Note
Go to the end to download the full example code.
Receptive Field Estimation and Prediction#
This example reproduces figures from Lalor et al.’s mTRF toolbox in
MATLAB [1]. We will show how the
mne.decoding.ReceptiveField
class
can perform a similar function along with scikit-learn. We will first fit a
linear encoding model using the continuously-varying speech envelope to predict
activity of a 128 channel EEG system. Then, we will take the reverse approach
and try to predict the speech envelope from the EEG (known in the literature
as a decoding model, or simply stimulus reconstruction).
# Authors: Chris Holdgraf <choldgraf@gmail.com>
# Eric Larson <larson.eric.d@gmail.com>
# Nicolas Barascud <nicolas.barascud@ens.fr>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
from os.path import join
import matplotlib.pyplot as plt
import numpy as np
from scipy.io import loadmat
from sklearn.model_selection import KFold
from sklearn.preprocessing import scale
import mne
from mne.decoding import ReceptiveField
Load the data from the publication#
First we will load the data collected in [1]. In this experiment subjects listened to natural speech. Raw EEG and the speech stimulus are provided. We will load these below, downsampling the data in order to speed up computation since we know that our features are primarily low-frequency in nature. Then we’ll visualize both the EEG and speech envelope.
path = mne.datasets.mtrf.data_path()
decim = 2
data = loadmat(join(path, "speech_data.mat"))
raw = data["EEG"].T
speech = data["envelope"].T
sfreq = float(data["Fs"].item())
sfreq /= decim
speech = mne.filter.resample(speech, down=decim, method="polyphase")
raw = mne.filter.resample(raw, down=decim, method="polyphase")
# Read in channel positions and create our MNE objects from the raw data
montage = mne.channels.make_standard_montage("biosemi128")
info = mne.create_info(montage.ch_names, sfreq, "eeg").set_montage(montage)
raw = mne.io.RawArray(raw, info)
n_channels = len(raw.ch_names)
# Plot a sample of brain and stimulus activity
fig, ax = plt.subplots(layout="constrained")
lns = ax.plot(scale(raw[:, :800][0].T), color="k", alpha=0.1)
ln1 = ax.plot(scale(speech[0, :800]), color="r", lw=2)
ax.legend([lns[0], ln1[0]], ["EEG", "Speech Envelope"], frameon=False)
ax.set(title="Sample activity", xlabel="Time (s)")
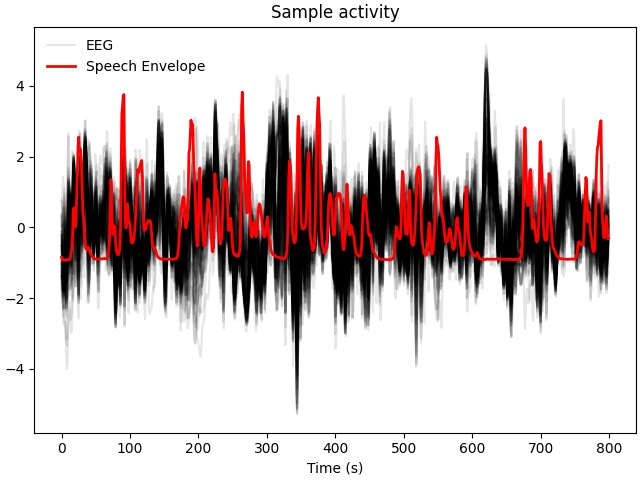
Polyphase resampling neighborhood: ±2 input samples
Polyphase resampling neighborhood: ±2 input samples
Creating RawArray with float64 data, n_channels=128, n_times=7677
Range : 0 ... 7676 = 0.000 ... 119.938 secs
Ready.
Create and fit a receptive field model#
We will construct an encoding model to find the linear relationship between a time-delayed version of the speech envelope and the EEG signal. This allows us to make predictions about the response to new stimuli.
