Note
Go to the end to download the full example code.
Extracting and visualizing subject head movement#
Continuous head movement can be encoded during MEG recordings by use of HPI coils that continuously emit sinusoidal signals. These signals can then be extracted from the recording and used to estimate head position as a function of time. Here we show an example of how to do this, and how to visualize the result.
HPI frequencies#
First let’s load a short bit of raw data where the subject intentionally moved their head during the recording. Its power spectral density shows five peaks (most clearly visible in the gradiometers) corresponding to the HPI coil frequencies, plus other peaks related to power line interference (60 Hz and harmonics).
# Authors: Eric Larson <larson.eric.d@gmail.com>
# Richard Höchenberger <richard.hoechenberger@gmail.com>
# Daniel McCloy <dan@mccloy.info>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
from os import path as op
import mne
data_path = op.join(mne.datasets.testing.data_path(verbose=True), "SSS")
fname_raw = op.join(data_path, "test_move_anon_raw.fif")
raw = mne.io.read_raw_fif(fname_raw, allow_maxshield="yes").load_data()
raw.compute_psd().plot(picks="data", exclude="bads", amplitude=False)
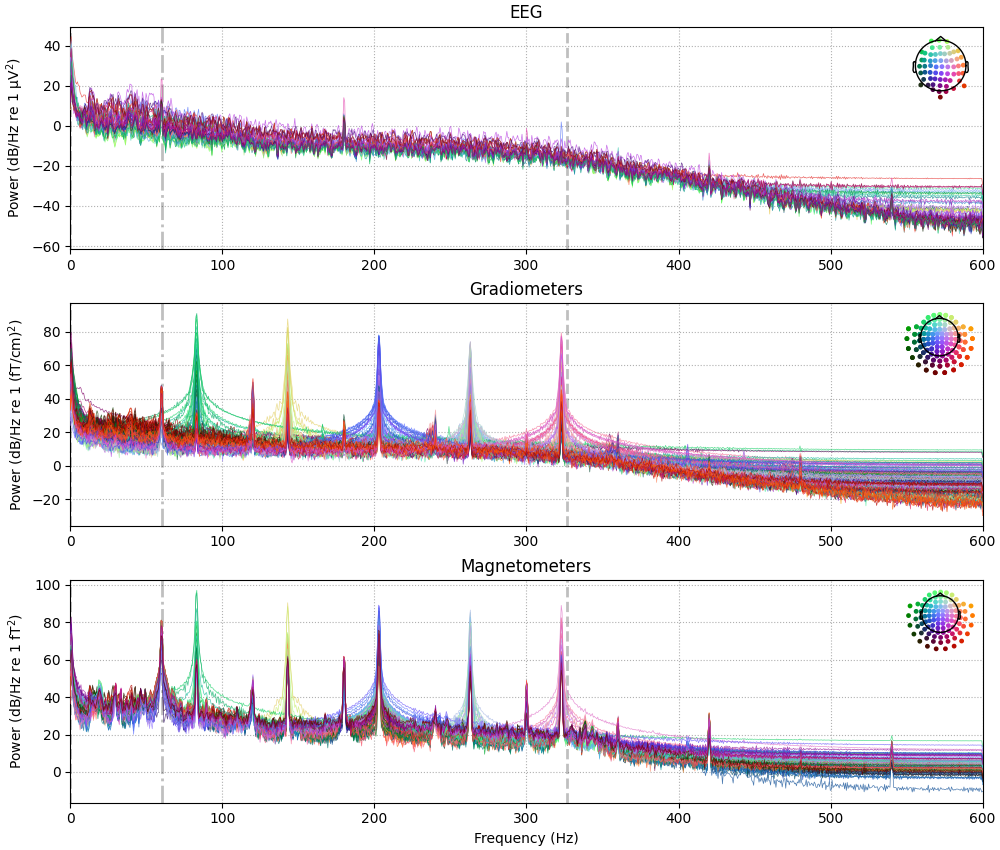
Opening raw data file /home/circleci/mne_data/MNE-testing-data/SSS/test_move_anon_raw.fif...
Read a total of 12 projection items:
mag.fif : PCA-v1 (1 x 306) idle
mag.fif : PCA-v2 (1 x 306) idle
mag.fif : PCA-v3 (1 x 306) idle
mag.fif : PCA-v4 (1 x 306) idle
mag.fif : PCA-v5 (1 x 306) idle
mag.fif : PCA-v6 (1 x 306) idle
mag.fif : PCA-v7 (1 x 306) idle
grad.fif : PCA-v1 (1 x 306) idle
grad.fif : PCA-v2 (1 x 306) idle
grad.fif : PCA-v3 (1 x 306) idle
grad.fif : PCA-v4 (1 x 306) idle
Average EEG reference (1 x 60) idle
Range : 10800 ... 31199 = 9.000 ... 25.999 secs
Ready.
Reading 0 ... 20399 = 0.000 ... 16.999 secs...
Effective window size : 1.707 (s)
Plotting power spectral density (dB=True).
