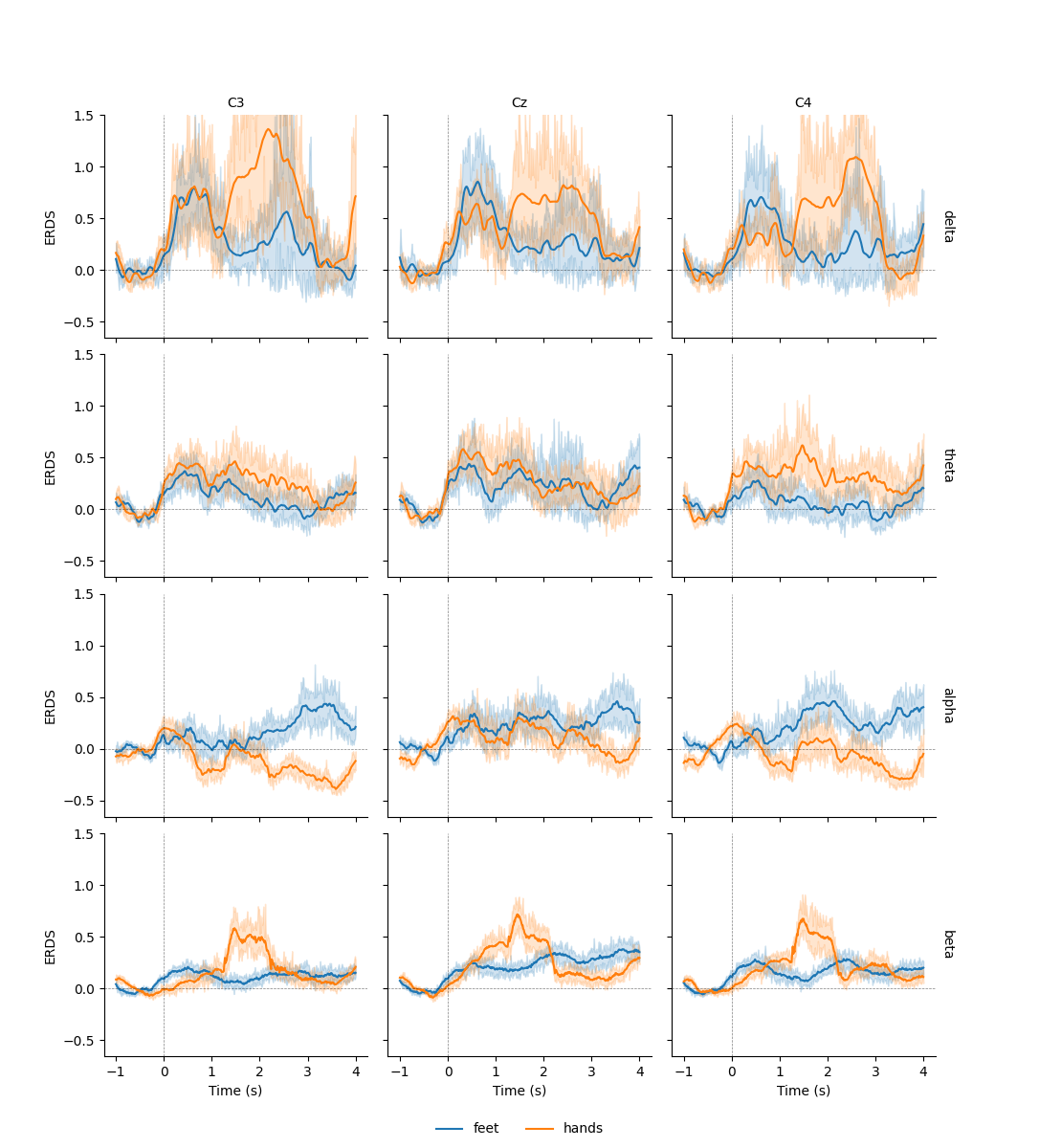
Converting "condition" to "category"...
Converting "epoch" to "category"...
Converting "channel" to "category"...
Converting "ch_type" to "category"...
Having the data as a DataFrame also facilitates subsetting, grouping, and other transforms. Here, we use seaborn to plot the average ERDS in the motor imagery interval as a function of frequency band and imagery condition:
df_mean = (
df.query("time > 1")
.groupby(["condition", "epoch", "band", "channel"], observed=False)[["value"]]
.mean()
.reset_index()
)
g = sns.FacetGrid(
df_mean, col="condition", col_order=["hands", "feet"], margin_titles=True
)
g = g.map(
sns.violinplot,
"channel",
"value",
"band",
cut=0,
palette="deep",
order=["C3", "Cz", "C4"],
hue_order=freq_bands_of_interest,
linewidth=0.5,
).add_legend(ncol=4, loc="lower center")
g.map(plt.axhline, **axline_kw)
g.set_axis_labels("", "ERDS")
g.set_titles(col_template="{col_name}", row_template="{row_name}")
g.fig.subplots_adjust(left=0.1, right=0.9, top=0.9, bottom=0.3)
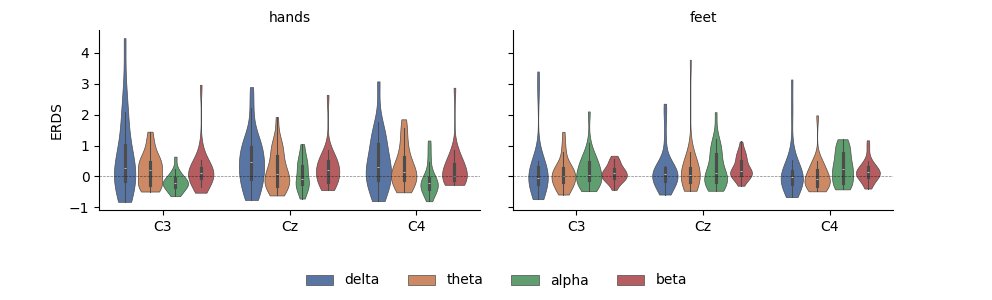
References#
Total running time of the script: (0 minutes 29.080 seconds)