# Define the delays that we will use in the receptive field
tmin, tmax = -0.2, 0.4
# Initialize the model
rf = ReceptiveField(
tmin, tmax, sfreq, feature_names=["envelope"], estimator=1.0, scoring="corrcoef"
)
# We'll have (tmax - tmin) * sfreq delays
# and an extra 2 delays since we are inclusive on the beginning / end index
n_delays = int((tmax - tmin) * sfreq) + 2
n_splits = 3
cv = KFold(n_splits)
# Prepare model data (make time the first dimension)
speech = speech.T
Y, _ = raw[:] # Outputs for the model
Y = Y.T
# Iterate through splits, fit the model, and predict/test on held-out data
coefs = np.zeros((n_splits, n_channels, n_delays))
scores = np.zeros((n_splits, n_channels))
for ii, (train, test) in enumerate(cv.split(speech)):
print(f"split {ii + 1} / {n_splits}")
rf.fit(speech[train], Y[train])
scores[ii] = rf.score(speech[test], Y[test])
# coef_ is shape (n_outputs, n_features, n_delays). we only have 1 feature
coefs[ii] = rf.coef_[:, 0, :]
times = rf.delays_ / float(rf.sfreq)
# Average scores and coefficients across CV splits
mean_coefs = coefs.mean(axis=0)
mean_scores = scores.mean(axis=0)
# Plot mean prediction scores across all channels
fig, ax = plt.subplots(layout="constrained")
ix_chs = np.arange(n_channels)
ax.plot(ix_chs, mean_scores)
ax.axhline(0, ls="--", color="r")
ax.set(title="Mean prediction score", xlabel="Channel", ylabel="Score ($r$)")
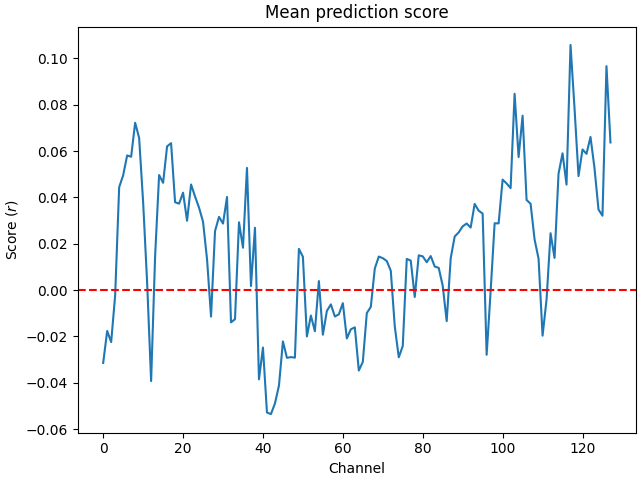
split 1 / 3
Fitting 1 epochs, 1 channels
0%| | Sample : 0/2 [00:00<?, ?it/s]
50%|█████ | Sample : 1/2 [00:02<00:02, 2.99s/it]split 2 / 3
Fitting 1 epochs, 1 channels
0%| | Sample : 0/2 [00:00<?, ?it/s]
50%|█████ | Sample : 1/2 [00:00<00:00, 57.95it/s]
100%|██████████| Sample : 2/2 [00:00<00:00, 58.37it/s]
100%|██████████| Sample : 2/2 [00:00<00:00, 57.98it/s]
split 3 / 3
Fitting 1 epochs, 1 channels
0%| | Sample : 0/2 [00:00<?, ?it/s]
100%|██████████| Sample : 2/2 [00:00<00:00, 67.34it/s]
100%|██████████| Sample : 2/2 [00:00<00:00, 66.87it/s]
Investigate model coefficients#
Finally, we will look at how the linear coefficients (sometimes referred to as beta values) are distributed across time delays as well as across the scalp. We will recreate figure 1 and figure 2 from [1].
# Print mean coefficients across all time delays / channels (see Fig 1)
time_plot = 0.180 # For highlighting a specific time.
fig, ax = plt.subplots(figsize=(4, 8), layout="constrained")
max_coef = mean_coefs.max()
ax.pcolormesh(
times,
ix_chs,
mean_coefs,
cmap="RdBu_r",
vmin=-max_coef,
vmax=max_coef,
shading="gouraud",
)
ax.axvline(time_plot, ls="--", color="k", lw=2)
ax.set(
xlabel="Delay (s)",
ylabel="Channel",
title="Mean Model\nCoefficients",
xlim=times[[0, -1]],
ylim=[len(ix_chs) - 1, 0],
xticks=np.arange(tmin, tmax + 0.2, 0.2),
)
plt.setp(ax.get_xticklabels(), rotation=45)
# Make a topographic map of coefficients for a given delay (see Fig 2C)
ix_plot = np.argmin(np.abs(time_plot - times))
fig, ax = plt.subplots(layout="constrained")
mne.viz.plot_topomap(
mean_coefs[:, ix_plot], pos=info, axes=ax, show=False, vlim=(-max_coef, max_coef)
)
ax.set(title=f"Topomap of model coefficients\nfor delay {time_plot}")
Create and fit a stimulus reconstruction model#
We will now demonstrate another use case for the for the
mne.decoding.ReceptiveField
class as we try to predict the stimulus
activity from the EEG data. This is known in the literature as a decoding, or
stimulus reconstruction model [1].