We can use mne.chpi.get_chpi_info
to retrieve the coil frequencies,
the index of the channel indicating when which coil was switched on, and the
respective “event codes” associated with each coil’s activity.
chpi_freqs, ch_idx, chpi_codes = mne.chpi.get_chpi_info(info=raw.info)
print(f"cHPI coil frequencies extracted from raw: {chpi_freqs} Hz")
Using 5 HPI coils: 83 143 203 263 323 Hz
cHPI coil frequencies extracted from raw: [ 83. 143. 203. 263. 323.] Hz
Estimating continuous head position#
First, let’s extract the HPI coil amplitudes as a function of time:
Using 5 HPI coils: 83 143 203 263 323 Hz
Line interference frequencies: 60.0 120.0 180.0 240.0 300.0 360.0 Hz
Using time window: 83.3 ms
Fitting 5 HPI coil locations at up to 1696 time points (17.0 s duration)
0%| | cHPI amplitudes : 0/1696 [00:00<?, ?it/s]
0%| | cHPI amplitudes : 1/1696 [00:03<1:43:08, 3.65s/it]
1%| | cHPI amplitudes : 15/1696 [00:03<06:31, 4.29it/s]
2%|▏ | cHPI amplitudes : 30/1696 [00:03<03:09, 8.78it/s]
3%|▎ | cHPI amplitudes : 45/1696 [00:03<02:02, 13.45it/s]
4%|▎ | cHPI amplitudes : 60/1696 [00:03<01:29, 18.32it/s]
4%|▍ | cHPI amplitudes : 75/1696 [00:03<01:09, 23.38it/s]
5%|▌ | cHPI amplitudes : 90/1696 [00:03<00:56, 28.65it/s]
6%|▌ | cHPI amplitudes : 105/1696 [00:03<00:46, 34.12it/s]
7%|▋ | cHPI amplitudes : 120/1696 [00:03<00:39, 39.81it/s]
8%|▊ | cHPI amplitudes : 135/1696 [00:03<00:34, 45.72it/s]
9%|▉ | cHPI amplitudes : 149/1696 [00:03<00:30, 51.44it/s]
10%|▉ | cHPI amplitudes : 164/1696 [00:03<00:26, 57.80it/s]
11%|█ | cHPI amplitudes : 179/1696 [00:03<00:23, 64.40it/s]
11%|█▏ | cHPI amplitudes : 194/1696 [00:03<00:21, 71.23it/s]
12%|█▏ | cHPI amplitudes : 209/1696 [00:03<00:18, 78.30it/s]
13%|█▎ | cHPI amplitudes : 224/1696 [00:03<00:17, 85.61it/s]
14%|█▍ | cHPI amplitudes : 239/1696 [00:03<00:15, 93.16it/s]
15%|█▍ | cHPI amplitudes : 254/1696 [00:03<00:14, 100.96it/s]
16%|█▌ | cHPI amplitudes : 269/1696 [00:03<00:13, 109.00it/s]
17%|█▋ | cHPI amplitudes : 284/1696 [00:03<00:12, 117.31it/s]
18%|█▊ | cHPI amplitudes : 299/1696 [00:03<00:11, 125.84it/s]
19%|█▊ | cHPI amplitudes : 314/1696 [00:04<00:10, 134.63it/s]
19%|█▉ | cHPI amplitudes : 329/1696 [00:04<00:09, 143.68it/s]
20%|██ | cHPI amplitudes : 344/1696 [00:04<00:08, 152.98it/s]
21%|██ | cHPI amplitudes : 359/1696 [00:04<00:08, 162.49it/s]
22%|██▏ | cHPI amplitudes : 373/1696 [00:04<00:07, 171.56it/s]
23%|██▎ | cHPI amplitudes : 388/1696 [00:04<00:07, 181.58it/s]
24%|██▍ | cHPI amplitudes : 403/1696 [00:04<00:06, 191.82it/s]
25%|██▍ | cHPI amplitudes : 418/1696 [00:04<00:06, 202.35it/s]
26%|██▌ | cHPI amplitudes : 433/1696 [00:04<00:05, 212.99it/s]
26%|██▋ | cHPI amplitudes : 448/1696 [00:04<00:05, 223.93it/s]
27%|██▋ | cHPI amplitudes : 463/1696 [00:04<00:05, 235.06it/s]
28%|██▊ | cHPI amplitudes : 478/1696 [00:04<00:04, 246.35it/s]
29%|██▉ | cHPI amplitudes : 493/1696 [00:04<00:04, 257.81it/s]
30%|██▉ | cHPI amplitudes : 508/1696 [00:04<00:04, 269.43it/s]
31%|███ | cHPI amplitudes : 523/1696 [00:04<00:04, 281.22it/s]
32%|███▏ | cHPI amplitudes : 538/1696 [00:04<00:03, 293.17it/s]
33%|███▎ | cHPI amplitudes : 553/1696 [00:04<00:03, 305.31it/s]
33%|███▎ | cHPI amplitudes : 568/1696 [00:04<00:03, 317.59it/s]
34%|███▍ | cHPI amplitudes : 583/1696 [00:04<00:03, 329.93it/s]
35%|███▌ | cHPI amplitudes : 598/1696 [00:04<00:03, 342.35it/s]
36%|███▌ | cHPI amplitudes : 613/1696 [00:04<00:03, 354.75it/s]
37%|███▋ | cHPI amplitudes : 628/1696 [00:04<00:02, 367.27it/s]
38%|███▊ | cHPI amplitudes : 643/1696 [00:04<00:02, 379.91it/s]
39%|███▉ | cHPI amplitudes : 658/1696 [00:04<00:02, 392.61it/s]
40%|███▉ | cHPI amplitudes : 673/1696 [00:04<00:02, 405.19it/s]
41%|████ | cHPI amplitudes : 688/1696 [00:04<00:02, 417.66it/s]
41%|████▏ | cHPI amplitudes : 703/1696 [00:04<00:02, 430.30it/s]
42%|████▏ | cHPI amplitudes : 717/1696 [00:04<00:02, 441.60it/s]
