Note
Go to the end to download the full example code.
Decoding in time-frequency space using Common Spatial Patterns (CSP)#
The time-frequency decomposition is estimated by iterating over raw data that has been band-passed at different frequencies. This is used to compute a covariance matrix over each epoch or a rolling time-window and extract the CSP filtered signals. A linear discriminant classifier is then applied to these signals.
# Authors: Laura Gwilliams <laura.gwilliams@nyu.edu>
# Jean-Rémi King <jeanremi.king@gmail.com>
# Alex Barachant <alexandre.barachant@gmail.com>
# Alexandre Gramfort <alexandre.gramfort@inria.fr>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
import matplotlib.pyplot as plt
import numpy as np
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.model_selection import StratifiedKFold, cross_val_score
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import LabelEncoder
from mne import Epochs, create_info
from mne.datasets import eegbci
from mne.decoding import CSP
from mne.io import concatenate_raws, read_raw_edf
from mne.time_frequency import AverageTFRArray
Set parameters and read data
subject = 1
runs = [6, 10, 14]
raw_fnames = eegbci.load_data(subject, runs)
raw = concatenate_raws([read_raw_edf(f) for f in raw_fnames])
raw.annotations.rename(dict(T1="hands", T2="feet"))
# Extract information from the raw file
sfreq = raw.info["sfreq"]
raw.pick(picks="eeg", exclude="bads")
raw.load_data()
# Assemble the classifier using scikit-learn pipeline
clf = make_pipeline(
CSP(n_components=4, reg=None, log=True, norm_trace=False),
LinearDiscriminantAnalysis(),
)
n_splits = 3 # for cross-validation, 5 is better, here we use 3 for speed
cv = StratifiedKFold(n_splits=n_splits, shuffle=True, random_state=42)
# Classification & time-frequency parameters
tmin, tmax = -0.200, 2.000
n_cycles = 10.0 # how many complete cycles: used to define window size
min_freq = 8.0
max_freq = 20.0
n_freqs = 6 # how many frequency bins to use
# Assemble list of frequency range tuples
freqs = np.linspace(min_freq, max_freq, n_freqs) # assemble frequencies
freq_ranges = list(zip(freqs[:-1], freqs[1:])) # make freqs list of tuples
# Infer window spacing from the max freq and number of cycles to avoid gaps
window_spacing = n_cycles / np.max(freqs) / 2.0
centered_w_times = np.arange(tmin, tmax, window_spacing)[1:]
n_windows = len(centered_w_times)
# Instantiate label encoder
le = LabelEncoder()
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R06.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R10.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/circleci/mne_data/MNE-eegbci-data/files/eegmmidb/1.0.0/S001/S001R14.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 59999 = 0.000 ... 374.994 secs...
Loop through frequencies, apply classifier and save scores
# init scores
freq_scores = np.zeros((n_freqs - 1,))
# Loop through each frequency range of interest
for freq, (fmin, fmax) in enumerate(freq_ranges):
# Infer window size based on the frequency being used
w_size = n_cycles / ((fmax + fmin) / 2.0) # in seconds
# Apply band-pass filter to isolate the specified frequencies
raw_filter = raw.copy().filter(
fmin, fmax, fir_design="firwin", skip_by_annotation="edge"
)
# Extract epochs from filtered data, padded by window size
epochs = Epochs(
raw_filter,
event_id=["hands", "feet"],
tmin=tmin - w_size,
tmax=tmax + w_size,
proj=False,
baseline=None,
preload=True,
)
epochs.drop_bad()
y = le.fit_transform(epochs.events[:, 2])
X = epochs.get_data(copy=False)
# Save mean scores over folds for each frequency and time window
freq_scores[freq] = np.mean(
cross_val_score(estimator=clf, X=X, y=y, scoring="roc_auc", cv=cv), axis=0
)
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 8 - 10 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 8.00
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 7.00 Hz)
- Upper passband edge: 10.40 Hz
- Upper transition bandwidth: 2.60 Hz (-6 dB cutoff frequency: 11.70 Hz)