A decoding model aims to find the
relationship between the speech signal and a time-delayed version of the EEG.
This can be useful as we exploit all of the available neural data in a
multivariate context, compared to the encoding case which treats each M/EEG
channel as an independent feature. Therefore, decoding models might provide a
better quality of fit (at the expense of not controlling for stimulus
covariance), especially for low SNR stimuli such as speech.
# We use the same lags as in :footcite:`CrosseEtAl2016`. Negative lags now
# index the relationship
# between the neural response and the speech envelope earlier in time, whereas
# positive lags would index how a unit change in the amplitude of the EEG would
# affect later stimulus activity (obviously this should have an amplitude of
# zero).
tmin, tmax = -0.2, 0.0
# Initialize the model. Here the features are the EEG data. We also specify
# ``patterns=True`` to compute inverse-transformed coefficients during model
# fitting (cf. next section and :footcite:`HaufeEtAl2014`).
# We'll use a ridge regression estimator with an alpha value similar to
# Crosse et al.
sr = ReceptiveField(
tmin,
tmax,
sfreq,
feature_names=raw.ch_names,
estimator=1e4,
scoring="corrcoef",
patterns=True,
)
# We'll have (tmax - tmin) * sfreq delays
# and an extra 2 delays since we are inclusive on the beginning / end index
n_delays = int((tmax - tmin) * sfreq) + 2
n_splits = 3
cv = KFold(n_splits)
# Iterate through splits, fit the model, and predict/test on held-out data
coefs = np.zeros((n_splits, n_channels, n_delays))
patterns = coefs.copy()
scores = np.zeros((n_splits,))
for ii, (train, test) in enumerate(cv.split(speech)):
print(f"split {ii + 1} / {n_splits}")
sr.fit(Y[train], speech[train])
scores[ii] = sr.score(Y[test], speech[test])[0]
# coef_ is shape (n_outputs, n_features, n_delays). We have 128 features
coefs[ii] = sr.coef_[0, :, :]
patterns[ii] = sr.patterns_[0, :, :]
times = sr.delays_ / float(sr.sfreq)
# Average scores and coefficients across CV splits
mean_coefs = coefs.mean(axis=0)
mean_patterns = patterns.mean(axis=0)
mean_scores = scores.mean(axis=0)
max_coef = np.abs(mean_coefs).max()
max_patterns = np.abs(mean_patterns).max()
split 1 / 3
Fitting 1 epochs, 128 channels
0%| | Sample : 0/8384 [00:00<?, ?it/s]
0%| | Sample : 1/8384 [00:00<02:26, 57.05it/s]
2%|▏ | Sample : 129/8384 [00:00<00:02, 3947.30it/s]
3%|▎ | Sample : 257/8384 [00:00<00:01, 5324.66it/s]
5%|▍ | Sample : 382/8384 [00:00<00:01, 5971.82it/s]
6%|▌ | Sample : 511/8384 [00:00<00:01, 6417.80it/s]
8%|▊ | Sample : 640/8384 [00:00<00:01, 6713.41it/s]
9%|▉ | Sample : 768/8384 [00:00<00:01, 6918.06it/s]
11%|█ | Sample : 899/8384 [00:00<00:01, 7100.55it/s]
12%|█▏ | Sample : 1028/8384 [00:00<00:01, 7227.00it/s]