43%|████▎ | cHPI amplitudes : 732/1696 [00:04<00:02, 454.05it/s]
44%|████▍ | cHPI amplitudes : 747/1696 [00:04<00:02, 466.43it/s]
45%|████▍ | cHPI amplitudes : 762/1696 [00:04<00:01, 478.79it/s]
46%|████▌ | cHPI amplitudes : 777/1696 [00:04<00:01, 491.27it/s]
47%|████▋ | cHPI amplitudes : 792/1696 [00:04<00:01, 503.43it/s]
48%|████▊ | cHPI amplitudes : 807/1696 [00:04<00:01, 515.50it/s]
48%|████▊ | cHPI amplitudes : 822/1696 [00:04<00:01, 527.46it/s]
49%|████▉ | cHPI amplitudes : 837/1696 [00:04<00:01, 539.05it/s]
50%|█████ | cHPI amplitudes : 852/1696 [00:04<00:01, 550.58it/s]
51%|█████ | cHPI amplitudes : 867/1696 [00:04<00:01, 561.92it/s]
52%|█████▏ | cHPI amplitudes : 882/1696 [00:04<00:01, 573.07it/s]
53%|█████▎ | cHPI amplitudes : 897/1696 [00:04<00:01, 584.09it/s]
54%|█████▍ | cHPI amplitudes : 912/1696 [00:04<00:01, 594.82it/s]
55%|█████▍ | cHPI amplitudes : 927/1696 [00:04<00:01, 605.29it/s]
56%|█████▌ | cHPI amplitudes : 942/1696 [00:04<00:01, 615.54it/s]
56%|█████▋ | cHPI amplitudes : 957/1696 [00:04<00:01, 625.68it/s]
57%|█████▋ | cHPI amplitudes : 972/1696 [00:04<00:01, 635.63it/s]
58%|█████▊ | cHPI amplitudes : 987/1696 [00:04<00:01, 645.08it/s]
59%|█████▉ | cHPI amplitudes : 1002/1696 [00:04<00:01, 654.62it/s]
60%|█████▉ | cHPI amplitudes : 1017/1696 [00:04<00:01, 664.78it/s]
61%|██████ | cHPI amplitudes : 1032/1696 [00:04<00:00, 674.04it/s]
62%|██████▏ | cHPI amplitudes : 1047/1696 [00:04<00:00, 682.78it/s]
63%|██████▎ | cHPI amplitudes : 1062/1696 [00:04<00:00, 691.46it/s]
64%|██████▎ | cHPI amplitudes : 1078/1696 [00:04<00:00, 703.06it/s]
65%|██████▍ | cHPI amplitudes : 1100/1696 [00:04<00:00, 727.79it/s]
66%|██████▌ | cHPI amplitudes : 1122/1696 [00:04<00:00, 751.96it/s]
67%|██████▋ | cHPI amplitudes : 1144/1696 [00:04<00:00, 775.40it/s]
69%|██████▉ | cHPI amplitudes : 1166/1696 [00:04<00:00, 798.06it/s]
70%|███████ | cHPI amplitudes : 1188/1696 [00:04<00:00, 820.03it/s]
71%|███████▏ | cHPI amplitudes : 1210/1696 [00:04<00:00, 841.20it/s]
73%|███████▎ | cHPI amplitudes : 1232/1696 [00:04<00:00, 861.46it/s]
74%|███████▍ | cHPI amplitudes : 1254/1696 [00:04<00:00, 881.11it/s]
75%|███████▌ | cHPI amplitudes : 1276/1696 [00:05<00:00, 900.11it/s]
77%|███████▋ | cHPI amplitudes : 1298/1696 [00:05<00:00, 918.31it/s]
78%|███████▊ | cHPI amplitudes : 1320/1696 [00:05<00:00, 936.01it/s]
79%|███████▉ | cHPI amplitudes : 1342/1696 [00:05<00:00, 952.90it/s]
80%|████████ | cHPI amplitudes : 1364/1696 [00:05<00:00, 969.17it/s]
82%|████████▏ | cHPI amplitudes : 1386/1696 [00:05<00:00, 984.93it/s]
83%|████████▎ | cHPI amplitudes : 1407/1696 [00:05<00:00, 998.87it/s]
84%|████████▍ | cHPI amplitudes : 1429/1696 [00:05<00:00, 1013.39it/s]
86%|████████▌ | cHPI amplitudes : 1451/1696 [00:05<00:00, 1027.05it/s]
87%|████████▋ | cHPI amplitudes : 1473/1696 [00:05<00:00, 1040.41it/s]
88%|████████▊ | cHPI amplitudes : 1494/1696 [00:05<00:00, 1052.15it/s]
89%|████████▉ | cHPI amplitudes : 1515/1696 [00:05<00:00, 1063.60it/s]
91%|█████████ | cHPI amplitudes : 1537/1696 [00:05<00:00, 1075.23it/s]
92%|█████████▏| cHPI amplitudes : 1559/1696 [00:05<00:00, 1086.53it/s]
93%|█████████▎| cHPI amplitudes : 1580/1696 [00:05<00:00, 1096.38it/s]
94%|█████████▍| cHPI amplitudes : 1602/1696 [00:05<00:00, 1106.78it/s]
96%|█████████▌| cHPI amplitudes : 1624/1696 [00:05<00:00, 1116.83it/s]
97%|█████████▋| cHPI amplitudes : 1646/1696 [00:05<00:00, 1126.22it/s]
98%|█████████▊| cHPI amplitudes : 1667/1696 [00:05<00:00, 1134.53it/s]
100%|█████████▉| cHPI amplitudes : 1689/1696 [00:05<00:00, 1143.43it/s]
100%|██████████| cHPI amplitudes : 1696/1696 [00:05<00:00, 318.33it/s]
Second, let’s compute time-varying HPI coil locations from these:
Computing 4385 HPI location guesses (1 cm grid in a 10.7 cm sphere)
HPIFIT: 5 coils digitized in order 5 1 4 3 2
HPI consistency of isotrak and hpifit is OK.