- Filter length: 265 samples (1.656 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 701 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00016 (2.2e-16 eps * 64 dim * 1.2e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00017 (2.2e-16 eps * 64 dim * 1.2e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00017 (2.2e-16 eps * 64 dim * 1.2e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 10 - 13 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.40
- Lower transition bandwidth: 2.60 Hz (-6 dB cutoff frequency: 9.10 Hz)
- Upper passband edge: 12.80 Hz
- Upper transition bandwidth: 3.20 Hz (-6 dB cutoff frequency: 14.40 Hz)
- Filter length: 205 samples (1.281 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 629 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00015 (2.2e-16 eps * 64 dim * 1.1e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00016 (2.2e-16 eps * 64 dim * 1.1e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00016 (2.2e-16 eps * 64 dim * 1.1e+10 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 13 - 15 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 12.80
- Lower transition bandwidth: 3.20 Hz (-6 dB cutoff frequency: 11.20 Hz)
- Upper passband edge: 15.20 Hz
- Upper transition bandwidth: 3.80 Hz (-6 dB cutoff frequency: 17.10 Hz)
- Filter length: 165 samples (1.031 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 581 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00013 (2.2e-16 eps * 64 dim * 9.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00014 (2.2e-16 eps * 64 dim * 9.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 15 - 18 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 15.20
- Lower transition bandwidth: 3.80 Hz (-6 dB cutoff frequency: 13.30 Hz)
- Upper passband edge: 17.60 Hz
- Upper transition bandwidth: 4.40 Hz (-6 dB cutoff frequency: 19.80 Hz)
- Filter length: 139 samples (0.869 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 549 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 0.00011 (2.2e-16 eps * 64 dim * 7.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 18 - 20 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 17.60
- Lower transition bandwidth: 4.40 Hz (-6 dB cutoff frequency: 15.40 Hz)
- Upper passband edge: 20.00 Hz
- Upper transition bandwidth: 5.00 Hz (-6 dB cutoff frequency: 22.50 Hz)
- Filter length: 121 samples (0.756 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 523 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 9.3e-05 (2.2e-16 eps * 64 dim * 6.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.5e-05 (2.2e-16 eps * 64 dim * 6.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 9.3e-05 (2.2e-16 eps * 64 dim * 6.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Plot frequency results
plt.bar(
freqs[:-1], freq_scores, width=np.diff(freqs)[0], align="edge", edgecolor="black"
)
plt.xticks(freqs)
plt.ylim([0, 1])
plt.axhline(
len(epochs["feet"]) / len(epochs), color="k", linestyle="--", label="chance level"
)
plt.legend()
plt.xlabel("Frequency (Hz)")
plt.ylabel("Decoding Scores")
plt.title("Frequency Decoding Scores")
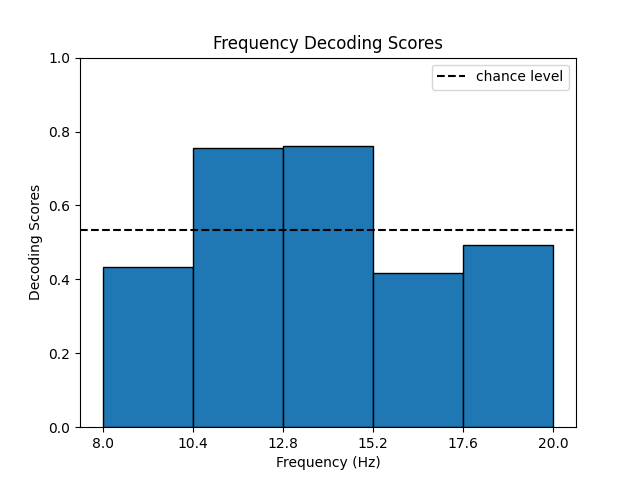
Loop through frequencies and time, apply classifier and save scores
# init scores
tf_scores = np.zeros((n_freqs - 1, n_windows))
# Loop through each frequency range of interest
for freq, (fmin, fmax) in enumerate(freq_ranges):
# Infer window size based on the frequency being used
w_size = n_cycles / ((fmax + fmin) / 2.0) # in seconds
# Apply band-pass filter to isolate the specified frequencies
raw_filter = raw.copy().filter(
fmin, fmax, fir_design="firwin", skip_by_annotation="edge"
)
# Extract epochs from filtered data, padded by window size
epochs = Epochs(
raw_filter,
event_id=["hands", "feet"],
tmin=tmin - w_size,
tmax=tmax + w_size,
proj=False,
baseline=None,
preload=True,
)
epochs.drop_bad()
y = le.fit_transform(epochs.events[:, 2])
# Roll covariance, csp and lda over time
for t, w_time in enumerate(centered_w_times):
# Center the min and max of the window
w_tmin = w_time - w_size / 2.0
w_tmax = w_time + w_size / 2.0
# Crop data into time-window of interest
X = epochs.get_data(tmin=w_tmin, tmax=w_tmax, copy=False)
# Save mean scores over folds for each frequency and time window
tf_scores[freq, t] = np.mean(