14%|█▍ | Sample : 1157/8384 [00:00<00:00, 7329.74it/s]
15%|█▌ | Sample : 1286/8384 [00:00<00:00, 7408.18it/s]
17%|█▋ | Sample : 1416/8384 [00:00<00:00, 7482.26it/s]
18%|█▊ | Sample : 1544/8384 [00:00<00:00, 7533.61it/s]
20%|█▉ | Sample : 1674/8384 [00:00<00:00, 7586.26it/s]
21%|██▏ | Sample : 1801/8384 [00:00<00:00, 7613.97it/s]
23%|██▎ | Sample : 1931/8384 [00:00<00:00, 7655.17it/s]
25%|██▍ | Sample : 2058/8384 [00:00<00:00, 7678.94it/s]
26%|██▌ | Sample : 2187/8384 [00:00<00:00, 7707.54it/s]
28%|██▊ | Sample : 2314/8384 [00:00<00:00, 7725.66it/s]
29%|██▉ | Sample : 2442/8384 [00:00<00:00, 7745.05it/s]
31%|███ | Sample : 2571/8384 [00:00<00:00, 7766.64it/s]
32%|███▏ | Sample : 2701/8384 [00:00<00:00, 7789.71it/s]
34%|███▍ | Sample : 2831/8384 [00:00<00:00, 7809.79it/s]
35%|███▌ | Sample : 2965/8384 [00:00<00:00, 7846.86it/s]
37%|███▋ | Sample : 3099/8384 [00:00<00:00, 7882.23it/s]
39%|███▊ | Sample : 3231/8384 [00:00<00:00, 7904.54it/s]
40%|████ | Sample : 3363/8384 [00:00<00:00, 7927.26it/s]
42%|████▏ | Sample : 3495/8384 [00:00<00:00, 7945.32it/s]
43%|████▎ | Sample : 3625/8384 [00:00<00:00, 7953.76it/s]
45%|████▍ | Sample : 3756/8384 [00:00<00:00, 7966.73it/s]
46%|████▋ | Sample : 3887/8384 [00:00<00:00, 7978.45it/s]
48%|████▊ | Sample : 4016/8384 [00:00<00:00, 7980.42it/s]
49%|████▉ | Sample : 4142/8384 [00:00<00:00, 7973.45it/s]
51%|█████ | Sample : 4268/8384 [00:00<00:00, 7965.48it/s]
52%|█████▏ | Sample : 4392/8384 [00:00<00:00, 7951.63it/s]
54%|█████▍ | Sample : 4519/8384 [00:00<00:00, 7950.54it/s]
55%|█████▌ | Sample : 4648/8384 [00:00<00:00, 7956.57it/s]
57%|█████▋ | Sample : 4779/8384 [00:00<00:00, 7966.58it/s]
59%|█████▊ | Sample : 4909/8384 [00:00<00:00, 7974.83it/s]
60%|██████ | Sample : 5045/8384 [00:00<00:00, 8002.13it/s]
62%|██████▏ | Sample : 5179/8384 [00:00<00:00, 8021.50it/s]
63%|██████▎ | Sample : 5313/8384 [00:00<00:00, 8038.48it/s]
65%|██████▌ | Sample : 5451/8384 [00:00<00:00, 8069.33it/s]
67%|██████▋ | Sample : 5588/8384 [00:00<00:00, 8094.68it/s]
68%|██████▊ | Sample : 5726/8384 [00:00<00:00, 8122.61it/s]
70%|██████▉ | Sample : 5862/8384 [00:00<00:00, 8139.93it/s]
71%|███████▏ | Sample : 5994/8384 [00:00<00:00, 8144.41it/s]
73%|███████▎ | Sample : 6126/8384 [00:00<00:00, 8149.87it/s]
75%|███████▍ | Sample : 6261/8384 [00:00<00:00, 8164.94it/s]
76%|███████▋ | Sample : 6398/8384 [00:00<00:00, 8183.74it/s]
78%|███████▊ | Sample : 6535/8384 [00:00<00:00, 8203.60it/s]
80%|███████▉ | Sample : 6674/8384 [00:00<00:00, 8227.00it/s]
81%|████████ | Sample : 6809/8384 [00:00<00:00, 8235.78it/s]
83%|████████▎ | Sample : 6944/8384 [00:00<00:00, 8246.25it/s]
84%|████████▍ | Sample : 7083/8384 [00:00<00:00, 8269.61it/s]
86%|████████▌ | Sample : 7216/8384 [00:00<00:00, 8271.73it/s]