0%| | cHPI locations : 0/1696 [00:00<?, ?it/s]
0%| | cHPI locations : 1/1696 [00:00<17:23, 1.62it/s]
4%|▍ | cHPI locations : 64/1696 [00:00<00:15, 106.44it/s]
6%|▌ | cHPI locations : 101/1696 [00:00<00:10, 153.80it/s]
10%|▉ | cHPI locations : 165/1696 [00:00<00:06, 253.06it/s]
12%|█▏ | cHPI locations : 201/1696 [00:00<00:05, 281.54it/s]
14%|█▍ | cHPI locations : 238/1696 [00:00<00:04, 332.18it/s]
16%|█▌ | cHPI locations : 273/1696 [00:00<00:03, 379.66it/s]
18%|█▊ | cHPI locations : 301/1696 [00:00<00:03, 371.60it/s]
20%|█▉ | cHPI locations : 334/1696 [00:00<00:03, 412.34it/s]
22%|██▏ | cHPI locations : 370/1696 [00:00<00:02, 457.63it/s]
24%|██▎ | cHPI locations : 401/1696 [00:01<00:02, 440.66it/s]
26%|██▌ | cHPI locations : 435/1696 [00:01<00:02, 479.72it/s]
28%|██▊ | cHPI locations : 470/1696 [00:01<00:02, 520.27it/s]
30%|██▉ | cHPI locations : 501/1696 [00:01<00:02, 494.96it/s]
32%|███▏ | cHPI locations : 536/1696 [00:01<00:02, 532.90it/s]
33%|███▎ | cHPI locations : 559/1696 [00:01<00:02, 481.35it/s]
33%|███▎ | cHPI locations : 568/1696 [00:01<00:02, 432.85it/s]
34%|███▍ | cHPI locations : 573/1696 [00:01<00:02, 387.71it/s]
34%|███▍ | cHPI locations : 577/1696 [00:01<00:03, 351.55it/s]
34%|███▍ | cHPI locations : 581/1696 [00:01<00:03, 317.71it/s]
35%|███▍ | cHPI locations : 587/1696 [00:01<00:03, 293.66it/s]
35%|███▌ | cHPI locations : 600/1696 [00:01<00:03, 277.31it/s]
38%|███▊ | cHPI locations : 647/1696 [00:01<00:03, 315.72it/s]
41%|████ | cHPI locations : 695/1696 [00:01<00:02, 355.81it/s]
41%|████▏ | cHPI locations : 700/1696 [00:02<00:03, 326.55it/s]
44%|████▍ | cHPI locations : 747/1696 [00:02<00:02, 365.08it/s]
47%|████▋ | cHPI locations : 789/1696 [00:02<00:02, 365.02it/s]
47%|████▋ | cHPI locations : 794/1696 [00:02<00:02, 337.21it/s]
47%|████▋ | cHPI locations : 800/1696 [00:02<00:02, 313.66it/s]
47%|████▋ | cHPI locations : 805/1696 [00:02<00:03, 291.00it/s]
48%|████▊ | cHPI locations : 810/1696 [00:02<00:03, 271.02it/s]
48%|████▊ | cHPI locations : 815/1696 [00:02<00:03, 253.12it/s]
48%|████▊ | cHPI locations : 819/1696 [00:02<00:03, 236.24it/s]
49%|████▊ | cHPI locations : 823/1696 [00:02<00:03, 227.20it/s]
49%|████▉ | cHPI locations : 827/1696 [00:02<00:03, 219.13it/s]
49%|████▉ | cHPI locations : 833/1696 [00:03<00:04, 212.42it/s]
50%|█████ | cHPI locations : 848/1696 [00:03<00:03, 212.87it/s]
54%|█████▍ | cHPI locations : 912/1696 [00:03<00:03, 257.70it/s]
56%|█████▌ | cHPI locations : 948/1696 [00:03<00:02, 269.77it/s]
60%|█████▉ | cHPI locations : 1012/1696 [00:03<00:02, 315.38it/s]
61%|██████▏ | cHPI locations : 1040/1696 [00:03<00:02, 319.76it/s]
62%|██████▏ | cHPI locations : 1051/1696 [00:03<00:02, 312.30it/s]
63%|██████▎ | cHPI locations : 1062/1696 [00:03<00:02, 304.62it/s]
63%|██████▎ | cHPI locations : 1072/1696 [00:03<00:02, 296.87it/s]
64%|██████▍ | cHPI locations : 1086/1696 [00:03<00:02, 292.54it/s]
68%|██████▊ | cHPI locations : 1149/1696 [00:03<00:01, 338.62it/s]
70%|██████▉ | cHPI locations : 1186/1696 [00:03<00:01, 348.57it/s]
74%|███████▎ | cHPI locations : 1249/1696 [00:03<00:01, 395.38it/s]
76%|███████▌ | cHPI locations : 1286/1696 [00:03<00:01, 403.33it/s]
76%|███████▋ | cHPI locations : 1296/1696 [00:03<00:01, 389.08it/s]
77%|███████▋ | cHPI locations : 1301/1696 [00:03<00:01, 373.19it/s]
77%|███████▋ | cHPI locations : 1306/1696 [00:03<00:01, 357.58it/s]