cross_val_score(estimator=clf, X=X, y=y, scoring="roc_auc", cv=cv), axis=0
)
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 8 - 10 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 8.00
- Lower transition bandwidth: 2.00 Hz (-6 dB cutoff frequency: 7.00 Hz)
- Upper passband edge: 10.40 Hz
- Upper transition bandwidth: 2.60 Hz (-6 dB cutoff frequency: 11.70 Hz)
- Filter length: 265 samples (1.656 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 701 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 8.8e-05 (2.2e-16 eps * 64 dim * 6.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.6e-05 (2.2e-16 eps * 64 dim * 6.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.8e-05 (2.2e-16 eps * 64 dim * 6.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.7e-05 (2.2e-16 eps * 64 dim * 6.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.5e-05 (2.2e-16 eps * 64 dim * 6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.6e-05 (2.2e-16 eps * 64 dim * 6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.3e-05 (2.2e-16 eps * 64 dim * 5.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.3e-05 (2.2e-16 eps * 64 dim * 5.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.1e-05 (2.2e-16 eps * 64 dim * 5.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8e-05 (2.2e-16 eps * 64 dim * 5.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.9e-05 (2.2e-16 eps * 64 dim * 5.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.9e-05 (2.2e-16 eps * 64 dim * 5.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.9e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.9e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.9e-05 (2.2e-16 eps * 64 dim * 5.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.6e-05 (2.2e-16 eps * 64 dim * 6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.5e-05 (2.2e-16 eps * 64 dim * 6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.6e-05 (2.2e-16 eps * 64 dim * 6.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.7e-05 (2.2e-16 eps * 64 dim * 6.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.6e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.5e-05 (2.2e-16 eps * 64 dim * 6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 8.8e-05 (2.2e-16 eps * 64 dim * 6.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 10 - 13 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 10.40
- Lower transition bandwidth: 2.60 Hz (-6 dB cutoff frequency: 9.10 Hz)
- Upper passband edge: 12.80 Hz
- Upper transition bandwidth: 3.20 Hz (-6 dB cutoff frequency: 14.40 Hz)
- Filter length: 205 samples (1.281 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 629 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 7.4e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.8e-05 (2.2e-16 eps * 64 dim * 5.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.6e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.4e-05 (2.2e-16 eps * 64 dim * 5.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.6e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.6e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.7e-05 (2.2e-16 eps * 64 dim * 4.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.8e-05 (2.2e-16 eps * 64 dim * 4.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.1e-05 (2.2e-16 eps * 64 dim * 5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.3e-05 (2.2e-16 eps * 64 dim * 5.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.9e-05 (2.2e-16 eps * 64 dim * 4.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.5e-05 (2.2e-16 eps * 64 dim * 5.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 7.7e-05 (2.2e-16 eps * 64 dim * 5.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 13 - 15 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 12.80
- Lower transition bandwidth: 3.20 Hz (-6 dB cutoff frequency: 11.20 Hz)
- Upper passband edge: 15.20 Hz
- Upper transition bandwidth: 3.80 Hz (-6 dB cutoff frequency: 17.10 Hz)