88%|████████▊ | Sample : 7356/8384 [00:00<00:00, 8293.49it/s]
89%|████████▉ | Sample : 7497/8384 [00:00<00:00, 8317.89it/s]
91%|█████████ | Sample : 7638/8384 [00:00<00:00, 8343.14it/s]
93%|█████████▎| Sample : 7780/8384 [00:00<00:00, 8369.54it/s]
95%|█████████▍| Sample : 7923/8384 [00:00<00:00, 8399.06it/s]
96%|█████████▌| Sample : 8068/8384 [00:00<00:00, 8430.87it/s]
98%|█████████▊| Sample : 8215/8384 [00:01<00:00, 8469.90it/s]
100%|█████████▉| Sample : 8364/8384 [00:01<00:00, 8513.19it/s]
100%|██████████| Sample : 8384/8384 [00:01<00:00, 8127.64it/s]
split 2 / 3
Fitting 1 epochs, 128 channels
0%| | Sample : 0/8384 [00:00<?, ?it/s]
0%| | Sample : 1/8384 [00:00<02:23, 58.42it/s]
2%|▏ | Sample : 130/8384 [00:00<00:02, 4020.15it/s]
3%|▎ | Sample : 261/8384 [00:00<00:01, 5443.78it/s]
5%|▍ | Sample : 392/8384 [00:00<00:01, 6163.68it/s]
6%|▌ | Sample : 523/8384 [00:00<00:01, 6597.05it/s]
8%|▊ | Sample : 657/8384 [00:00<00:01, 6921.96it/s]
9%|▉ | Sample : 787/8384 [00:00<00:01, 7117.45it/s]
11%|█ | Sample : 917/8384 [00:00<00:01, 7262.61it/s]
12%|█▏ | Sample : 1047/8384 [00:00<00:00, 7372.61it/s]
14%|█▍ | Sample : 1178/8384 [00:00<00:00, 7472.98it/s]
16%|█▌ | Sample : 1311/8384 [00:00<00:00, 7564.95it/s]
17%|█▋ | Sample : 1429/8384 [00:00<00:00, 7539.61it/s]
19%|█▊ | Sample : 1557/8384 [00:00<00:00, 7584.13it/s]
20%|██ | Sample : 1687/8384 [00:00<00:00, 7633.02it/s]
22%|██▏ | Sample : 1819/8384 [00:00<00:00, 7685.91it/s]
23%|██▎ | Sample : 1947/8384 [00:00<00:00, 7712.02it/s]
25%|██▍ | Sample : 2077/8384 [00:00<00:00, 7745.26it/s]
26%|██▋ | Sample : 2205/8384 [00:00<00:00, 7765.38it/s]
28%|██▊ | Sample : 2335/8384 [00:00<00:00, 7793.34it/s]
29%|██▉ | Sample : 2464/8384 [00:00<00:00, 7813.06it/s]
31%|███ | Sample : 2596/8384 [00:00<00:00, 7843.34it/s]
33%|███▎ | Sample : 2726/8384 [00:00<00:00, 7861.62it/s]
34%|███▍ | Sample : 2857/8384 [00:00<00:00, 7882.26it/s]
36%|███▌ | Sample : 2985/8384 [00:00<00:00, 7890.16it/s]
37%|███▋ | Sample : 3118/8384 [00:00<00:00, 7915.94it/s]
39%|███▉ | Sample : 3256/8384 [00:00<00:00, 7959.36it/s]
40%|████ | Sample : 3394/8384 [00:00<00:00, 8001.14it/s]
42%|████▏ | Sample : 3531/8384 [00:00<00:00, 8035.50it/s]
44%|████▎ | Sample : 3664/8384 [00:00<00:00, 8052.59it/s]
45%|████▌ | Sample : 3799/8384 [00:00<00:00, 8076.04it/s]
47%|████▋ | Sample : 3935/8384 [00:00<00:00, 8101.67it/s]
49%|████▊ | Sample : 4074/8384 [00:00<00:00, 8135.12it/s]
50%|█████ | Sample : 4206/8384 [00:00<00:00, 8140.60it/s]
52%|█████▏ | Sample : 4343/8384 [00:00<00:00, 8163.20it/s]
53%|█████▎ | Sample : 4481/8384 [00:00<00:00, 8189.28it/s]
55%|█████▌ | Sample : 4617/8384 [00:00<00:00, 8203.89it/s]
57%|█████▋ | Sample : 4752/8384 [00:00<00:00, 8217.56it/s]
58%|█████▊ | Sample : 4888/8384 [00:00<00:00, 8230.46it/s]