77%|███████▋ | cHPI locations : 1311/1696 [00:04<00:01, 341.54it/s]
78%|███████▊ | cHPI locations : 1315/1696 [00:04<00:01, 317.91it/s]
78%|███████▊ | cHPI locations : 1319/1696 [00:04<00:01, 296.64it/s]
78%|███████▊ | cHPI locations : 1325/1696 [00:04<00:01, 277.24it/s]
78%|███████▊ | cHPI locations : 1331/1696 [00:04<00:01, 261.39it/s]
79%|███████▉ | cHPI locations : 1341/1696 [00:04<00:01, 246.32it/s]
81%|████████ | cHPI locations : 1375/1696 [00:04<00:01, 267.74it/s]
83%|████████▎ | cHPI locations : 1411/1696 [00:04<00:00, 291.20it/s]
85%|████████▍ | cHPI locations : 1441/1696 [00:04<00:00, 288.26it/s]
87%|████████▋ | cHPI locations : 1474/1696 [00:04<00:00, 309.40it/s]
89%|████████▉ | cHPI locations : 1508/1696 [00:04<00:00, 331.83it/s]
91%|█████████ | cHPI locations : 1541/1696 [00:04<00:00, 326.11it/s]
92%|█████████▏| cHPI locations : 1565/1696 [00:04<00:00, 311.92it/s]
93%|█████████▎| cHPI locations : 1573/1696 [00:05<00:00, 292.59it/s]
93%|█████████▎| cHPI locations : 1580/1696 [00:05<00:00, 278.48it/s]
94%|█████████▎| cHPI locations : 1586/1696 [00:05<00:00, 262.51it/s]
94%|█████████▍| cHPI locations : 1590/1696 [00:05<00:00, 244.78it/s]
94%|█████████▍| cHPI locations : 1594/1696 [00:05<00:00, 228.65it/s]
94%|█████████▍| cHPI locations : 1599/1696 [00:05<00:00, 214.70it/s]
95%|█████████▍| cHPI locations : 1609/1696 [00:05<00:00, 205.64it/s]
97%|█████████▋| cHPI locations : 1645/1696 [00:05<00:00, 225.82it/s]
97%|█████████▋| cHPI locations : 1652/1696 [00:05<00:00, 213.95it/s]
99%|█████████▉| cHPI locations : 1686/1696 [00:05<00:00, 233.13it/s]
100%|██████████| cHPI locations : 1696/1696 [00:05<00:00, 285.43it/s]
Lastly, compute head positions from the coil locations:
head_pos = mne.chpi.compute_head_pos(raw.info, chpi_locs, verbose=True)
t=9.000: 5/5 good HPI fits, movements [mm/s] = 0.1 / 0.2 / 0.5 / 0.2 / 0.3
t=10.000: 5/5 good HPI fits, movements [mm/s] = 0.1 / 0.2 / 0.0 / 0.2 / 0.1
t=11.000: 5/5 good HPI fits, movements [mm/s] = 0.0 / 0.1 / 0.1 / 0.0 / 0.0
t=12.000: 5/5 good HPI fits, movements [mm/s] = 0.0 / 0.0 / 0.1 / 0.0 / 0.0
t=13.000: 5/5 good HPI fits, movements [mm/s] = 0.0 / 0.1 / 0.0 / 0.0 / 0.0
t=14.000: 5/5 good HPI fits, movements [mm/s] = 0.0 / 0.1 / 0.1 / 0.1 / 0.1
t=14.580: 5/5 good HPI fits, movements [mm/s] = 5.0 / 4.8 / 6.1 / 5.5 / 4.9
t=14.670: 5/5 good HPI fits, movements [mm/s] = 33.3 / 33.8 / 39.9 / 37.9 / 30.2
t=14.720: 5/5 good HPI fits, movements [mm/s] = 49.3 / 47.7 / 60.2 / 59.1 / 44.3
t=14.760: 5/5 good HPI fits, movements [mm/s] = 64.9 / 63.6 / 73.0 / 71.3 / 56.6
t=14.800: 5/5 good HPI fits, movements [mm/s] = 67.4 / 59.1 / 83.1 / 78.2 / 60.9
t=14.860: 5/5 good HPI fits, movements [mm/s] = 54.4 / 52.2 / 57.6 / 62.0 / 47.2
t=14.990: 5/5 good HPI fits, movements [mm/s] = 23.3 / 22.6 / 24.2 / 27.0 / 19.8
t=15.990: 5/5 good HPI fits, movements [mm/s] = 0.7 / 0.9 / 0.8 / 0.8 / 0.9
t=16.880: 5/5 good HPI fits, movements [mm/s] = 2.5 / 3.4 / 3.0 / 3.8 / 3.3
t=16.930: 5/5 good HPI fits, movements [mm/s] = 52.2 / 55.2 / 58.3 / 67.0 / 59.7
t=16.990: 5/5 good HPI fits, movements [mm/s] = 57.1 / 53.8 / 62.0 / 65.1 / 57.7
t=17.040: 5/5 good HPI fits, movements [mm/s] = 53.0 / 50.7 / 60.0 / 61.9 / 49.3
t=17.090: 5/5 good HPI fits, movements [mm/s] = 57.9 / 49.2 / 66.6 / 64.8 / 51.6
t=17.140: 5/5 good HPI fits, movements [mm/s] = 60.4 / 54.7 / 72.6 / 67.3 / 57.7
t=17.180: 5/5 good HPI fits, movements [mm/s] = 63.6 / 58.2 / 74.5 / 72.2 / 61.7