- Filter length: 165 samples (1.031 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 581 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.3e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.1e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.6e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.7e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.9e-05 (2.2e-16 eps * 64 dim * 4.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.5e-05 (2.2e-16 eps * 64 dim * 3.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.8e-05 (2.2e-16 eps * 64 dim * 4.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.5e-05 (2.2e-16 eps * 64 dim * 4.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.6e-05 (2.2e-16 eps * 64 dim * 4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.4e-05 (2.2e-16 eps * 64 dim * 4.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 6.2e-05 (2.2e-16 eps * 64 dim * 4.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 15 - 18 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 15.20
- Lower transition bandwidth: 3.80 Hz (-6 dB cutoff frequency: 13.30 Hz)
- Upper passband edge: 17.60 Hz
- Upper transition bandwidth: 4.40 Hz (-6 dB cutoff frequency: 19.80 Hz)
- Filter length: 139 samples (0.869 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 549 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.6e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.1e-05 (2.2e-16 eps * 64 dim * 2.9e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.7e-05 (2.2e-16 eps * 64 dim * 3.3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.1e-05 (2.2e-16 eps * 64 dim * 3.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5.1e-05 (2.2e-16 eps * 64 dim * 3.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.5e-05 (2.2e-16 eps * 64 dim * 3.2e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 5e-05 (2.2e-16 eps * 64 dim * 3.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.8e-05 (2.2e-16 eps * 64 dim * 3.4e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Filtering raw data in 3 contiguous segments
Setting up band-pass filter from 18 - 20 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 17.60
- Lower transition bandwidth: 4.40 Hz (-6 dB cutoff frequency: 15.40 Hz)
- Upper passband edge: 20.00 Hz
- Upper transition bandwidth: 5.00 Hz (-6 dB cutoff frequency: 22.50 Hz)
- Filter length: 121 samples (0.756 s)
Used Annotations descriptions: [np.str_('T0'), np.str_('feet'), np.str_('hands')]
Ignoring annotation durations and creating fixed-duration epochs around annotation onsets.
Not setting metadata
45 matching events found
No baseline correction applied
Using data from preloaded Raw for 45 events and 523 original time points ...
0 bad epochs dropped
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.8e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.8e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.6e-05 (2.2e-16 eps * 64 dim * 2.5e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.9e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.8e-05 (2.2e-16 eps * 64 dim * 2.7e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 3.7e-05 (2.2e-16 eps * 64 dim * 2.6e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.3e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4e-05 (2.2e-16 eps * 64 dim * 2.8e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.4e-05 (2.2e-16 eps * 64 dim * 3.1e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Computing rank from data with rank=None
Using tolerance 4.2e-05 (2.2e-16 eps * 64 dim * 3e+09 max singular value)
Estimated rank (data): 64
data: rank 64 computed from 64 data channels with 0 projectors
Reducing data rank from 64 -> 64
Estimating class=0 covariance using EMPIRICAL
Done.
Estimating class=1 covariance using EMPIRICAL
Done.
Plot time-frequency results
# Set up time frequency object
av_tfr = AverageTFRArray(
info=create_info(["freq"], sfreq),
data=tf_scores[np.newaxis, :],
times=centered_w_times,
freqs=freqs[1:],
nave=1,
)
chance = np.mean(y) # set chance level to white in the plot
av_tfr.plot(
[0], vlim=(chance, None), title="Time-Frequency Decoding Scores", cmap=plt.cm.Reds
)
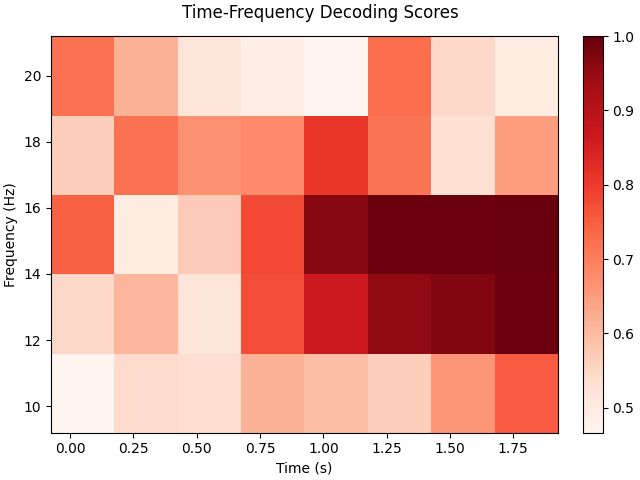
No baseline correction applied
Total running time of the script: (0 minutes 14.985 seconds)