60%|█████▉ | Sample : 5019/8384 [00:00<00:00, 8227.79it/s]
61%|██████▏ | Sample : 5150/8384 [00:00<00:00, 8224.31it/s]
63%|██████▎ | Sample : 5282/8384 [00:00<00:00, 8222.91it/s]
65%|██████▍ | Sample : 5421/8384 [00:00<00:00, 8247.47it/s]
66%|██████▋ | Sample : 5557/8384 [00:00<00:00, 8260.03it/s]
68%|██████▊ | Sample : 5690/8384 [00:00<00:00, 8260.56it/s]
70%|██████▉ | Sample : 5827/8384 [00:00<00:00, 8274.98it/s]
71%|███████ | Sample : 5961/8384 [00:00<00:00, 8278.70it/s]
73%|███████▎ | Sample : 6096/8384 [00:00<00:00, 8284.09it/s]
74%|███████▍ | Sample : 6229/8384 [00:00<00:00, 8284.89it/s]
76%|███████▌ | Sample : 6369/8384 [00:00<00:00, 8308.02it/s]
78%|███████▊ | Sample : 6508/8384 [00:00<00:00, 8325.86it/s]
79%|███████▉ | Sample : 6647/8384 [00:00<00:00, 8343.91it/s]
81%|████████ | Sample : 6784/8384 [00:00<00:00, 8354.83it/s]
83%|████████▎ | Sample : 6924/8384 [00:00<00:00, 8374.01it/s]
84%|████████▍ | Sample : 7067/8384 [00:00<00:00, 8400.72it/s]
86%|████████▌ | Sample : 7208/8384 [00:00<00:00, 8422.25it/s]
88%|████████▊ | Sample : 7351/8384 [00:00<00:00, 8449.11it/s]
89%|████████▉ | Sample : 7494/8384 [00:00<00:00, 8474.19it/s]
91%|█████████ | Sample : 7634/8384 [00:00<00:00, 8487.04it/s]
93%|█████████▎| Sample : 7779/8384 [00:00<00:00, 8516.45it/s]
95%|█████████▍| Sample : 7923/8384 [00:00<00:00, 8539.03it/s]
96%|█████████▌| Sample : 8069/8384 [00:00<00:00, 8567.07it/s]
98%|█████████▊| Sample : 8218/8384 [00:00<00:00, 8605.03it/s]
100%|█████████▉| Sample : 8368/8384 [00:01<00:00, 8644.57it/s]
100%|██████████| Sample : 8384/8384 [00:01<00:00, 8262.37it/s]
split 3 / 3
Fitting 1 epochs, 128 channels
0%| | Sample : 0/8384 [00:00<?, ?it/s]
0%| | Sample : 1/8384 [00:00<02:17, 61.13it/s]
2%|▏ | Sample : 133/8384 [00:00<00:01, 4200.73it/s]
3%|▎ | Sample : 264/8384 [00:00<00:01, 5574.54it/s]
5%|▍ | Sample : 392/8384 [00:00<00:01, 6222.74it/s]
6%|▌ | Sample : 519/8384 [00:00<00:01, 6594.49it/s]
8%|▊ | Sample : 651/8384 [00:00<00:01, 6897.64it/s]
9%|▉ | Sample : 780/8384 [00:00<00:01, 7087.68it/s]
11%|█ | Sample : 910/8384 [00:00<00:01, 7240.43it/s]
12%|█▏ | Sample : 1041/8384 [00:00<00:00, 7363.28it/s]
14%|█▍ | Sample : 1172/8384 [00:00<00:00, 7459.76it/s]
16%|█▌ | Sample : 1306/8384 [00:00<00:00, 7564.87it/s]
17%|█▋ | Sample : 1443/8384 [00:00<00:00, 7671.04it/s]
19%|█▉ | Sample : 1580/8384 [00:00<00:00, 7759.19it/s]
20%|██ | Sample : 1709/8384 [00:00<00:00, 7788.61it/s]
22%|██▏ | Sample : 1838/8384 [00:00<00:00, 7812.34it/s]
23%|██▎ | Sample : 1968/8384 [00:00<00:00, 7837.29it/s]
25%|██▌ | Sample : 2099/8384 [00:00<00:00, 7865.43it/s]
27%|██▋ | Sample : 2231/8384 [00:00<00:00, 7895.24it/s]
28%|██▊ | Sample : 2363/8384 [00:00<00:00, 7920.23it/s]