t=17.220: 5/5 good HPI fits, movements [mm/s] = 61.0 / 60.0 / 75.2 / 71.3 / 63.3
t=17.260: 5/5 good HPI fits, movements [mm/s] = 67.9 / 66.3 / 73.4 / 68.9 / 68.9
t=17.320: 5/5 good HPI fits, movements [mm/s] = 49.7 / 50.9 / 54.8 / 55.0 / 52.6
t=17.470: 5/5 good HPI fits, movements [mm/s] = 18.4 / 21.5 / 19.3 / 20.5 / 17.3
t=18.470: 5/5 good HPI fits, movements [mm/s] = 1.9 / 2.7 / 2.0 / 2.4 / 1.9
t=19.390: 5/5 good HPI fits, movements [mm/s] = 2.4 / 2.7 / 1.9 / 2.9 / 2.5
t=19.500: 5/5 good HPI fits, movements [mm/s] = 23.0 / 25.3 / 19.4 / 28.9 / 27.7
t=19.610: 5/5 good HPI fits, movements [mm/s] = 21.2 / 25.7 / 21.8 / 31.6 / 31.3
t=19.710: 5/5 good HPI fits, movements [mm/s] = 22.0 / 20.7 / 23.0 / 29.7 / 27.8
t=19.850: 5/5 good HPI fits, movements [mm/s] = 16.5 / 14.7 / 18.5 / 21.9 / 20.1
t=20.850: 5/5 good HPI fits, movements [mm/s] = 2.3 / 1.9 / 2.0 / 2.5 / 2.2
t=21.850: 5/5 good HPI fits, movements [mm/s] = 0.6 / 1.7 / 1.7 / 2.0 / 1.9
t=21.950: 5/5 good HPI fits, movements [mm/s] = 25.8 / 27.1 / 24.1 / 32.2 / 26.4
t=22.000: 5/5 good HPI fits, movements [mm/s] = 66.0 / 58.9 / 46.4 / 64.8 / 51.9
t=22.050: 5/5 good HPI fits, movements [mm/s] = 59.7 / 62.9 / 51.0 / 69.1 / 57.9
t=22.100: 5/5 good HPI fits, movements [mm/s] = 61.5 / 65.4 / 58.5 / 75.0 / 64.8
t=22.140: 5/5 good HPI fits, movements [mm/s] = 74.4 / 73.6 / 62.3 / 79.9 / 68.2
t=22.180: 5/5 good HPI fits, movements [mm/s] = 63.9 / 59.6 / 52.9 / 67.2 / 53.2
t=22.240: 5/5 good HPI fits, movements [mm/s] = 38.3 / 39.0 / 36.5 / 48.3 / 42.2
t=22.300: 5/5 good HPI fits, movements [mm/s] = 42.0 / 40.4 / 33.7 / 47.1 / 38.9
t=22.400: 5/5 good HPI fits, movements [mm/s] = 31.7 / 28.5 / 22.1 / 30.8 / 25.0
t=23.400: 5/5 good HPI fits, movements [mm/s] = 1.8 / 1.8 / 1.1 / 1.6 / 1.2
t=24.400: 5/5 good HPI fits, movements [mm/s] = 1.2 / 0.8 / 0.7 / 0.8 / 0.7
t=24.640: 5/5 good HPI fits, movements [mm/s] = 12.6 / 11.6 / 11.5 / 13.0 / 11.0
t=24.720: 5/5 good HPI fits, movements [mm/s] = 30.3 / 33.0 / 31.4 / 39.0 / 35.0
t=24.790: 5/5 good HPI fits, movements [mm/s] = 31.3 / 35.7 / 33.0 / 43.0 / 40.8
t=24.850: 5/5 good HPI fits, movements [mm/s] = 37.2 / 41.4 / 35.6 / 44.6 / 42.4
t=24.890: 5/5 good HPI fits, movements [mm/s] = 50.1 / 51.9 / 49.7 / 60.1 / 52.9
t=24.930: 5/5 good HPI fits, movements [mm/s] = 56.4 / 55.3 / 59.1 / 68.3 / 61.9
t=24.980: 5/5 good HPI fits, movements [mm/s] = 47.2 / 47.1 / 51.0 / 58.7 / 53.7
t=25.080: 5/5 good HPI fits, movements [mm/s] = 23.8 / 19.4 / 23.3 / 28.2 / 26.3
t=25.510: 5/5 good HPI fits, movements [mm/s] = 2.4 / 6.6 / 7.4 / 6.5 / 7.8
Note that these can then be written to disk or read from disk with
mne.chpi.write_head_pos()
and mne.chpi.read_head_pos()
,
respectively.
Visualizing continuous head position#
We can plot as traces, which is especially useful for long recordings:
mne.viz.plot_head_positions(head_pos, mode="traces", totals=True)
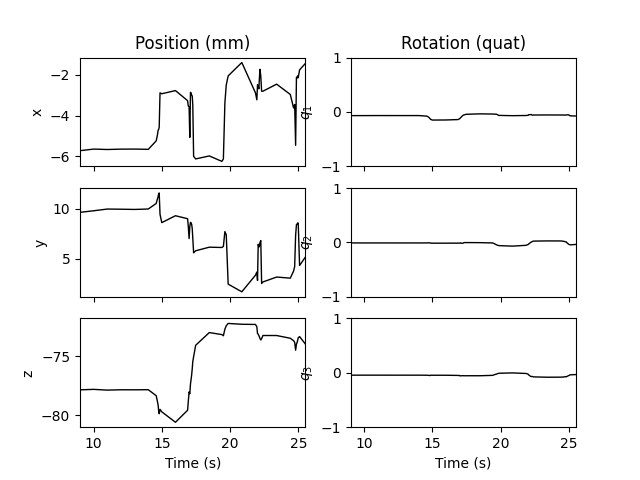
Or we can visualize them as a continuous field (with the vectors pointing in the head-upward direction):
mne.viz.plot_head_positions(head_pos, mode="field")
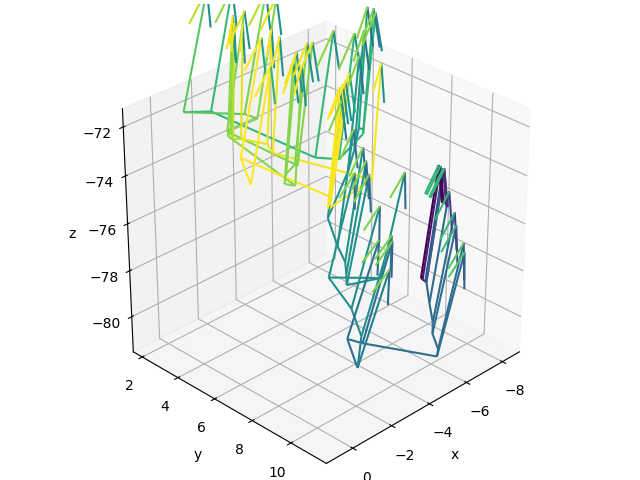
These head positions can then be used with
mne.preprocessing.maxwell_filter()
to compensate for movement,
or with mne.preprocessing.annotate_movement()
to mark segments as
bad that deviate too much from the average head position.