30%|██▉ | Sample : 2498/8384 [00:00<00:00, 7960.04it/s]
31%|███▏ | Sample : 2635/8384 [00:00<00:00, 8003.73it/s]
33%|███▎ | Sample : 2770/8384 [00:00<00:00, 8030.75it/s]
35%|███▍ | Sample : 2903/8384 [00:00<00:00, 8047.39it/s]
36%|███▌ | Sample : 3037/8384 [00:00<00:00, 8070.43it/s]
38%|███▊ | Sample : 3173/8384 [00:00<00:00, 8095.16it/s]
39%|███▉ | Sample : 3310/8384 [00:00<00:00, 8123.72it/s]
41%|████ | Sample : 3444/8384 [00:00<00:00, 8139.97it/s]
43%|████▎ | Sample : 3580/8384 [00:00<00:00, 8163.28it/s]
44%|████▍ | Sample : 3719/8384 [00:00<00:00, 8196.14it/s]
46%|████▌ | Sample : 3861/8384 [00:00<00:00, 8236.19it/s]
48%|████▊ | Sample : 4002/8384 [00:00<00:00, 8270.30it/s]
49%|████▉ | Sample : 4143/8384 [00:00<00:00, 8301.77it/s]
51%|█████ | Sample : 4282/8384 [00:00<00:00, 8324.24it/s]
53%|█████▎ | Sample : 4419/8384 [00:00<00:00, 8336.90it/s]
54%|█████▍ | Sample : 4555/8384 [00:00<00:00, 8345.81it/s]
56%|█████▌ | Sample : 4690/8384 [00:00<00:00, 8350.86it/s]
58%|█████▊ | Sample : 4828/8384 [00:00<00:00, 8364.31it/s]
59%|█████▉ | Sample : 4963/8384 [00:00<00:00, 8366.45it/s]
61%|██████ | Sample : 5099/8384 [00:00<00:00, 8371.39it/s]
62%|██████▏ | Sample : 5231/8384 [00:00<00:00, 8363.12it/s]
64%|██████▍ | Sample : 5366/8384 [00:00<00:00, 8366.70it/s]
66%|██████▌ | Sample : 5502/8384 [00:00<00:00, 8371.82it/s]
67%|██████▋ | Sample : 5634/8384 [00:00<00:00, 8364.95it/s]
69%|██████▉ | Sample : 5769/8384 [00:00<00:00, 8368.32it/s]
70%|███████ | Sample : 5910/8384 [00:00<00:00, 8390.82it/s]
72%|███████▏ | Sample : 6048/8384 [00:00<00:00, 8402.14it/s]
74%|███████▎ | Sample : 6183/8384 [00:00<00:00, 8403.28it/s]
75%|███████▌ | Sample : 6319/8384 [00:00<00:00, 8407.18it/s]
77%|███████▋ | Sample : 6454/8384 [00:00<00:00, 8408.27it/s]
79%|███████▊ | Sample : 6590/8384 [00:00<00:00, 8411.39it/s]
80%|████████ | Sample : 6725/8384 [00:00<00:00, 8412.58it/s]
82%|████████▏ | Sample : 6866/8384 [00:00<00:00, 8432.10it/s]
84%|████████▎ | Sample : 7008/8384 [00:00<00:00, 8452.82it/s]
85%|████████▌ | Sample : 7147/8384 [00:00<00:00, 8465.10it/s]
87%|████████▋ | Sample : 7288/8384 [00:00<00:00, 8483.33it/s]
89%|████████▊ | Sample : 7430/8384 [00:00<00:00, 8503.43it/s]
90%|█████████ | Sample : 7572/8384 [00:00<00:00, 8520.66it/s]
92%|█████████▏| Sample : 7719/8384 [00:00<00:00, 8554.78it/s]
94%|█████████▍| Sample : 7865/8384 [00:00<00:00, 8583.03it/s]
96%|█████████▌| Sample : 8012/8384 [00:00<00:00, 8613.61it/s]
97%|█████████▋| Sample : 8161/8384 [00:00<00:00, 8647.49it/s]
99%|█████████▉| Sample : 8309/8384 [00:00<00:00, 8677.01it/s]
100%|██████████| Sample : 8384/8384 [00:01<00:00, 8353.04it/s]
Visualize stimulus reconstruction#
To get a sense of our model performance, we can plot the actual and predicted stimulus envelopes side by side.
y_pred = sr.predict(Y[test])
time = np.linspace(0, 2.0, 5 * int(sfreq))
fig, ax = plt.subplots(figsize=(8, 4), layout="constrained")
ax.plot(
time, speech[test][sr.valid_samples_][: int(5 * sfreq)], color="grey", lw=2, ls="--"
)
ax.plot(time, y_pred[sr.valid_samples_][: int(5 * sfreq)], color="r", lw=2)
ax.legend([lns[0], ln1[0]], ["Envelope", "Reconstruction"], frameon=False)
ax.set(title="Stimulus reconstruction")
ax.set_xlabel("Time (s)")
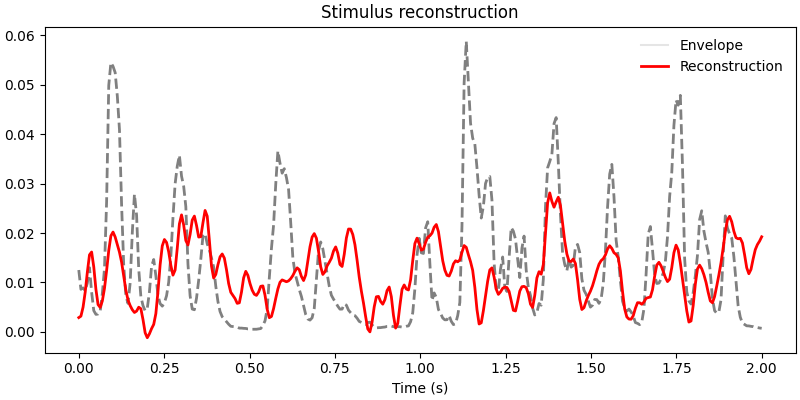
Investigate model coefficients#
Finally, we will look at how the decoding model coefficients are distributed across the scalp. We will attempt to recreate figure 5 from [1]. The decoding model weights reflect the channels that contribute most toward reconstructing the stimulus signal, but are not directly interpretable in a neurophysiological sense. Here we also look at the coefficients obtained via an inversion procedure [2], which have a more straightforward interpretation as their value (and sign) directly relates to the stimulus signal’s strength (and effect direction).
time_plot = (-0.140, -0.125) # To average between two timepoints.
ix_plot = np.arange(
np.argmin(np.abs(time_plot[0] - times)), np.argmin(np.abs(time_plot[1] - times))
)
fig, ax = plt.subplots(1, 2)
mne.viz.plot_topomap(
np.mean(mean_coefs[:, ix_plot], axis=1),
pos=info,
axes=ax[0],
show=False,
vlim=(-max_coef, max_coef),
)
ax[0].set(title=f"Model coefficients\nbetween delays {time_plot[0]} and {time_plot[1]}")
mne.viz.plot_topomap(
np.mean(mean_patterns[:, ix_plot], axis=1),
pos=info,
axes=ax[1],
show=False,
vlim=(-max_patterns, max_patterns),
)
ax[1].set(
title=(
f"Inverse-transformed coefficients\nbetween delays {time_plot[0]} and "
f"{time_plot[1]}"
)
)
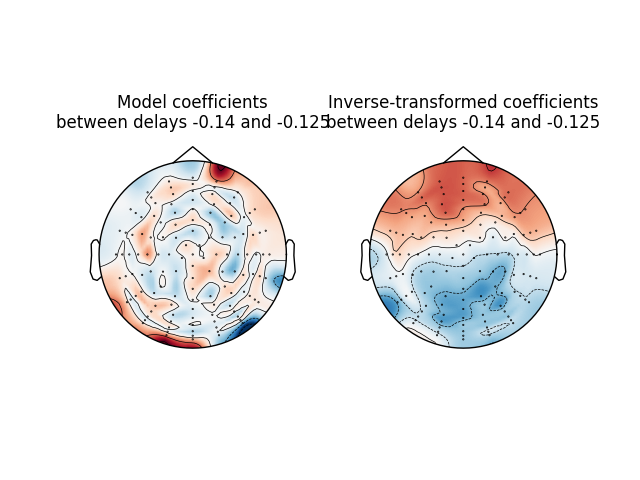
References#
Total running time of the script: (0 minutes 12.010 seconds)