Computing SNR of the HPI signal#
It is also possible to compute the SNR of the continuous HPI measurements.
This can be a useful proxy for head position along the vertical dimension,
i.e., it can indicate the distance between the HPI coils and the MEG sensors.
Using compute_chpi_snr
, the HPI power and SNR are computed
separately for each MEG sensor type and each HPI coil (frequency), along with
the residual power for each sensor type. The results can then be visualized
with plot_chpi_snr
. Here we’ll just show a few seconds, for speed:
raw.crop(tmin=5, tmax=10)
snr_dict = mne.chpi.compute_chpi_snr(raw)
fig = mne.viz.plot_chpi_snr(snr_dict)
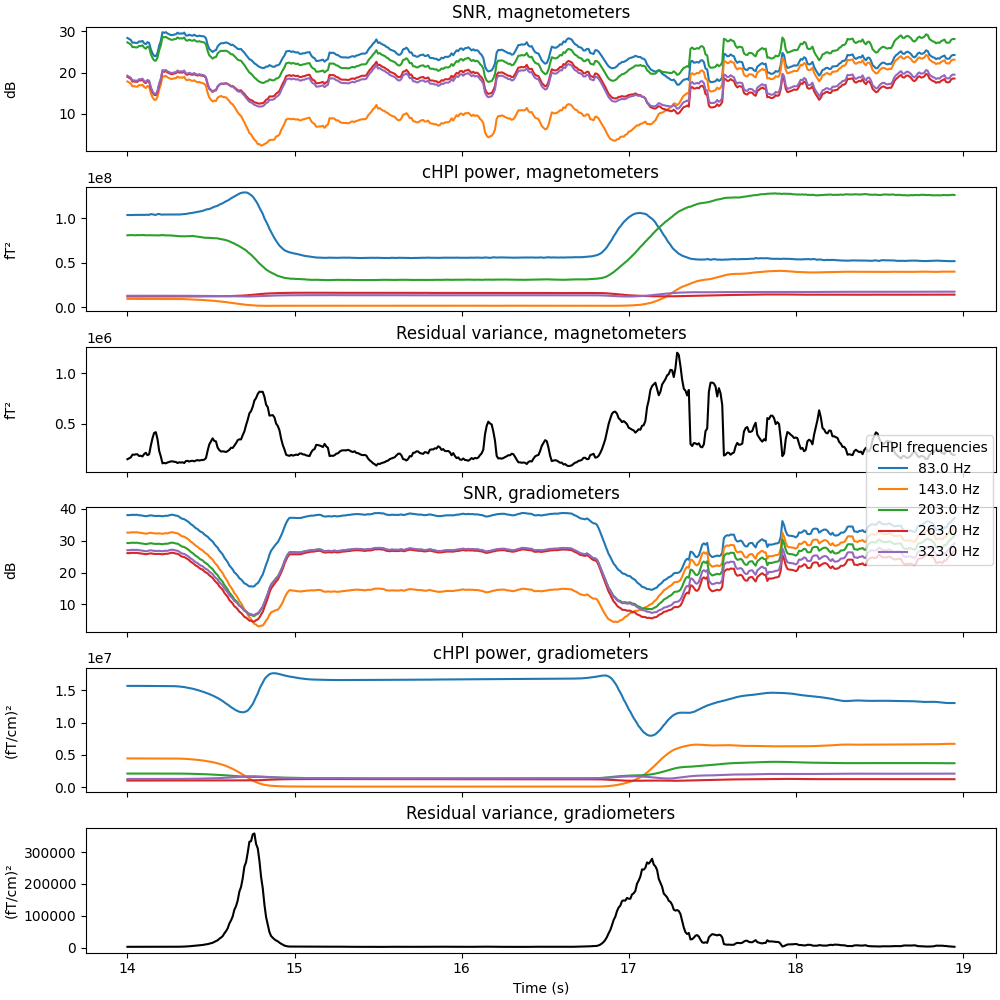
Using 5 HPI coils: 83 143 203 263 323 Hz
Line interference frequencies: 60.0 120.0 180.0 240.0 300.0 360.0 Hz
Using time window: 83.3 ms
Fitting 5 HPI coil locations at up to 496 time points (5.0 s duration)
0%| | cHPI SNRs : 0/496 [00:00<?, ?it/s]
0%| | cHPI SNRs : 1/496 [00:02<22:27, 2.72s/it]
2%|▏ | cHPI SNRs : 9/496 [00:02<02:21, 3.44it/s]
3%|▎ | cHPI SNRs : 17/496 [00:02<01:12, 6.62it/s]
5%|▌ | cHPI SNRs : 25/496 [00:02<00:47, 9.93it/s]
7%|▋ | cHPI SNRs : 33/496 [00:02<00:34, 13.35it/s]
8%|▊ | cHPI SNRs : 41/496 [00:02<00:26, 16.90it/s]
10%|▉ | cHPI SNRs : 49/496 [00:02<00:21, 20.58it/s]
11%|█▏ | cHPI SNRs : 56/496 [00:02<00:18, 23.90it/s]
13%|█▎ | cHPI SNRs : 64/496 [00:02<00:15, 27.83it/s]
15%|█▍ | cHPI SNRs : 72/496 [00:02<00:13, 31.89it/s]
16%|█▌ | cHPI SNRs : 80/496 [00:02<00:11, 36.08it/s]
18%|█▊ | cHPI SNRs : 88/496 [00:02<00:10, 40.41it/s]
19%|█▉ | cHPI SNRs : 96/496 [00:02<00:08, 44.87it/s]
21%|██ | cHPI SNRs : 104/496 [00:02<00:07, 49.45it/s]
23%|██▎ | cHPI SNRs : 112/496 [00:02<00:07, 54.17it/s]
24%|██▍ | cHPI SNRs : 120/496 [00:02<00:06, 59.01it/s]
26%|██▌ | cHPI SNRs : 128/496 [00:03<00:05, 63.97it/s]
27%|██▋ | cHPI SNRs : 136/496 [00:03<00:05, 69.08it/s]
29%|██▉ | cHPI SNRs : 144/496 [00:03<00:04, 74.33it/s]
31%|███ | cHPI SNRs : 152/496 [00:03<00:04, 79.68it/s]
32%|███▏ | cHPI SNRs : 160/496 [00:03<00:03, 85.15it/s]
34%|███▎ | cHPI SNRs : 167/496 [00:03<00:03, 89.99it/s]
35%|███▌ | cHPI SNRs : 175/496 [00:03<00:03, 95.72it/s]
37%|███▋ | cHPI SNRs : 183/496 [00:03<00:03, 101.58it/s]
39%|███▊ | cHPI SNRs : 191/496 [00:03<00:02, 107.47it/s]
40%|████ | cHPI SNRs : 199/496 [00:03<00:02, 113.53it/s]
42%|████▏ | cHPI SNRs : 207/496 [00:03<00:02, 119.68it/s]
43%|████▎ | cHPI SNRs : 215/496 [00:03<00:02, 125.84it/s]
45%|████▍ | cHPI SNRs : 223/496 [00:03<00:02, 132.17it/s]
47%|████▋ | cHPI SNRs : 231/496 [00:03<00:01, 138.48it/s]
48%|████▊ | cHPI SNRs : 239/496 [00:03<00:01, 144.83it/s]
50%|████▉ | cHPI SNRs : 247/496 [00:03<00:01, 151.28it/s]
51%|█████▏ | cHPI SNRs : 255/496 [00:03<00:01, 157.74it/s]
53%|█████▎ | cHPI SNRs : 263/496 [00:03<00:01, 164.20it/s]
55%|█████▍ | cHPI SNRs : 271/496 [00:03<00:01, 170.80it/s]
56%|█████▋ | cHPI SNRs : 279/496 [00:03<00:01, 177.43it/s]
58%|█████▊ | cHPI SNRs : 286/496 [00:03<00:01, 183.13it/s]
59%|█████▉ | cHPI SNRs : 294/496 [00:03<00:01, 189.86it/s]
61%|██████ | cHPI SNRs : 302/496 [00:03<00:00, 196.50it/s]
62%|██████▎ | cHPI SNRs : 310/496 [00:03<00:00, 203.26it/s]
64%|██████▍ | cHPI SNRs : 318/496 [00:03<00:00, 209.92it/s]
66%|██████▌ | cHPI SNRs : 326/496 [00:03<00:00, 216.57it/s]
67%|██████▋ | cHPI SNRs : 334/496 [00:03<00:00, 223.04it/s]
69%|██████▉ | cHPI SNRs : 341/496 [00:03<00:00, 228.59it/s]
70%|███████ | cHPI SNRs : 349/496 [00:03<00:00, 235.18it/s]
72%|███████▏ | cHPI SNRs : 357/496 [00:03<00:00, 241.65it/s]
74%|███████▎ | cHPI SNRs : 365/496 [00:03<00:00, 248.17it/s]
75%|███████▌ | cHPI SNRs : 373/496 [00:03<00:00, 254.31it/s]
77%|███████▋ | cHPI SNRs : 381/496 [00:03<00:00, 260.50it/s]
78%|███████▊ | cHPI SNRs : 389/496 [00:03<00:00, 266.66it/s]
80%|████████ | cHPI SNRs : 397/496 [00:03<00:00, 272.55it/s]
82%|████████▏ | cHPI SNRs : 405/496 [00:03<00:00, 278.75it/s]
83%|████████▎ | cHPI SNRs : 413/496 [00:03<00:00, 284.69it/s]
85%|████████▍ | cHPI SNRs : 421/496 [00:03<00:00, 290.18it/s]
86%|████████▋ | cHPI SNRs : 428/496 [00:03<00:00, 294.80it/s]
88%|████████▊ | cHPI SNRs : 436/496 [00:03<00:00, 300.15it/s]
90%|████████▉ | cHPI SNRs : 444/496 [00:03<00:00, 305.51it/s]
91%|█████████ | cHPI SNRs : 452/496 [00:03<00:00, 310.88it/s]
93%|█████████▎| cHPI SNRs : 460/496 [00:03<00:00, 316.24it/s]
94%|█████████▍| cHPI SNRs : 467/496 [00:03<00:00, 320.36it/s]
96%|█████████▌| cHPI SNRs : 475/496 [00:03<00:00, 325.21it/s]
97%|█████████▋| cHPI SNRs : 482/496 [00:03<00:00, 328.95it/s]
99%|█████████▉| cHPI SNRs : 490/496 [00:03<00:00, 333.68it/s]
100%|██████████| cHPI SNRs : 496/496 [00:03<00:00, 129.49it/s]
Total running time of the script: (0 minutes 30.503 seconds)